自然语言处理从入门到应用——LangChain:链(Chains)-[通用功能:LLMChain、RouterChain和SequentialChain]
分类目录:《自然语言处理从入门到应用》总目录
LLMChain
LLMChain是查询LLM对象最流行的方式之一。它使用提供的输入键值(如果有的话,还包括内存键值)格式化提示模板,将格式化的字符串传递给LLM,并返回LLM的输出。下面我们展示了LLMChain类的附加功能:
from langchain import PromptTemplate, OpenAI, LLMChainprompt_template = "What is a good name for a company that makes {product}?"llm = OpenAI(temperature=0)
llm_chain = LLMChain(llm=llm,prompt=PromptTemplate.from_template(prompt_template)
)
llm_chain("colorful socks")
输出:
{'product': 'colorful socks', 'text': '\n\nSocktastic!'}
LLM链条的额外运行方式
除了所有Chain对象共享的__call__
和run
方法之外,LLMChain还提供了几种调用链条逻辑的方式:
apply
:允许我们对一组输入运行链:
input_list = [{"product": "socks"},{"product": "computer"},{"product": "shoes"}
]llm_chain.apply(input_list)
[{'text': '\n\nSocktastic!'},{'text': '\n\nTechCore Solutions.'},{'text': '\n\nFootwear Factory.'}]
generate
:与apply
类似,但返回一个LLMResult而不是字符串。LLMResult通常包含有用的生成信息,例如令牌使用情况和完成原因。
llm_chain.generate(input_list)
输出:
LLMResult(generations=[[Generation(text='\n\nSocktastic!', generation_info={'finish_reason': 'stop', 'logprobs': None})], [Generation(text='\n\nTechCore Solutions.', generation_info={'finish_reason': 'stop', 'logprobs': None})], [Generation(text='\n\nFootwear Factory.', generation_info={'finish_reason': 'stop', 'logprobs': None})]], llm_output={'token_usage': {'prompt_tokens': 36, 'total_tokens': 55, 'completion_tokens': 19}, 'model_name': 'text-davinci-003'})
predict
:与run
方法类似,只是输入键被指定为关键字参数,而不是Python字典。
# Single input example
llm_chain.predict(product="colorful socks")
输出:
'\n\nSocktastic!'
输入:
# Multiple inputs exampletemplate = """Tell me a {adjective} joke about {subject}."""
prompt = PromptTemplate(template=template, input_variables=["adjective", "subject"])
llm_chain = LLMChain(prompt=prompt, llm=OpenAI(temperature=0))llm_chain.predict(adjective="sad", subject="ducks")
输出:
'\n\nQ: What did the duck say when his friend died?\nA: Quack, quack, goodbye.'
解析输出结果
默认情况下,即使底层的prompt
对象具有输出解析器,LLMChain也不会解析输出结果。如果你想在LLM输出上应用输出解析器,可以使用predict_and_parse
代替predict
,以及apply_and_parse
代替apply
。
仅使用predict
方法:
from langchain.output_parsers import CommaSeparatedListOutputParseroutput_parser = CommaSeparatedListOutputParser()
template = """List all the colors in a rainbow"""
prompt = PromptTemplate(template=template, input_variables=[], output_parser=output_parser)
llm_chain = LLMChain(prompt=prompt, llm=llm)llm_chain.predict()
输出:
'\n\nRed, orange, yellow, green, blue, indigo, violet'
使用predict_and_parser
方法:
llm_chain.predict_and_parse()
输出:
['Red', 'orange', 'yellow', 'green', 'blue', 'indigo', 'violet']
从字符串模板初始化
我们还可以直接使用字符串模板构建一个LLMChain。
template = """Tell me a {adjective} joke about {subject}."""
llm_chain = LLMChain.from_string(llm=llm, template=template)
llm_chain.predict(adjective="sad", subject="ducks")
输出:
'\n\nQ: What did the duck say when his friend died?\nA: Quack, quack, goodbye.'
RouterChain
本节演示了如何使用RouterChain创建一个根据给定输入动态选择下一个链条的链条。RouterChain通常由两个组件组成:
- 路由链本身(负责选择下一个要调用的链条)
- 目标链条,即路由链可以路由到的链条
本节中,我们将重点介绍不同类型的路由链。我们将展示这些路由链在MultiPromptChain
中的应用,创建一个问题回答链条,根据给定的问题选择最相关的提示,然后使用该提示回答问题。
from langchain.chains.router import MultiPromptChain
from langchain.llms import OpenAI
from langchain.chains import ConversationChain
from langchain.chains.llm import LLMChain
from langchain.prompts import PromptTemplate
physics_template = """You are a very smart physics professor. \
You are great at answering questions about physics in a concise and easy to understand manner. \
When you don't know the answer to a question you admit that you don't know.Here is a question:
{input}"""math_template = """You are a very good mathematician. You are great at answering math questions. \
You are so good because you are able to break down hard problems into their component parts, \
answer the component parts, and then put them together to answer the broader question.Here is a question:
{input}"""
prompt_infos = [{"name": "physics", "description": "Good for answering questions about physics", "prompt_template": physics_template},{"name": "math", "description": "Good for answering math questions", "prompt_template": math_template}
]
llm = OpenAI()
destination_chains = {}
for p_info in prompt_infos:name = p_info["name"]prompt_template = p_info["prompt_template"]prompt = PromptTemplate(template=prompt_template, input_variables=["input"])chain = LLMChain(llm=llm, prompt=prompt)destination_chains[name] = chain
default_chain = ConversationChain(llm=llm, output_key="text")
LLMRouterChain
LLMRouterChain链条使用一个LLM来确定如何进行路由。
from langchain.chains.router.llm_router import LLMRouterChain, RouterOutputParser
from langchain.chains.router.multi_prompt_prompt import MULTI_PROMPT_ROUTER_TEMPLATE
destinations = [f"{p['name']}: {p['description']}" for p in prompt_infos]
destinations_str = "\n".join(destinations)
router_template = MULTI_PROMPT_ROUTER_TEMPLATE.format(destinations=destinations_str
)
router_prompt = PromptTemplate(template=router_template,input_variables=["input"],output_parser=RouterOutputParser(),
)
router_chain = LLMRouterChain.from_llm(llm, router_prompt)
chain = MultiPromptChain(router_chain=router_chain, destination_chains=destination_chains, default_chain=default_chain, verbose=True)
print(chain.run("What is black body radiation?"))
日志输出:
> Entering new MultiPromptChain chain...
physics: {'input': 'What is black body radiation?'}
> Finished chain.
输出:
Black body radiation is the term used to describe the electromagnetic radiation emitted by a “black body”—an object that absorbs all radiation incident upon it. A black body is an idealized physical body that absorbs all incident electromagnetic radiation, regardless of frequency or angle of incidence. It does not reflect, emit or transmit energy. This type of radiation is the result of the thermal motion of the body's atoms and molecules, and it is emitted at all wavelengths. The spectrum of radiation emitted is described by Planck's law and is known as the black body spectrum.
输入:
print(chain.run("What is the first prime number greater than 40 such that one plus the prime number is divisible by 3"))
输出:
> Entering new MultiPromptChain chain...
math: {'input': 'What is the first prime number greater than 40 such that one plus the prime number is divisible by 3'}
> Finished chain.
输出:
The answer is 43. One plus 43 is 44 which is divisible by 3.
输入:
print(chain.run("What is the name of the type of cloud that rins"))
日志输出:
> Entering new MultiPromptChain chain...
None: {'input': 'What is the name of the type of cloud that rains?'}
> Finished chain.
输出:
The type of cloud that rains is called a cumulonimbus cloud. It is a tall and dense cloud that is often accompanied by thunder and lightning.
EmbeddingRouterChain
EmbeddingRouterChain使用嵌入和相似性来在目标链条之间进行路由。
from langchain.chains.router.embedding_router import EmbeddingRouterChain
from langchain.embeddings import CohereEmbeddings
from langchain.vectorstores import Chroma
names_and_descriptions = [("physics", ["for questions about physics"]),("math", ["for questions about math"]),
]
router_chain = EmbeddingRouterChain.from_names_and_descriptions(names_and_descriptions, Chroma, CohereEmbeddings(), routing_keys=["input"]
)
chain = MultiPromptChain(router_chain=router_chain, destination_chains=destination_chains, default_chain=default_chain, verbose=True)
print(chain.run("What is black body radiation?"))
日志输出:
> Entering new MultiPromptChain chain...
physics: {'input': 'What is black body radiation?'}
> Finished chain.
输出:
Black body radiation is the emission of energy from an idealized physical body (known as a black body) that is in thermal equilibrium with its environment. It is emitted in a characteristic pattern of frequencies known as a black-body spectrum, which depends only on the temperature of the body. The study of black body radiation is an important part of astrophysics and atmospheric physics, as the thermal radiation emitted by stars and planets can often be approximated as black body radiation.
输入:
print(chain.run("What is the first prime number greater than 40 such that one plus the prime number is divisible by 3"))
日志输出:
> Entering new MultiPromptChain chain...
math: {'input': 'What is the first prime number greater than 40 such that one plus the prime number is divisible by 3'}
> Finished chain.
输出:
Answer: The first prime number greater than 40 such that one plus the prime number is divisible by 3 is 43.
参考文献:
[1] LangChain官方网站:https://www.langchain.com/
[2] LangChain 🦜️🔗 中文网,跟着LangChain一起学LLM/GPT开发:https://www.langchain.com.cn/
[3] LangChain中文网 - LangChain 是一个用于开发由语言模型驱动的应用程序的框架:http://www.cnlangchain.com/
相关文章:
自然语言处理从入门到应用——LangChain:链(Chains)-[通用功能:LLMChain、RouterChain和SequentialChain]
分类目录:《自然语言处理从入门到应用》总目录 LLMChain LLMChain是查询LLM对象最流行的方式之一。它使用提供的输入键值(如果有的话,还包括内存键值)格式化提示模板,将格式化的字符串传递给LLM,并返回LLM…...
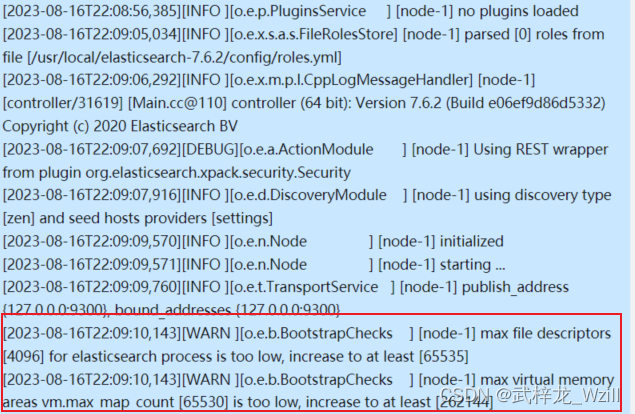
ElasticSearch-安装部署全过程
本文已收录于专栏 《中间件合集》 目录 概念说明什么是ElasticSearch什么是Kibana什么是RESTful API 提供服务安装过程安装ElasticSearch1.下载ElasticSearch 安装包2.解压安装包3.进入解压之后的文件夹4.创建一个data文件夹用来存储数据5.进入config文件夹编辑elasticsearch.y…...
mathematica报错:Tag Plus is \ Protected
在使用化简函数Simplify的时候使用了规则的语法,但是规则可能没有使用等号。 例如 Simplify[(1 - c^2)/d^2, c^2 d^2 1]等号被认为是赋值符号,要修改为两个等号: Simplify[(1 - c^2)/d^2, c^2 d^2 1]这样就不会报错了。...
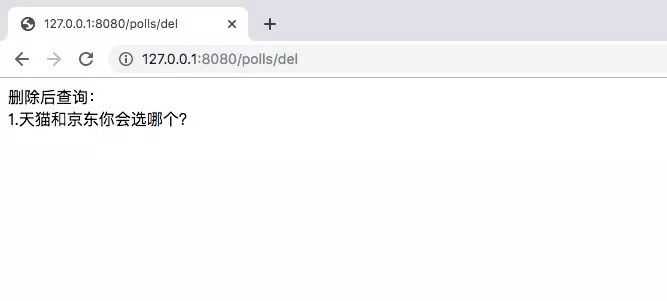
Python Django 模型概述与应用
今天来为大家介绍 Django 框架的模型部分,模型是真实数据的简单明确的描述,它包含了储存的数据所必要的字段和行为,Django 遵循 DRY Principle 。它的目标是你只需要定义数据模型,然后其它的杂七杂八代码你都不用关心,…...
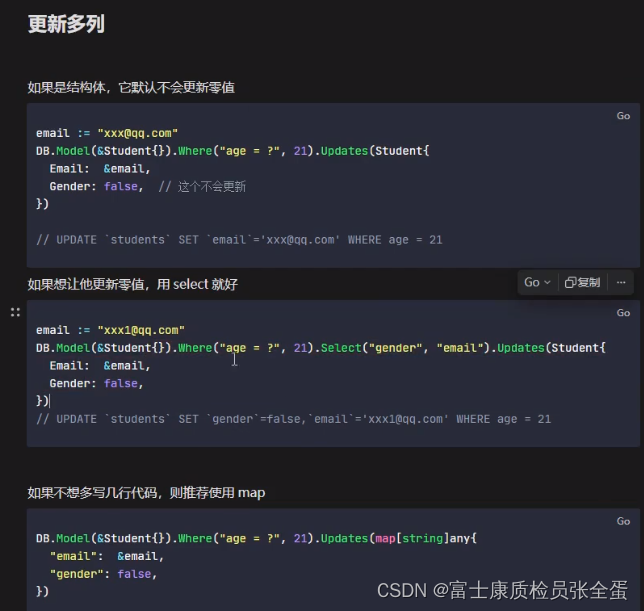
Golang Gorm 更新字段 save update updates
更新和删除操作的前提条件都是要在找到数据的情况下,先要查询到数据才可以做操作。 更新的前提的先查询到记录,Save保存所有字段,用于单个记录的全字段更新它会保控所有字段,即使零值也会保存。 在更新和删除之前,要利…...
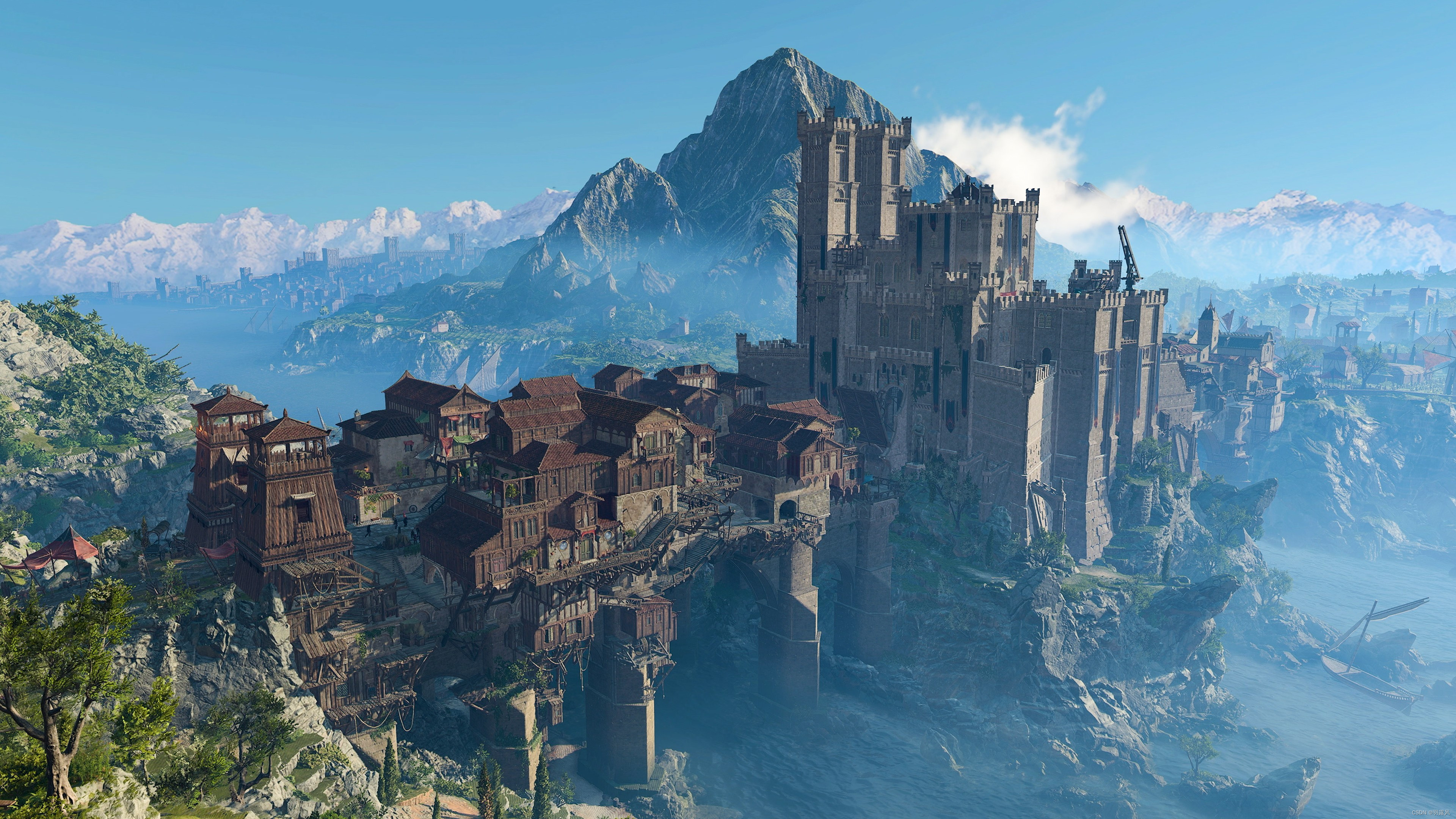
springBoot 配置文件引入 redis 的相关参数说明
在Spring Boot应用中使用Redis作为缓存或数据存储时,可以在应用的配置文件中配置相关参数。下面是常用的Redis配置参数及其说明: spring.redis.host: Redis服务器主机地址,默认为localhost。spring.redis.port: Redis服务器端口,…...
Docker的使用心得:简化开发与部署的利器
开发与测试的无缝衔接: Docker让开发与测试之间的切换变得前所未有的顺畅。我可以在本地开发环境中创建一个与生产环境一致的Docker容器,这样不仅可以确保开发过程中不会出现意外问题,还可以在测试阶段避免不必要的繁琐配置。 跨平台的可移植…...
vue3 基于element plus对el-pagination进行二次封装
vue3 基于element plus对el-pagination进行二次封装 1、前言2、在components文件夹中新建pagination.vue文件3、在组件内使用分页 1、前言 在vue3项目中,如果每个列表页都敲一遍分页方法,显然是不合理的,那么,下面我将基于elemen…...
RuntimeError: result type Float can‘t be cast to the desired output type __int64报错解决方法
小白刚开始学习YOLOv5,跟随老哥的步骤走了一遍目标检测--手把手教你搭建自己的YOLOv5目标检测平台 最后训练最后一步出现RuntimeError: result type Float can‘t be cast to the desired output type __int64报错 解决方法:找到5.0版报错的loss.py中最…...
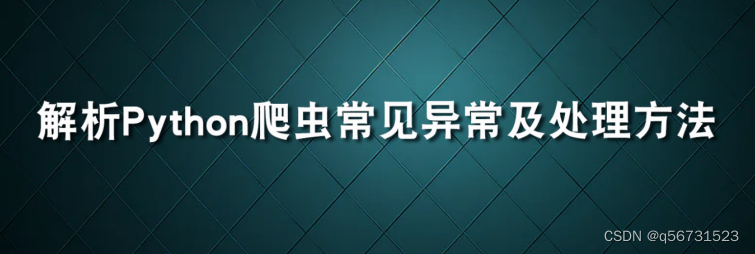
解析Python爬虫常见异常及处理方法
作为专业爬虫程序猿长期混迹于爬虫ip解决方案中,我们经常会遇到各种各样的异常情况。在爬虫开发过程中,处理这些异常是不可或缺的一部分。本文将为大家总结常见的Python爬虫异常,并分享相应的处理方法,帮助你避免绊倒在爬虫之路上…...
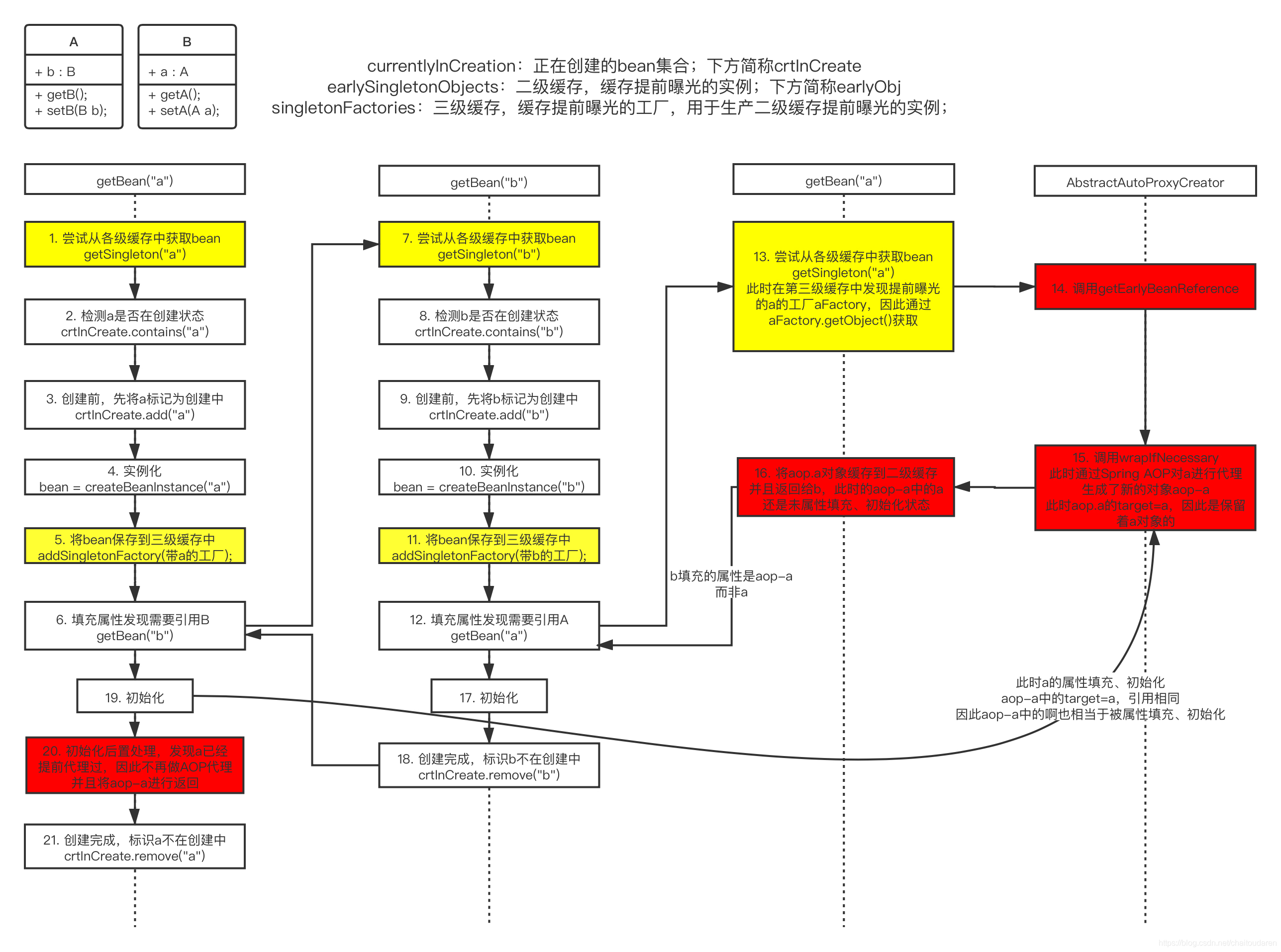
详解Spring的循环依赖问题、三级缓存解决方案源码分析
0、基础:Bean的生命周期 在Spring中,由于IOC的控制反转,创建对象不再是简单的new出来,而是交给Spring去创建,会经历一系列Bean的生命周期才创建出相应的对象。而循环依赖问题也是由Bean的生命周期过程导致的问题&#…...
oracle分析函数学习
0、建表及插入测试数据 --CREATE TEST TABLE AND INSERT TEST DATA. create table students (id number(15,0), area varchar2(10), stu_type varchar2(2), score number(20,2));insert into students values(1, 111, g, 80 ); insert into students values(1, 111, j, 80 ); …...
代码随想录训练营day17|110.平衡二叉树 257. 二叉树的所有路径 404.左叶子之和 v...
TOC 前言 代码随想录算法训练营day17 一、Leetcode 110.平衡二叉树 1.题目 给定一个二叉树,判断它是否是高度平衡的二叉树。 本题中,一棵高度平衡二叉树定义为: 一个二叉树每个节点 的左右两个子树的高度差的绝对值不超过 1 。 示例 1&#x…...
C# Thread用法
C# 中的线程(Thread)是一种并发执行的机制,允许同时执行多个代码块,从而提高程序的性能和响应性。下面是关于如何使用 C# 线程的一些基本用法: 1. 创建线程: 使用 System.Threading 命名空间中的 Thread 类…...
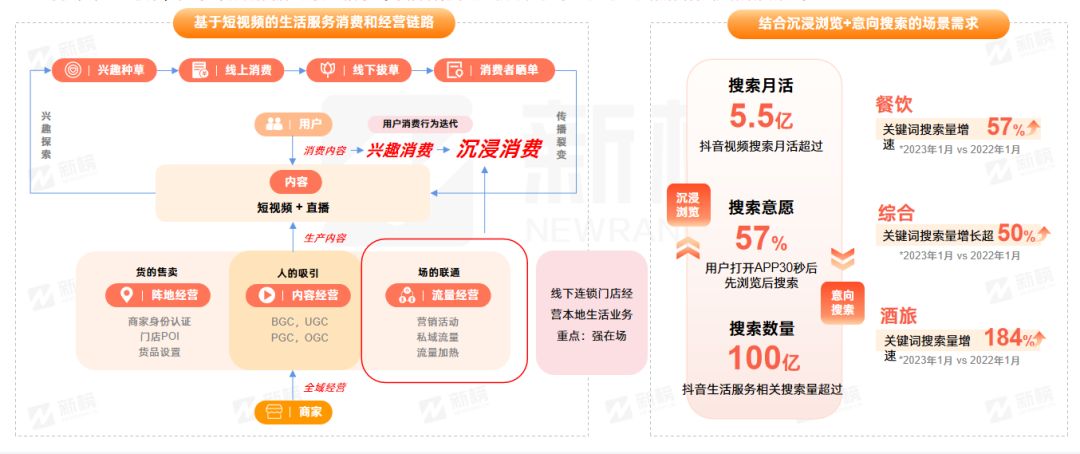
新榜 | CityWalk本地生活商业价值洞察报告
如果说现在有人问,最新的网络热词是什么? “CityWalk”,这可能是大多数人的答案。 近段时间,“CityWalk”刷屏了各种社交媒体,给网友们带来了一场“城市漫步”之旅。 脱离群体狂欢,这个在社交媒体引发热议的词汇背后又…...
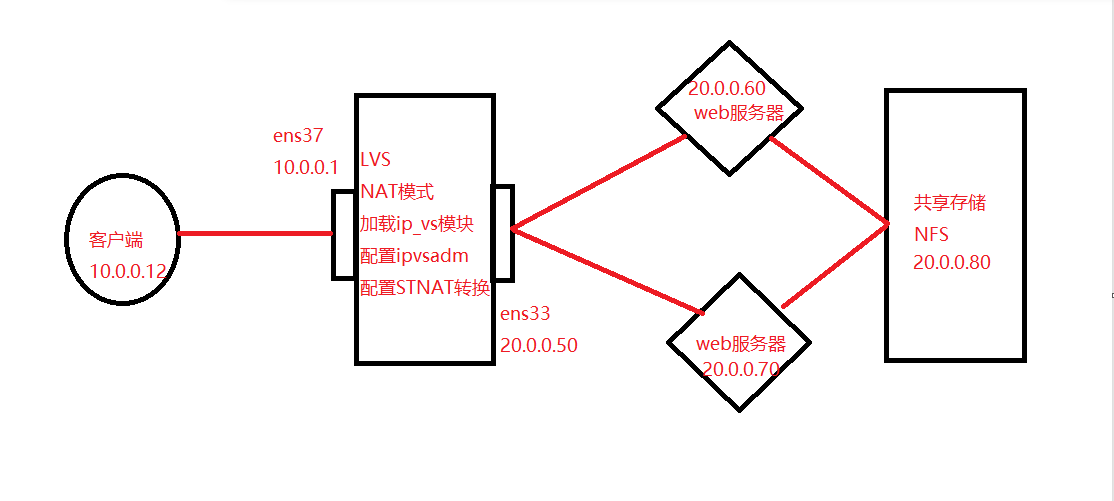
LVS负载均衡集群-NAT模式部署
集群 集群:将多台主机作为一个整体,然后对外提供相同的服务 集群使用场景:高并发的场景 集群的分类 1.负载均衡器集群 减少响应延迟,提高并发处理的能力 2,高可用集群 增强系统的稳定性可靠性&…...
C++学习笔记总结练习:effective 学习日志
准则 1.少使用define define所定义的常量会在预处理的时候被替代,出错编译器不容易找到错误。而且还没有作用范围限制,推荐使用constdefine宏定义的函数,容易出错,而且参数需要加上小括号,推荐使用inline有的类中例如…...
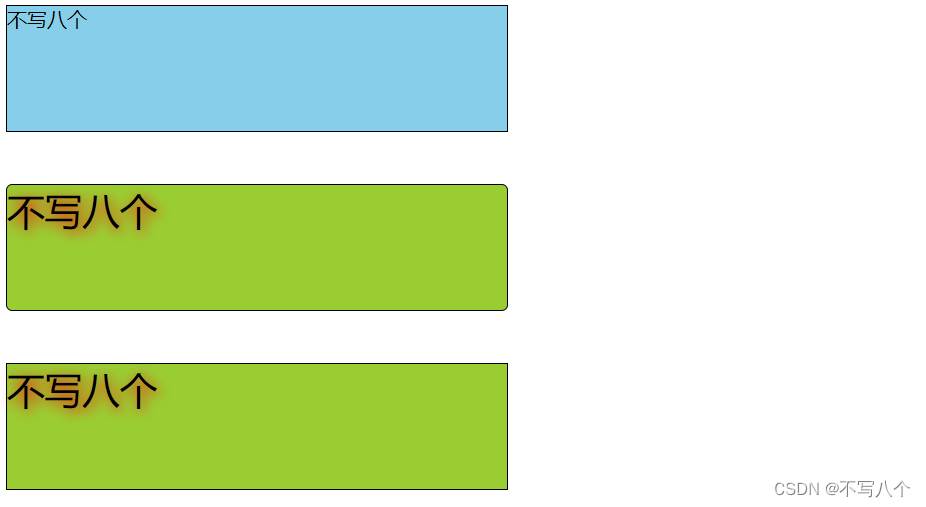
Vue教程(五):样式绑定——class和style
1、样式代码准备 样式提前准备 <style>.basic{width: 400px;height: 100px;border: 1px solid black;}.happy{border: 4px solid red;background-color: rgba(255, 255, 0, 0.644);background: linear-gradient(30deg, yellow, pink, orange, yellow);}.sad{border: 4px …...
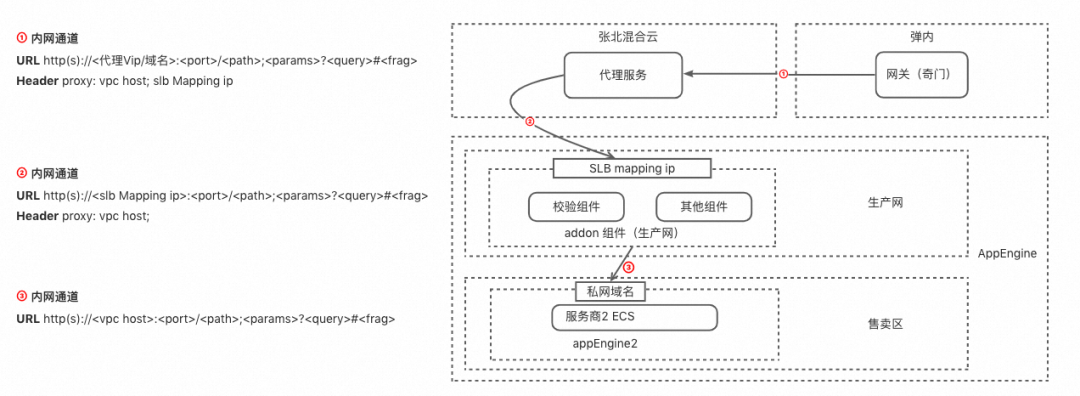
开放网关架构演进
作者:庄文弘(弘智) 淘宝开放平台是阿里与外部生态互联互通的重要开放途径,通过开放的产品技术把阿里经济体一系列基础服务,像水、电、煤一样输送给我们的商家、开发者、社区媒体以及其他合作伙伴,推动行业的…...
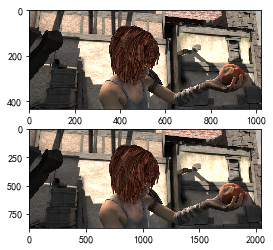
torch一些操作
Pytorch文档 Pytorch 官方文档 https://pytorch.org/docs/stable/index.html pytorch 里的一些基础tensor操作讲的不错 https://blog.csdn.net/abc13526222160/category_8614343.html 关于pytorch的Broadcast,合并与分割,数学运算,属性统计以及高阶操作 https://blog.csd…...
蓝桥杯 2024 15届国赛 A组 儿童节快乐
P10576 [蓝桥杯 2024 国 A] 儿童节快乐 题目描述 五彩斑斓的气球在蓝天下悠然飘荡,轻快的音乐在耳边持续回荡,小朋友们手牵着手一同畅快欢笑。在这样一片安乐祥和的氛围下,六一来了。 今天是六一儿童节,小蓝老师为了让大家在节…...
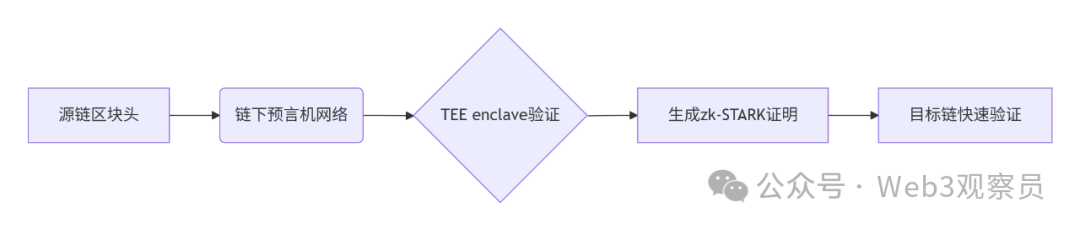
跨链模式:多链互操作架构与性能扩展方案
跨链模式:多链互操作架构与性能扩展方案 ——构建下一代区块链互联网的技术基石 一、跨链架构的核心范式演进 1. 分层协议栈:模块化解耦设计 现代跨链系统采用分层协议栈实现灵活扩展(H2Cross架构): 适配层…...

[10-3]软件I2C读写MPU6050 江协科技学习笔记(16个知识点)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16...
【C++从零实现Json-Rpc框架】第六弹 —— 服务端模块划分
一、项目背景回顾 前五弹完成了Json-Rpc协议解析、请求处理、客户端调用等基础模块搭建。 本弹重点聚焦于服务端的模块划分与架构设计,提升代码结构的可维护性与扩展性。 二、服务端模块设计目标 高内聚低耦合:各模块职责清晰,便于独立开发…...
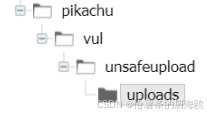
Unsafe Fileupload篇补充-木马的详细教程与木马分享(中国蚁剑方式)
在之前的皮卡丘靶场第九期Unsafe Fileupload篇中我们学习了木马的原理并且学了一个简单的木马文件 本期内容是为了更好的为大家解释木马(服务器方面的)的原理,连接,以及各种木马及连接工具的分享 文件木马:https://w…...
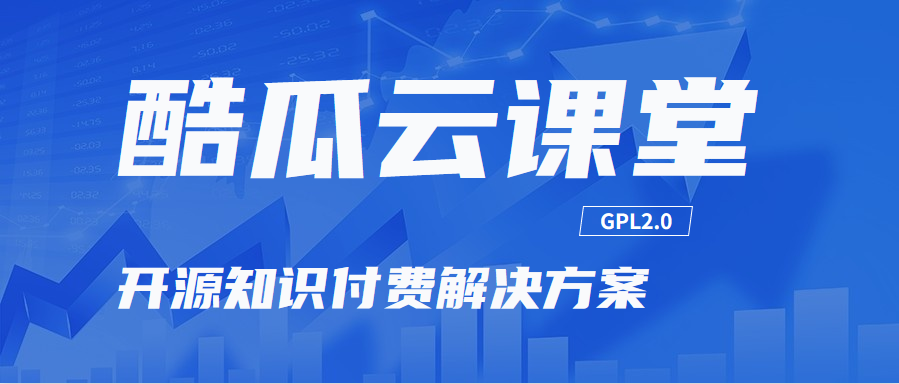
Linux 中如何提取压缩文件 ?
Linux 是一种流行的开源操作系统,它提供了许多工具来管理、压缩和解压缩文件。压缩文件有助于节省存储空间,使数据传输更快。本指南将向您展示如何在 Linux 中提取不同类型的压缩文件。 1. Unpacking ZIP Files ZIP 文件是非常常见的,要在 …...
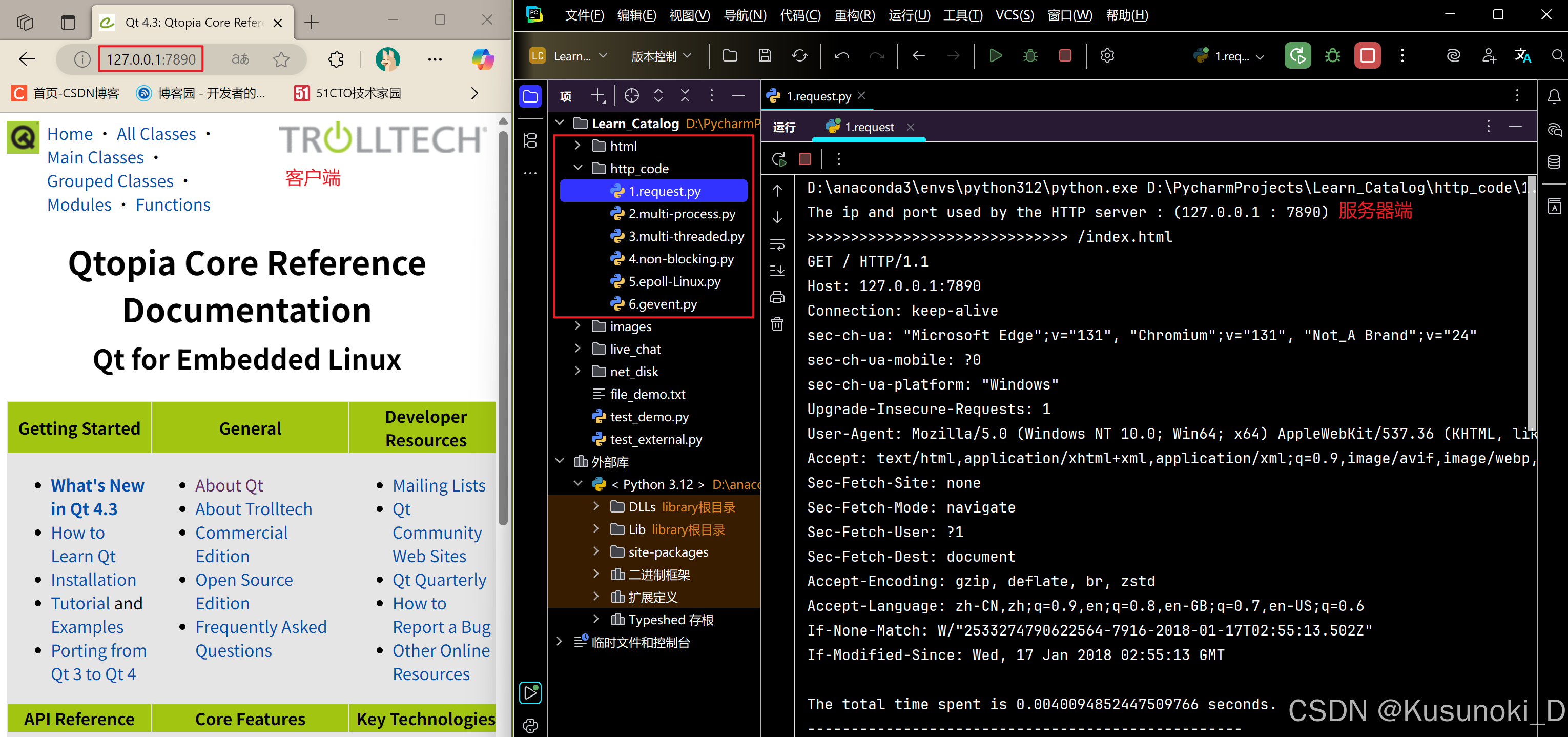
Python 实现 Web 静态服务器(HTTP 协议)
目录 一、在本地启动 HTTP 服务器1. Windows 下安装 node.js1)下载安装包2)配置环境变量3)安装镜像4)node.js 的常用命令 2. 安装 http-server 服务3. 使用 http-server 开启服务1)使用 http-server2)详解 …...
libfmt: 现代C++的格式化工具库介绍与酷炫功能
libfmt: 现代C的格式化工具库介绍与酷炫功能 libfmt 是一个开源的C格式化库,提供了高效、安全的文本格式化功能,是C20中引入的std::format的基础实现。它比传统的printf和iostream更安全、更灵活、性能更好。 基本介绍 主要特点 类型安全:…...
鸿蒙HarmonyOS 5军旗小游戏实现指南
1. 项目概述 本军旗小游戏基于鸿蒙HarmonyOS 5开发,采用DevEco Studio实现,包含完整的游戏逻辑和UI界面。 2. 项目结构 /src/main/java/com/example/militarychess/├── MainAbilitySlice.java // 主界面├── GameView.java // 游戏核…...
webpack面试题
面试题:webpack介绍和简单使用 一、webpack(模块化打包工具)1. webpack是把项目当作一个整体,通过给定的一个主文件,webpack将从这个主文件开始找到你项目当中的所有依赖文件,使用loaders来处理它们&#x…...