QT系列教程(11) TextEdit实现Qt 文本高亮
文本高亮
对于textedit里录入的部分单词我们可以实现高亮,实现高亮主要依赖于QSyntaxHighlighter。
我们先创建一个Qt Application类,类名MainWindow, 然后新增一个C++类,类名为MySyntaxHighlighter。
#ifndef MYSYNTAXHIGHLIGHTER_H
#define MYSYNTAXHIGHLIGHTER_H
#include <QSyntaxHighlighter>
#include <QTextDocument>
class MySyntaxHighlighter:public QSyntaxHighlighter
{Q_OBJECT
public:explicit MySyntaxHighlighter(QTextDocument* parent = 0);//重写实现高亮
protected:void highlightBlock(const QString& text);
};#endif // MYSYNTAXHIGHLIGHTER_H
这个类声明了highlightBlock函数,这是一个虚函数继承自QSyntaxHighlighter。每次我们录入文字时,会自动调用这个函数。下面实现MySyntaxHighlighter类
#include "mysyntaxhighlighter.h"
#include <QFont>MySyntaxHighlighter::MySyntaxHighlighter(QTextDocument* parent):QSyntaxHighlighter (parent)
{}void MySyntaxHighlighter::highlightBlock(const QString &text)
{QTextCharFormat myFormat;myFormat.setFont(QFont("微软雅黑"));myFormat.setFontWeight(QFont::Bold);myFormat.setForeground(Qt::green);//匹配charQString pattern = "\\bchar\\b";//创建正则表达式QRegExp express(pattern);//从索引0的位置开始匹配int index = text.indexOf(express);while (index>0) {int matchLen = express.matchedLength();//对匹配的字符串设置高亮setFormat(index, matchLen, myFormat);index = text.indexOf(express, index+matchLen);}
}
在highlightBlock函数中,我们实现了一个高亮的文字模式,当录入的字符串包含char时,char会被高亮。
我们在mainwindow.ui中添加一个textedit,然后在mainwindow的构造函数中添加我们刚才编写的高亮模块。
MainWindow::MainWindow(QWidget *parent) :QMainWindow(parent),ui(new Ui::MainWindow)
{ui->setupUi(this);m_ligher = new MySyntaxHighlighter(ui->textEdit->document());
}
程序运行后,在编辑器中输入hello char,会看到char高亮了
实现代码编辑器
Qt 的案例中有提供过文本高亮和显示行号的demo,我把它整理起来了。
我们先声明codeeditor类,以及行号显示的类
#ifndef CODEEDITOR_H
#define CODEEDITOR_H#include <QPlainTextEdit>QT_BEGIN_NAMESPACE
class QPaintEvent;
class QResizeEvent;
class QSize;
class QWidget;
QT_END_NAMESPACEclass LineNumberArea;class CodeEditor : public QPlainTextEdit
{Q_OBJECTpublic:CodeEditor(QWidget *parent = nullptr);void lineNumberAreaPaintEvent(QPaintEvent *event);int lineNumberAreaWidth();protected:void resizeEvent(QResizeEvent *event) override;private slots:void updateLineNumberAreaWidth(int newBlockCount);void highlightCurrentLine();void updateLineNumberArea(const QRect &rect, int dy);private:QWidget *lineNumberArea;
};class LineNumberArea : public QWidget
{
public:LineNumberArea(CodeEditor *editor) : QWidget(editor), codeEditor(editor){}QSize sizeHint() const override{return QSize(codeEditor->lineNumberAreaWidth(), 0);}protected:void paintEvent(QPaintEvent *event) override{codeEditor->lineNumberAreaPaintEvent(event);}private:CodeEditor *codeEditor;
};#endif
具体代码类的实现
#include "codeeditor.h"#include <QPainter>
#include <QTextBlock>CodeEditor::CodeEditor(QWidget *parent) : QPlainTextEdit(parent)
{lineNumberArea = new LineNumberArea(this);connect(this, &CodeEditor::blockCountChanged, this, &CodeEditor::updateLineNumberAreaWidth);connect(this, &CodeEditor::updateRequest, this, &CodeEditor::updateLineNumberArea);connect(this, &CodeEditor::cursorPositionChanged, this, &CodeEditor::highlightCurrentLine);updateLineNumberAreaWidth(0);highlightCurrentLine();
}int CodeEditor::lineNumberAreaWidth()
{int digits = 1;int max = qMax(1, blockCount());while (max >= 10) {max /= 10;++digits;}int space = 3 + fontMetrics().horizontalAdvance(QLatin1Char('9')) * digits;return space;
}void CodeEditor::updateLineNumberAreaWidth(int /* newBlockCount */)
{setViewportMargins(lineNumberAreaWidth(), 0, 0, 0);
}void CodeEditor::updateLineNumberArea(const QRect &rect, int dy)
{if (dy)lineNumberArea->scroll(0, dy);elselineNumberArea->update(0, rect.y(), lineNumberArea->width(), rect.height());if (rect.contains(viewport()->rect()))updateLineNumberAreaWidth(0);
}void CodeEditor::resizeEvent(QResizeEvent *e)
{QPlainTextEdit::resizeEvent(e);QRect cr = contentsRect();lineNumberArea->setGeometry(QRect(cr.left(), cr.top(), lineNumberAreaWidth(), cr.height()));
}void CodeEditor::highlightCurrentLine()
{QList<QTextEdit::ExtraSelection> extraSelections;if (!isReadOnly()) {QTextEdit::ExtraSelection selection;QColor lineColor = QColor(Qt::yellow).lighter(160);selection.format.setBackground(lineColor);selection.format.setProperty(QTextFormat::FullWidthSelection, true);selection.cursor = textCursor();selection.cursor.clearSelection();extraSelections.append(selection);}setExtraSelections(extraSelections);
}void CodeEditor::lineNumberAreaPaintEvent(QPaintEvent *event)
{QPainter painter(lineNumberArea);painter.fillRect(event->rect(), Qt::lightGray);QTextBlock block = firstVisibleBlock();int blockNumber = block.blockNumber();int top = qRound(blockBoundingGeometry(block).translated(contentOffset()).top());int bottom = top + qRound(blockBoundingRect(block).height());while (block.isValid() && top <= event->rect().bottom()) {if (block.isVisible() && bottom >= event->rect().top()) {QString number = QString::number(blockNumber + 1);painter.setPen(Qt::black);painter.drawText(0, top, lineNumberArea->width(), fontMetrics().height(),Qt::AlignHCenter, number);}block = block.next();top = bottom;bottom = top + qRound(blockBoundingRect(block).height());++blockNumber;}
}
上面实现了代码编辑器的行号和当前行黄色高亮显示。
接下来实现高亮显示类
#ifndef HIGHLIGHTER_H
#define HIGHLIGHTER_H#include <QSyntaxHighlighter>
#include <QTextCharFormat>
#include <QRegularExpression>QT_BEGIN_NAMESPACE
class QTextDocument;
QT_END_NAMESPACE//! [0]
class Highlighter : public QSyntaxHighlighter
{Q_OBJECTpublic:Highlighter(QTextDocument *parent = 0);protected:void highlightBlock(const QString &text) override;private:struct HighlightingRule{QRegularExpression pattern;QTextCharFormat format;};QVector<HighlightingRule> highlightingRules;QRegularExpression commentStartExpression;QRegularExpression commentEndExpression;QTextCharFormat keywordFormat;QTextCharFormat classFormat;QTextCharFormat singleLineCommentFormat;QTextCharFormat multiLineCommentFormat;QTextCharFormat quotationFormat;QTextCharFormat functionFormat;
};
//! [0]#endif // HIGHLIGHTER_H
具体实现细节如下,先定义高亮的正则规则,然后在highlightBlock函数里根据规则点亮不同的单词
#include "highlighter.h"//! [0]
Highlighter::Highlighter(QTextDocument *parent): QSyntaxHighlighter(parent)
{HighlightingRule rule;keywordFormat.setForeground(Qt::darkBlue);keywordFormat.setFontWeight(QFont::Bold);const QString keywordPatterns[] = {QStringLiteral("\\bchar\\b"), QStringLiteral("\\bclass\\b"), QStringLiteral("\\bconst\\b"),QStringLiteral("\\bdouble\\b"), QStringLiteral("\\benum\\b"), QStringLiteral("\\bexplicit\\b"),QStringLiteral("\\bfriend\\b"), QStringLiteral("\\binline\\b"), QStringLiteral("\\bint\\b"),QStringLiteral("\\blong\\b"), QStringLiteral("\\bnamespace\\b"), QStringLiteral("\\boperator\\b"),QStringLiteral("\\bprivate\\b"), QStringLiteral("\\bprotected\\b"), QStringLiteral("\\bpublic\\b"),QStringLiteral("\\bshort\\b"), QStringLiteral("\\bsignals\\b"), QStringLiteral("\\bsigned\\b"),QStringLiteral("\\bslots\\b"), QStringLiteral("\\bstatic\\b"), QStringLiteral("\\bstruct\\b"),QStringLiteral("\\btemplate\\b"), QStringLiteral("\\btypedef\\b"), QStringLiteral("\\btypename\\b"),QStringLiteral("\\bunion\\b"), QStringLiteral("\\bunsigned\\b"), QStringLiteral("\\bvirtual\\b"),QStringLiteral("\\bvoid\\b"), QStringLiteral("\\bvolatile\\b"), QStringLiteral("\\bbool\\b")};for (const QString &pattern : keywordPatterns) {rule.pattern = QRegularExpression(pattern);rule.format = keywordFormat;highlightingRules.append(rule);
//! [0] //! [1]}
//! [1]//! [2]classFormat.setFontWeight(QFont::Bold);classFormat.setForeground(Qt::darkMagenta);rule.pattern = QRegularExpression(QStringLiteral("\\bQ[A-Za-z]+\\b"));rule.format = classFormat;highlightingRules.append(rule);
//! [2]//! [3]singleLineCommentFormat.setForeground(Qt::red);rule.pattern = QRegularExpression(QStringLiteral("//[^\n]*"));rule.format = singleLineCommentFormat;highlightingRules.append(rule);multiLineCommentFormat.setForeground(Qt::red);
//! [3]//! [4]quotationFormat.setForeground(Qt::darkGreen);rule.pattern = QRegularExpression(QStringLiteral("\".*\""));rule.format = quotationFormat;highlightingRules.append(rule);
//! [4]//! [5]functionFormat.setFontItalic(true);functionFormat.setForeground(Qt::blue);rule.pattern = QRegularExpression(QStringLiteral("\\b[A-Za-z0-9_]+(?=\\()"));rule.format = functionFormat;highlightingRules.append(rule);
//! [5]//! [6]commentStartExpression = QRegularExpression(QStringLiteral(" /\\*"));commentEndExpression = QRegularExpression(QStringLiteral("\\*/"));
}
//! [6]//! [7]
void Highlighter::highlightBlock(const QString &text)
{for (const HighlightingRule &rule : qAsConst(highlightingRules)) {QRegularExpressionMatchIterator matchIterator = rule.pattern.globalMatch(text);while (matchIterator.hasNext()) {QRegularExpressionMatch match = matchIterator.next();setFormat(match.capturedStart(), match.capturedLength(), rule.format);}}
//! [7] //! [8]setCurrentBlockState(0);
//! [8]//! [9]int startIndex = 0;if (previousBlockState() != 1)startIndex = text.indexOf(commentStartExpression);//! [9] //! [10]while (startIndex >= 0) {
//! [10] //! [11]QRegularExpressionMatch match = commentEndExpression.match(text, startIndex);int endIndex = match.capturedStart();int commentLength = 0;if (endIndex == -1) {setCurrentBlockState(1);commentLength = text.length() - startIndex;} else {commentLength = endIndex - startIndex+ match.capturedLength();}setFormat(startIndex, commentLength, multiLineCommentFormat);startIndex = text.indexOf(commentStartExpression, startIndex + commentLength);}
}
//! [11]
接下来在MainWindow里添加editor
void MainWindow::setupEditor()
{QFont font;font.setFamily("Courier");font.setFixedPitch(true);font.setPointSize(10);editor = new CodeEditor();editor->setFont(font);highlighter = new Highlighter(editor->document());QFile file("mainwindow.h");if (file.open(QFile::ReadOnly | QFile::Text))editor->setPlainText(file.readAll());
}
运行程序后,输入部分代码显示如下
具体细节大家可以参考代码理解即可。
总结
源码链接https://gitee.com/secondtonone1/qt-learning-notes
相关文章:
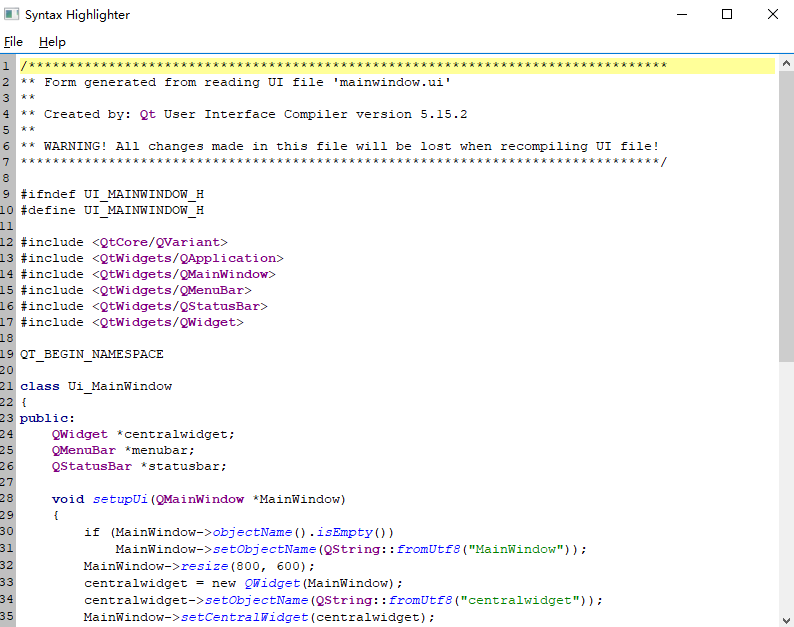
QT系列教程(11) TextEdit实现Qt 文本高亮
文本高亮 对于textedit里录入的部分单词我们可以实现高亮,实现高亮主要依赖于QSyntaxHighlighter。 我们先创建一个Qt Application类,类名MainWindow, 然后新增一个C类,类名为MySyntaxHighlighter。 #ifndef MYSYNTAXHIGHLIGHTER_H #define …...
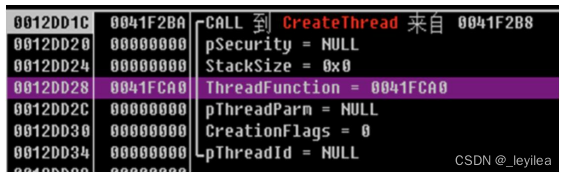
蓝队-溯源技巧
溯源技巧 大致思想 通常情况下,接到溯源任务时,获得的信息如下 攻击时间 攻击 IP 预警平台 攻击类型 恶意文件 受攻击域名/IP其中攻击 IP、攻击类型、恶意文件、攻击详情是溯源入手的点。 通过攻击类型分析攻击详情的请求包,看有没有攻击者…...

【5】JDK、JRE和JVM的区别与联系
JDK、JRE和JVM的区别与联系 Java是一种广泛使用的编程语言,它的跨平台特性得益于Java虚拟机(JVM)。然而,在Java的世界里,JDK、JRE和JVM这三个术语常常让人感到困惑。本文将阐述它们各自的功能,以及它们是如…...

【DevOps】Logstash详解:高效日志管理与分析工具
在现代软件开发和运维过程中,日志管理与分析是至关重要的环节。日志可以帮助我们追踪系统行为、诊断问题、优化性能以及确保安全合规。Logstash,作为ELK Stack(Elasticsearch、Logstash、Kibana)的核心组件之一,是一个…...

Vue3 之 Pinia 核心概念(八)
核心概念 State:这是你的应用程序的状态,是一个响应式的对象。 Getters:类似于 Vuex 中的 getters,它们是基于 state 的计算属性。 Actions:类似于 Vuex 中的 mutations 和 actions,它们用于改变 state。但…...
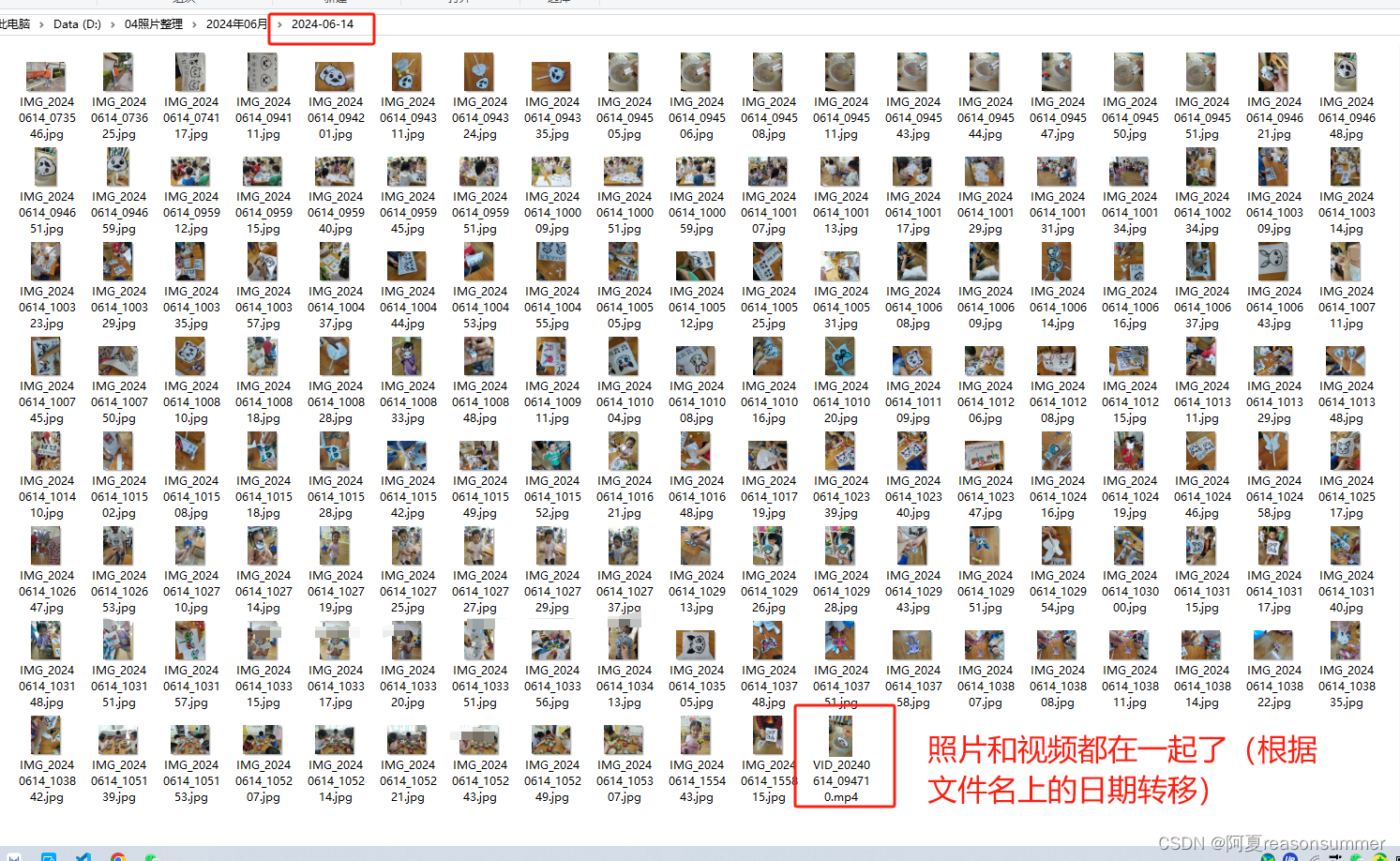
【办公类-04-03】华为助手导出照片视频分类(根据图片、视频的文件名日期分类导出)
背景需求: 用华为手机助手导出的照片视频,只能将jpg照片(exifread读取图片的exif拍摄日期,Png、JPEG、mp4都无法识别到exif信息) 【办公类-04-02】华为助手导出照片(jpg)读取拍摄时间分类导出…...
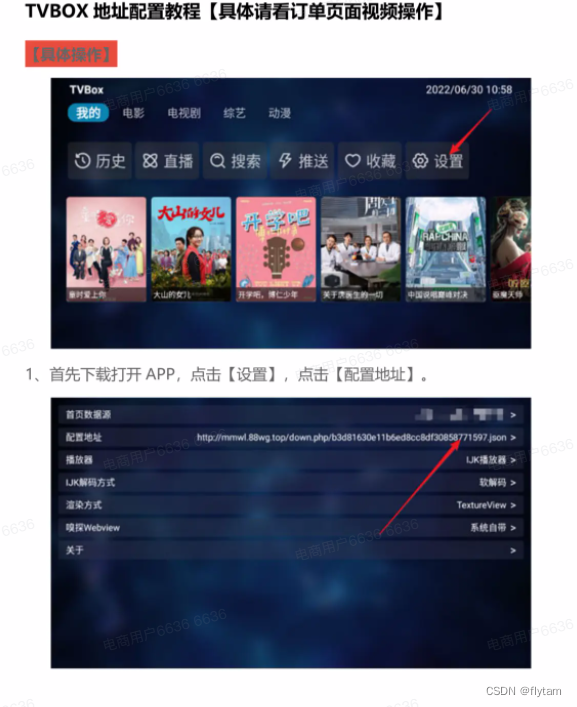
TVBOX 最新版下载+视频源教程
下载链接 wx 搜索 Geek 前端 发送电视资源进行获取 操作教程...
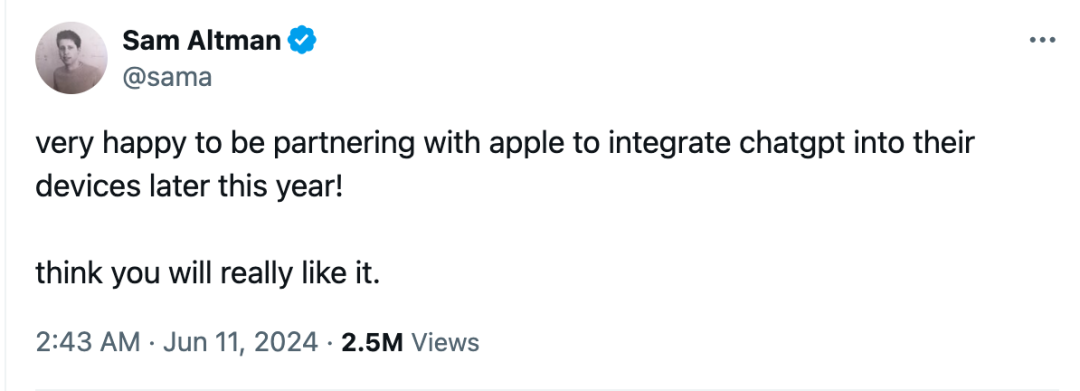
2024年了,苹果可以通话录音了
人不走空 🌈个人主页:人不走空 💖系列专栏:算法专题 ⏰诗词歌赋:斯是陋室,惟吾德馨 6月11日凌晨,苹果在WWDC24大会上,密集输出了酝酿多时的AI应用更新。苹果对通话、对话、图…...
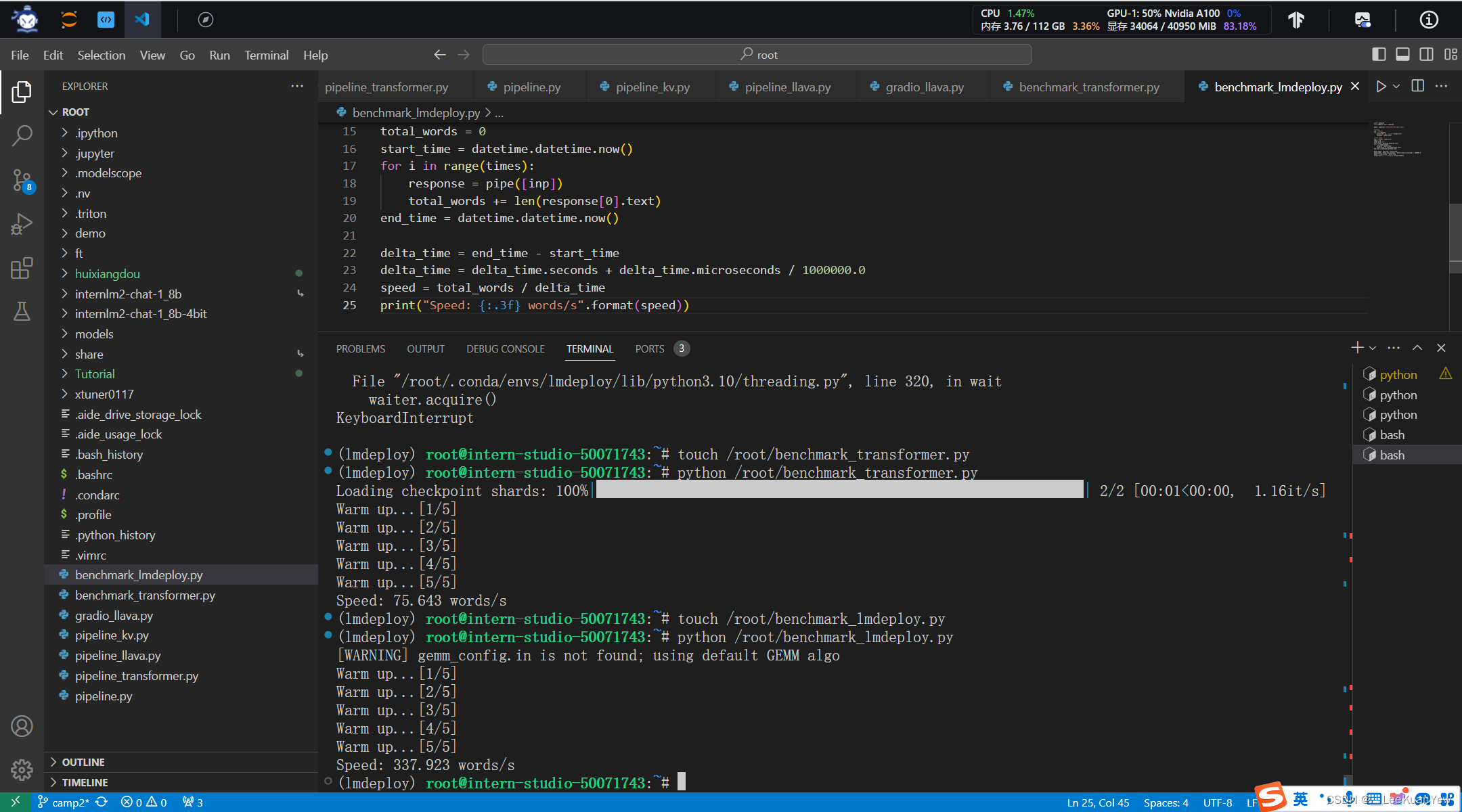
书生·浦语大模型实战营第二期作业五
1、开发机创建conda环境: 2、安装第三方库: 3、新建pipeline_transformer.py文件,并运行: 4、运行结果: 5、执行模型: 6、与大模型进行对话: 7、默认占有的显存: 8、--cache-max-en…...
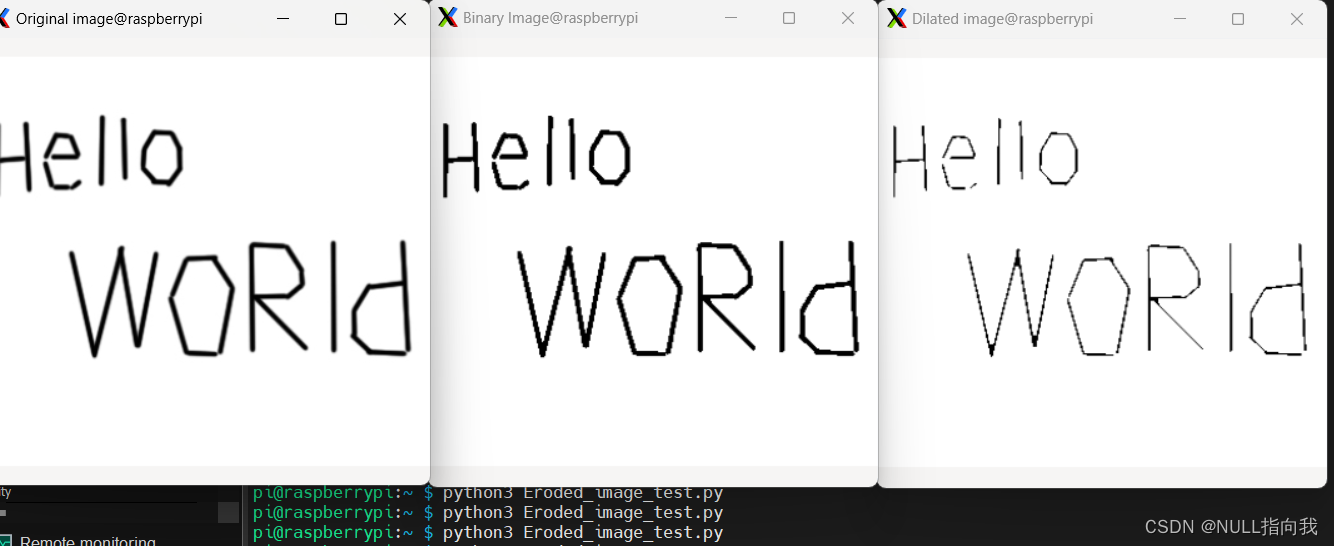
树莓派4B_OpenCv学习笔记9:图片的腐蚀与膨胀
今日继续学习树莓派4B 4G:(Raspberry Pi,简称RPi或RasPi) 本人所用树莓派4B 装载的系统与版本如下: 版本可用命令 (lsb_release -a) 查询: Opencv 版本是4.5.1: 图像的膨胀与腐蚀一般用于灰度图或者二值图,今日便来学习…...
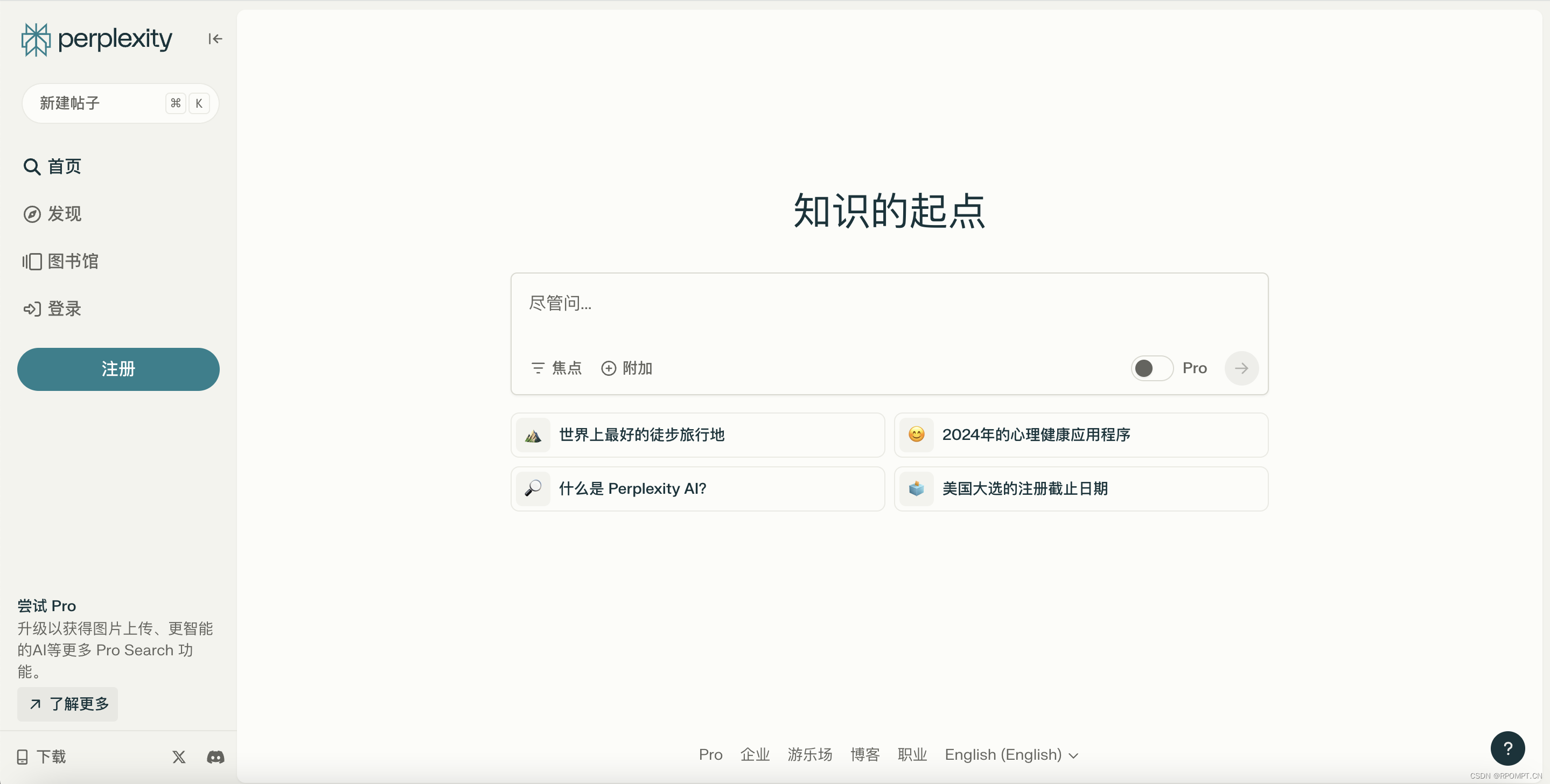
Perplexity AI — 探索网络,发掘知识,沟通思想
体验地址:Perplexity AI (国外网站访问需要梯子) Perplexity AI是一款功能强大的人工智能搜索引擎,其特点和优势主要体现在以下几个方面: 功能: 自然语言搜索:Perplexity AI可以理解用户的自然…...
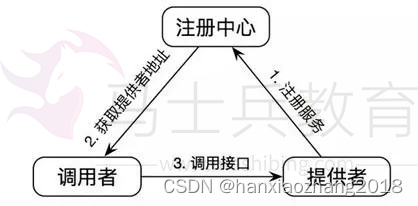
RPC知识
一、为什么要有RPC: HTTP协议的接口,在接口不多、系统与系统交互较少的情况下,解决信息孤岛初期常使用的一种通信手段;优点就是简单、直接、开发方便,利用现成的HTTP协议进行传输。 但是,如果是一个大型的网…...
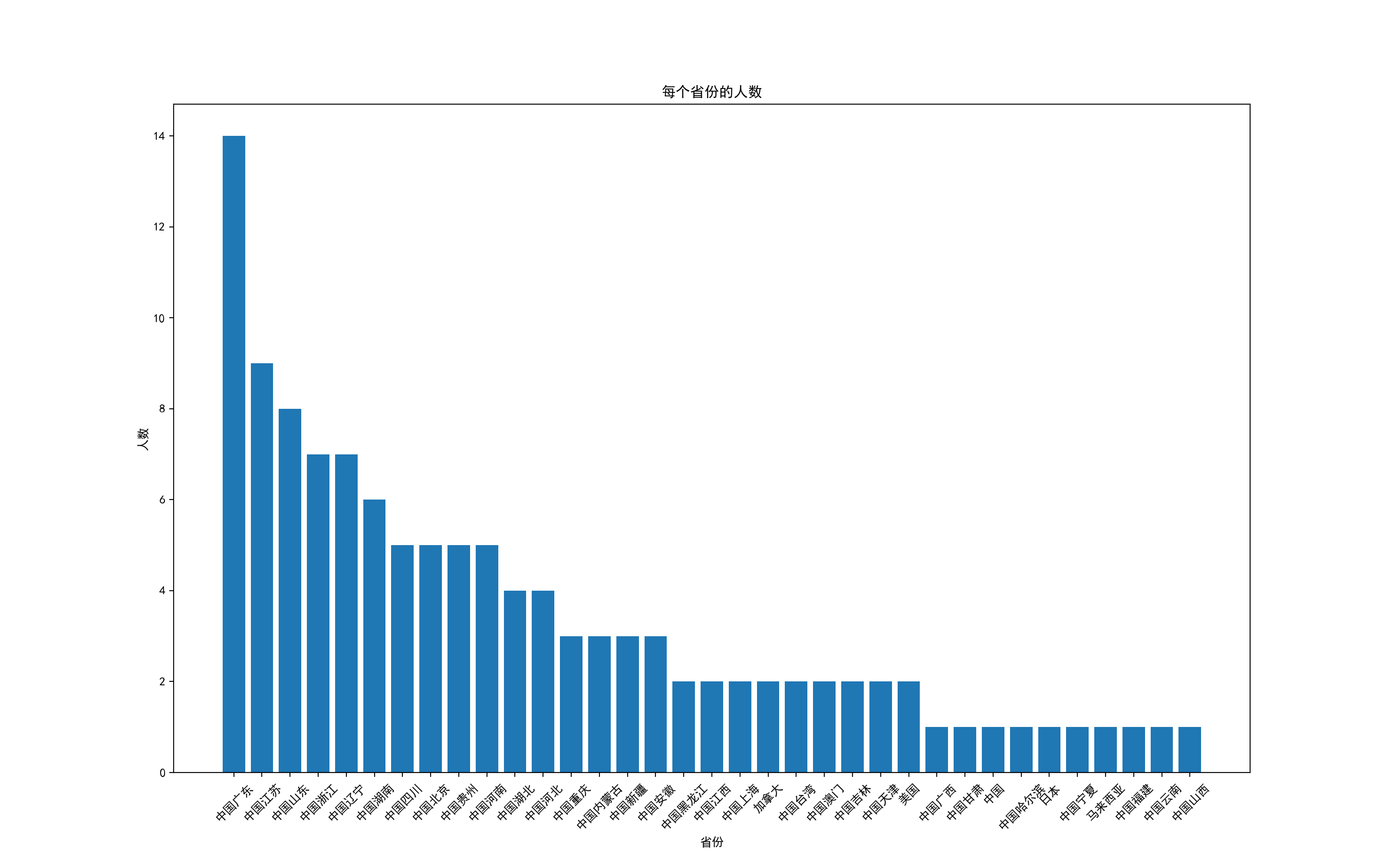
【爬虫】requests 结合 BeautifulSoup抓取网页数据
一、BeautifulSoup使用步骤 BeautifulSoup 是一个用于从 HTML 或 XML 文件中提取数据的 Python 库。以下是如何使用 BeautifulSoup 来解析 HTML 并提取信息的基本步骤: 1、安装: 如果你还没有安装 BeautifulSoup,你可以使用 pip 来安装它。…...

安全测试框架 二
使用安全测试框架进行测试,可以遵循以下步骤进行,以确保测试的全面性和系统性: 一、明确测试目标和需求 确定测试的范围和重点,明确要测试的系统或应用的安全性方面的关键点和重要性。根据业务需求和安全标准,制定详…...

安徽京准-NTP网络授时服务器助力助力甘南州公共资源交易
安徽京准-NTP网络授时服务器助力助力甘南州公共资源交易 安徽京准-NTP网络授时服务器助力助力甘南州公共资源交易 2024年5月中旬,我安徽京准科技生产研发的NTP时钟服务器成功投运甘南州公共资源交易中心,为该中心的计算机网络系统及其他各业务子系统提供…...

大数据—什么是大数据?
大数据是指所涉及的资料量规模巨大到无法透过主流软件工具,在合理时间内达到撷取、管理、处理、并整理成为帮助企业经营决策更积极目的的资讯。想要更加全面地了解大数据的概念,可以从以下几个维度进行介绍: 大数据的定义: 基本…...

德克萨斯大学奥斯汀分校自然语言处理硕士课程汉化版(第十一周) - 自然语言处理扩展研究
自然语言处理扩展研究 1. 多语言研究2. 语言锚定3. 伦理问题 1. 多语言研究 多语言(Multilinguality)是NLP的一个重要研究方向,旨在开发能够处理多种语言的模型和算法。由于不同语言在语法、词汇和语义结构上存在差异,这成为一个复杂且具有挑战性的研究…...

支持向量机(SVM)中核函数的本质意义
本质上在做什么? 内积是距离度量,核函数相当于将低维空间的距离映射到高维空间的距离,并非对特征直接映射。 为什么要求核函数是对称且Gram矩阵是半正定? 核函数对应某一特征空间的内积,要求①核函数对称;②…...
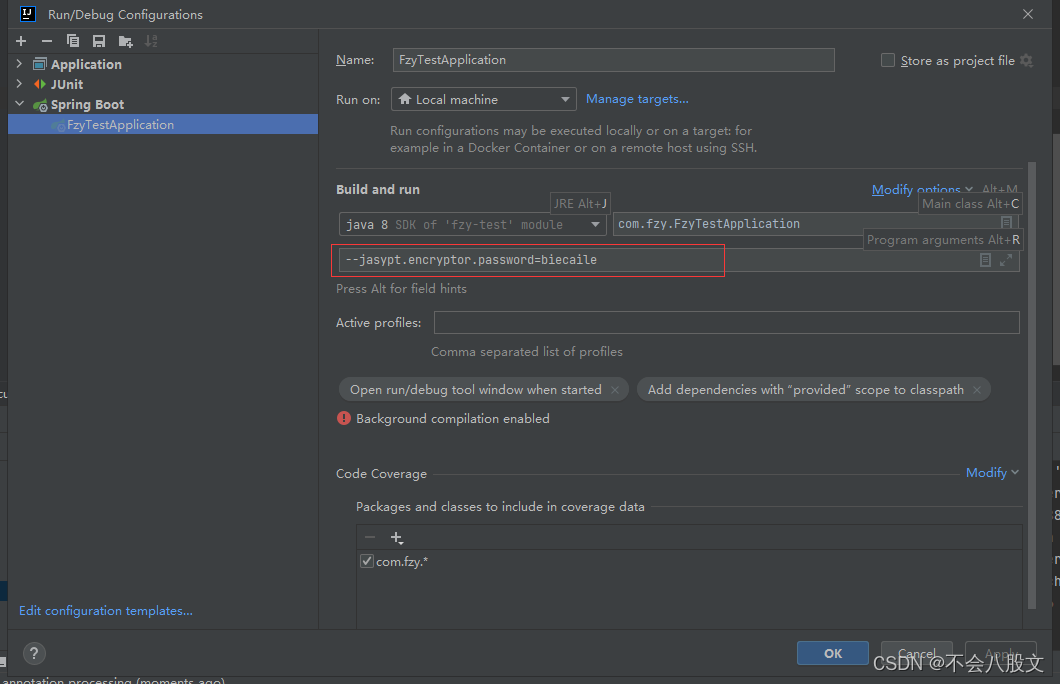
SpringBoot使用jasypt实现数据库信息的脱敏,以此来保护数据库的用户名username和密码password(容易上手,详细)
1.为什么要有这个需求? 一般当我们自己练习的时候,username和password直接是爆露出来的 假如别人路过你旁边时看到了你的数据库账号密码,他跑到他的电脑打开navicat直接就是一顿连接,直接疯狂删除你的数据库,那可就废…...

Python日志配置策略
1 三种情况下都能实现日志打印: 被库 A 调用,使用库 A 的日志配置。被库 B 调用,使用库 B 的日志配置。独立运行,使用自己的日志配置。 需要实现一个灵活的日志配置策略,使得日志记录器可以根据调用者或运行环境自动…...
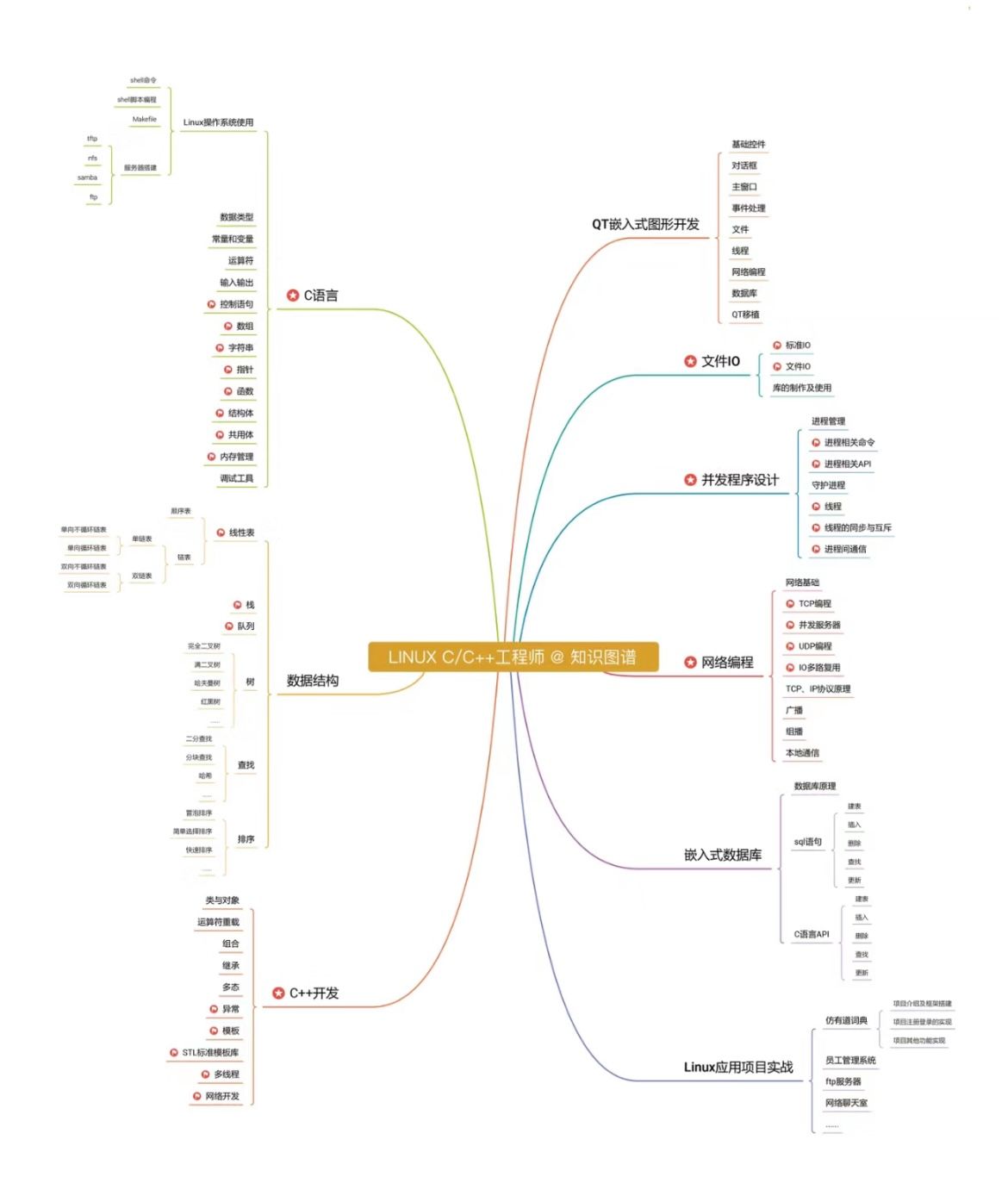
想学编程,什么语言最好上手?
Python是许多初学者的首选,因为它的语法简洁易懂,而且有丰富的资源和社区支持。我这里有一套编程入门教程,不仅包含了详细的视频 讲解,项目实战。如果你渴望学习编程,不妨点个关注,给个评论222,…...

binlog和redolog有什么区别
在数据库管理系统中,binlog(binary log)和 redolog(redo log)是两种重要的日志机制,它们在数据持久性和故障恢复方面扮演着关键角色。虽然它们都用于记录数据库的变化,但它们的目的和使用方式有…...
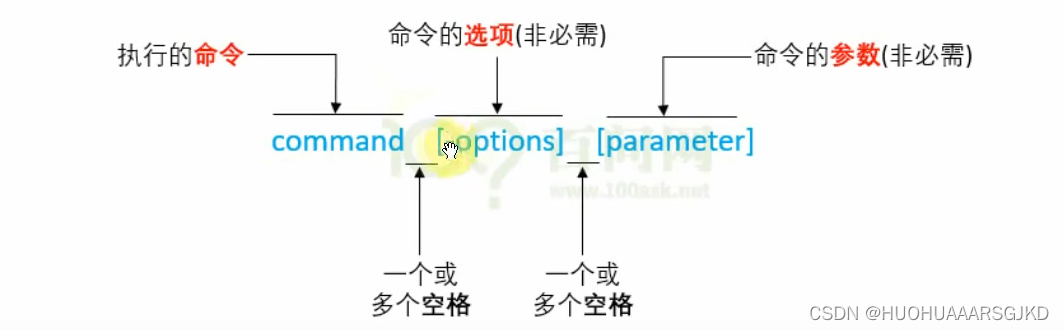
Linux笔记--ubuntu文件目录+命令行介绍
文件目录 命令行介绍 当我们在ubuntu中命令行处理位置输入ls后会显示出其所有目录,那么处理这些命令的程序就是shell,它负责接收用户的输入,并根据输入找到其他程序并运行 命令行格式 linux的命令一般由三部分组成:command命令、…...

71、最长上升子序列II
最长上升子序列II 题目描述 给定一个长度为N的数列,求数值严格单调递增的子序列的长度最长是多少。 输入格式 第一行包含整数N。 第二行包含N个整数,表示完整序列。 输出格式 输出一个整数,表示最大长度。 数据范围 1 ≤ N ≤ 100000…...
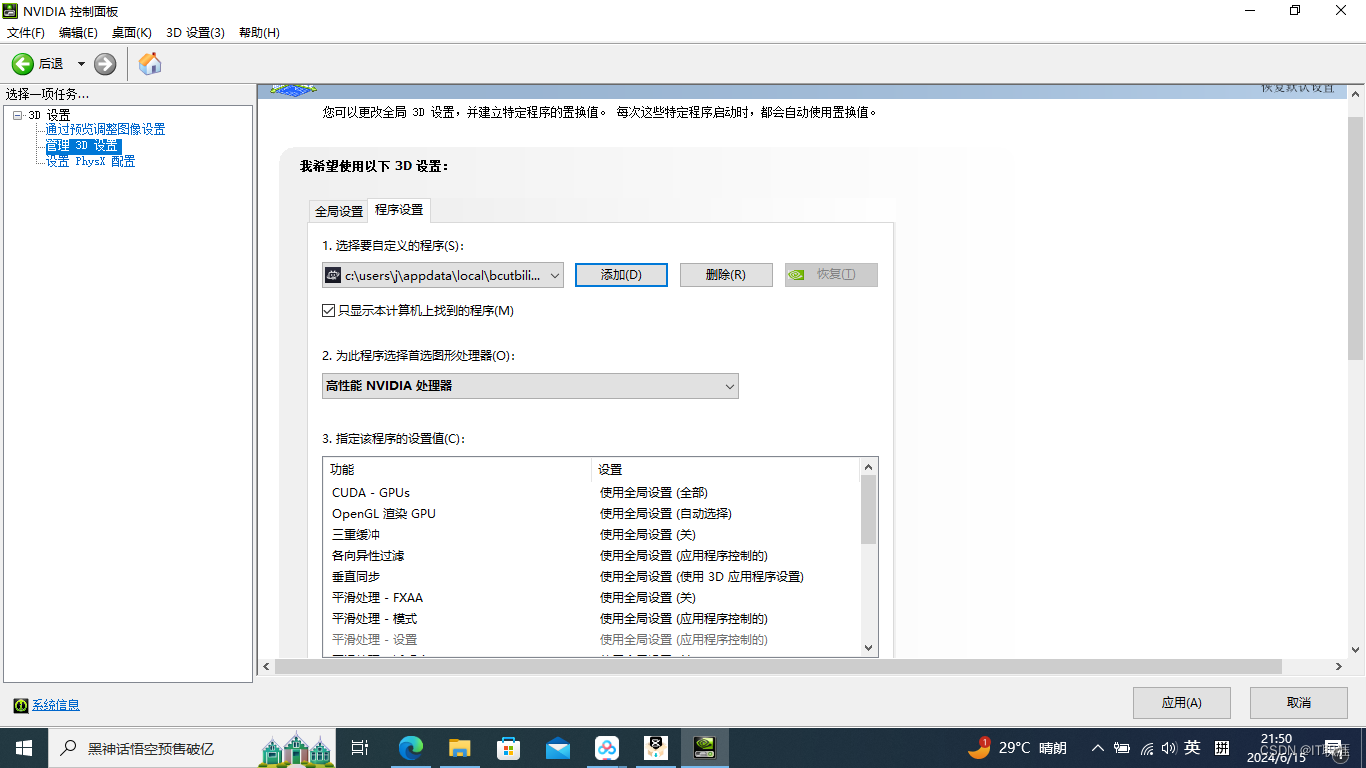
解决必剪电脑版导出视频缺斤少两的办法
背景 前几天将电脑重置了,今天想要剪辑一下视频,于是下载了必剪,将视频、音频都调整好,导出,结果15分钟的视频只能导出很短的时长,调整参数最多也只能导出10分钟,My God! 解决 首…...

新人学习笔记之(常量)
一、什么是常量 1.常量:在程序的执行过程中,其值不能发生改变的数据 二、常量的分类 常量类型说明举例整型常量整数、负数、0123 456实型常量所有带小数点的数字1.93 18.2字符常量单引号引起来的字母、数字、英文符号S B字符串常量双引号引起来的&…...
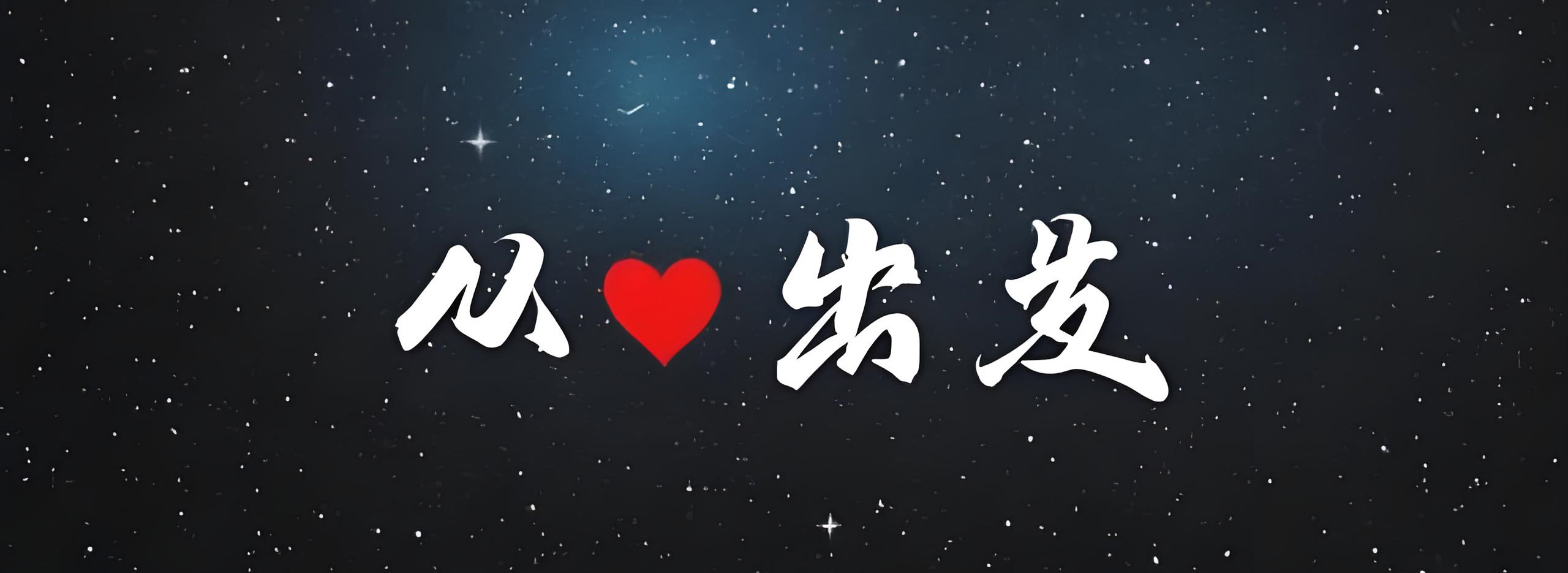
Lua解释器裁剪
本文目录 1、引言2、文件功能3、选择需要初始化的库4、结论 文章对应视频教程: 已更新。见下方 点击图片或链接访问我的B站主页~~~ Lua解释器裁剪,很简单~ 1、引言 在嵌入式中使用lua解释器,很多时候会面临资源紧张的情况。 同时,…...

web前端设计nav:深入探索导航栏设计的艺术与技术
web前端设计nav:深入探索导航栏设计的艺术与技术 在web前端设计中,导航栏(nav)扮演着至关重要的角色,它不仅是用户浏览网站的指引,更是网站整体设计的点睛之笔。本文将从四个方面、五个方面、六个方面和七…...

分析解读NCCL_SHM_Disable与NCCL_P2P_Disable
在NVIDIA的NCCL(NVIDIA Collective Communications Library)库中,NCCL_SHM_Disable 和 NCCL_P2P_Disable 是两个重要的环境变量,它们控制着NCCL在多GPU通信中的行为和使用的通信机制。下面是对这两个环境变量的详细解读࿱…...
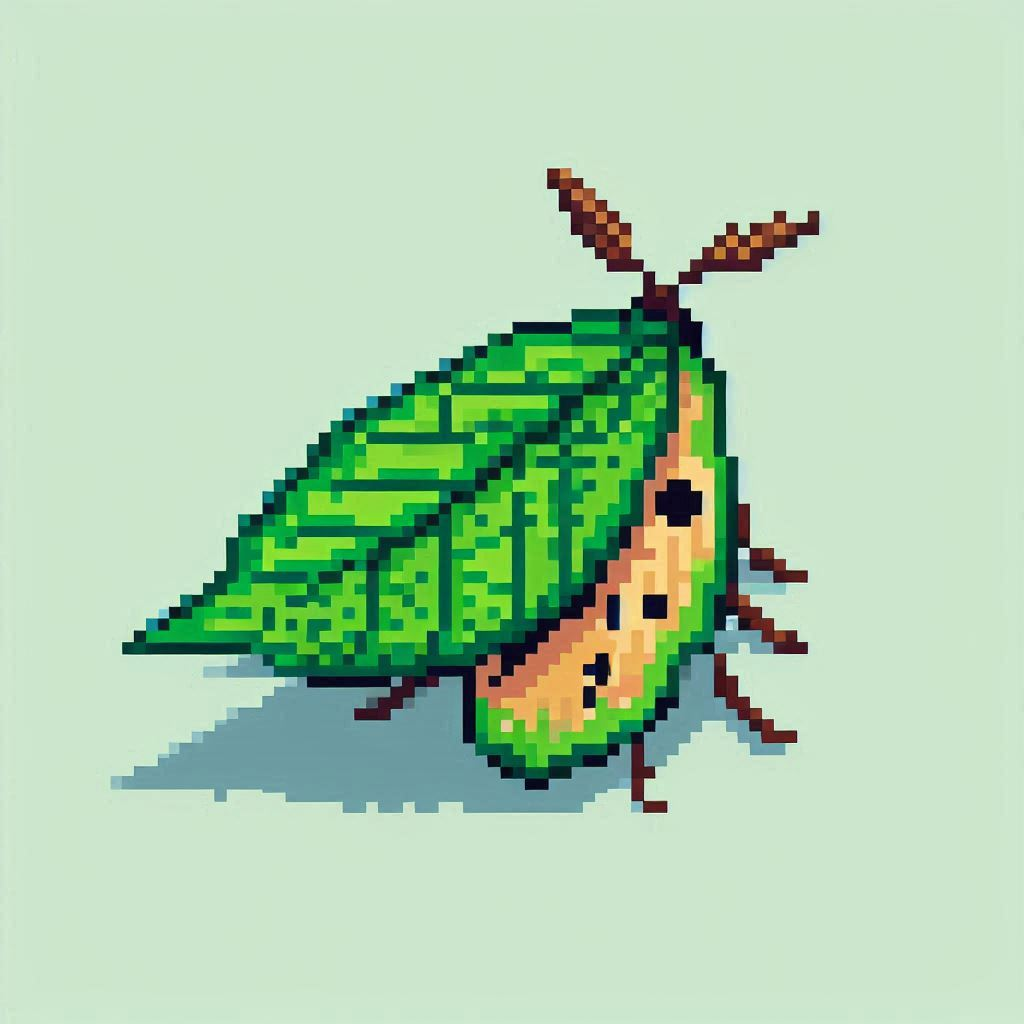
使用 Python 进行测试(6)Fake it...
总结 如果我有: # my_life_work.py def transform(param):return param * 2def check(param):return "bad" not in paramdef calculate(param):return len(param)def main(param, option):if option:param transform(param)if not check(param):raise ValueError(…...