东莞建站/seo查询 站长之家
一、Vue3 基础
1.1 创建 Vue3 项目
- 使用 Vite 创建
npm create vite@latest my-vue-app -- --template vue
cd my-vue-app
npm install
npm run dev
- 使用 Vue CLI 创建
npm install -g @vue/cli
vue create my-vue-app
1.2 项目结构
my-vue-app
├── node_modules
├── public
│ └── favicon.ico
├── src
│ ├── assets
│ ├── components
│ ├── views
│ ├── App.vue
│ ├── main.js
│ └── router
├── .gitignore
├── index.html
├── package.json
├── vite.config.js
└── README.md
1.3 main.js 配置
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
import store from './store'const app = createApp(App)app.use(router)
app.use(store)app.mount('#app')
二、组件
2.1 组件注册
- 全局注册
import { createApp } from 'vue'
import MyComponent from './components/MyComponent.vue'const app = createApp(App)
app.component('MyComponent', MyComponent)
app.mount('#app')
- 局部注册
<template><MyComponent />
</template><script>
import MyComponent from './components/MyComponent.vue'export default {components: {MyComponent}
}
</script>
2.2 组件通信
- 父传子: props
// 父组件
<template><ChildComponent :message="parentMessage" />
</template><script>
import ChildComponent from './ChildComponent.vue'export default {components: { ChildComponent },data() {return {parentMessage: 'Hello from parent'}}
}
</script>
// 子组件
<template><div>{{ message }}</div>
</template><script>
export default {props: {message: {type: String,required: true}}
}
</script>
- 子传父: emit
// 子组件
<template><button @click="sendMessage">Send Message</button>
</template><script>
export default {emits: ['message'],methods: {sendMessage() {this.$emit('message', 'Hello from child')}}
}
</script>
// 父组件
<template><ChildComponent @message="handleMessage" />
</template><script>
import ChildComponent from './ChildComponent.vue'export default {components: { ChildComponent },methods: {handleMessage(message) {console.log(message)}}
}
</script>
- 兄弟组件通信: EventBus
// event-bus.js
import { EventEmitter } from 'vue'
export const EventBus = new EventEmitter()
// 组件 A
<template><button @click="sendMessage">Send Message</button>
</template><script>
import { EventBus } from './event-bus'export default {methods: {sendMessage() {EventBus.emit('message', 'Hello from component A')}}
}
</script>
// 组件 B
<template><div>{{ message }}</div>
</template><script>
import { onMounted, onUnmounted } from 'vue'
import { EventBus } from './event-bus'export default {data() {return {message: ''}},setup() {const handleMessage = (msg) => {message.value = msg}onMounted(() => {EventBus.on('message', handleMessage)})onUnmounted(() => {EventBus.off('message', handleMessage)})}
}
</script>
2.3 插槽
- 默认插槽
// 子组件
<template><slot></slot>
</template>
// 父组件
<ChildComponent><p>默认插槽内容</p>
</ChildComponent>
- 具名插槽
// 子组件
<template><slot name="header"></slot><slot></slot><slot name="footer"></slot>
</template>
// 父组件
<ChildComponent><template #header><h1>Header</h1></template><p>默认插槽内容</p><template #footer><p>Footer</p></template>
</ChildComponent>
- 作用域插槽
// 子组件
<template><slot :user="user"></slot>
</template><script>
export default {data() {return {user: { name: 'Alice', age: 25 }}}
}
</script>
// 父组件
<ChildComponent v-slot:default="slotProps"><p>{{ slotProps.user.name }}</p>
</ChildComponent>
三、Vue Router
3.1 基本配置
// router/index.js
import { createRouter, createWebHistory } from 'vue-router'
import Home from '../views/Home.vue'
import About from '../views/About.vue'const routes = [{ path: '/', name: 'Home', component: Home },{ path: '/about', name: 'About', component: About }
]const router = createRouter({history: createWebHistory(),routes
})export default router
// main.js
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'const app = createApp(App)
app.use(router)
app.mount('#app')
3.2 导航守卫
- 全局守卫
router.beforeEach((to, from, next) => {console.log('全局前置守卫')next()
})
- 路由独享守卫
const routes = [{path: '/',component: Home,beforeEnter: (to, from, next) => {console.log('路由独享守卫')next()}}
]
3.3 动态路由
- 定义动态路由
const routes = [{ path: '/user/:id', name: 'User', component: User },
]
- 获取路由参数
<template><div>User ID: {{ id }}</div>
</template><script>
import { useRoute } from 'vue-router'export default {setup() {const route = useRoute()return {id: route.params.id}}
}
</script>
- 路由跳转
<template><router-link to="/user/123">Go to User 123</router-link>
</template>
<script>
import { useRouter } from 'vue-router'export default {setup() {const router = useRouter()const goToUser = () => {router.push({ name: 'User', params: { id: 123 } })}return { goToUser }}
}
</script>
3.4 嵌套路由
const routes = [{path: '/dashboard',component: Dashboard,children: [{path: 'profile',component: Profile},{path: 'settings',component: Settings}]}
]
<!-- Dashboard.vue -->
<template><div><h1>Dashboard</h1><router-view></router-view></div>
</template>
3.5 路由懒加载
const Home = () => import('../views/Home.vue')
const routes = [{ path: '/', name: 'Home', component: Home },
]
四、Vuex
4.1 基本配置
// store/index.js
import { createStore } from 'vuex'export default createStore({state: {count: 0},mutations: {increment(state) {state.count++}},actions: {increment({ commit }) {commit('increment')}},getters: {doubleCount(state) {return state.count * 2}}
})
// main.js
import { createApp } from 'vue'
import App from './App.vue'
import store from './store'const app = createApp(App)
app.use(store)
app.mount('#app')
4.2 使用 Vuex
- 在组件中获取 state
<template><div>Count: {{ count }}</div>
</template><script>
import { mapState } from 'vuex'export default {computed: {...mapState(['count'])}
}
</script>
- 在组件中提交 mutation
<template><button @click="increment">Increment</button>
</template><script>
import { mapMutations } from 'vuex'export default {methods: {...mapMutations(['increment'])}
}
</script>
- 在组件中分发 action
<template><button @click="increment">Increment</button>
</template><script>
import { mapActions } from 'vuex'export default {methods: {...mapActions(['increment'])}
}
</script>
- 使用 getters
<template><div>Double Count: {{ doubleCount }}</div>
</template><script>
import { mapGetters } from 'vuex'export default {computed: {...mapGetters(['doubleCount'])}
}
</script>
4.3 模块化
// store/modules/user.js
export default {namespaced: true,state: {username: ''},mutations: {setUsername(state, username) {state.username = username}},actions: {setUsername({ commit }, username) {commit('setUsername', username)}},getters: {username: (state) => state.username}
}
// store/index.js
import { createStore } from 'vuex'
import user from './modules/user'export default createStore({modules: {user}
})
// 使用模块中的 state, mutation, action, getter
<template><div>Username: {{ username }}</div><button @click="setUsername('Alice')">Set Username</button>
</template><script>
import { mapState, mapMutations, mapActions, mapGetters } from 'vuex'export default {computed: {...mapState('user', ['username']),...mapGetters('user', ['username'])},methods: {...mapMutations('user', ['setUsername']),...mapActions('user', ['setUsername'])}
}
</script>
五、组合式 API
5.1 setup 函数
<template><div>{{ count }}</div><button @click="increment">Increment</button>
</template><script>
import { ref } from 'vue'export default {setup() {const count = ref(0)const increment = () => {count.value++}return {count,increment}}
}
</script>
5.2 响应式数据
- ref
const count = ref(0)
- reactive
const state = reactive({count: 0,user: {name: 'Alice'}
})
- computed
const doubleCount = computed(() => count.value * 2)
- watch
watch(count, (newVal, oldVal) => {console.log(`count changed from ${oldVal} to ${newVal}`)
})
5.3 生命周期钩子
- onMounted
onMounted(() => {console.log('mounted')
})
- onUnmounted
onUnmounted(() => {console.log('unmounted')
})
- 其他钩子: onBeforeMount, onBeforeUnmount, onActivated, onDeactivated, onErrorCaptured, onRenderTracked, onRenderTriggered
六、其他常用功能
6.1 指令
- v-bind
<img v-bind:src="imageSrc" />
- v-model
<input v-model="message" />
- v-if, v-else-if, v-else
<div v-if="isLoggedIn">Welcome!</div>
<div v-else>Please log in.</div>
- v-for
<ul><li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
-
v-on 修饰符
-
.stop
:阻止事件冒泡<button @click.stop="handleClick">Click</button>
-
.prevent
:阻止默认事件<form @submit.prevent="handleSubmit"></form>
-
.once
:事件只触发一次<button @click.once="handleClick">Click</button>
-
.self
:只有事件是从元素本身触发时才触发处理函数<div @click.self="handleClick"><button>Click</button> </div>
-
按键修饰符
<input @keyup.enter="submit" />
-
-
v-for 进阶用法
-
遍历对象
<ul><li v-for="(value, key, index) in object" :key="key">{{ index }} - {{ key }}: {{ value }}</li> </ul>
-
遍历数字范围
<div v-for="n in 10" :key="n">{{ n }}</div>
-
使用
v-for
和template
结合<ul><template v-for="item in items" :key="item.id"><li>{{ item.name }}</li><li class="divider" role="presentation"></li></template> </ul>
-
-
v-slot 插槽
-
具名插槽
<!-- 子组件 --> <slot name="header"></slot> <slot></slot> <slot name="footer"></slot>
<!-- 父组件 --> <ChildComponent><template #header><h1>Header</h1></template><p>默认插槽内容</p><template #footer><p>Footer</p></template> </ChildComponent>
-
作用域插槽
<!-- 子组件 --> <slot :user="user"></slot>
<!-- 父组件 --> <ChildComponent v-slot:default="slotProps"><p>{{ slotProps.user.name }}</p> </ChildComponent>
-
6.2 过渡与动画
-
单元素过渡
<transition name="fade"><div v-if="isVisible">Hello</div> </transition>
.fade-enter-active, .fade-leave-active {transition: opacity 0.5s; } .fade-enter-from, .fade-leave-to {opacity: 0; }
-
列表过渡
<transition-group name="list" tag="ul"><li v-for="item in items" :key="item.id">{{ item.name }}</li> </transition-group>
.list-enter-active, .list-leave-active {transition: all 0.5s; } .list-enter-from, .list-leave-to {opacity: 0;transform: translateY(30px); }
-
使用 JavaScript 钩子
<transition @before-enter="beforeEnter" @enter="enter" @leave="leave"><div v-if="isVisible">Hello</div> </transition>
export default {methods: {beforeEnter(el) {el.style.opacity = 0},enter(el, done) {Velocity(el, { opacity: 1, fontSize: '1.4em' }, { duration: 300, complete: done })},leave(el, done) {Velocity(el, { opacity: 0, fontSize: '1em' }, { duration: 300, complete: done })}} }
6.3 混入(Mixin)
-
定义混入
// mixins/log.js export default {created() {console.log('Mixin created')},methods: {log(message) {console.log(message)}} }
-
使用混入
<script> import logMixin from './mixins/log'export default {mixins: [logMixin],created() {this.log('Hello from component')} } </script>
6.4 自定义指令
-
定义自定义指令
// directives/focus.js export default {mounted(el) {el.focus()} }
-
注册全局指令
import { createApp } from 'vue' import App from './App.vue' import focusDirective from './directives/focus'const app = createApp(App) app.directive('focus', focusDirective) app.mount('#app')
-
使用自定义指令
<input v-focus />
6.5 插件
-
创建插件
// plugins/logger.js export default {install(app, options) {console.log(options)app.config.globalProperties.$logger = (message) => {console.log(message)}} }
-
使用插件
// main.js import { createApp } from 'vue' import App from './App.vue' import loggerPlugin from './plugins/logger'const app = createApp(App) app.use(loggerPlugin, { someOption: 'someValue' }) app.mount('#app')
// 在组件中使用 export default {mounted() {this.$logger('Hello from plugin')} }
七、Vue3 新特性
7.1 Teleport
-
使用 Teleport 将组件内容渲染到指定位置
<!-- App.vue --> <div><h1>App</h1><Teleport to="body"><div id="modal"><p>Modal Content</p></div></Teleport> </div>
7.2 Suspense
-
使用 Suspense 处理异步组件
<template><Suspense><template #default><AsyncComponent /></template><template #fallback><div>Loading...</div></template></Suspense> </template>
-
异步组件示例
// components/AsyncComponent.vue <template><div>Async Component</div> </template><script> export default {async setup() {// 模拟异步操作await new Promise((resolve) => setTimeout(resolve, 2000))return {}} } </script>
<!-- 使用 Suspense 包裹异步组件 --> <template><Suspense><template #default><AsyncComponent /></template><template #fallback><div>Loading...</div></template></Suspense> </template>
-
错误处理
<template><Suspense><template #default><AsyncComponent /></template><template #fallback><div>Loading...</div></template><template #error><div>Error occurred!</div></template></Suspense> </template>
// components/AsyncComponent.vue <script> export default {async setup() {await new Promise((_, reject) => setTimeout(() => reject(new Error('Async Error')), 2000))} } </script>
7.3 Fragment
-
Vue3 支持组件返回多个根节点,无需再使用包裹元素
<template><header>Header</header><main>Main Content</main><footer>Footer</footer> </template>
7.4 组合式 API 进阶
-
响应式 API
-
reactive
:创建响应式对象import { reactive } from 'vue'const state = reactive({count: 0,user: {name: 'Alice'} })
-
ref
:创建响应式引用import { ref } from 'vue'const count = ref(0)
-
computed
:创建计算属性import { ref, computed } from 'vue'const count = ref(0) const doubleCount = computed(() => count.value * 2)
-
watch
:监听数据变化import { ref, watch } from 'vue'const count = ref(0) watch(count, (newVal, oldVal) => {console.log(`count changed from ${oldVal} to ${newVal}`) })
-
-
生命周期钩子
-
onMounted
:组件挂载完成后调用import { onMounted } from 'vue'onMounted(() => {console.log('mounted') })
-
onUnmounted
:组件卸载后调用import { onUnmounted } from 'vue'onUnmounted(() => {console.log('unmounted') })
-
其他钩子:
onBeforeMount
,onBeforeUnmount
,onActivated
,onDeactivated
,onErrorCaptured
,onRenderTracked
,onRenderTriggered
-
-
依赖注入
-
provide
和inject
// 父组件 import { provide, ref } from 'vue'const theme = ref('dark') provide('theme', theme)
// 子组件 import { inject, onMounted } from 'vue'const theme = inject('theme') onMounted(() => {console.log(`Theme is ${theme.value}`) })
-
-
自定义 Hooks
-
创建可复用的组合式函数
// hooks/useMousePosition.js import { ref, onMounted, onUnmounted } from 'vue'export function useMousePosition() {const x = ref(0)const y = ref(0)const updatePosition = (e) => {x.value = e.pageXy.value = e.pageY}onMounted(() => {window.addEventListener('mousemove', updatePosition)})onUnmounted(() => {window.removeEventListener('mousemove', updatePosition)})return { x, y } }
// 使用自定义 Hook import { useMousePosition } from '../hooks/useMousePosition'export default {setup() {const { x, y } = useMousePosition()return { x, y }} }
-
八、性能优化
8.1 组件懒加载
-
使用动态导入实现组件懒加载
const Home = () => import('./views/Home.vue') const routes = [{ path: '/', component: Home } ]
8.2 避免不必要的渲染
-
使用
v-once
渲染静态内容<div v-once>{{ message }}</div>
-
使用
shallowRef
和shallowReactive
避免深层响应式import { shallowRef } from 'vue'const data = shallowRef({ count: 0 })
相关文章:

Vue3 常用代码指南手抄,超详细 cheatsheet
一、Vue3 基础 1.1 创建 Vue3 项目 使用 Vite 创建 npm create vitelatest my-vue-app -- --template vue cd my-vue-app npm install npm run dev使用 Vue CLI 创建 npm install -g vue/cli vue create my-vue-app1.2 项目结构 my-vue-app ├── node_modules ├── pu…...

结构体是否包含特定类型的成员变量
结构体是否包含特定类型的成员变量 在C中,可以使用模板元编程和类型特性(type traits)来判断一个结构体是否包含特定类型的成员变量。这通常通过std::is_member_object_pointer类型特性来实现,它可以用来检查给定的成员指针是否指…...
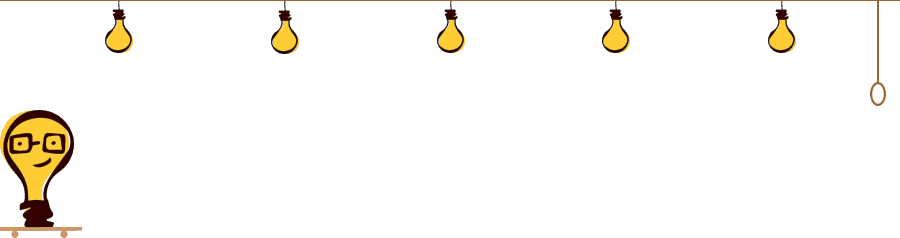
堆排序与链式二叉树:数据结构与排序算法的双重探索
大家好,我是小卡皮巴拉 文章目录 目录 引言 一.堆排序 1.1 版本一 核心概念 堆排序过程 1.2 版本二 堆排序函数 HeapSort 向下调整算法 AdjustDown 向上调整算法 AdjustUp 二.链式二叉树 2.1 前中后序遍历 链式二叉树的结构 创建链式二叉树 前序遍历…...
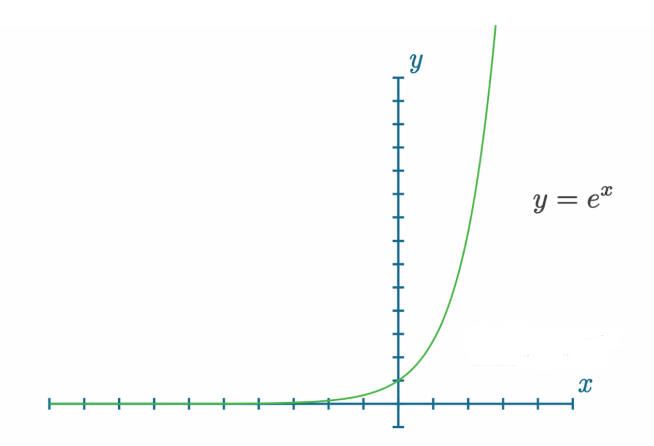
用 Python 从零开始创建神经网络(四):激活函数(Activation Functions)
激活函数(Activation Functions) 引言1. 激活函数的种类a. 阶跃激活功能b. 线性激活函数c. Sigmoid激活函数d. ReLU 激活函数e. more 2. 为什么使用激活函数3. 隐藏层的线性激活4. 一对神经元的 ReLU 激活5. 在隐蔽层中激活 ReLU6. ReLU 激活函数代码7. …...
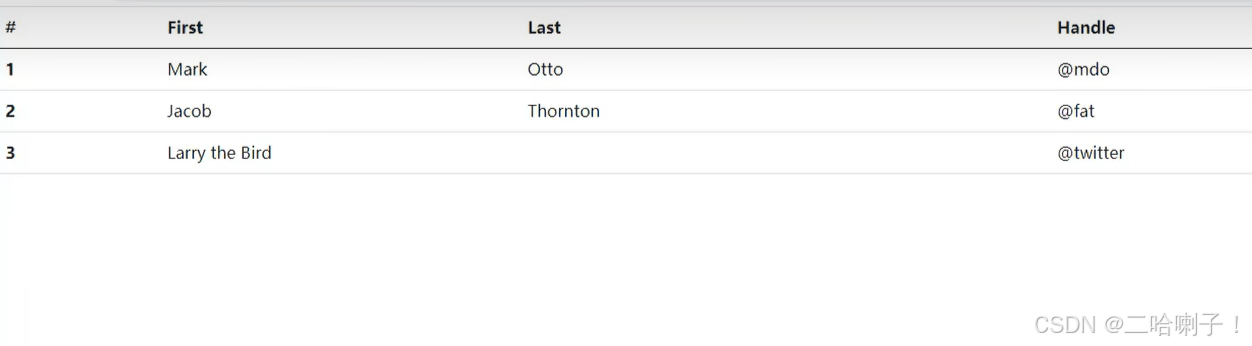
使用 Flask 和 ONLYOFFICE 实现文档在线编辑功能
提示:CSDN 博主测评ONLYOFFICE 文章目录 引言技术栈环境准备安装 ONLYOFFICE 文档服务器获取 API 密钥安装 Flask 和 Requests 创建 Flask 应用项目结构编写 app.py创建模板 templates/index.html 运行应用功能详解文档上传生成编辑器 URL显示编辑器回调处理 安全性…...

【C++】【算法基础】序列编辑距离
编辑距离 题目 给定 n n n个长度不超过 10 10 10 的字符串以及 m m m 次询问,每次询问给出一个字符串和一个操作次数上限。 对于每次询问,请你求出给定的 n n n个字符串中有多少个字符串可以在上限操作次数内经过操作变成询问给出的字符串。 每个…...
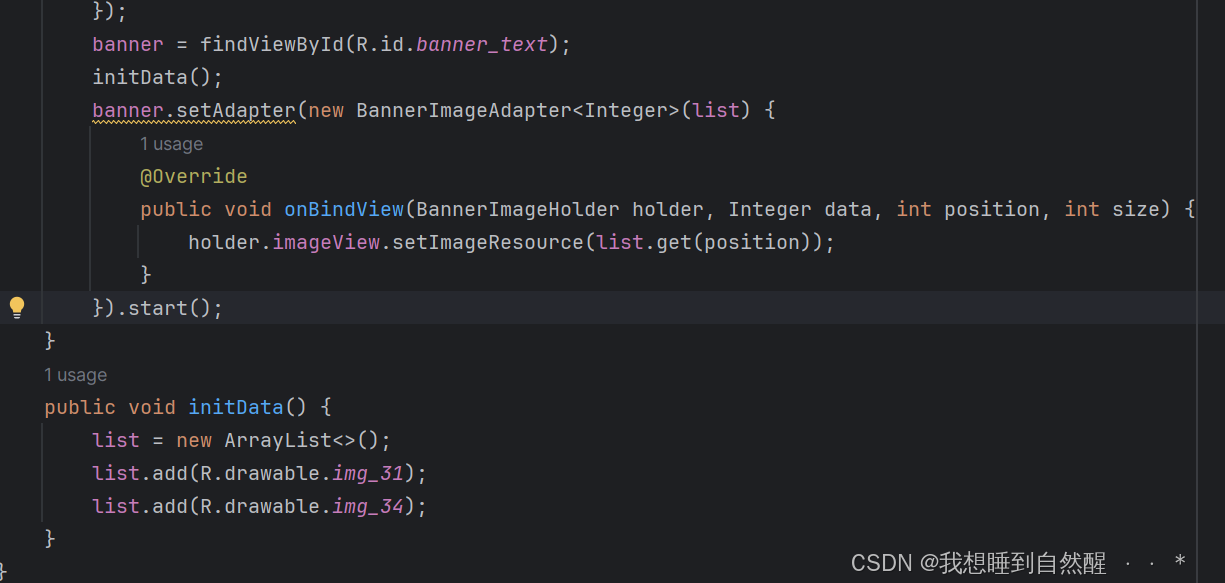
【Android】轮播图——Banner
引言 Banner轮播图是一种在网页和移动应用界面设计中常见的元素,主要用于在一个固定的区域内自动或手动切换一系列图片,以展示不同的内容或信息。这个控件在软件当中经常看到,商品促销、热门歌单、头像新闻等等。它不同于ViewPgaer在于无需手…...
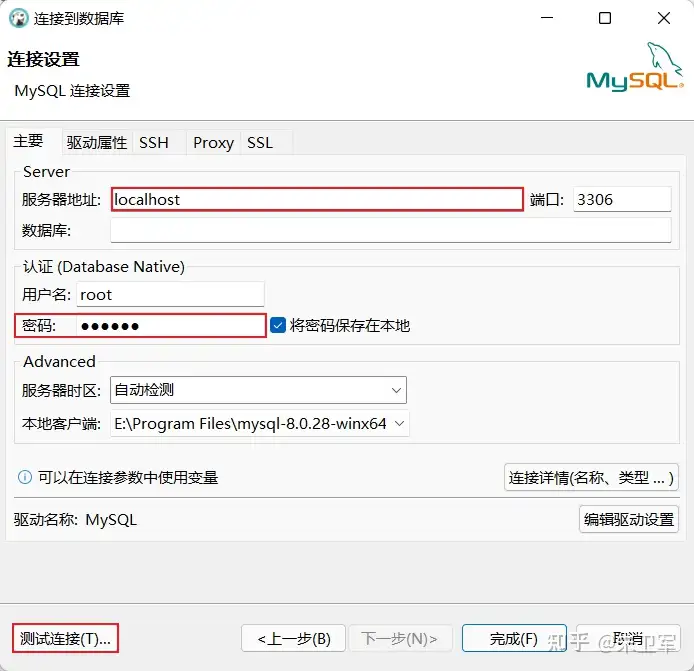
学SQL,要安装什么软件?
先上结论,推荐MySQLDbeaver的组合。 学SQL需要安装软件吗? 记得几年前我学习SQL的时候,以为像Java、Python一样需要安装SQL软件包,后来知道并没有所谓SQL软件,因为SQL是一种查询语言,它用来对数据库进行操…...

webstorm 设置总结
编辑器-》文件类型-》忽略的文件和文件夹-》加上node_modules 修改WebStorm 内存有两种方式。 1. 点击菜单中的Help -> change memory settings 弹出设置内存窗口,修改最大内存大小。然后点击Save and Restart 即可。 2. 点击菜单中的Help -> Edit Custom V…...
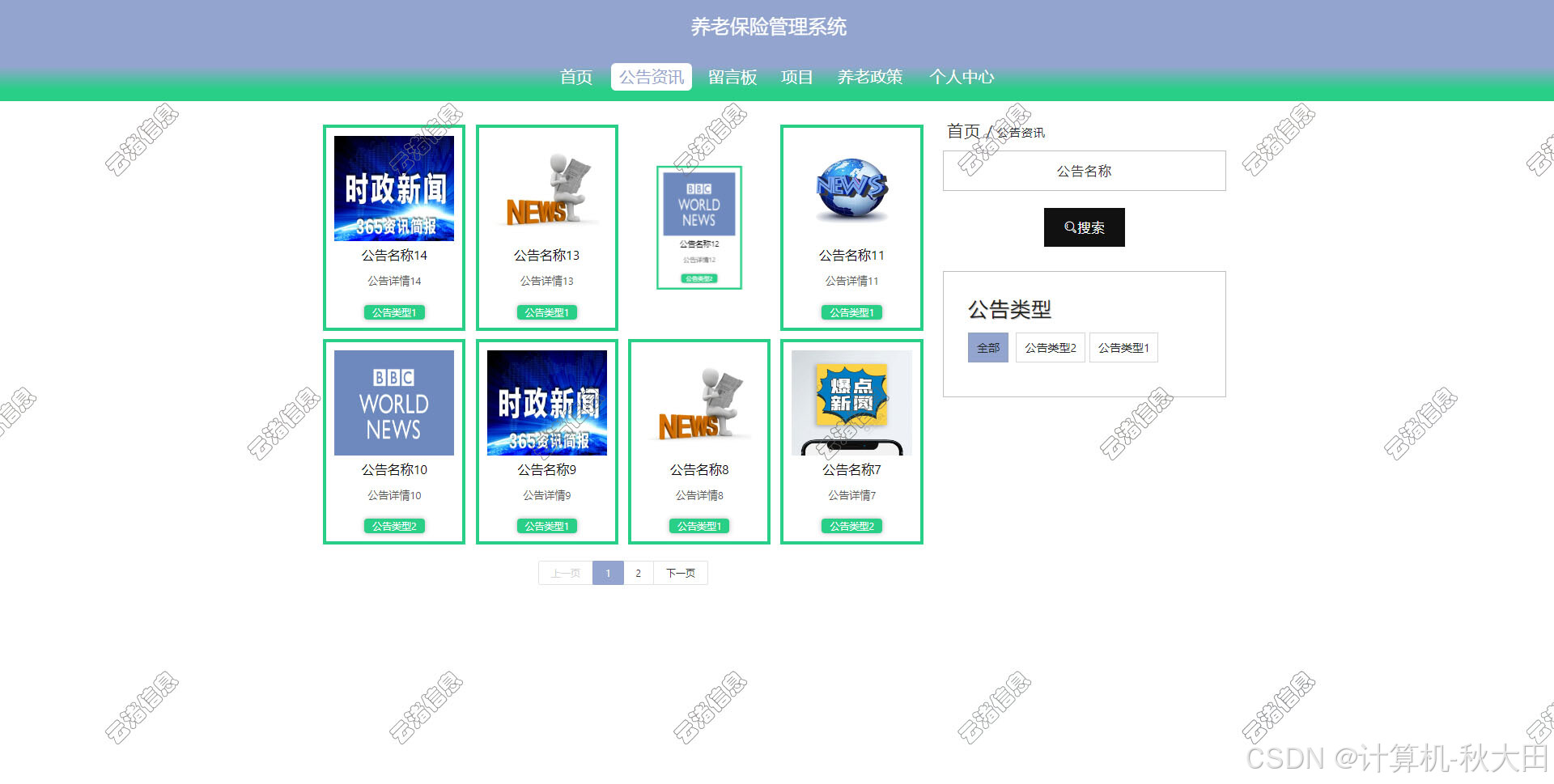
基于Spring Boot的养老保险管理系统的设计与实现,LW+源码+讲解
摘 要 如今社会上各行各业,都喜欢用自己行业的专属软件工作,互联网发展到这个时候,人们已经发现离不开了互联网。新技术的产生,往往能解决一些老技术的弊端问题。因为传统养老保险管理系统信息管理难度大,容错率低&a…...
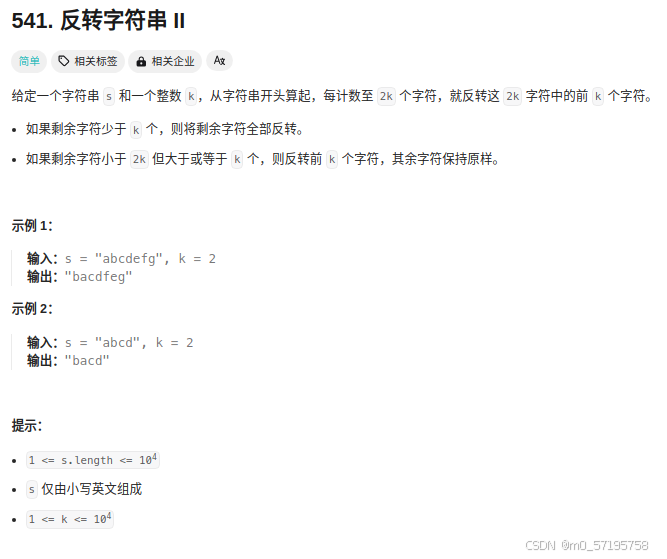
Java | Leetcode Java题解之第541题反转字符串II
题目: 题解: class Solution {public String reverseStr(String s, int k) {int n s.length();char[] arr s.toCharArray();for (int i 0; i < n; i 2 * k) {reverse(arr, i, Math.min(i k, n) - 1);}return new String(arr);}public void reve…...

sql分区
将学员表student按所在城市使用PARTITION BY LIST 1、创建分区表。 CREATE TABLE public.student( sno numeric(4,0), sname character varying(20 char),gender character varying(2 char), phone numeric(11,0), …...
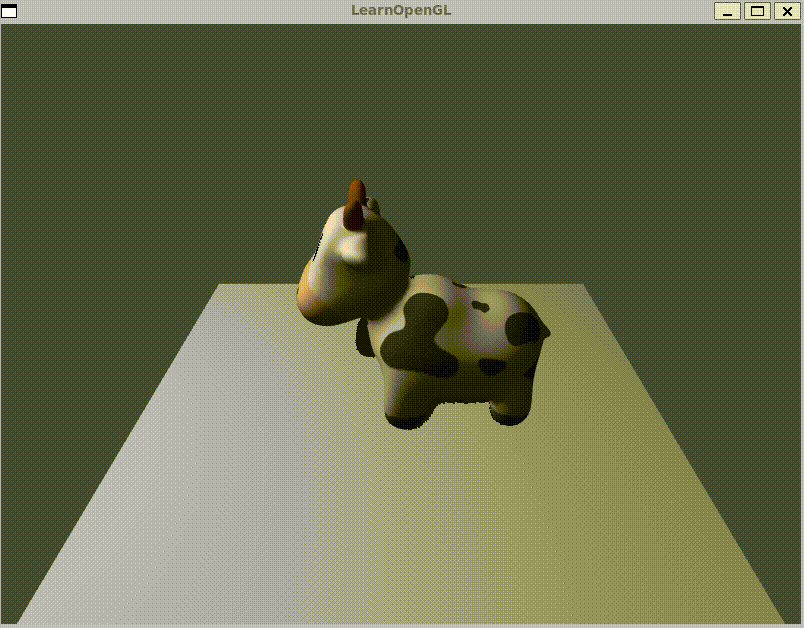
[OpenGL]使用OpenGL实现硬阴影效果
一、简介 本文介绍了如何使用OpenGL实现硬阴影效果,并在最后给出了全部的代码。本文基于[OpenGL]渲染Shadow Map,实现硬阴影的流程如下: 首先,以光源为视角,渲染场景的深度图,将light space中的深度图存储…...
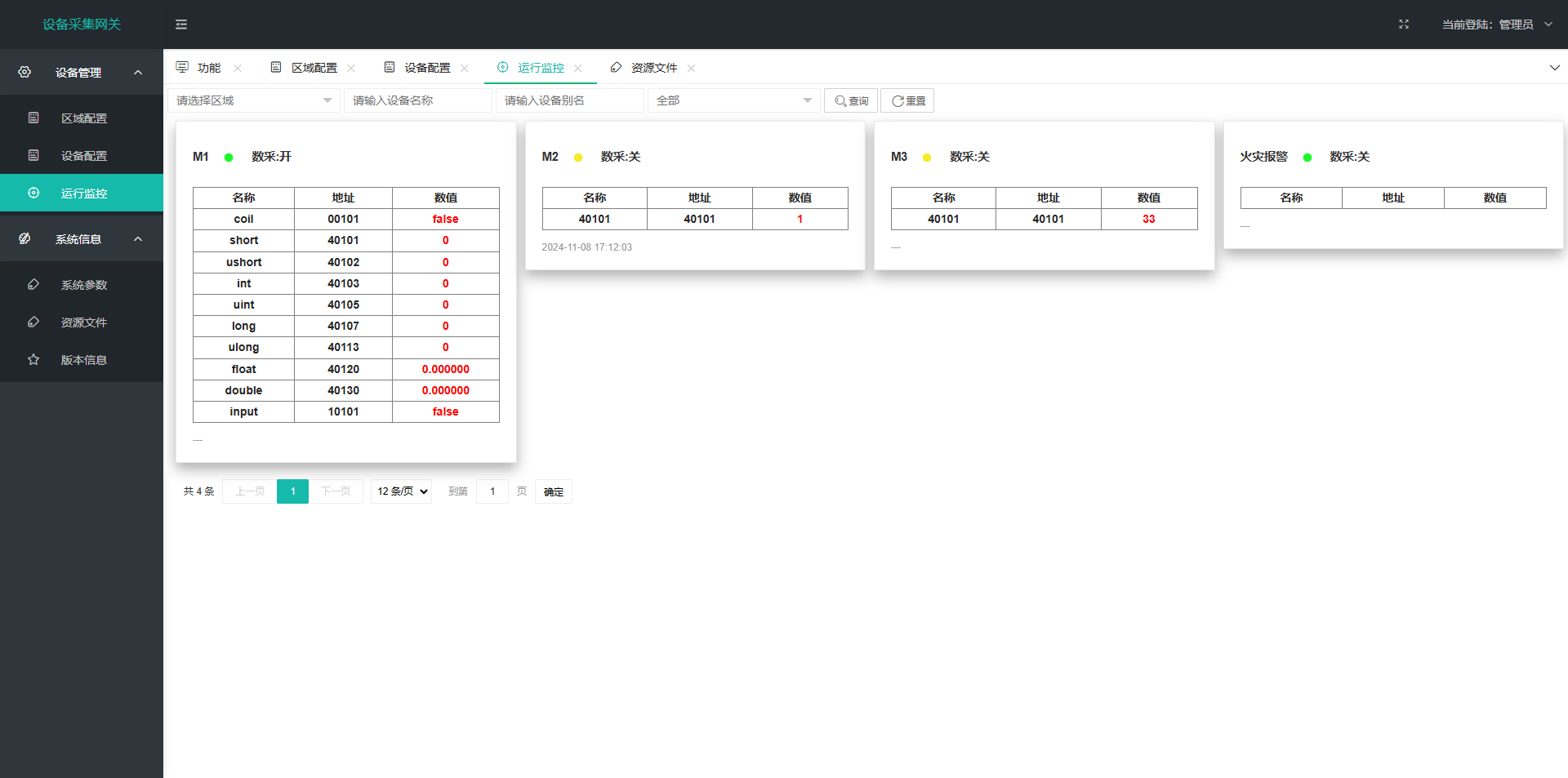
嵌入式采集网关(golang版本)
为了一次编写到处运行,使用纯GO编写,排除CGO,解决在嵌入式中交叉编译难问题 硬件设备:移远EC200A-CN LTE Cat 4 无线通信模块,搭载openwrt操作系统,90M内存...
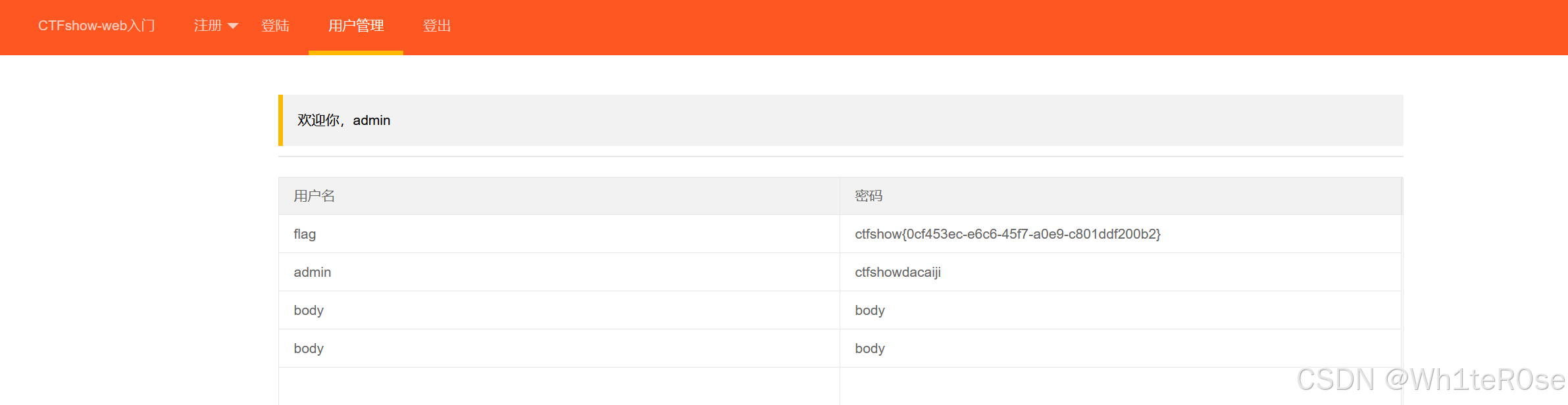
ctfshow(328)--XSS漏洞--存储型XSS
Web328 简单阅读一下页面。 是一个登录系统,存在一个用户管理数据库。 那么我们注册一个账号,在账号或者密码中植入HTML恶意代码,当管理员访问用户管理数据库页面时,就会触发我们的恶意代码。 思路 我们向数据库中写入盗取管理员…...

【C#】Thread.CurrentThread的用法
Thread.CurrentThread 是 System.Threading.Thread 类的一个静态属性,它返回当前正在执行的线程对象。通过 Thread.CurrentThread,可以访问和修改当前线程的各种属性和方法。 下面是一些常见的用法和示例: 1. 获取当前线程的信息 使用 Thr…...
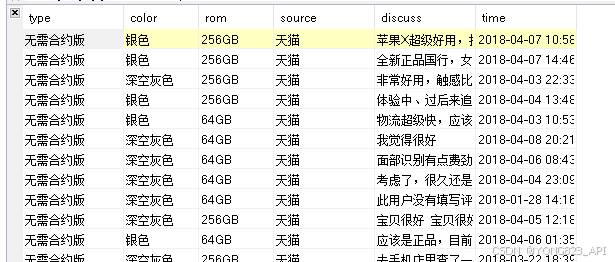
简单分享一下淘宝商品数据自动化抓取的技术实现与挑战
在电子商务领域,数据是驱动决策的关键。淘宝作为国内最大的电商平台之一,其商品数据对电商从业者来说具有极高的价值。然而,从淘宝平台自动化抓取商品数据并非易事,涉及多重技术和法律挑战。本文将从技术层面分析实现淘宝商品数据…...
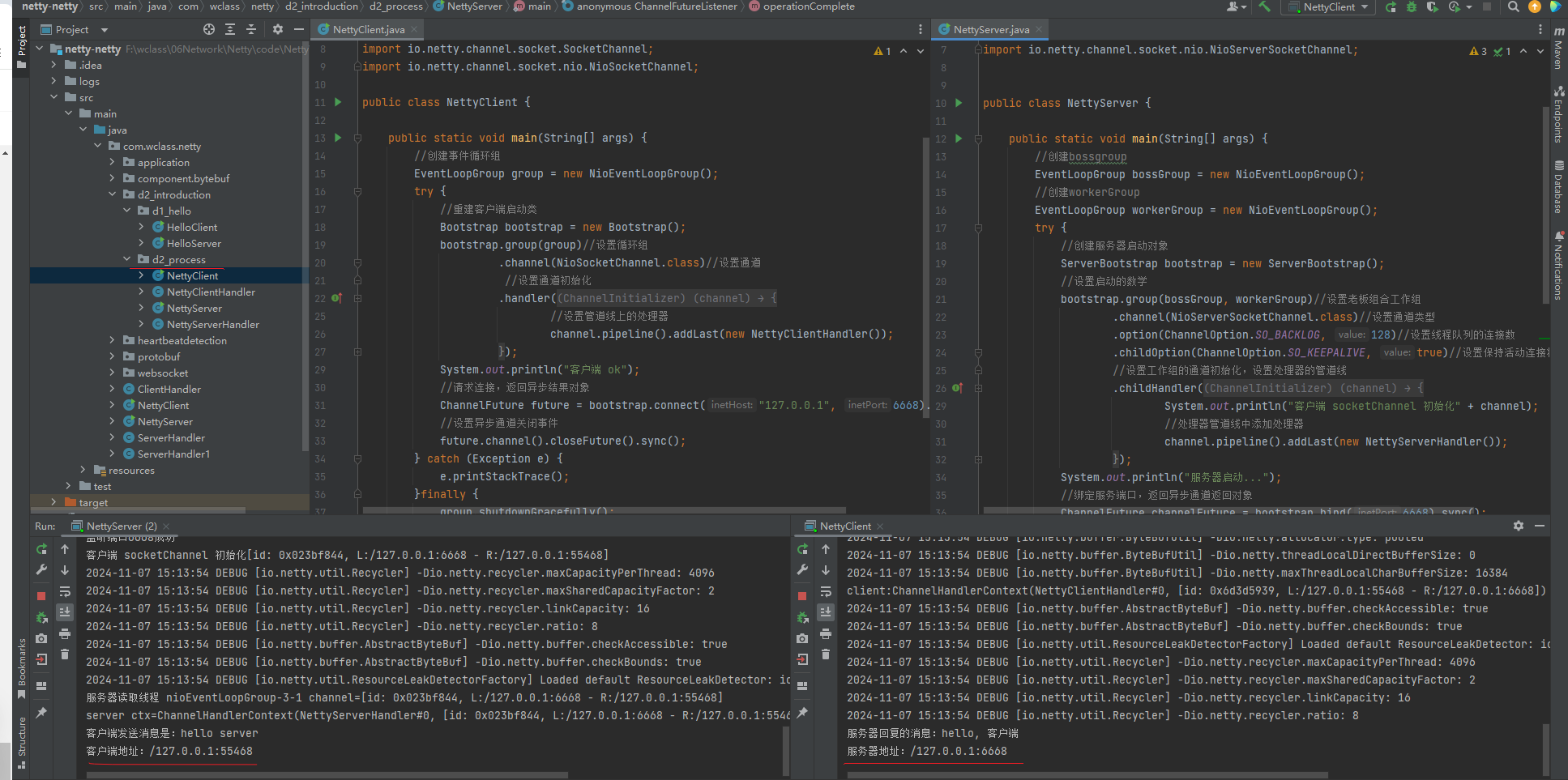
Netty篇(入门编程)
目录 一、Hello World 1. 目标 2. 服务器端 3. 客户端 4. 流程梳理 💡 提示 5. 运行结果截图 二、Netty执行流程 1. 流程分析 2. 代码案例 2.1. 引入依赖 2.2. 服务端 服务端 服务端处理器 2.3. 客户端 客户端 客户端处理器 2.4. 代码截图 一、Hel…...

【渗透测试】payload记录
Java开发使用char[]代替String保存敏感数据 Java Jvm会提供内存转储功能,当Java程序dump后,会生成堆内存的快照,保存在.hprof后缀的文件中,进而导致敏感信息的泄露。char[]可以在存储敏感数据后手动清零,String对象会…...

2024自动驾驶线控底盘行业研究报告
自动驾驶线控底盘是实现自动驾驶的关键技术之一,它通过电子信号来控制车辆的行驶,包括转向、制动、驱动、换挡和悬架等系统。线控底盘技术的发展对于自动驾驶汽车的实现至关重要,因为它提供了快速响应和精确控制的能力,这是自动驾驶系统所必需的。 线控底盘由五大系统组成…...

css3D变换用法
文章目录 CSS3D变换详解及代码案例一、CSS3D变换的基本概念二、3D变换的开启与景深设置三、代码案例 CSS3D变换详解及代码案例 CSS3D变换是CSS3中引入的一种强大功能,它允许开发者在网页上创建三维空间中的动画和交互效果。通过CSS3D变换,你可以实现元素…...

Rust:启动与关闭线程
在 Rust 编程中,启动和关闭线程是并发编程的重要部分。Rust 提供了强大的线程支持,允许你轻松地创建和管理线程。下面将详细解释如何在 Rust 中启动和关闭线程。 启动线程 在 Rust 中,你可以使用标准库中的 std::thread 模块来创建和启动新…...
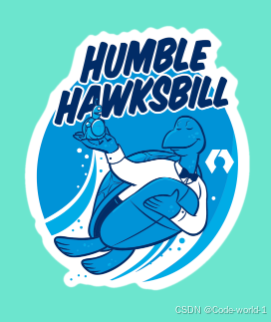
Ubuntu 的 ROS 2 操作系统安装与测试
引言 机器人操作系统(ROS, Robot Operating System)是一种广泛应用于机器人开发的开源框架,提供了丰富的库和工具,支持开发者快速构建、控制机器人并实现智能功能。 当前,ROS 2 的最新长期支持版本为 Humble Hawksbil…...
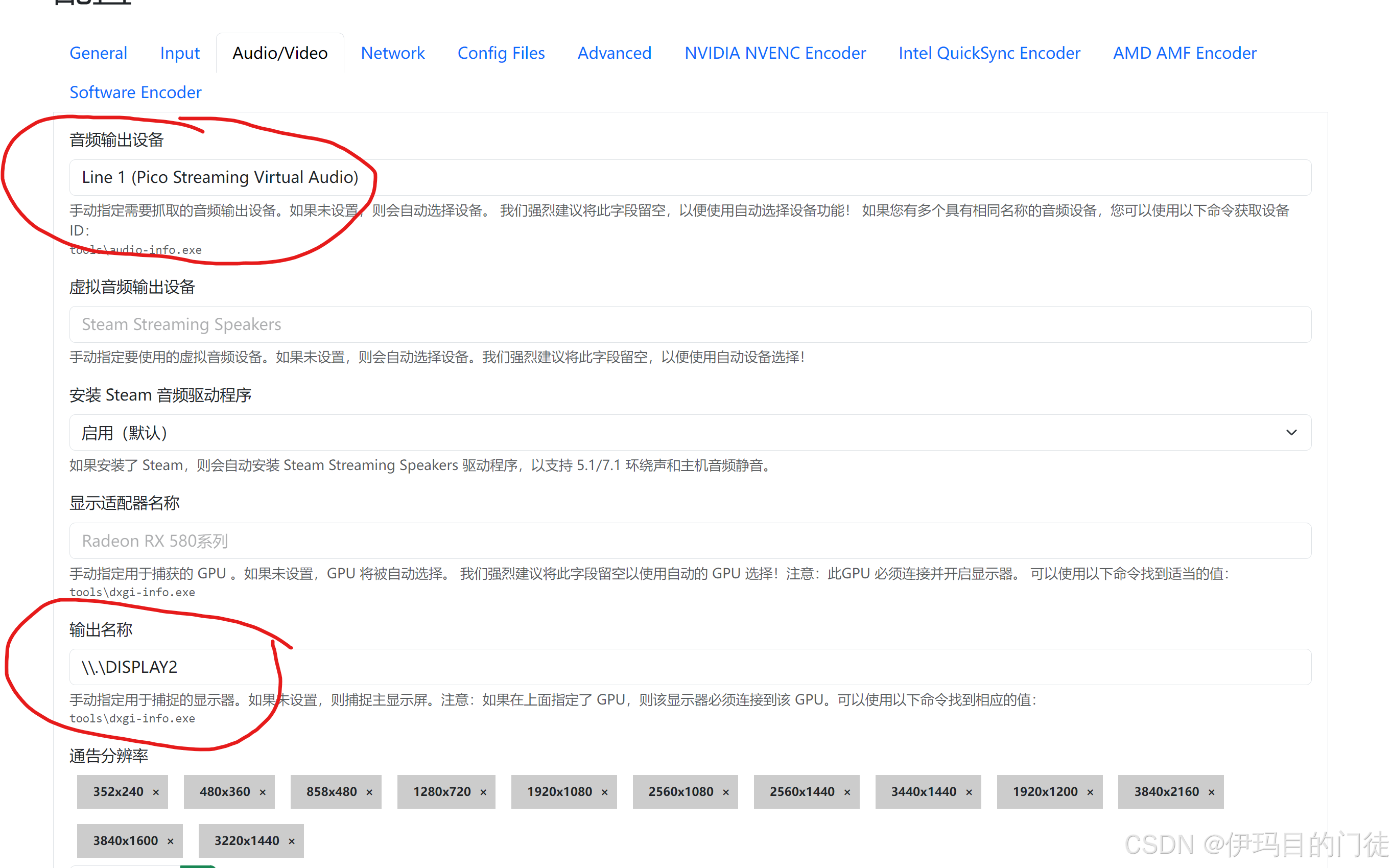
在双显示器环境中利用Sunshine与Moonlight实现游戏串流的同时与电脑其他任务互不干扰
我和老婆经常会同时需要操作家里的电脑,在周末老婆有时要用电脑加班上网办公,而我想在难得的周末好好地Game一下(在客厅用电视机或者平板串流),但是电脑只有一个,以往我一直都是把电脑让给老婆,…...
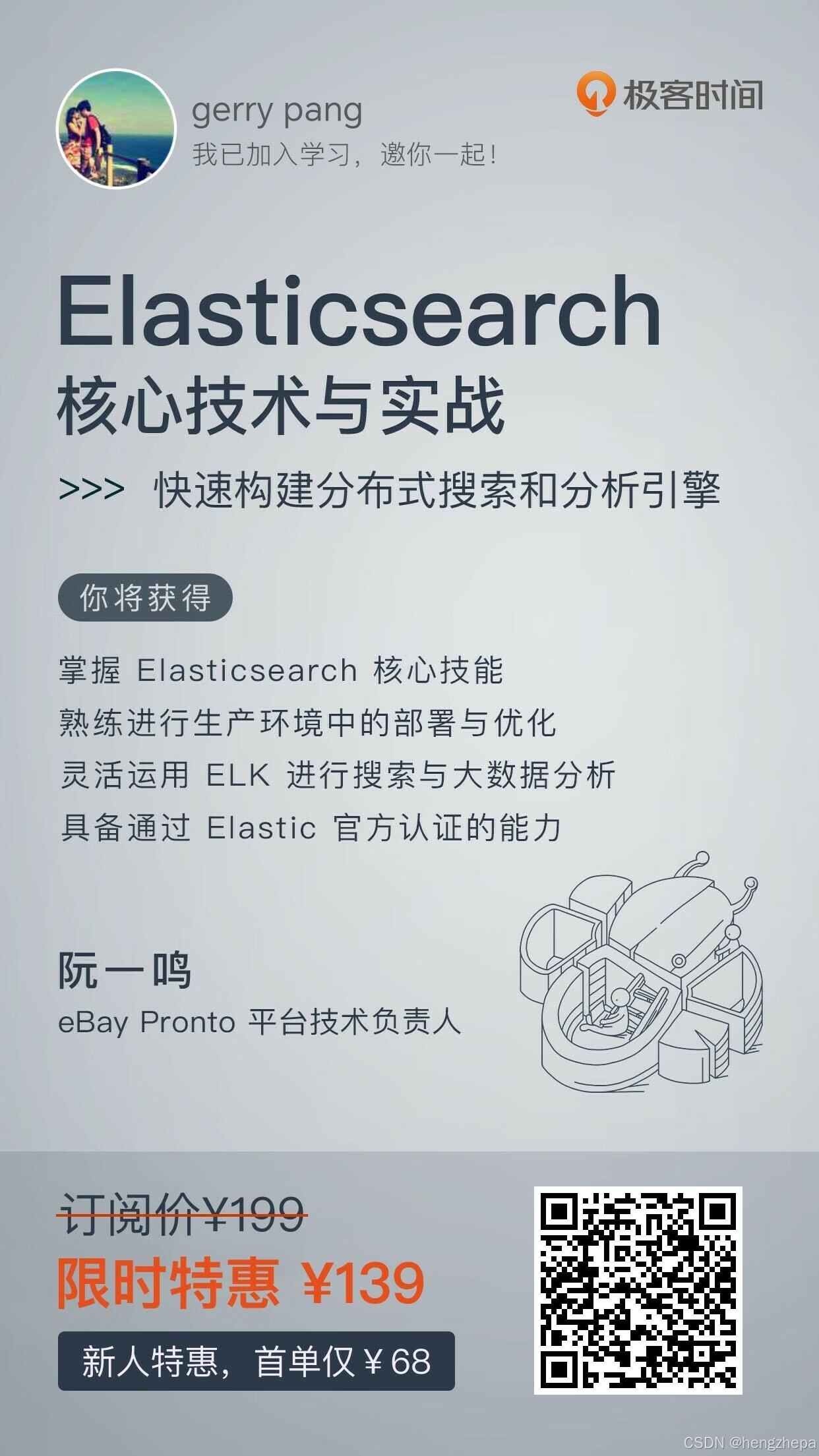
ElasticSearch备考 -- Cross cluster replication(CCR)
一、题目 操作在cluster1(local)中操作索引task,复制到cluster2(remote)中 二、思考 CCR 我们可以对标MySQL 理解为为主从,后者备份。主节点负责写入数据,从/备节点负责同步时主节点的数据。 …...

windows C#-异常处理
C# 程序员使用 try 块来对可能受异常影响的代码进行分区。 关联的 catch 块用于处理生成的任何异常。 finally 块包含无论 try 块中是否引发异常都会运行的代码,如发布 try 块中分配的资源。 try 块需要一个或多个关联的 catch 块或一个 finally 块,或两…...
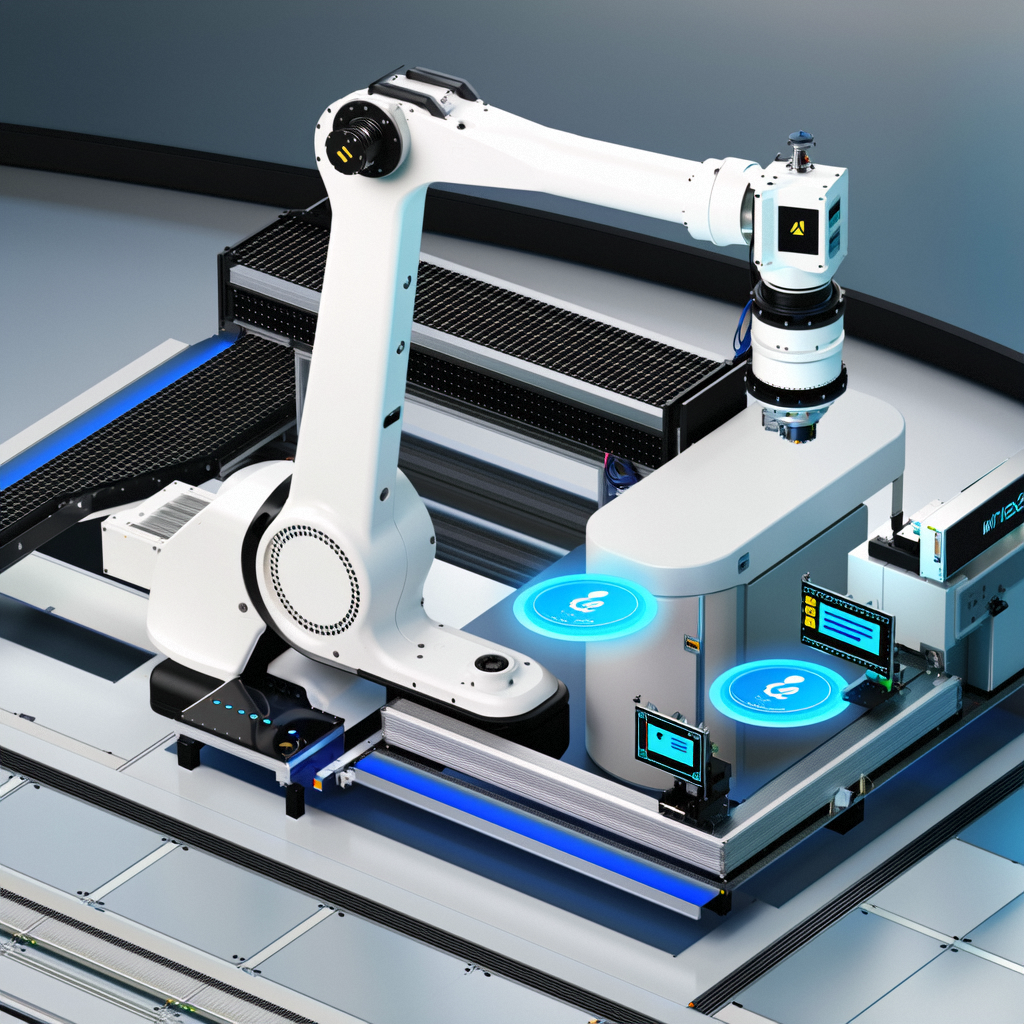
边缘计算在智能制造中的应用
💓 博客主页:瑕疵的CSDN主页 📝 Gitee主页:瑕疵的gitee主页 ⏩ 文章专栏:《热点资讯》 边缘计算在智能制造中的应用 边缘计算在智能制造中的应用 边缘计算在智能制造中的应用 引言 边缘计算概述 定义与原理 发展历程 …...

点云开发:从入门到精通的全面教程
简介 点云技术已成为计算机视觉、自动驾驶、3D重建等领域的重要组成部分。本教程旨在引导你从零基础开始学习点云开发,深入理解其背后的数学原理,并提供实用的开发技巧。 章节目录 点云技术概述 点云的定义及应用场景点云数据的来源和采集工具点云数据…...
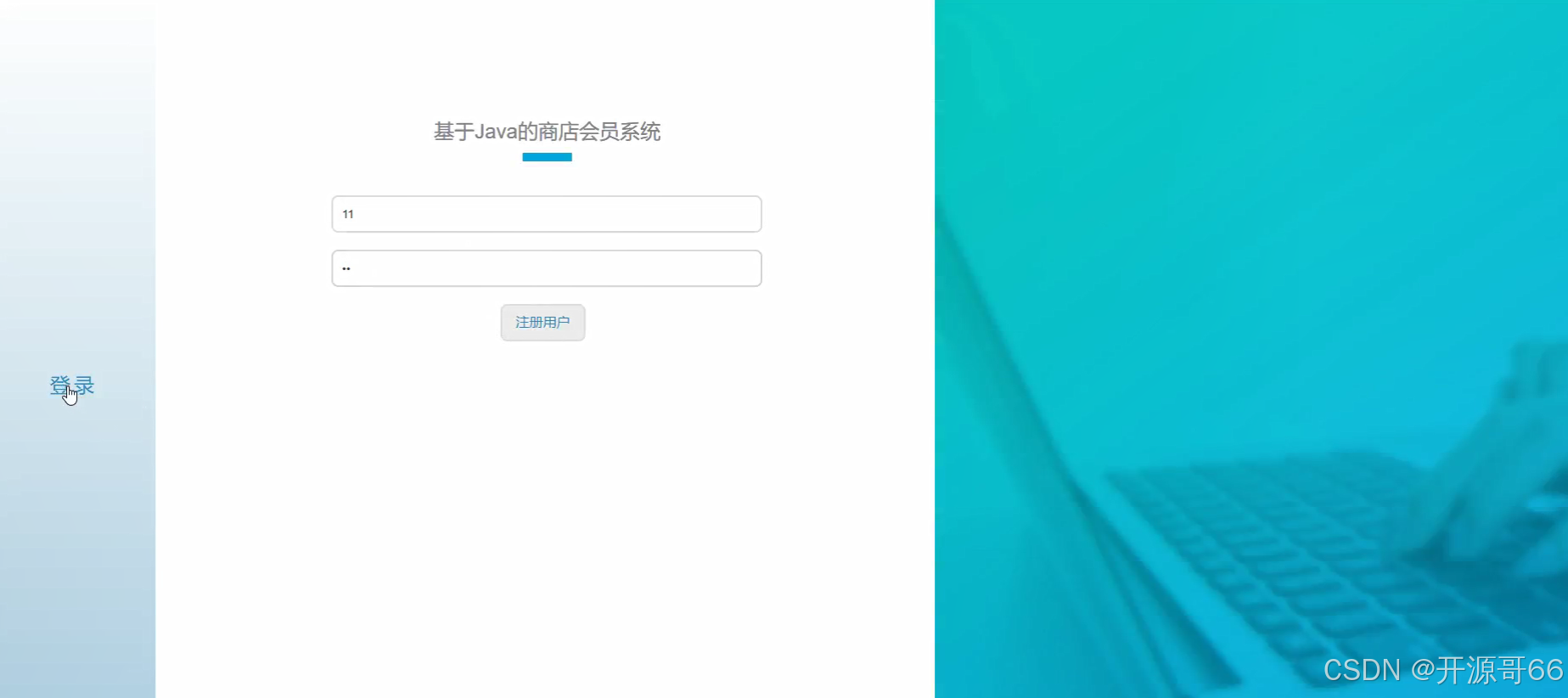
【含文档】基于ssm+jsp的商店会员系统(含源码+数据库+lw)
1.开发环境 开发系统:Windows10/11 架构模式:MVC/前后端分离 JDK版本: Java JDK1.8 开发工具:IDEA 数据库版本: mysql5.7或8.0 数据库可视化工具: navicat 服务器: apache tomcat 主要技术: Java,Spring,SpringMvc,mybatis,mysql,vue 2.视频演示地址 3.功能 系统定义了两个…...
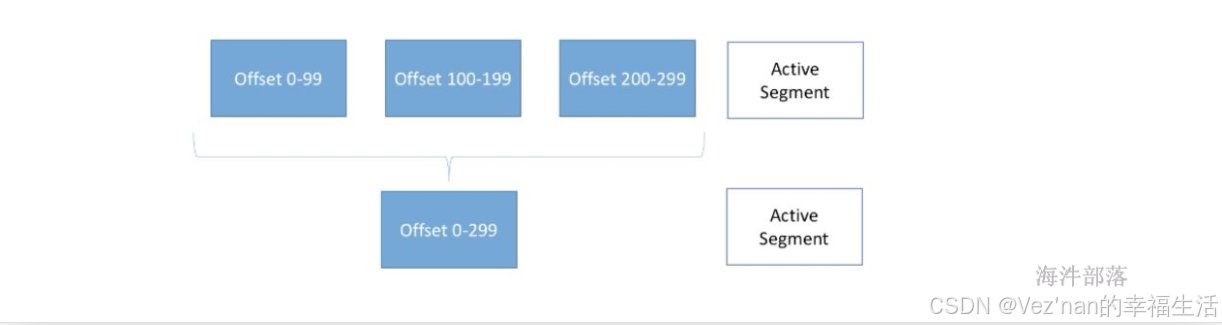
【大数据学习 | kafka高级部分】文件清除原理
2. 两种文件清除策略 kafka数据并不是为了做大量存储使用的,主要的功能是在流式计算中进行数据的流转,所以kafka中的数据并不做长期存储,默认存储时间为7天 那么问题来了,kafka中的数据是如何进行删除的呢? 在Kafka…...