Java Email 发HTML邮件工具 采用 freemarker模板引擎渲染
Java Email 发HTML邮件工具 采用 freemarker模板引擎
1.常用方式对比
Java发送邮件有很多的实现方式
-
第一种:Java 原生发邮件
mail.jar
和activation.jar
<!-- https://mvnrepository.com/artifact/javax.mail/mail --> <dependency><groupId>javax.mail</groupId><artifactId>mail</artifactId><version>1.4.7</version> </dependency> <!-- https://mvnrepository.com/artifact/javax.activation/activation --> <dependency><groupId>javax.activation</groupId><artifactId>activation</artifactId><version>1.1.1</version> </dependency>
原生的java可以发送普通文本和HTML邮件,同时也可以发送带附件的邮件,但是缺点也很明显,配置非常繁琐,不同的邮件需要不同的实现类去完成,不适合项目中使用。
-
第二种:使用框架提供的去实现,在SpringBoot中有实现这个功能的组件
spring-boot-starter-mail
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-mail --> <dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-mail</artifactId> </dependency>
这种实现起来特别方便,仅仅只要在SpringBoot项目中引入组件,在配置文件中配置好各种参数,就可以实现依赖注入,调用接口完成发送邮件,同时支持普通文本、HTML邮件、以及携带附件的邮件。同时缺点就是和框架集成太高了,如果项目中没有使用SpringBoot就不是那么好用了。
-
第三种:采用Apache提供的邮件工具
commons-email
,项目中已经封装好了一些常用的发邮件的接口供开发者使用,并且配置起来也比较简单,和其他技术耦合低是比较好的解决问题的方案。<dependency><groupId>org.apache.commons</groupId><artifactId>commons-email</artifactId><version>1.5</version> </dependency>
2.项目中使用
项目使用的jar包,采用maven工程。
<dependency><groupId>org.apache.commons</groupId><artifactId>commons-email</artifactId><version>1.5</version> </dependency>
第三方jar包引入时下载:https://mvnrepository.com/artifact/org.apache.commons/commons-email
(1)创建配置文件email.properties
- 建议将配置文件统一放置在
resources
文件夹中
# 邮件服务器的地址
host=smtp.exmail.qq.com
# 邮件服务器的端口(百度上有,或者邮件提供商开发文档有)
port=456
# 发件人邮箱
username=
# 密码或授权码
password=
# 发件人名称,如果不提供会使用发件人邮件作为发件人
formName=
(2)发邮件的核心代码
package com.zhongcode.demo.email;import org.apache.commons.mail.*;import java.util.Collections;
import java.util.List;
import java.util.Properties;/*** 邮件发送实例*/
public class SendEmail {/*** 邮件服务器 host*/private String host;/*** 邮件服务器端口 port*/private int port;/*** 用户名*/private String username;/*** 密码(授权码)*/private String password;/*** 发件人名称*/private String formName;/*** 是否开启debug*/private boolean debug;public SendEmail() {}public SendEmail(Properties pro) {this.host = pro.getProperty("host");this.port = Integer.parseInt(pro.getProperty("port", "0"));this.username = pro.getProperty("username");this.password = pro.getProperty("password");this.formName = pro.getProperty("formName");}public SendEmail(String host, int port, String username, String password, String formName, boolean debug) {this.host = host;this.port = port;this.username = username;this.password = password;this.formName = formName;this.debug = debug;}public SendEmail(String host, int port, String username, String password, String formName) {this.host = host;this.port = port;this.username = username;this.password = password;this.formName = formName;}public SendEmail(String host, int port, String username, String password) {this.host = host;this.port = port;this.username = username;this.password = password;}public String getHost() {return host;}public void setHost(String host) {this.host = host;}public int getPort() {return port;}public void setPort(int port) {this.port = port;}public String getUsername() {return username;}public void setUsername(String username) {this.username = username;}public String getPassword() {return password;}public void setPassword(String password) {this.password = password;}public String getFormName() {return formName;}public void setFormName(String formName) {this.formName = formName;}public boolean isDebug() {return debug;}public void setDebug(boolean debug) {this.debug = debug;}/*** 发邮件** @param to 收件件人* @param subject 主题* @param msg 消息* @throws EmailException 邮件异常*/public synchronized void sendEmail(String to, String subject, String msg) throws EmailException {sendEmail(Collections.singletonList(to), subject, msg, null, null);}/*** 发邮件** @param toEmails 发送列表* @param subject 主题* @param msg 内容支持html* @param attaches 附件* @param fromName 发送名称* @throws EmailException 邮件异常*/public synchronized void sendEmail(List<String> toEmails, String subject, String msg, List<String> attaches, String fromName) throws EmailException {// 创建邮件对象MultiPartEmail email;// 当附件不为空时,使用MultiPartEmail添加附件if (attaches != null) {email = new MultiPartEmail();for (String att : attaches) {EmailAttachment attachment = new EmailAttachment();attachment.setPath(att);// 截取文件名attachment.setName(att.substring(att.lastIndexOf("/") + 1));email.attach(attachment);}// 当使用MultiPartEmail时渲染html要使用Part进行添加email.addPart(msg, "text/html; charset=UTF-8");} else {// 默认使用HtmlEmail创建email = new HtmlEmail();email.setMsg(msg);}// 设置主机email.setHostName(host);// 设置端口email.setSmtpPort(port);email.setDebug(debug);email.setAuthenticator(new DefaultAuthenticator(username, password));email.setCharset("UTF-8");email.setSSLOnConnect(true);email.setFrom(username, fromName == null ? (this.formName == null ? username : this.formName) : fromName);email.setSubject(subject);for (String to : toEmails) {email.addTo(to);}email.send();}
}
(3)程序发邮件测试
public static void main(String[] args) throws EmailException, IOException, TemplateException {// 加载配置文件InputStream is = Main.class.getClassLoader().getResourceAsStream("email.properties");Properties pro = new Properties();pro.load(is);// 创建邮件SendEmail sendEmail = new SendEmail(pro);sendEmail.sendEmail("收件人", "test", "测试邮件");
}
3.发送HTML模板邮件
当我们想发送模板HTML时,需要发送HTML格式的代码,主要是纯文本的邮件并不好看,而且项目中经常使用的是好看的模板,比如系统用户注册时需要发送验证码,很多情况下都要发邮件。
这里我们采用的freemarker
模板引擎来渲染我们的HTML模板,下面是以发送邮箱验证码为例。
(1)引入freemarker模板引擎
<dependency><groupId>org.freemarker</groupId><artifactId>freemarker</artifactId><version>2.3.32</version>
</dependency>
(2)创建HTML模板
文件名:code.ftl
<meta charset="utf-8"><table width="100%"><tr><td style="width: 100%;"><center><table class="content-wrap" style="margin: 0px auto; width: 600px;"><tr><td style="margin: 0px auto; overflow: hidden; padding: 0px; border: 0px dotted rgb(238, 238, 238);"><!----><div class="full" tindex="1" style="margin: 0px auto; max-width: 600px;"><table align="center" border="0" cellpadding="0" cellspacing="0" class="fullTable" style="width: 600px;"><tbody><tr><td class="fullTd" style="direction: ltr; width: 600px; font-size: 0px; padding-bottom: 0px; text-align: center; vertical-align: top; background-color: rgb(170, 0, 170); background-image: url(""); background-repeat: no-repeat; background-size: 100px; background-position: 10% 50%;"><table border="0" cellpadding="0" cellspacing="0" width="100%" style="vertical-align: top;"><tr><td align="left" style="font-size: 0px; padding: 20px;"><div class="text" style="font-family: 微软雅黑, "Microsoft YaHei"; overflow-wrap: break-word; margin: 0px; text-align: center; line-height: 1.6; color: rgb(255, 255, 255); font-size: 18px; font-weight: normal;"><div><p style="text-size-adjust: none; word-break: break-word; line-height: 1.6; font-size: 18px; margin: 0px;"><strong>系统提示</strong></p></div></div></td></tr></table></td></tr></tbody></table></div><div class="full" tindex="2" style="margin: 0px auto; max-width: 600px;"><table align="center" border="0" cellpadding="0" cellspacing="0" class="fullTable" style="width: 600px;"><tbody><tr><td class="fullTd" style="direction: ltr; width: 600px; font-size: 0px; padding-bottom: 0px; text-align: center; vertical-align: top; background-image: url(""); background-repeat: no-repeat; background-size: 100px; background-position: 10% 50%;"><table border="0" cellpadding="0" cellspacing="0" width="100%" style="vertical-align: top;"><tr><td align="left" style="font-size: 0px; padding: 20px 20px 5px;"><div class="text" style="font-family: 微软雅黑, "Microsoft YaHei"; overflow-wrap: break-word; margin: 0px; text-align: left; line-height: 20px; color: rgb(102, 102, 102); font-size: 14px; font-weight: normal;"><div><p style="text-size-adjust: none; word-break: break-word; line-height: 20px; font-size: 14px; margin: 0px;">您的验证码为:</p></div></div></td></tr></table></td></tr></tbody></table></div><div class="full" tindex="3" style="margin: 0px auto; max-width: 600px;"><table align="center" border="0" cellpadding="0" cellspacing="0" class="fullTable" style="width: 600px;"><tbody><tr><td class="fullTd" style="direction: ltr; width: 600px; font-size: 0px; padding-bottom: 0px; text-align: center; vertical-align: top; background-image: url(""); background-repeat: no-repeat; background-size: 100px; background-position: 10% 50%;"><table border="0" cellpadding="0" cellspacing="0" width="100%" style="vertical-align: top;"><tr><td align="left" style="font-size: 0px; padding: 20px;"><div class="text" style="font-family: 微软雅黑, "Microsoft YaHei"; overflow-wrap: break-word; margin: 0px; text-align: center; line-height: 20px; color: rgb(0, 0, 0); font-size: 24px; font-weight: normal;"><div><p style="text-size-adjust: none; word-break: break-word; line-height: 20px; font-size: 24px; margin: 0px;"><strong>${code}</strong></p></div></div></td></tr></table></td></tr></tbody></table></div><div class="full" tindex="4" style="margin: 0px auto; max-width: 600px;"><table align="center" border="0" cellpadding="0" cellspacing="0" class="fullTable" style="width: 600px;"><tbody><tr><td class="fullTd" style="direction: ltr; width: 600px; font-size: 0px; padding-bottom: 0px; text-align: center; vertical-align: top; background-image: url(""); background-repeat: no-repeat; background-size: 100px; background-position: 10% 50%;"><table border="0" cellpadding="0" cellspacing="0" width="100%" style="vertical-align: top;"><tr><td align="left" style="font-size: 0px; padding: 8px 20px 20px;"><div class="text" style="font-family: 微软雅黑, "Microsoft YaHei"; overflow-wrap: break-word; margin: 0px; text-align: left; line-height: 20px; color: rgb(102, 102, 102); font-size: 12px; font-weight: normal;"><div><p style="text-size-adjust: none; word-break: break-word; line-height: 20px; font-size: 12px; margin: 0px;"><span style="color: #333333;">验证码10分钟内有效。</span></p></div></div></td></tr></table></td></tr></tbody></table></div><div class="full" tindex="5" style="margin: 0px auto; line-height: 0px; max-width: 600px;"><table align="center" border="0" cellpadding="0" cellspacing="0" class="fullTable" style="width: 600px;"><tbody><tr><td align="center" class="fullTd" style="direction: ltr; font-size: 0px; padding: 10px 20px; text-align: center; vertical-align: top; word-break: break-word; width: 600px; background-image: url(""); background-repeat: no-repeat; background-size: 100px; background-position: 10% 50%;"><table align="center" border="0" cellpadding="0" cellspacing="0" style="border-collapse: collapse; border-spacing: 0px;"><tbody><tr><td style="width: 600px; border-top: 1px solid rgb(204, 204, 204);"></td></tr></tbody></table></td></tr></tbody></table></div><div class="full" tindex="6" style="margin: 0px auto; max-width: 600px;"><table align="center" border="0" cellpadding="0" cellspacing="0" class="fullTable" style="width: 600px;"><tbody><tr><td class="fullTd" style="direction: ltr; width: 600px; font-size: 0px; padding-bottom: 0px; text-align: center; vertical-align: top; background-image: url(""); background-repeat: no-repeat; background-size: 100px; background-position: 10% 50%;"><table border="0" cellpadding="0" cellspacing="0" width="100%" style="vertical-align: top;"><tr><td align="left" style="font-size: 0px; padding: 10px 20px 0px;"><div class="text" style="font-family: 微软雅黑, "Microsoft YaHei"; overflow-wrap: break-word; margin: 0px; text-align: left; line-height: 20px; color: rgb(170, 170, 170); font-size: 12px; font-weight: normal;"><div><p style="text-size-adjust: none; word-break: break-word; line-height: 20px; font-size: 12px; margin: 0px;">此为系统邮件请勿回复</p></div></div></td></tr></table></td></tr></tbody></table></div><div class="full" tindex="7" style="margin: 0px auto; max-width: 600px;"><table align="center" border="0" cellpadding="0" cellspacing="0" class="fullTable" style="width: 600px;"><tbody><tr><td class="fullTd" style="direction: ltr; width: 600px; font-size: 0px; padding-bottom: 0px; text-align: center; vertical-align: top; background-image: url(""); background-repeat: no-repeat; background-size: 100px; background-position: 10% 50%;"><table border="0" cellpadding="0" cellspacing="0" width="100%" style="vertical-align: top;"><tr><td align="left" style="font-size: 0px; padding: 20px;"><div class="text" style="font-family: 微软雅黑, "Microsoft YaHei"; overflow-wrap: break-word; margin: 0px; text-align: center; line-height: 20px; color: rgb(170, 170, 170); font-size: 12px; font-weight: normal;"><div><p style="text-size-adjust: none; word-break: break-word; line-height: 20px; font-size: 12px; margin: 0px;">Copyright © zhongcode 2020 All Right Reserved</p></div></div></td></tr></table></td></tr></tbody></table></div></td></tr></table></center></td></tr></table>
(3)代码测试
public class Main {public static void main(String[] args) throws EmailException, IOException, TemplateException {//1.创建配置类Configuration configuration = new Configuration(Configuration.DEFAULT_INCOMPATIBLE_IMPROVEMENTS);//2.设置模板所在的目录configuration.setDirectoryForTemplateLoading(new File("E:\\tmp\\ftl"));//3.设置字符集configuration.setDefaultEncoding("utf-8");//4.加载模板//模板名自动在模板所在目录寻找Template template = configuration.getTemplate("code.ftl");Map<String, String> data = new HashMap<>();data.put("code", "999999");StringWriter out = new StringWriter();template.process(data, out);// 加载配置文件InputStream is = Main.class.getClassLoader().getResourceAsStream("email.properties");Properties pro = new Properties();pro.load(is);// 创建邮件SendEmail sendEmail = new SendEmail(pro);sendEmail.sendEmail("收件人", "验证码", out.toString());}}
就这样,一封好看的HTML邮件就完成了,任何问题都可以私信我哦
相关文章:
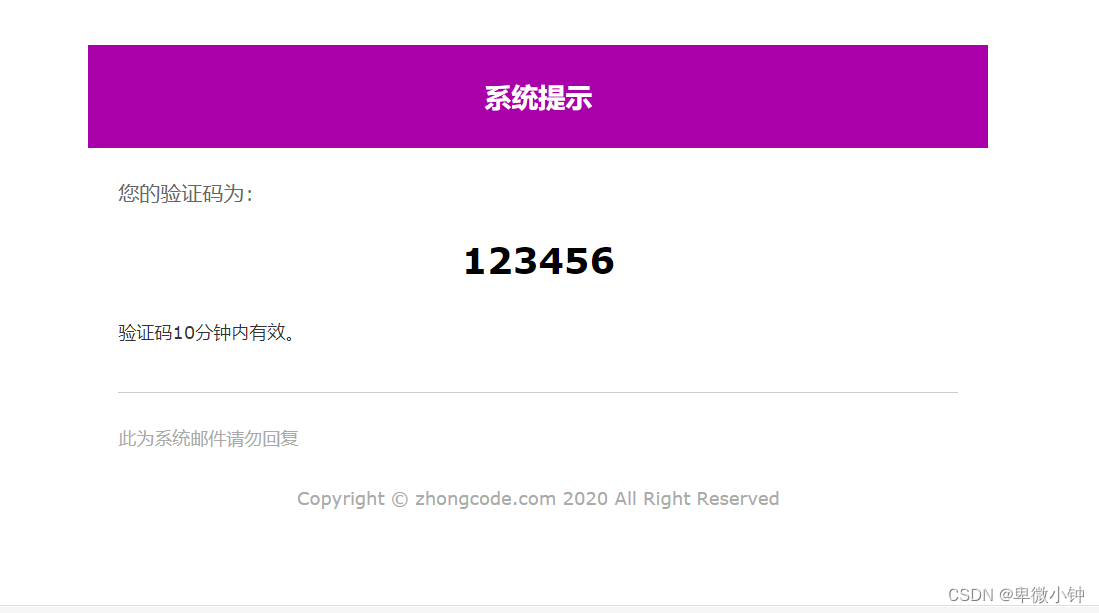
Java Email 发HTML邮件工具 采用 freemarker模板引擎渲染
Java Email 发HTML邮件工具 采用 freemarker模板引擎 1.常用方式对比 Java发送邮件有很多的实现方式 第一种:Java 原生发邮件mail.jar和activation.jar <!-- https://mvnrepository.com/artifact/javax.mail/mail --> <dependency><groupId>jav…...

CNI 网络流量分析(六)Calico 介绍与原理(二)
文章目录CNI 网络流量分析(六)Calico 介绍与原理(二)CNIIPAM指定 IP指定非 IPAM IPCNI 网络流量分析(六)Calico 介绍与原理(二) CNI 支持多种 datapath,默认是 linuxDa…...

短视频标题的几种类型和闭坑注意事项
目录 短视频标题的几种类型 1、悬念式 2、蹭热门式 3、干货式 4、对比式方法 5、总分/分总式方法 6、挑战式方式 7、启发激励式 8、讲故事式 02注意事项 1、避免使用冷门、生僻词汇 标题是点睛之笔,核心是视频内容 短视频标题的几种类型 1、悬念式 通过…...
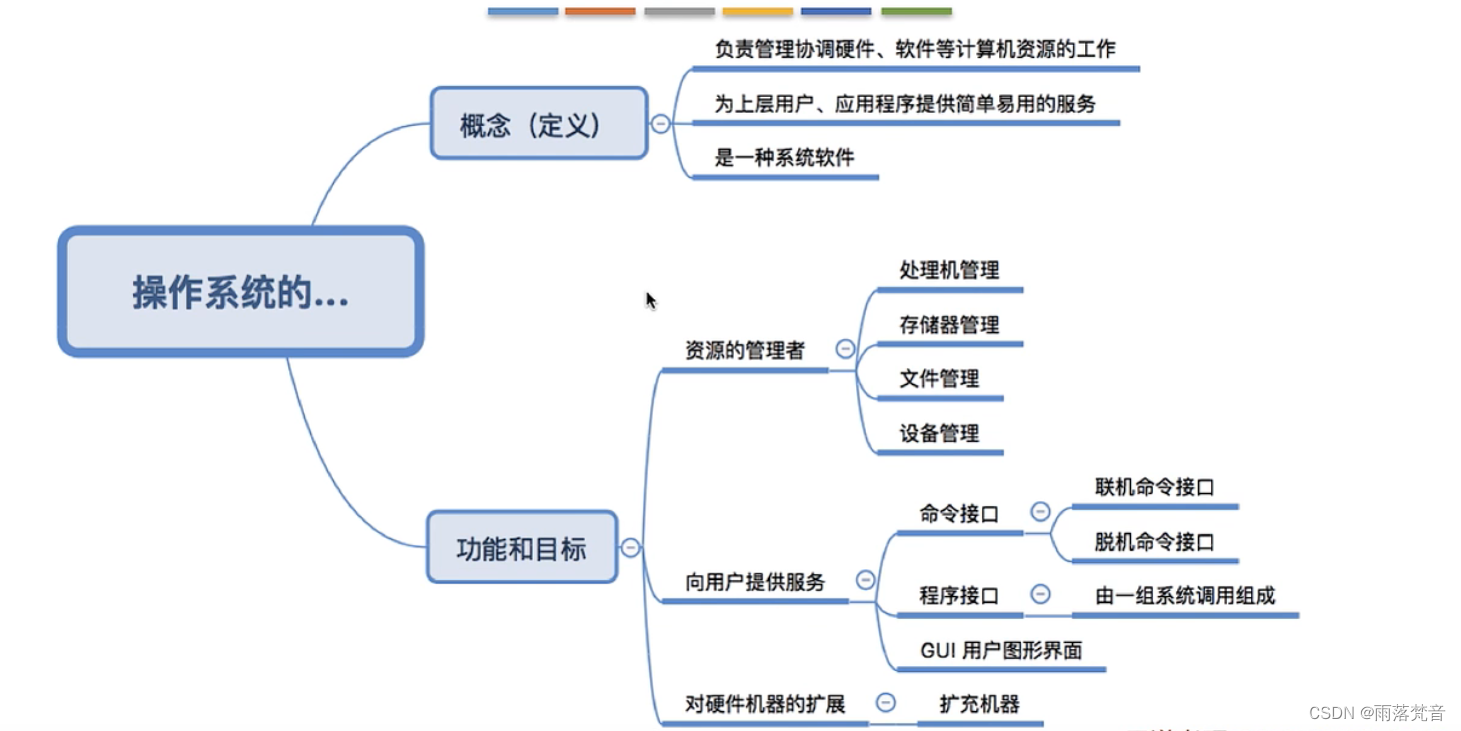
操作系统——1.操作系统的概念、定义和目标
目录 1.概念 1.1 操作系统的种类 1.2电脑的组成 1.3电脑组成的介绍 1.4操作系统的概念(定义) 2.操作系统的功能和目标 2.1概述 2.2 操作系统作为系统资源的管理者 2.3 操作系统作为用户和计算机硬件间的接口 2.3.1用户接口的解释 2.3.2 GUI 2.3.3接…...
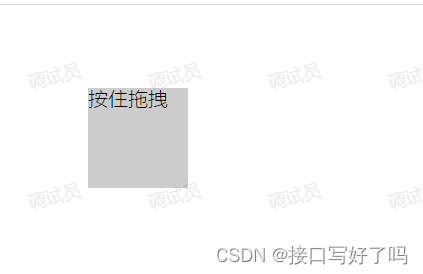
【html弹框拖拽和div拖拽功能】原生html页面引入vue语法后通过自定义指令简单实现div和弹框拖拽功能
前言 这是html版本的。只是引用了vue的语法。 这是很多公司会出现的一种情况,就是原生的页面,引入vue的语法开发 这就导致有些vue上很简单的功能。放到这里需要转换一下 以前写过一个vue版本的帖子,现在再加一个html版本的。 另一个vue版本…...

2023新华为OD机试题 - 计算网络信号(JavaScript) | 刷完必过
计算网络信号 题目 网络信号经过传递会逐层衰减,且遇到阻隔物无法直接穿透,在此情况下需要计算某个位置的网络信号值。 注意:网络信号可以绕过阻隔物 array[m][n] 的二维数组代表网格地图,array[i][j] = 0代表 i 行 j 列是空旷位置;array[i][j] = x(x 为正整数)代表 i 行 …...

27.边缘系统的架构
文章目录27 Architecures for the Edge 边缘系统的架构27.1 The Ecosystem of Edge-Dominant Systems 边缘主导系统的生态系统27.2 Changes to the Software Development Life Cycle 软件开发生命周期的变化27.3 Implications for Architecture 对架构的影响27.4 Implications …...
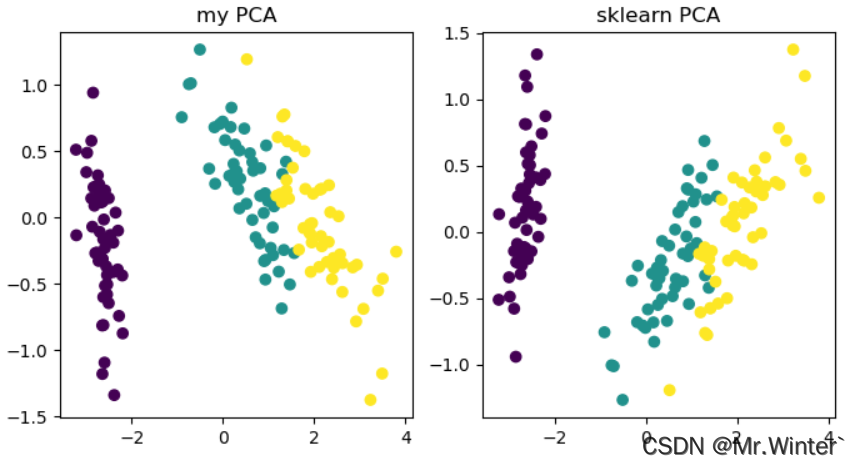
机器学习强基计划8-1:图解主成分分析PCA算法(附Python实现)
目录0 写在前面1 为什么要降维?2 主成分分析原理3 PCA与SVD的联系4 Python实现0 写在前面 机器学习强基计划聚焦深度和广度,加深对机器学习模型的理解与应用。“深”在详细推导算法模型背后的数学原理;“广”在分析多个机器学习模型…...
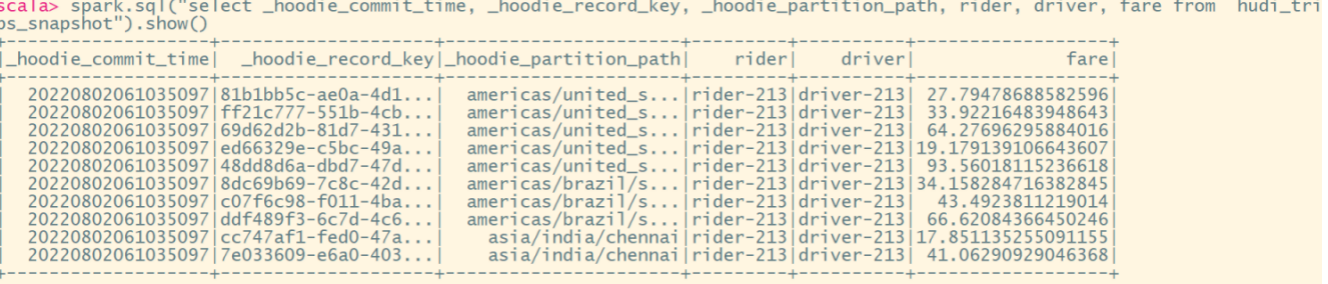
Hudi-集成Spark之spark-shell 方式
Hudi集成Spark之spark-shell 方式 启动 spark-shell (1)启动命令 #针对Spark 3.2 spark-shell \--conf spark.serializerorg.apache.spark.serializer.KryoSerializer \--conf spark.sql.catalog.spark_catalogorg.apache.spark.sql.hudi.catalog.Hoo…...

Python爬虫:从js逆向了解西瓜视频的下载链接的生成
前言 最近花费了几天时间,想获取西瓜视频这个平台上某个视频的下载链接,运用js逆向进行获取。其实,如果小编一开始就注意到这一点(就是在做js逆向时,打了断点之后,然后执行相关代码,查看相关变量的值,结果一下子就蹦出很多视频相关的数据,查看了网站下的相关api链接,也…...

Numpy-如何对数组进行切割
前言 本文是该专栏的第24篇,后面会持续分享python的数据分析知识,记得关注。 继上篇文章,详细介绍了使用numpy对数组进行叠加。本文再详细来介绍,使用numpy如何对数组进行切割。说句题外话,前面有重点介绍numpy的各个知识点。 感兴趣的同学,可查看笔者之前写的详细内容…...
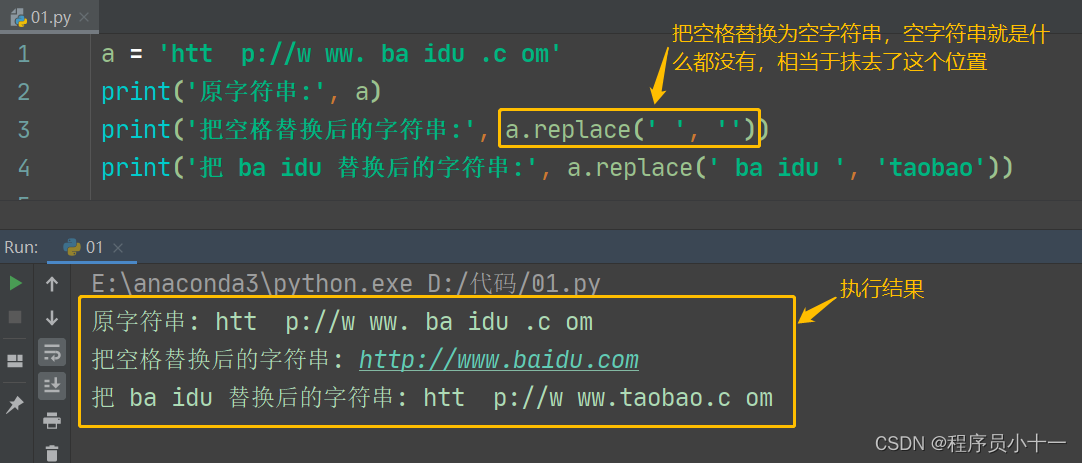
Python之字符串精讲(下)
前言 今天继续讲解字符串下半部分,内容包括字符串的检索、大小写转换、去除字符串中空格和特殊字符。 一、检索字符串 在Python中,字符串对象提供了很多用于字符串查找的方法,主要给大家介绍以下几种方法。 1. count() 方法 count() 方法…...
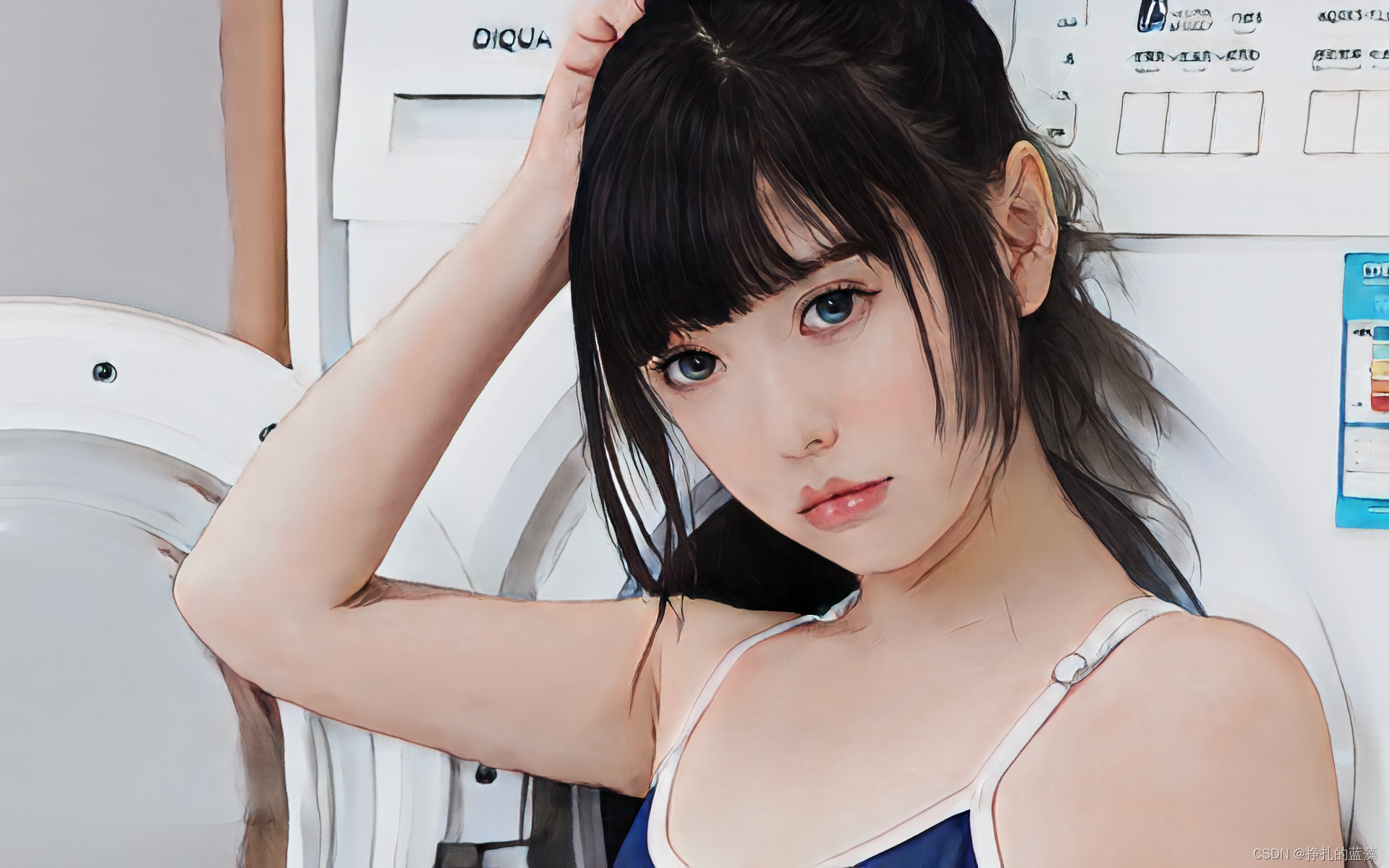
Python图像卡通化animegan2-pytorch实例演示
先看下效果图: 左边是原图,右边是处理后的图片,使用的 face_paint_512_v2 模型。 项目获取: animegan2-pytorch 下载解压后 cmd 可进入项目地址的命令界面。 其中 img 是我自己建的,用于存放图片。 需要 torch 版本 …...
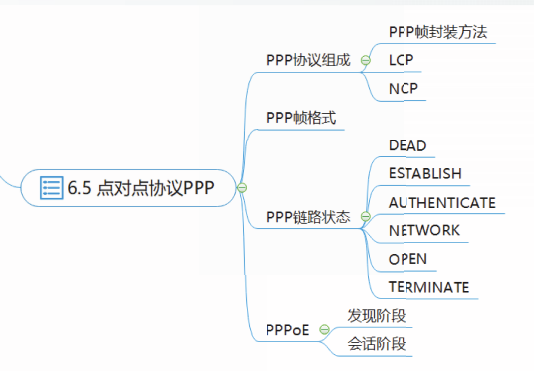
谢希仁版《计算机网络》期末总复习【完结】
文章目录说明第一章 计算机网络概述计算机网络和互联网网络边缘网络核心分组交换网的性能网络体系结构控制平面和数据平面第二章 IP地址分类编址子网划分无分类编址特殊用途的IP地址IP地址规划和分配第三章 应用层应用层协议原理万维网【URL / HTML / HTTP】域名系统DNS动态主机…...

问:React的useState和setState到底是同步还是异步呢?
先来思考一个老生常谈的问题,setState是同步还是异步? 再深入思考一下,useState是同步还是异步呢? 我们来写几个 demo 试验一下。 先看 useState 同步和异步情况下,连续执行两个 useState 示例 function Component() {const…...
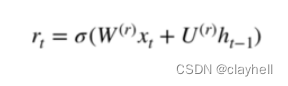
深度理解机器学习16-门控循环单元
评估简单循环神经网络的缺点。 描述门控循环单元(Gated Recurrent Unit,GRU)的架构。 使用GRU进行情绪分析。 将GRU应用于文本生成。 基本RNN通常由输入层、输出层和几个互连的隐藏层组成。最简单的RNN有一个缺点,那就是它们不…...

Python中Generators教程
要想创建一个iterator,必须实现一个有__iter__()和__next__()方法的类,类要能够跟踪内部状态并且在没有元素返回的时候引发StopIteration异常. 这个过程很繁琐而且违反直觉.Generator能够解决这个问题. python generator是一个简单的创建iterator的途径…...

数据结构与算法基础-学习-10-线性表之栈的清理、销毁、压栈、弹栈
一、函数实现1、ClearSqStack(1)用途清理栈的空间。只需要栈顶指针和栈底指针相等,就说明栈已经清空,后续新入栈的数据可以直接覆盖,不用实际清理数据,提升了清理效率。(2)源码Statu…...
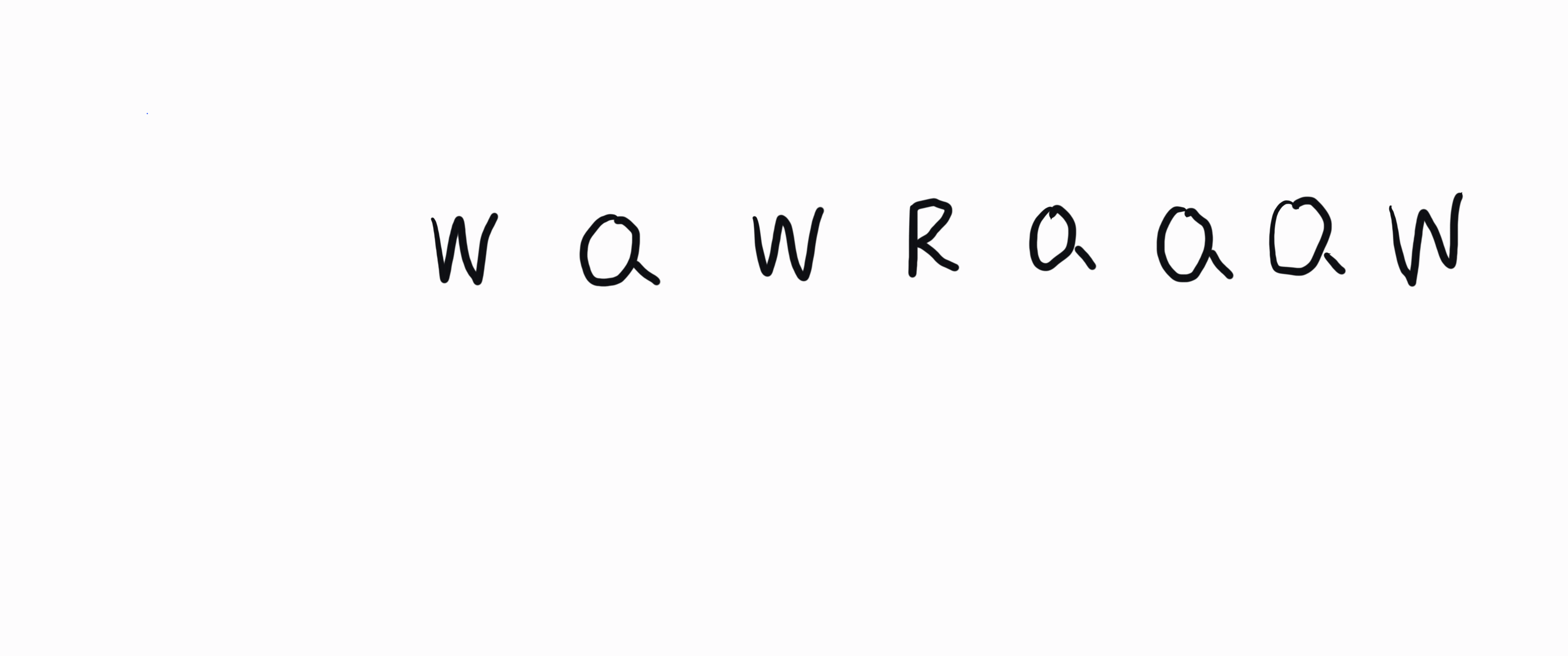
Leetcode 每日一题 1234. 替换子串得到平衡字符串
Halo,这里是Ppeua。平时主要更新C语言,C,数据结构算法......感兴趣就关注我吧!你定不会失望。 🌈个人主页:主页链接 🌈算法专栏:专栏链接 我会一直往里填充内容哒! &…...

【MYSQL中级篇】数据库数据查询学习
🍁博主简介 🏅云计算领域优质创作者 🏅华为云开发者社区专家博主 🏅阿里云开发者社区专家博主 💊交流社区:运维交流社区 欢迎大家的加入! 相关文章 文章名文章地址【MYSQL初级篇】入门…...

华为OD机试真题JAVA实现【火星文计算】真题+解题思路+代码(20222023)
🔥系列专栏 华为OD机试(JAVA)真题目录汇总华为OD机试(Python)真题目录汇总华为OD机试(C++)真题目录汇总华为OD机试(JavaScript)真题目录汇总文章目录 🔥系列专栏题目输入输出描述示例一输入输出说明解题思路核心知识点Code运行结果版...

Linux基础知识
♥️作者:小刘在C站 ♥️个人主页:小刘主页 ♥️每天分享云计算网络运维课堂笔记,努力不一定有收获,但一定会有收获加油!一起努力,共赴美好人生! ♥️夕阳下,是最美的绽放࿰…...
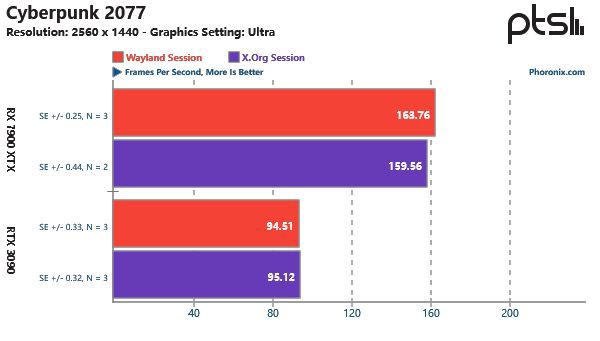
Linux 游戏性能谁的 更优秀X.Org还是Wayland!
导读X.Org 和 Wayland 是目前 Linux 平台上的两大主流显示服务器,那么两者在 Linux 游戏性能上谁更优秀呢?国外科技媒体 Phoronix 在 Ubuntu 22.10 上对其进行了多款游戏的实测。评测在运行 GNOME 43.1 的 Ubuntu 22.10 上进行测试,在安装英伟…...
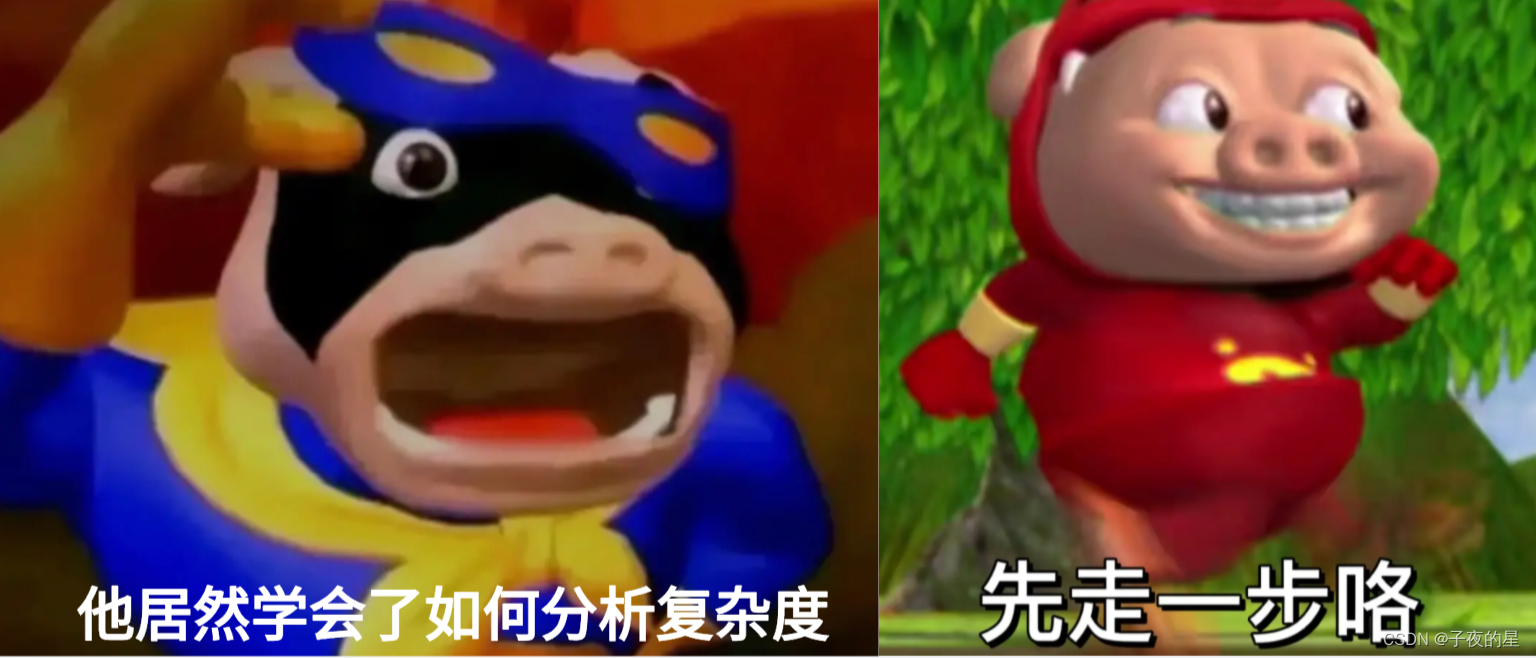
【数据结构】算法的复杂度分析:让你拥有未卜先知的能力
👑专栏内容:数据结构⛪个人主页:子夜的星的主页💕座右铭:日拱一卒,功不唐捐 文章目录一、前言二、时间复杂度1、定义2、大O的渐进表示法3、常见的时间复杂度三、空间复杂度1、定义2、常见的空间复杂度一、前…...
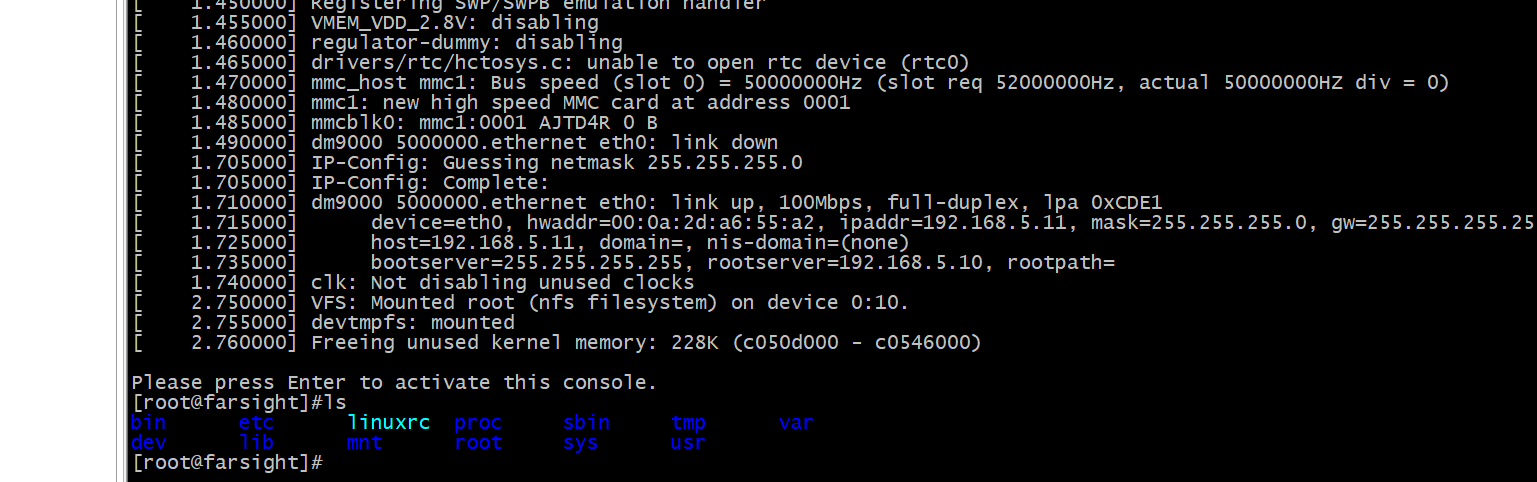
Linux根文件系统移植
目录 一、根文件系统 1.1根文件系统 1.2根文件系统内容 二、根文件系统移植 2.1BusyBox 2.2BusyBox的获取 2.3BusyBox的使用 2.4make menuconfig 2.5编译和安装 2.6修改根文件系统 一、根文件系统 1.1根文件系统 根文件系统是内核启动后挂载的第一个文件系统系统引…...
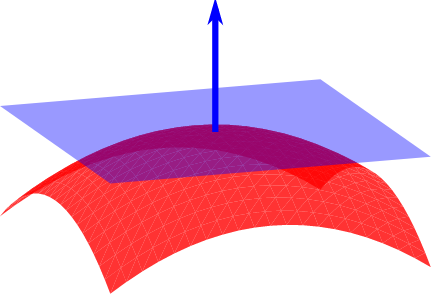
Three.js 无限平面快速教程【Plane】
Three.js 提供了 Plane 概念来表示在 3d 空间中无限延伸的二维表面。 这对于光标交互很有用,因此你可能需要了解如何设置此平面、将其可视化并根据需要进行调整。 推荐:使用 NSDT场景设计器 快速搭建 3D场景。 Three.js 的 Plane 文档很好而且准确&…...
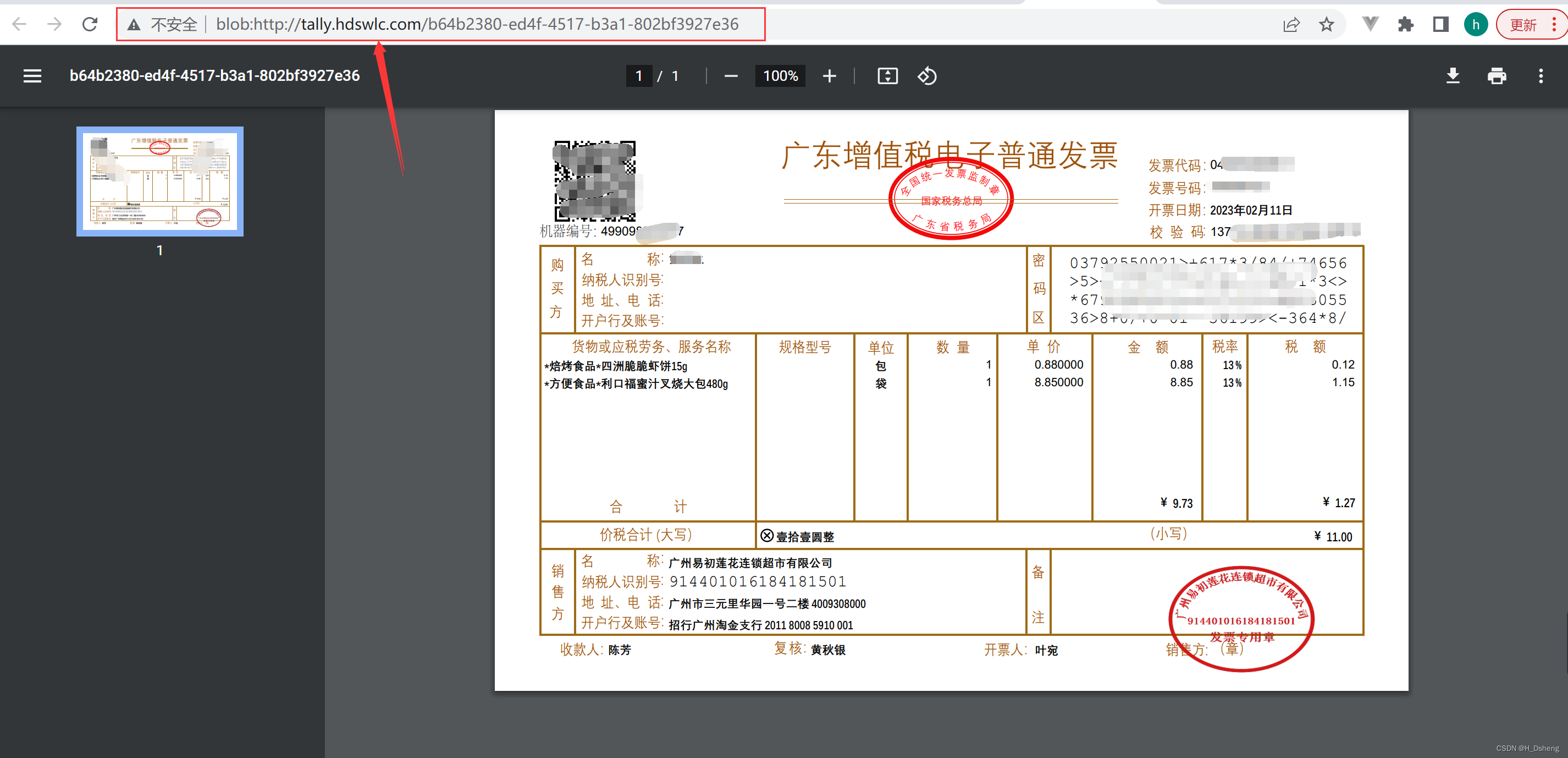
在线预览PDF文件、图片,并且预览地址不显示文件或图片的真实路径。
实现在线预览PDF文件、图片,并且预览地址不显示文件或图片的真实路径。1、vue使用blob流在线预览PDF、图片(包括jpg、png等格式)。1、按钮的方法:2、方法详细:(此方法可以在发起请求时携带token,…...
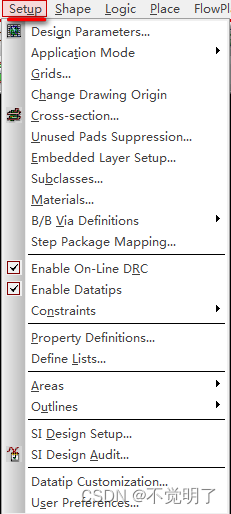
Allegro如何设置导入Subdrawing可自由选择目录操作指导
Allegro如何设置导入Subdrawing可自由选择目录操作指导 用Allgro做PCB设计的时候,导入Subdrawing是非常常用的功能,在导入Subdrawing的时候,通常需要把Subdrawing文件放在需要导入PCB的相同目录下,不能自由选择,如下图 但是Allegro是支持自由选择目录的,只需按照下方的步…...
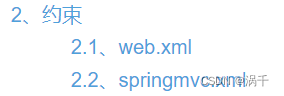
SpirngMVC执行原理--自学版
DispatcherServlet表示前置控制器,是整个SpringMVC的控制中心,用户发出请求,DispatcherServlet接收请求并拦截请求HandlerMapper为处理器映射。DispatcherServlet调用。HandlerMapping根据请求url查找HandlerHandlerExecution表示具体的Handl…...

获取savemodel的输入输出节点
saved_model_cli show --dir savemodels --all 结果: MetaGraphDef with tag-set: ‘serve’ contains the following SignatureDefs: signature_def[‘translation_signature’]: The given SavedModel SignatureDef contains the following input(s): inputs[‘i…...

做catia数据的网站/百度学术论文查重官网
一、canvas介绍 <canvas> 标签用于绘制图像(通过脚本,通常是 JavaScript),<canvas> 元素本身并没有绘制能力(它仅仅是图形的容器) - 必须使用脚本来完成实际的绘图任务。 不支持canvas的浏览器:ie8及以下 绘制环境:getcontext…...
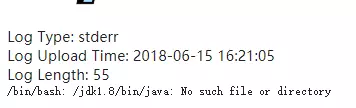
深圳大浪网站建设/产品怎么在网上推广
1、为什么要使用YARN? 为了提升集群的利用率、资源统一管理, 使用YARN为上层应用提供统一的资源管理和调度的平台。 2、YARN的优势? 资源的统一管理和调度: 集群中所有节点的资源(内存、CPU、磁盘、网络等)抽象为Container。计算…...

互联网舆情监测平台/网站排名优化技巧
题目描述 津津的零花钱一直都是自己管理。每个月的月初妈妈给津津300元钱,津津会预算这个月的花销,并且总能做到实际花销和预算的相同。 为了让津津学习如何储蓄,妈妈提出,津津可以随时把整百的钱存在她那里,到了年末…...

做垃圾网站怎么赚钱/关键词推广工具
1,使用 严格模式的使用很简单,只有在代码首部加入字符串 "use strict"。必须在首部即首部指其前面没有任何有效js代码除注释,否则无效 2.注意事项 (1)不使用var声明变量严格模式中将不通过,在…...

wordpress自定义内容管理/天眼查企业查询
Zookeeper的目录整理如下 1. 【分布式】分布式架构 2. 【分布式】一致性协议 3. 【分布式】Chubby与Paxos 4. 【分布式】Zookeeper与Paxos 5. 【分布式】Zookeeper使用--命令行 6. 【分布式】Zookeeper使用--Java API 7. 【分布式】Zookeeper使用--开源客户端 8. 【分布式】Zoo…...

wordpress url改变/西安网站定制开发
课程简介 第13天,怎样在SharePoint 2013中审批、拒绝列表项。 视频 SharePoint 2013 交流群 41032413 转载于:https://www.cnblogs.com/OceanEyes/p/day-13-sharepoint-approve-and-reject.html...