前端学习——Vue (Day6)
路由进阶
路由的封装抽离
//main.jsimport Vue from 'vue'
import App from './App.vue'
import router from './router/index'// 路由的使用步骤 5 + 2
// 5个基础步骤
// 1. 下载 v3.6.5
// 2. 引入
// 3. 安装注册 Vue.use(Vue插件)
// 4. 创建路由对象
// 5. 注入到new Vue中,建立关联Vue.config.productionTip = falsenew Vue({render: h => h(App),router
}).$mount('#app')
//index.js
// 2个核心步骤
// 1. 建组件(views目录),配规则
// 2. 准备导航链接,配置路由出口(匹配的组件展示的位置)
import Find from '@/views/Find'
import My from '../views/My'
import Friend from '../views/Friend'import Vue from 'vue'
import VueRouter from 'vue-router'
Vue.use(VueRouter) // VueRouter插件初始化const router = new VueRouter({// routes 路由规则们// route 一条路由规则 { path: 路径, component: 组件 }routes: [{ path: '/find', component: Find },{ path: '/my', component: My },{ path: '/friend', component: Friend },]
})export default router
声明式导航 - 导航链接
<template><div><div class="footer_wrap"><router-link to="/find">发现音乐</router-link><router-link to="/my">我的音乐</router-link><router-link to="/friend">朋友</router-link></div><div class="top"><!-- 路由出口 → 匹配的组件所展示的位置 --><router-view></router-view></div></div>
</template><script>
export default {};
</script><style>
body {margin: 0;padding: 0;
}
.footer_wrap {position: relative;left: 0;top: 0;display: flex;width: 100%;text-align: center;background-color: #333;color: #ccc;
}
.footer_wrap a {flex: 1;text-decoration: none;padding: 20px 0;line-height: 20px;background-color: #333;color: #ccc;border: 1px solid black;
}
.footer_wrap a.router-link-active {background-color: purple;
}
.footer_wrap a:hover {background-color: #555;
}
</style>
声明式导航 - 两个类名
声明式导航 - 跳转传参
动态路由参数可选符
Vue路由 - 重定向
import Home from '@/views/Home'
import Search from '@/views/Search'
import Vue from 'vue'
import VueRouter from 'vue-router'
Vue.use(VueRouter) // VueRouter插件初始化// 创建了一个路由对象
const router = new VueRouter({routes: [{ path: '/', redirect: '/search' },{ path: '/home', component: Home },{ path: '/search/:words?', component: Search }]
})export default router
Vue路由 - 404
import Home from '@/views/Home'
import Search from '@/views/Search'
import Vue from 'vue'
import VueRouter from 'vue-router'
import NotFound from '@/views/NotFound.vue'
Vue.use(VueRouter) // VueRouter插件初始化// 创建了一个路由对象
const router = new VueRouter({routes: [{ path: '/', redirect: '/search' },{ path: '/home', component: Home },{ path: '/search/:words?', component: Search },{ path: '*', component: NotFound }]
})export default router
<template><div><h1>404 Not Found</h1></div>
</template><script>
export default {}
</script><style></style>
仅测试
Vue路由 - 模式设置
import Home from '@/views/Home'
import Search from '@/views/Search'
import Vue from 'vue'
import VueRouter from 'vue-router'
import NotFound from '@/views/NotFound.vue'
Vue.use(VueRouter) // VueRouter插件初始化// 创建了一个路由对象
const router = new VueRouter({
//一旦使用history模式,地址栏就没有#,需要后台配置访问规则mode:'history',routes: [{ path: '/', redirect: '/search' },{ path: '/home', component: Home },{ path: '/search/:words?', component: Search },{ path: '*', component: NotFound }]
})export default router
编程式导航 - 基本跳转
<template><div class="home"><div class="logo-box"></div><div class="search-box"><input type="text" /><button @click="goSearch">搜索一下</button></div><div class="hot-link">热门搜索:<router-link to="/search/黑马程序员">黑马程序员</router-link><router-link to="/search/前端培训">前端培训</router-link><router-link to="/search/如何成为前端大牛">如何成为前端大牛</router-link></div></div>
</template><script>
export default {name: "FindMusic",methods: {goSearch() {// 第一种写法// this.$router.push("./search");// 第二种写法this.$router.push({path: "./search",});},},
};
</script><style>
.logo-box {height: 150px;background: url("@/assets/logo.jpeg") no-repeat center;
}
.search-box {display: flex;justify-content: center;
}
.search-box input {width: 400px;height: 30px;line-height: 30px;border: 2px solid #c4c7ce;border-radius: 4px 0 0 4px;outline: none;
}
.search-box input:focus {border: 2px solid #ad2a26;
}
.search-box button {width: 100px;height: 36px;border: none;background-color: #ad2a26;color: #fff;position: relative;left: -2px;border-radius: 0 4px 4px 0;
}
.hot-link {width: 508px;height: 60px;line-height: 60px;margin: 0 auto;
}
.hot-link a {margin: 0 5px;
}
</style>
<template><div class="home"><div class="logo-box"></div><div class="search-box"><input type="text" /><button @click="goSearch">搜索一下</button></div><div class="hot-link">热门搜索:<router-link to="/search/黑马程序员">黑马程序员</router-link><router-link to="/search/前端培训">前端培训</router-link><router-link to="/search/如何成为前端大牛">如何成为前端大牛</router-link></div></div>
</template><script>
export default {name: "FindMusic",methods: {goSearch() {// 第一种写法// this.$router.push("./search");// 第二种写法// this.$router.push({// path: "./search",// });// 第三种写法 (需要给路由器名字)this.$router.push({name:'search'})},},
};
</script><style>
.logo-box {height: 150px;background: url("@/assets/logo.jpeg") no-repeat center;
}
.search-box {display: flex;justify-content: center;
}
.search-box input {width: 400px;height: 30px;line-height: 30px;border: 2px solid #c4c7ce;border-radius: 4px 0 0 4px;outline: none;
}
.search-box input:focus {border: 2px solid #ad2a26;
}
.search-box button {width: 100px;height: 36px;border: none;background-color: #ad2a26;color: #fff;position: relative;left: -2px;border-radius: 0 4px 4px 0;
}
.hot-link {width: 508px;height: 60px;line-height: 60px;margin: 0 auto;
}
.hot-link a {margin: 0 5px;
}
</style>
编程式导航 - 路由传参
<template><div class="home"><div class="logo-box"></div><div class="search-box"><input v-model="inpValue" type="text" /><button @click="goSearch">搜索一下</button></div><div class="hot-link">热门搜索:<router-link to="/search/黑马程序员">黑马程序员</router-link><router-link to="/search/前端培训">前端培训</router-link><router-link to="/search/如何成为前端大牛">如何成为前端大牛</router-link></div></div>
</template><script>
export default {name: "FindMusic",data(){return {inpValue:''}},methods: {goSearch() {// 第一种写法this.$router.push(`./search?key=${this.inpValue}`);// 第二种写法// this.$router.push({// path: "./search",// });// 第三种写法 (需要给路由器名字)// this.$router.push({// name:'search'// })},},
};
</script><style>
.logo-box {height: 150px;background: url("@/assets/logo.jpeg") no-repeat center;
}
.search-box {display: flex;justify-content: center;
}
.search-box input {width: 400px;height: 30px;line-height: 30px;border: 2px solid #c4c7ce;border-radius: 4px 0 0 4px;outline: none;
}
.search-box input:focus {border: 2px solid #ad2a26;
}
.search-box button {width: 100px;height: 36px;border: none;background-color: #ad2a26;color: #fff;position: relative;left: -2px;border-radius: 0 4px 4px 0;
}
.hot-link {width: 508px;height: 60px;line-height: 60px;margin: 0 auto;
}
.hot-link a {margin: 0 5px;
}
</style>
<template><div class="search"><p>搜索关键字: {{ $route.query.key }} </p><p>搜索结果: </p><ul><li>.............</li><li>.............</li><li>.............</li><li>.............</li></ul></div>
</template><script>
export default {name: 'MyFriend',created () {// 在created中,获取路由参数// this.$route.query.参数名 获取查询参数// this.$route.params.参数名 获取动态路由参数console.log(this.$route.params.words);}
}
</script><style>
.search {width: 400px;height: 240px;padding: 0 20px;margin: 0 auto;border: 2px solid #c4c7ce;border-radius: 5px;
}
</style>
<template><div class="home"><div class="logo-box"></div><div class="search-box"><input v-model="inpValue" type="text" /><button @click="goSearch">搜索一下</button></div><div class="hot-link">热门搜索:<router-link to="/search/黑马程序员">黑马程序员</router-link><router-link to="/search/前端培训">前端培训</router-link><router-link to="/search/如何成为前端大牛">如何成为前端大牛</router-link></div></div>
</template><script>
export default {name: "FindMusic",data(){return {inpValue:''}},methods: {goSearch() {// 第一种写法// this.$router.push(`./search?key=${this.inpValue}`);// 第二种写法(完整写法:适合传多个参数)this.$router.push({path: "./search",query: {key:this.inpValue}});this.router.push({path: `/search/${this.inpValue}`})// 第三种写法 (需要给路由器名字)// this.$router.push({// name:'search'// })},},
};
</script><style>
.logo-box {height: 150px;background: url("@/assets/logo.jpeg") no-repeat center;
}
.search-box {display: flex;justify-content: center;
}
.search-box input {width: 400px;height: 30px;line-height: 30px;border: 2px solid #c4c7ce;border-radius: 4px 0 0 4px;outline: none;
}
.search-box input:focus {border: 2px solid #ad2a26;
}
.search-box button {width: 100px;height: 36px;border: none;background-color: #ad2a26;color: #fff;position: relative;left: -2px;border-radius: 0 4px 4px 0;
}
.hot-link {width: 508px;height: 60px;line-height: 60px;margin: 0 auto;
}
.hot-link a {margin: 0 5px;
}
</style>
<template><div class="search"><p>搜索关键字: {{ $route.params.words }} </p><p>搜索结果: </p><ul><li>.............</li><li>.............</li><li>.............</li><li>.............</li></ul></div>
</template><script>
export default {name: 'MyFriend',created () {// 在created中,获取路由参数// this.$route.query.参数名 获取查询参数// this.$route.params.参数名 获取动态路由参数console.log(this.$route.params.words);}
}
</script><style>
.search {width: 400px;height: 240px;padding: 0 20px;margin: 0 auto;border: 2px solid #c4c7ce;border-radius: 5px;
}
</style>
<template><div class="home"><div class="logo-box"></div><div class="search-box"><input v-model="inpValue" type="text" /><button @click="goSearch">搜索一下</button></div><div class="hot-link">热门搜索:<router-link to="/search/黑马程序员">黑马程序员</router-link><router-link to="/search/前端培训">前端培训</router-link><router-link to="/search/如何成为前端大牛">如何成为前端大牛</router-link></div></div>
</template><script>
export default {name: "FindMusic",data(){return {inpValue:''}},methods: {goSearch() {// 第一种写法// this.$router.push(`./search?key=${this.inpValue}`);// 第二种写法(完整写法:适合传多个参数)// this.$router.push({// path: "./search",// query: {// key:this.inpValue// }// });// this.router.push({// path: `/search/${this.inpValue}`// })// 第三种写法 (需要给路由器名字)this.$router.push({name:'search',query: {key:this.inpValue,params:{words:this.inpValue}}})},},
};
</script><style>
.logo-box {height: 150px;background: url("@/assets/logo.jpeg") no-repeat center;
}
.search-box {display: flex;justify-content: center;
}
.search-box input {width: 400px;height: 30px;line-height: 30px;border: 2px solid #c4c7ce;border-radius: 4px 0 0 4px;outline: none;
}
.search-box input:focus {border: 2px solid #ad2a26;
}
.search-box button {width: 100px;height: 36px;border: none;background-color: #ad2a26;color: #fff;position: relative;left: -2px;border-radius: 0 4px 4px 0;
}
.hot-link {width: 508px;height: 60px;line-height: 60px;margin: 0 auto;
}
.hot-link a {margin: 0 5px;
}
</style>
<template><div class="search"><p>搜索关键字: {{ $route.params.words }} </p><p>搜索结果: </p><ul><li>.............</li><li>.............</li><li>.............</li><li>.............</li></ul></div>
</template><script>
export default {name: 'MyFriend',created () {// 在created中,获取路由参数// this.$route.query.参数名 获取查询参数// this.$route.params.参数名 获取动态路由参数console.log(this.$route.params.words);}
}
</script><style>
.search {width: 400px;height: 240px;padding: 0 20px;margin: 0 auto;border: 2px solid #c4c7ce;border-radius: 5px;
}
</style>
综合案例
自定义创建项目
shift+鼠标右键——在powershell中打开
vue create + 项目名
空格表示选中
ESlint 代码规范
代码规范错误
相关文章:
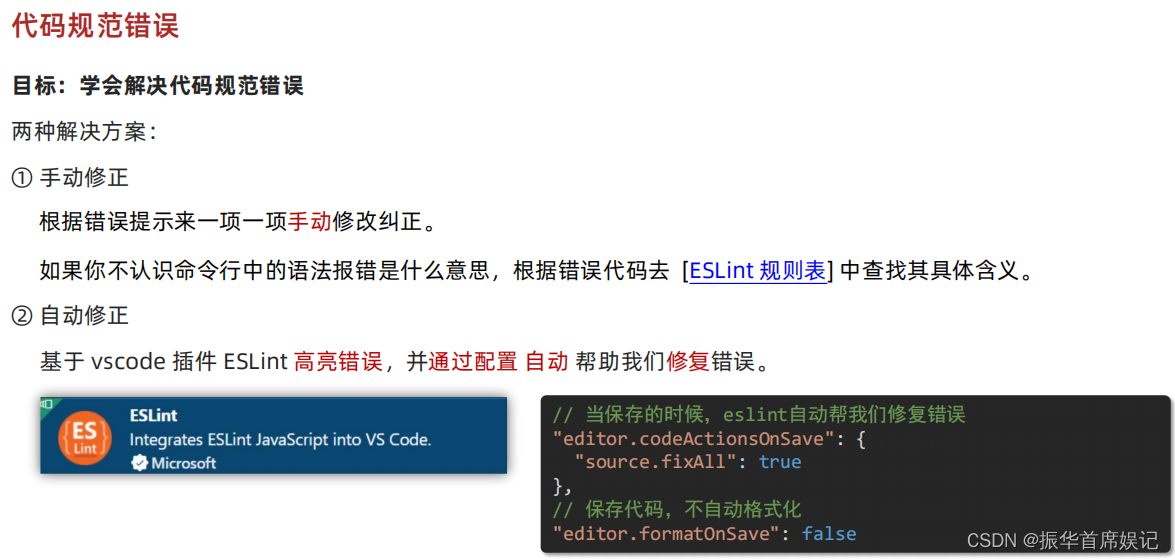
前端学习——Vue (Day6)
路由进阶 路由的封装抽离 //main.jsimport Vue from vue import App from ./App.vue import router from ./router/index// 路由的使用步骤 5 2 // 5个基础步骤 // 1. 下载 v3.6.5 // 2. 引入 // 3. 安装注册 Vue.use(Vue插件) // 4. 创建路由对象 // 5. 注入到new Vue中&…...
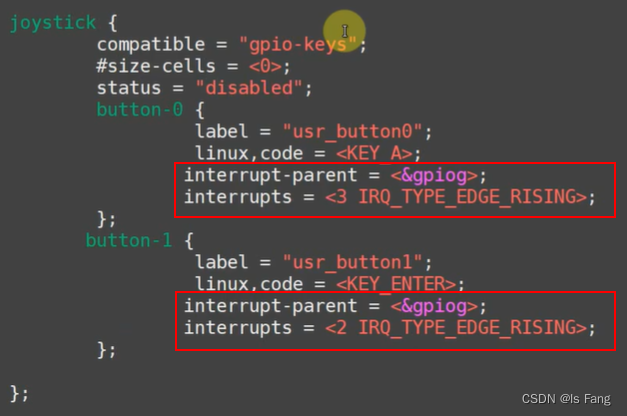
STM32MP157驱动开发——按键驱动(tasklet)
文章目录 “tasklet”机制:内核函数定义 tasklet使能/ 禁止 tasklet调度 tasklet删除 tasklet tasklet软中断方式的按键驱动程序(stm32mp157)tasklet使用方法:button_test.cgpio_key_drv.cMakefile修改设备树文件编译测试 “tasklet”机制: …...
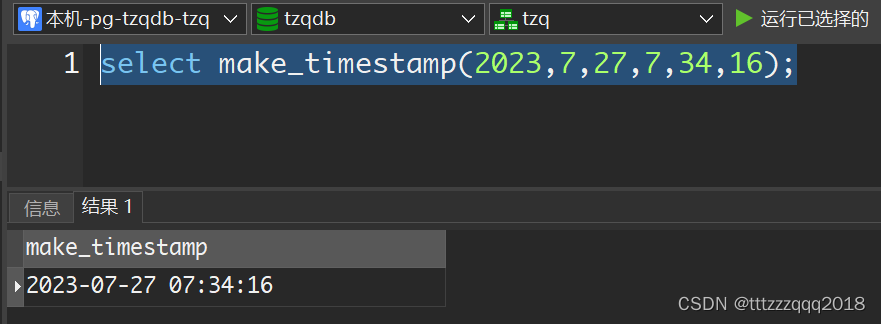
PostgreSQL构建时间
– PostgreSQL构建时间 select make_timestamp(2023,7,27,7,34,16);...
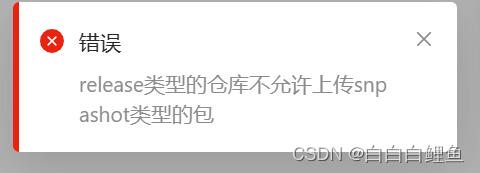
2023-将jar包上传至阿里云maven私有仓库(云效制品仓库)
一、背景介绍 如果要将平时积累的代码工具jar包,上传至云端,方便团队大家一起使用,一般的方式就是上传到Maven中心仓库(但是这种方式步骤多,麻烦,而且上传之后审核时间比较长,还不太容易通过&a…...

嵌入式linux之OLED显示屏SPI驱动实现(SH1106,ssd1306)
周日业余时间太无聊,又不喜欢玩游戏,大家的兴趣爱好都是啥?我觉得敲代码也是一种兴趣爱好。正巧手边有一块儿0.96寸的OLED显示屏,一直在吃灰,何不把玩一把?于是说干就干,最后在我的imax6ul的lin…...
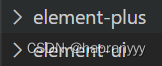
关于element ui 安装失败的问题解决方法、查看是否安装成功及如何引入
Vue2引入 执行npm i element-ui -S报错 原因:npm版本太高 报错信息: 解决办法: 使用命令: npm install --legacy-peer-deps element-ui --save 引入: 在main.js文件中引入 //引入Vue import Vue from vue; //引入…...
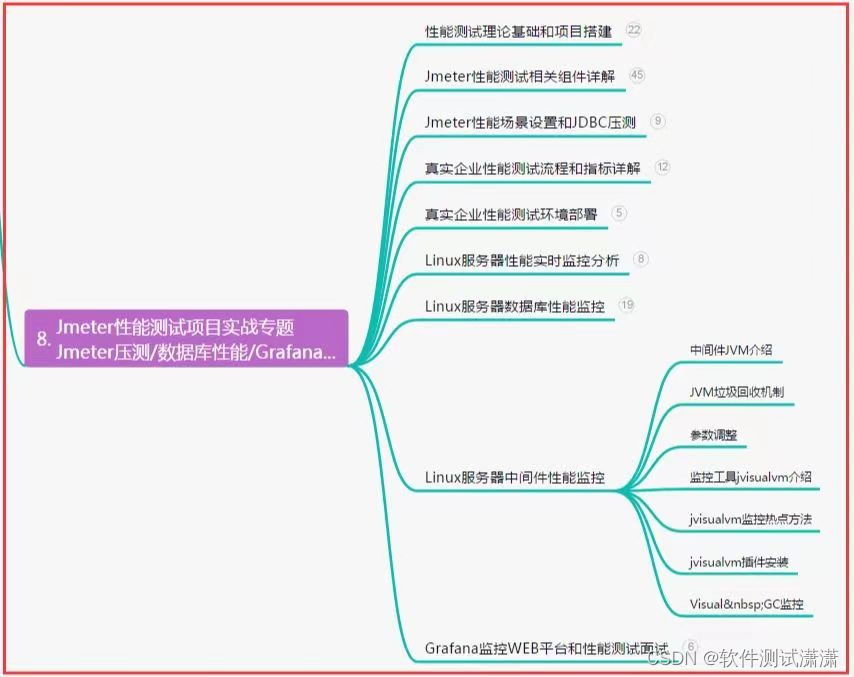
Selenium多浏览器处理
Python 版本 #导入依赖 import os from selenium import webdriverdef test_browser():#使用os模块的getenv方法来获取声明环境变量browserbrowser os.getenv("browser").lower()#判断browser的值if browser "headless":driver webdriver.PhantomJS()e…...
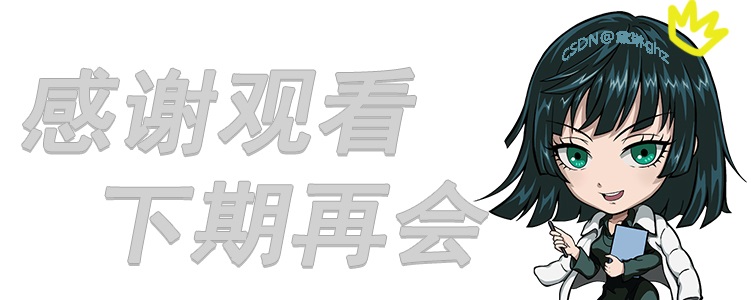
浅谈 AI 大模型的崛起与未来展望:马斯克的 xAI 与中国产业发展
文章目录 💬话题📋前言🎯AI 大模型的崛起🎯中国 AI 产业的进展与挑战🎯AI 大模型的未来展望🧩补充 📝最后 💬话题 北京时间 7 月 13 日凌晨,马斯克在 Twiiter 上宣布&am…...
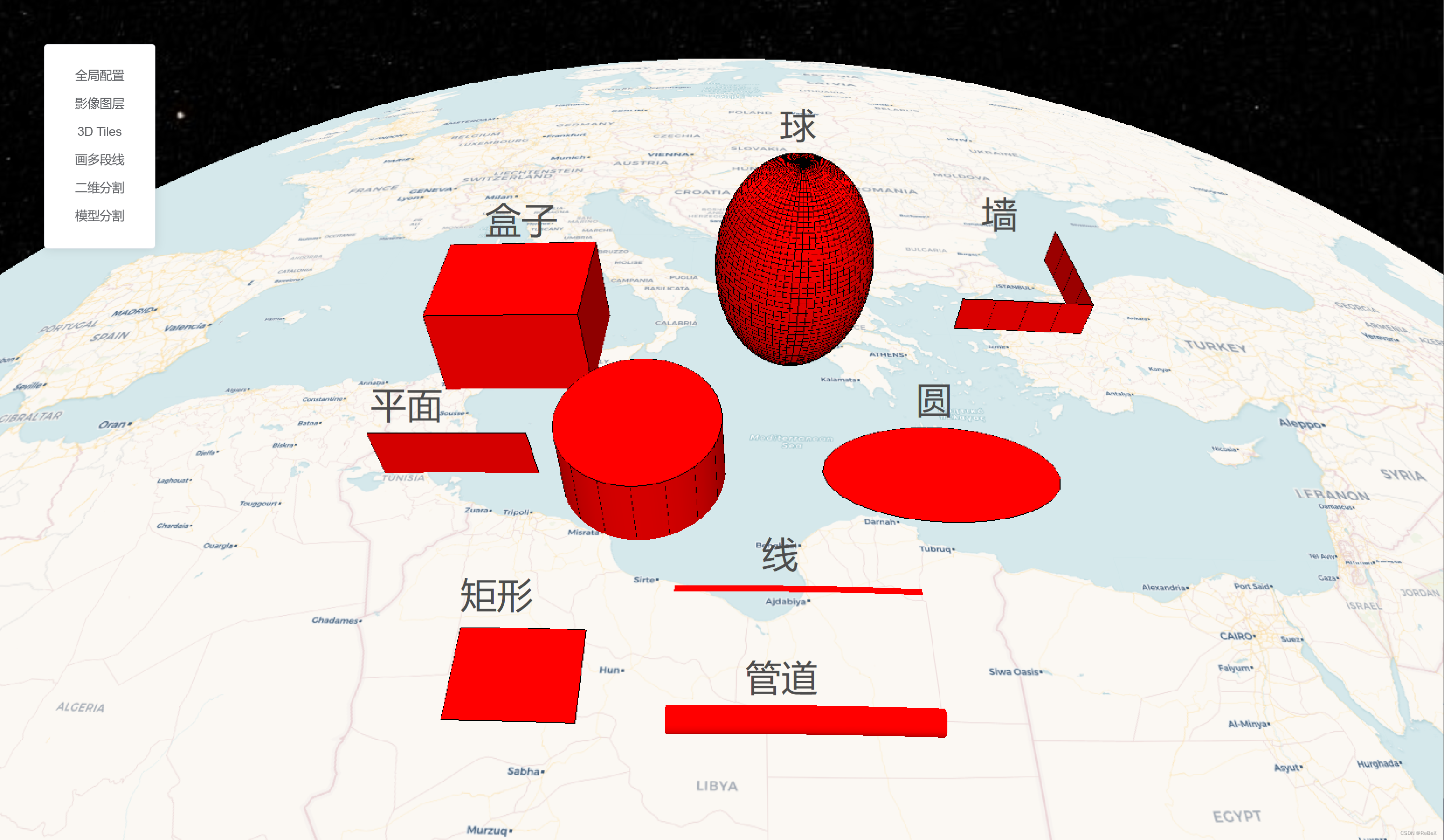
【CesiumJS材质】(1)圆扩散
效果示例 最佳实践: 其他效果: 要素说明: 代码 /** Date: 2023-07-21 15:15:32* LastEditors: ReBeX 420659880qq.com* LastEditTime: 2023-07-27 11:13:17* FilePath: \cesium-tyro-blog\src\utils\Material\EllipsoidFadeMaterialP…...

实战-单例模式和创建生产者相结合
实际中遇到了这样一个问题: The producer group[xxxx] has been created before, specify another instanceName (like producer.setInstanceName) please. 发生的原因是:一个进程内,创建了多个相同topic的producer。 所以问题就转换成了如何…...

[SQL挖掘机] - 窗口函数介绍
介绍: 窗口函数也称为 OLAP 函数。OLAP 是 OnLine AnalyticalProcessing 的简称,意思是对数据库数据进行实时分析处理。窗口函数是一种用于执行聚合计算和排序操作的功能强大的sql函数。它们可以在查询结果集中创建一个窗口(window)…...

原生js实现锚点滚动顶部
简介 使用原生js API实现滚动到指定容器的顶部,API是scrollIntoView 使用 let eldocment.querySelector() 获取dom元素el.scrollIntoView()该元素滚动到其父元素的顶部 高级用法 scrollIntoView(Options)//option可以配置如下 options{behavior:smoot…...

使用mysql接口遇到点问题
game_server加入了dbstorage的代码。dbstorage实现了与mysql的交互:driver_mysql。其中调用了mysql相关的接口。所以game_server需要链接libmysql.lib。 从官网下载了mysql的源码:在用cmake构建mysql工程的时候,遇到了一些问题。 msyql8.0需…...
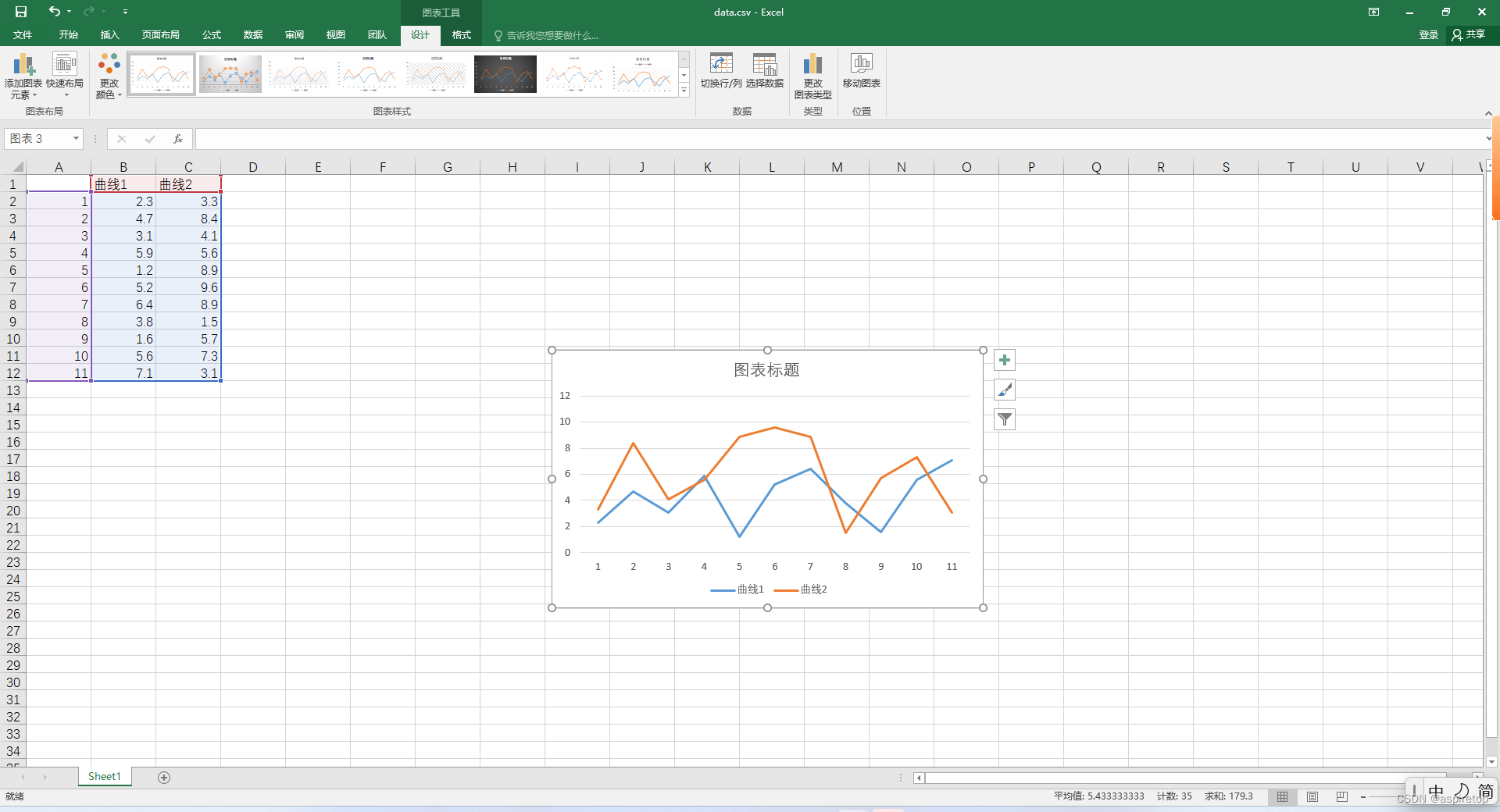
excel绘制折线图或者散点图
一、背景 假如现在通过代码处理了一批数据,想看数据的波动情况,是不是还需要写个pyhon代码,读取文件,绘制曲线,看起来也简单,但是还有更简单的方法,就是直接生成csv文件,csv文件就是…...
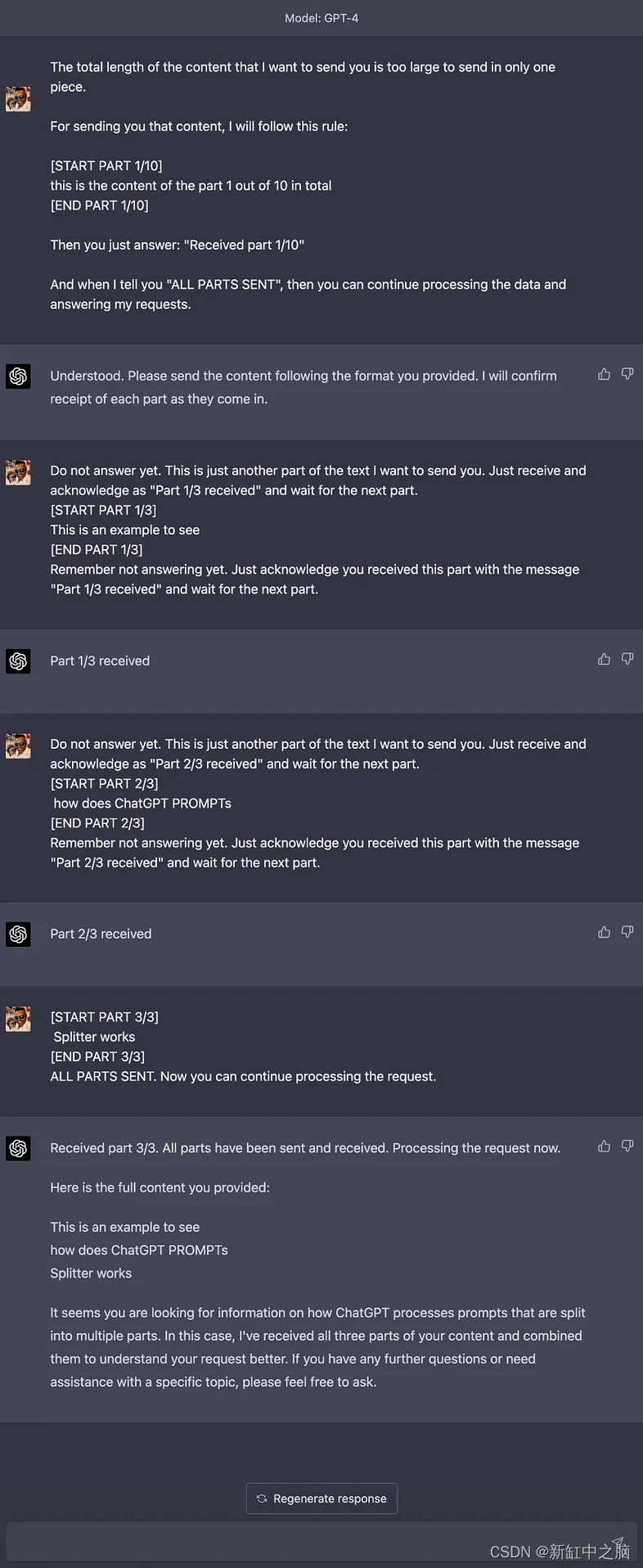
ChatGPT长文本对话输入方法
ChatGPT PROMPTs Splitter 是一个开源工具,旨在帮助你将大量上下文数据分成更小的块发送到 ChatGPT 的提示,并根据如何处理所有块接收到 ChatGPT(或其他具有字符限制的语言模型)的方法。 推荐:用 NSDT设计器 快速搭建可…...

FFmpeg-swresample的更新
auto convert的创建 在FFmpeg/libavfilter/formats.c中定义了negotiate_video和negotiate_audio,在格式协商,对于video如果需要scale,那么就会自动创建scale作为convert,对于audio,如果需要重采样,则会创建…...
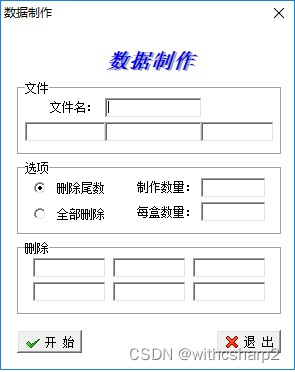
回答网友 修改一个exe
网友说:他有个很多年前的没有源码的exe,在win10上没法用,让俺看一下。 俺看了一下,发现是窗体设计的背景色的问题。这个程序的背景色用的是clInactiveCaptionText。clInactiveCaptionText 在win10之前的系统上是灰色,但…...
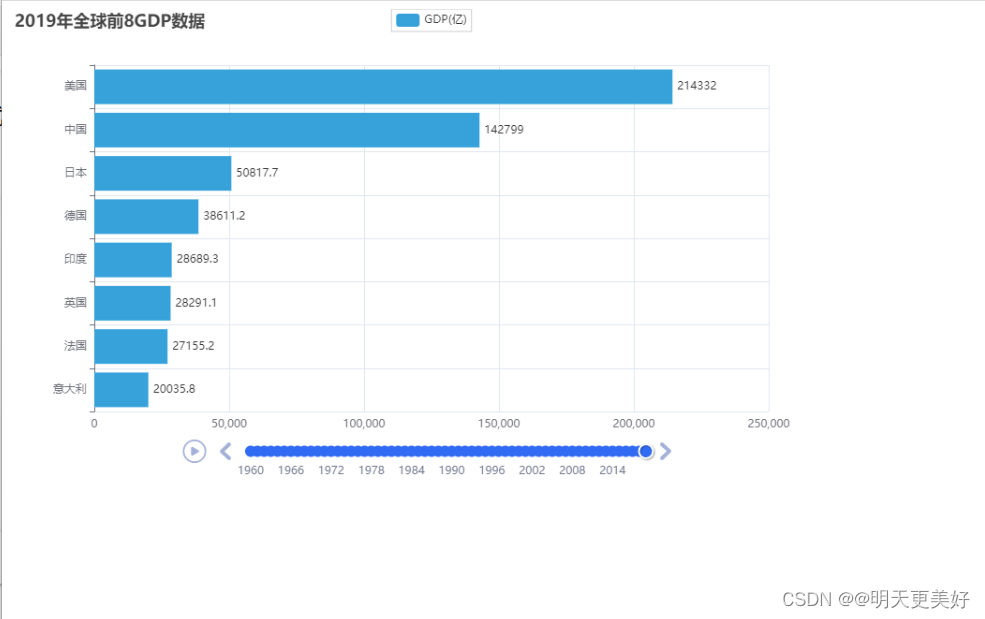
数据可视化 - 动态柱状图
基础柱状图 通过Bar构建基础柱状图 from pyecharts.charts import Bar from pyecharts.options import LabelOpts # 使用Bar构建基础柱状图 bar Bar() # 添加X轴 bar.add_xaxis(["中国", "美国", "英国"]) # 添加Y轴 # 设置数值标签在右侧 b…...

【JVM】JVM五大内存区域介绍
目录 一、程序计数器(线程私有) 二、java虚拟机栈(线程私有) 2.1、虚拟机栈 2.2、栈相关测试 2.2.1、栈溢出 三、本地方法栈(线程私有) 四、java堆(线程共享) 五、方法区&…...
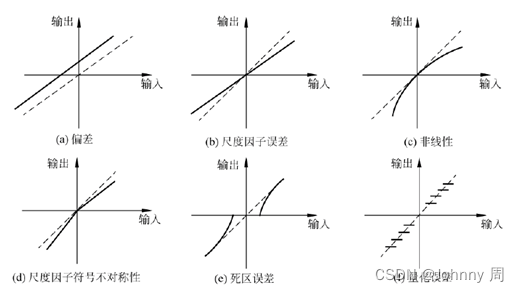
自动驾驶感知系统--惯性导航定位系统
惯性导航定位 惯性是所有质量体本身的基本属性,所以建立在牛顿定律基础上的惯性导航系统(Inertial Navigation System,INS)(简称惯导系统)不与外界发生任何光电联系,仅靠系统本身就能对车辆进行连续的三维定位和三维定向。卫星导…...
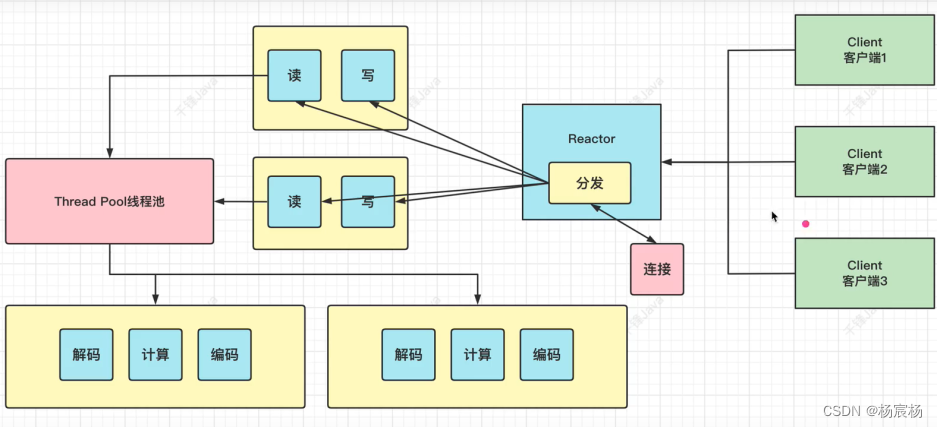
Netty简介
Netty Netty初体验基础概念Reactor模型传统的阻塞IO模型基础Reactor模型多线程Reactor模型 为什么要使用Netty? (NIO的框架,用于解决高并发出现的问题) *BIO:同步且阻塞的IO NIO:同步且非阻塞的IO(不是说线程&#x…...

基于TCP/IP对等模型对计算机网络知识点的整合
目录 前言 应用层 Telnet SSH FTP/TFTP SNMP:简单的网络管理协议 HTTP:超文本传输协议 SMTP:电子邮件传输协议 DNS:域名解析协议 DHCP:动态主机配置协议 NTP:网络时钟协议 传输层 TCP UDP 端…...
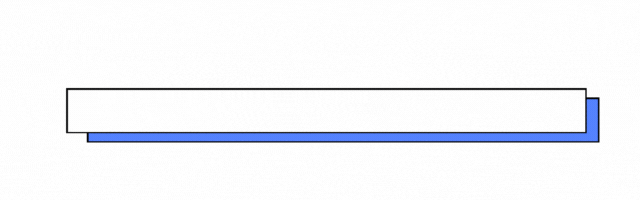
【SQL应知应会】表分区(一)• Oracle版
欢迎来到爱书不爱输的程序猿的博客, 本博客致力于知识分享,与更多的人进行学习交流 本文收录于SQL应知应会专栏,本专栏主要用于记录对于数据库的一些学习,有基础也有进阶,有MySQL也有Oracle 分区表 • Oracle版 前言一、分区表1.什么是表分区…...

PostgreSQL 常用空间处理函数
1.OGC标准函数 管理函数: 添加几何字段 AddGeometryColumn(, , , , , ) 删除几何字段 DropGeometryColumn(, , ) 检查数据库几何字段并在geometry_columns中归档 Probe_Geometry_Columns() 给几何对象设置空间参考(在通过一个范围做空间查询时常用&…...

ubuntu初始化/修改root密码
1.登录ubuntu后,使用sudo passwd root命令,进行root密码的初始化/修改,注:这里需要保证两次输入的密码都是同一个,才可成功 ubuntugt-ubuntu22-04-cmd-v1-0-32gb-100m:~/ocr$ sudo passwd root New password: Retype…...
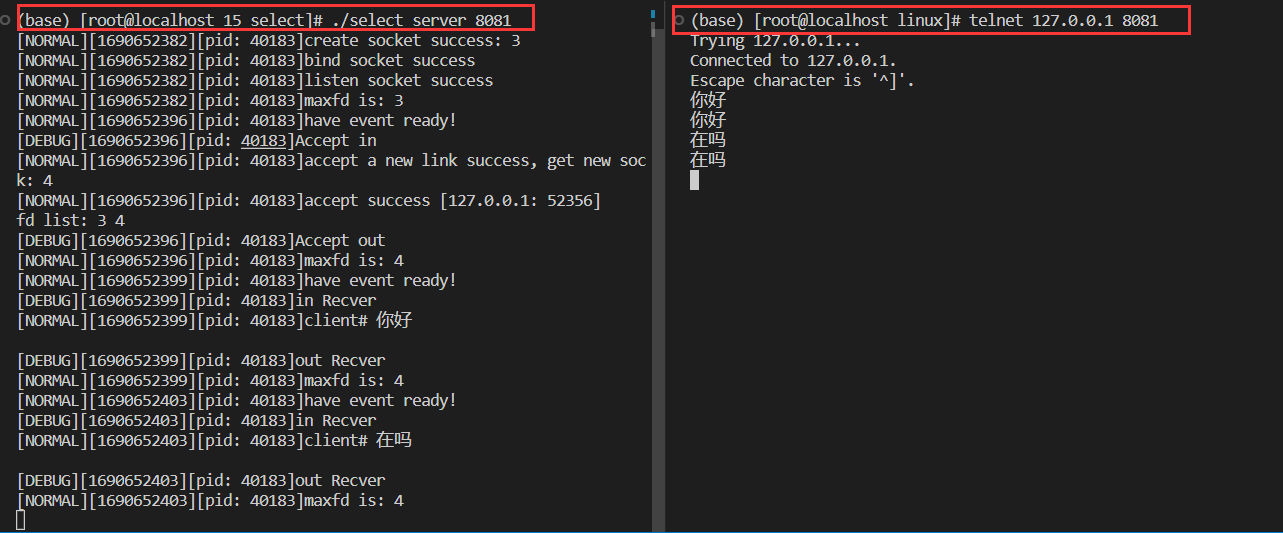
【Linux后端服务器开发】select多路转接IO服务器
目录 一、高级IO 二、fcntl 三、select函数接口 四、select实现多路转接IO服务器 一、高级IO 在介绍五种IO模型之前,我们先讲解一个钓鱼例子。 有一条大河,河里有很多鱼,分布均匀。张三是一个钓鱼新手,他钓鱼的时候很紧张&a…...
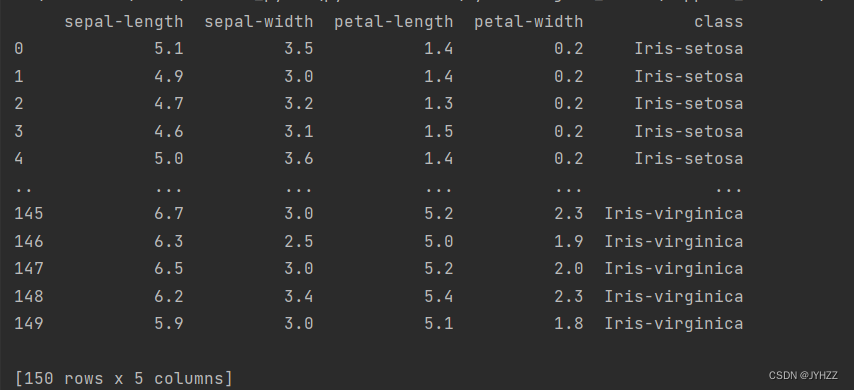
支持向量机(iris)
代码: import pandas as pd from sklearn.preprocessing import StandardScaler from sklearn import svm import numpy as np# 定义每一列的属性 colnames [sepal-length, sepal-width, petal-length, petal-width, class] # 读取数据 iris pd.read_csv(data\\i…...
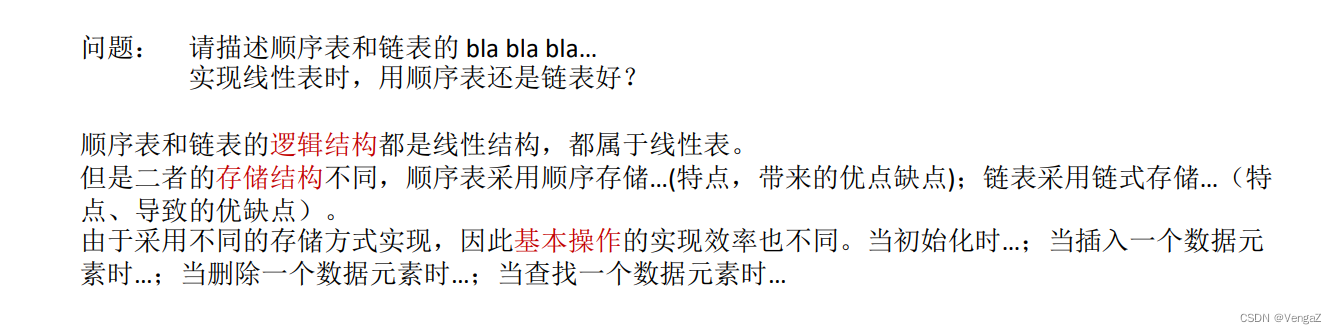
24考研数据结构-第二章:线性表
目录 第二章:线性表2.1线性表的定义(逻辑结构)2.2 线性表的基本操作(运算)2.3 线性表的物理/存储结构(确定了才确定数据结构)2.3.1 顺序表的定义2.3.1.1 静态分配2.3.1.2 动态分配2.3.1.3 mallo…...

Mybatis 动态 sql 是做什么的?都有哪些动态 sql?能简述动态 sql 的执行原理不?
OGNL表达式 OGNL,全称为Object-Graph Navigation Language,它是一个功能强大的表达式语言,用来获取和设置Java对象的属性,它旨在提供一个更高的更抽象的层次来对Java对象图进行导航。 OGNL表达式的基本单位是"导航链"&a…...

250_C++_typedef std::function<int(std::vector<int> vtBits)> fnChkSstStt
假设我们需要定义一个函数类型来表示一个能够计算整数向量中所有元素之和的函数。 首先,我们定义一个函数,它的参数是一个 std::vector 类型的整数向量,返回值是 int 类型,表示所有元素之和: int sumVectorElements(std::vector<int> vt) {int sum = 0;for (int n…...

莱州网站开发/app拉新推广
php中$_FIELS函数在php中上传一个文件建一个表单要比ASP中灵活的多。具体的看代码。然后upload.php中可以直接用$_FIELS,$_POST,$_GET等函数获取表单内容。当客户端提交后,我们获得了一个$_FILES数组$_FILES数组内容如下:$_FILES[‘myFile’][‘name’]:…...

如何在网站做引流/百度后台管理
Vehicle veh1 new Vehicle(); 通常把这条语句的动作称之为创建一个对象,其实,它包含了四个动作。 1)右边的“new Vehicle”,是以Vehicle类为模板,在堆空间里创建一个Vehicle类对象(也简称为Vehicle对象&am…...

网站定制论文1500字左右/推广公司产品
问题:我在视图画的图象或者文字,当窗口改变后为什么不见了?OnDraw()和OnPaint()两个都是解决上面的问题,有什么不同? 答:OnDraw()和OnPaint()好象兄弟俩,因为它们的工作类似。至于不见了的问题…...
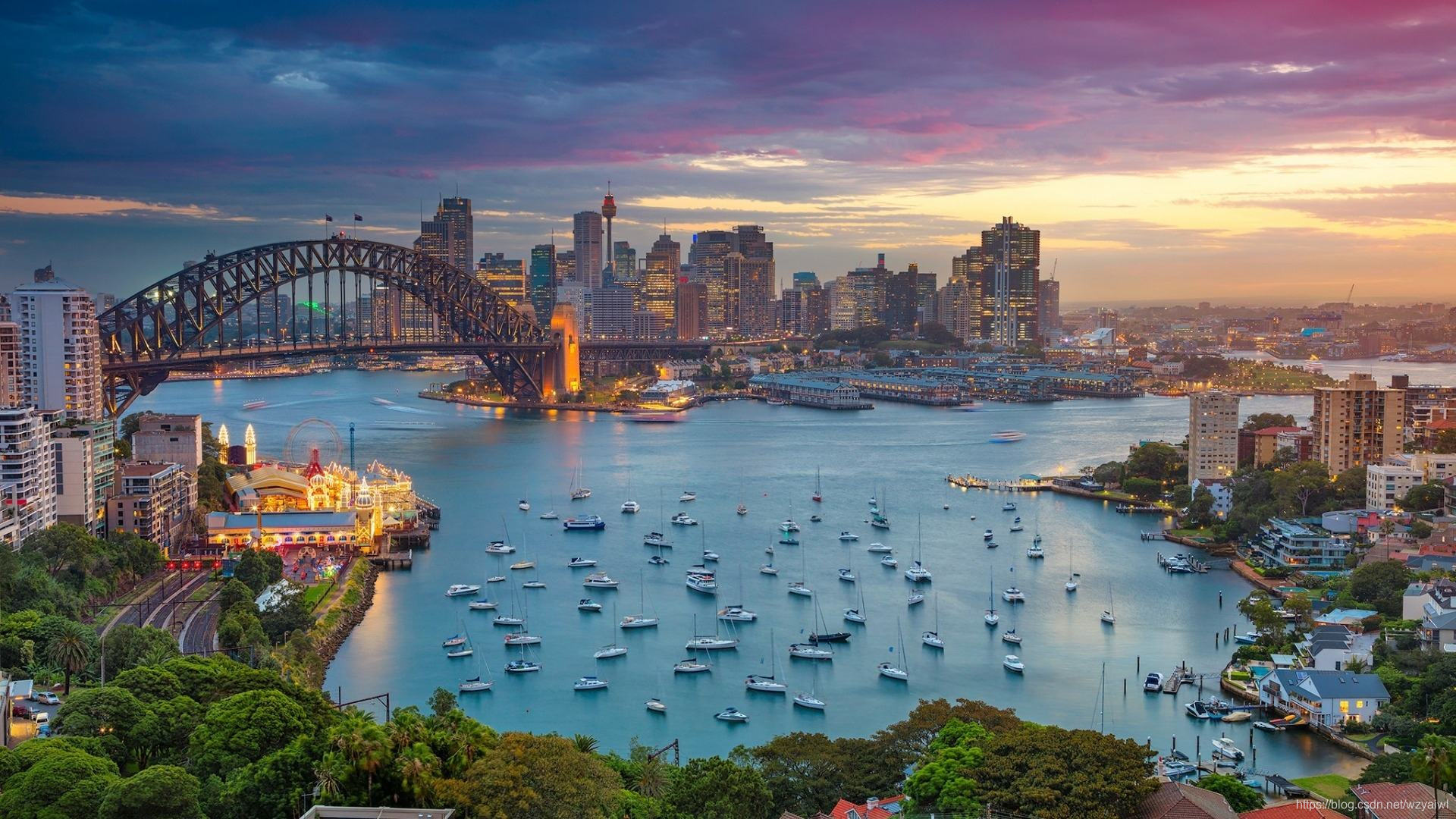
沈阳做网站的公司推荐/上海网站排名推广
选择器(Selector) 在parse方法中,我们经常要用到xpath、CSS、re来提取数据。在Scrapy中就为我们封装了这些方法于Selector,而且Selector是基于lxml构建的,这就意味着性能上不会有太大问题。在这里,我就不再…...
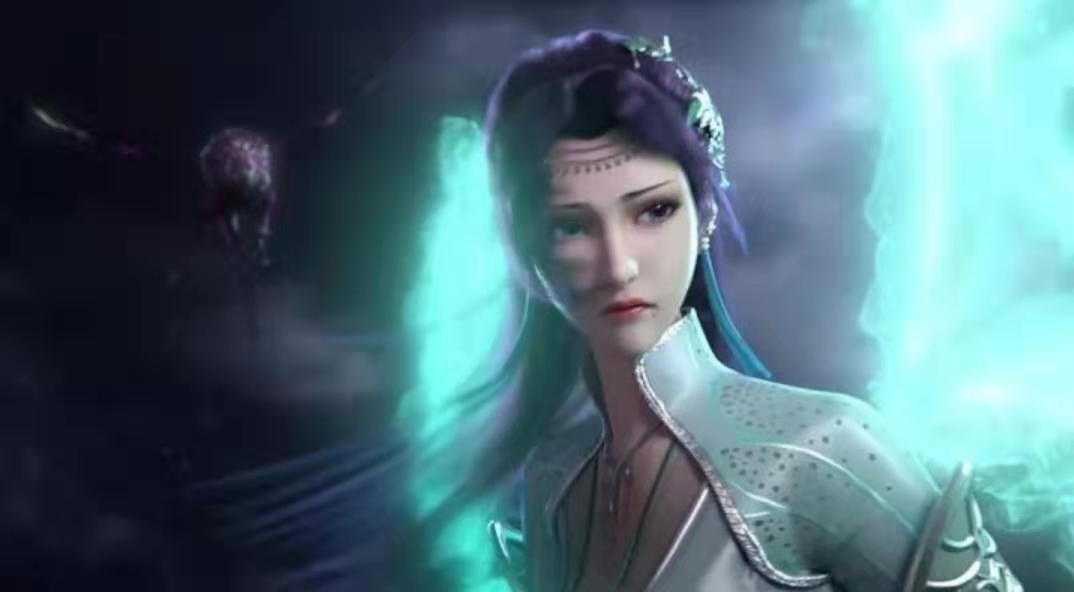
做搜狗pc网站优化点/品牌推广方案ppt
国漫 --《斗破苍穹》 这真的是我比较喜欢的作品了,第三季,云韵是我认为最遗憾的女主没有之一了。 转载于:https://www.cnblogs.com/Soar-Pang/p/11376406.html...

机械网站开发/网络广告文案
在python中没有类似sub()或者subString()的方法,但是字符串的截取操作却是更加简单。 只需要把字符串看作是一个字符数组,截取子串非常方便。 多余的话就不啰嗦了,看下面的例子就明白了。 str ’0123456789′ print str[0:3] #截取第一位到第…...