【java】【项目实战】[外卖九]项目优化(缓存)
目录
一、问题说明
二、环境搭建
2.1 Git管理代码
2.1.1 创建本地仓库
2.1.2 创建远程仓库
2.1.3 创建分支--》推送到远程仓库
2.2 maven坐标
2.3 配置文件application.yml
2.4 配置类RedisConfig
三、缓存短信验证码
3.1 实现思路
3.2 代码改造
3.2.1 UserController
3.3 功能测试
四、缓存菜品数据
4.1 实现思路
4.2 代码改造
4.2.1 第一步改造DishController 中list方法
4.2.2 第三步改造DishController中的save和update方法
4.3 功能测试
五、Spring Cache
5.1 Spring Cache介绍
5.2 Spring Cache常用注解
5.3 Spring Cache使用方式
5.3.1 pom
六、缓存套餐数据
6.1 实现思路
6.2 代码改造
6.2.1 pom 坐标
6.2.2 application.yml配置缓存数据过期时间
6.2.3 开启缓存注解功能 ReggieApplication
6.2.4 R实现 Serializable 接口
6.2.5 在SetmealController的list方法加入@Cacheable注解
6.2.6 在SetmealController的delete方法加入@CacheEvict注解
6.2.7 在SetmealController的save方法加入@CacheEvict注解
6.3 功能测试
前言:使用缓存进行项目优化
一、问题说明
二、环境搭建
2.1 Git管理代码
2.1.1 创建本地仓库
2.1.2 创建远程仓库
略。。后续都省略
2.1.3 创建分支--》推送到远程仓库
略
2.2 maven坐标
<!--redis--><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-redis</artifactId></dependency>
2.3 配置文件application.yml
server:port: 8080
spring:application:# 应用名称 可选name: reggie_take_out_spuerdatasource:druid:driver-class-name: com.mysql.cj.jdbc.Driverurl: jdbc:mysql://localhost:3306/reggie?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf-8&zeroDateTimeBehavior=convertToNull&useSSL=false&allowPublicKeyRetrieval=trueusername: rotpassword: ruredis:host: 127.0.0.1port: 6379password: 123database: 0
mybatis-plus:configuration:#在映射实体或者属性时,将数据库中表名和字段名中的下划线去掉,按照驼峰命名法映射map-underscore-to-camel-case: truelog-impl: org.apache.ibatis.logging.stdout.StdOutImplglobal-config:db-config:id-type: ASSIGN_ID
# 文件上传目录
reggie:path: D:\images\
2.4 配置类RedisConfig
package com.runa.reggie.config;import org.springframework.cache.annotation.CachingConfigurerSupport;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;@Configuration
public class RedisConfig extends CachingConfigurerSupport {@Beanpublic RedisTemplate<Object, Object> redisTemplate(RedisConnectionFactory connectionFactory) {RedisTemplate<Object, Object> redisTemplate = new RedisTemplate<>();//默认的Key序列化器为:JdkSerializationRedisSerializerredisTemplate.setKeySerializer(new StringRedisSerializer());redisTemplate.setConnectionFactory(connectionFactory);return redisTemplate;}
}
三、缓存短信验证码
3.1 实现思路
3.2 代码改造
3.2.1 UserController
package com.runa.reggie.controller;import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.runa.reggie.common.R;
import com.runa.reggie.entity.User;
import com.runa.reggie.service.UserService;
import com.runa.reggie.utils.SMSUtils;
import com.runa.reggie.utils.ValidateCodeUtils;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;import javax.servlet.http.HttpSession;
import java.util.Map;
import java.util.concurrent.TimeUnit;@RestController
@RequestMapping("/user")
@Slf4j
public class UserController {@Autowiredprivate UserService userService;@Autowiredprivate RedisTemplate redisTemplate;/*** 发送手机短信验证码* @param user* @return*/@PostMapping("/sendMsg")public R<String> sendMsg(@RequestBody User user, HttpSession session){//获取手机号String phone = user.getPhone();if(StringUtils.isNotEmpty(phone)){//生成随机的4位验证码String code = ValidateCodeUtils.generateValidateCode(4).toString();log.info("code={}",code);//调用阿里云提供的短信服务API完成发送短信//SMSUtils.sendMessage("瑞吉外卖","",phone,code);//需要将生成的验证码保存到Session
// session.setAttribute(phone,code);// 将生成的验证码缓存到Redis中,并且设置有效期为5分钟redisTemplate.opsForValue().set(phone,code,5, TimeUnit.MINUTES);return R.success("手机验证码短信发送成功");}return R.error("短信发送失败");}/*** 移动端用户登录* @param map* @param session* @return*/@PostMapping("/login")public R<User> login(@RequestBody Map map, HttpSession session){log.info(map.toString());//获取手机号String phone = map.get("phone").toString();//获取验证码String code = map.get("code").toString();//从Session中获取保存的验证码
// Object codeInSession = session.getAttribute(phone);//从redis中获取缓存的验证码Object codeInSession = redisTemplate.opsForValue().get(phone);//进行验证码的比对(页面提交的验证码和Session中保存的验证码比对)if(codeInSession != null && codeInSession.equals(code)){//如果能够比对成功,说明登录成功LambdaQueryWrapper<User> queryWrapper = new LambdaQueryWrapper<>();queryWrapper.eq(User::getPhone,phone);User user = userService.getOne(queryWrapper);if(user == null){//判断当前手机号对应的用户是否为新用户,如果是新用户就自动完成注册user = new User();user.setPhone(phone);user.setStatus(1);userService.save(user);}session.setAttribute("user",user.getId());// 如果用户登录成功,删除redis缓存的验证码redisTemplate.delete(phone);return R.success(user);}return R.error("登录失败");}}
3.3 功能测试
启动后端服务、启动redis
http://localhost:8080/front/page/login.html
四、缓存菜品数据
4.1 实现思路
4.2 代码改造
4.2.1 第一步改造DishController 中list方法
package com.runa.reggie.controller;import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.runa.reggie.common.R;
import com.runa.reggie.dto.DishDto;
import com.runa.reggie.entity.Category;
import com.runa.reggie.entity.Dish;
import com.runa.reggie.entity.DishFlavor;
import com.runa.reggie.service.CategoryService;
import com.runa.reggie.service.DishFlavorService;
import com.runa.reggie.service.DishService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.web.bind.annotation.*;import java.util.List;
import java.util.concurrent.TimeUnit;
import java.util.stream.Collectors;/*** 菜品管理*/
@RestController
@RequestMapping("/dish")
@Slf4j
public class DishController {@Autowiredprivate DishService dishService;@Autowiredprivate DishFlavorService dishFlavorService;@Autowiredprivate CategoryService categoryService;@Autowiredprivate RedisTemplate redisTemplate;/*** 新增菜品* @param dishDto* @return*/@PostMappingpublic R<String> save(@RequestBody DishDto dishDto){log.info(dishDto.toString());dishService.saveWithFlavor(dishDto);return R.success("新增菜品成功");}@GetMapping("/page")public R<Page> page(int page, int pageSize, String name){// 构造分页构造器对象Page<Dish> pageInfo = new Page<>(page, pageSize);Page<DishDto> dishDtoPage = new Page<>();//条件构造器LambdaQueryWrapper<Dish> queryWrapper = new LambdaQueryWrapper<>();// 添加过滤条件queryWrapper.like(name != null,Dish::getName,name);// 添加排序条件queryWrapper.orderByDesc(Dish::getUpdateTime);//执行分页查询dishService.page(pageInfo,queryWrapper);// 对象拷贝BeanUtils.copyProperties(pageInfo, dishDtoPage,"records");List<Dish> records = pageInfo.getRecords();List<DishDto> list = records.stream().map((item) -> {DishDto dishDto = new DishDto();BeanUtils.copyProperties(item,dishDto);Long categoryId = item.getCategoryId();// 分类idCategory category = categoryService.getById(categoryId);String categoryName = category.getName();dishDto.setCategoryName(categoryName);return dishDto;}).collect(Collectors.toList());dishDtoPage.setRecords(list);return R.success(dishDtoPage);}/*** 根据ID 查询菜品和对应口味信息 回显* @param id* @return*/@GetMapping("/{id}")public R<DishDto> get(@PathVariable Long id){log.info("要查询显示的菜品信息是:{}",id);DishDto dishDto = dishService.getByIdWithFlavor(id);return R.success(dishDto);}/*** 修改菜品* @param dishDto* @return*/@PutMappingpublic R<String> update(@RequestBody DishDto dishDto){log.info(dishDto.toString());dishService.updateWithFlavor(dishDto);return R.success("修改菜品成功");}// /**
// * 根据条件查询对于菜品数据
// * @param dish
// * @return
// */
// @GetMapping("/list")
// public R<List<Dish>> list(Dish dish){
//
// // 构造查询条件
// LambdaQueryWrapper<Dish> queryWrapper =new LambdaQueryWrapper<>();
// queryWrapper.eq(dish.getCategoryId() != null,Dish::getCategoryId,dish.getCategoryId());
// // 添加条件,查询状态为1(起售状态)的菜品
// queryWrapper.eq(Dish::getStatus,1);
// // 天添加排序条件
// queryWrapper.orderByAsc(Dish::getSort).orderByDesc(Dish::getUpdateTime);
// List<Dish> list = dishService.list(queryWrapper);
// return R.success(list);
// }
//}/*** 根据条件查询对于菜品数据* @param dish* @return*/@GetMapping("/list")public R<List<DishDto>> list(Dish dish){List<DishDto> dishDtoList = null;// 动态构造keyString key = "dish_" + dish.getCategoryId() + "_" + dish.getStatus();// 先从redis获取缓存数据dishDtoList = (List<DishDto>) redisTemplate.opsForValue().get(key);if(dishDtoList != null){// 1 如果存在,直接返回,无需查询数据库return R.success(dishDtoList);}// 2 如果不存在,需要查询数据库,// 构造查询条件LambdaQueryWrapper<Dish> queryWrapper =new LambdaQueryWrapper<>();queryWrapper.eq(dish.getCategoryId() != null,Dish::getCategoryId,dish.getCategoryId());// 添加条件,查询状态为1(起售状态)的菜品queryWrapper.eq(Dish::getStatus,1);// 天添加排序条件queryWrapper.orderByAsc(Dish::getSort).orderByDesc(Dish::getUpdateTime);List<Dish> list = dishService.list(queryWrapper);dishDtoList = list.stream().map((item) -> {DishDto dishDto = new DishDto();BeanUtils.copyProperties(item,dishDto);Long categoryId = item.getCategoryId();// 分类id//根据id查询分类对象Category category = categoryService.getById(categoryId);if(category != null){String categoryName = category.getName();dishDto.setCategoryName(categoryName);}// 当前菜品的idLong dishId = item.getId();LambdaQueryWrapper<DishFlavor> lambdaQueryWrapper = new LambdaQueryWrapper<>();lambdaQueryWrapper.eq(DishFlavor::getDishId,dishId);List<DishFlavor> dishFlavorList = dishFlavorService.list(lambdaQueryWrapper);dishDto.setFlavors(dishFlavorList);return dishDto;}).collect(Collectors.toList());// 2-1 不存在,将查询到的菜品数据缓存到redisredisTemplate.opsForValue().set(key,dishDtoList,60, TimeUnit.MINUTES);return R.success(dishDtoList);}
}
4.2.2 第三步改造DishController中的save和update方法
package com.runa.reggie.controller;import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.runa.reggie.common.R;
import com.runa.reggie.dto.DishDto;
import com.runa.reggie.entity.Category;
import com.runa.reggie.entity.Dish;
import com.runa.reggie.entity.DishFlavor;
import com.runa.reggie.service.CategoryService;
import com.runa.reggie.service.DishFlavorService;
import com.runa.reggie.service.DishService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.web.bind.annotation.*;import java.util.List;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import java.util.stream.Collectors;/*** 菜品管理*/
@RestController
@RequestMapping("/dish")
@Slf4j
public class DishController {@Autowiredprivate DishService dishService;@Autowiredprivate DishFlavorService dishFlavorService;@Autowiredprivate CategoryService categoryService;@Autowiredprivate RedisTemplate redisTemplate;/*** 新增菜品* @param dishDto* @return*/@PostMappingpublic R<String> save(@RequestBody DishDto dishDto){log.info(dishDto.toString());dishService.saveWithFlavor(dishDto);// 清理Redis所有菜品的缓存数据
// Set keys = redisTemplate.keys("dish_*");// 清理Redis某个分类下的菜品的缓存数据// 动态构造keyString key = "dish_" + dishDto.getCategoryId() + "_1";redisTemplate.delete(key);return R.success("新增菜品成功");}@GetMapping("/page")public R<Page> page(int page, int pageSize, String name){// 构造分页构造器对象Page<Dish> pageInfo = new Page<>(page, pageSize);Page<DishDto> dishDtoPage = new Page<>();//条件构造器LambdaQueryWrapper<Dish> queryWrapper = new LambdaQueryWrapper<>();// 添加过滤条件queryWrapper.like(name != null,Dish::getName,name);// 添加排序条件queryWrapper.orderByDesc(Dish::getUpdateTime);//执行分页查询dishService.page(pageInfo,queryWrapper);// 对象拷贝BeanUtils.copyProperties(pageInfo, dishDtoPage,"records");List<Dish> records = pageInfo.getRecords();List<DishDto> list = records.stream().map((item) -> {DishDto dishDto = new DishDto();BeanUtils.copyProperties(item,dishDto);Long categoryId = item.getCategoryId();// 分类idCategory category = categoryService.getById(categoryId);String categoryName = category.getName();dishDto.setCategoryName(categoryName);return dishDto;}).collect(Collectors.toList());dishDtoPage.setRecords(list);return R.success(dishDtoPage);}/*** 根据ID 查询菜品和对应口味信息 回显* @param id* @return*/@GetMapping("/{id}")public R<DishDto> get(@PathVariable Long id){log.info("要查询显示的菜品信息是:{}",id);DishDto dishDto = dishService.getByIdWithFlavor(id);return R.success(dishDto);}/*** 修改菜品* @param dishDto* @return*/@PutMappingpublic R<String> update(@RequestBody DishDto dishDto){log.info(dishDto.toString());dishService.updateWithFlavor(dishDto);// 清理Redis所有菜品的缓存数据
// Set keys = redisTemplate.keys("dish_*");// 清理Redis某个分类下的菜品的缓存数据// 动态构造keyString key = "dish_" + dishDto.getCategoryId() + "_1";redisTemplate.delete(key);return R.success("修改菜品成功");}// /**
// * 根据条件查询对于菜品数据
// * @param dish
// * @return
// */
// @GetMapping("/list")
// public R<List<Dish>> list(Dish dish){
//
// // 构造查询条件
// LambdaQueryWrapper<Dish> queryWrapper =new LambdaQueryWrapper<>();
// queryWrapper.eq(dish.getCategoryId() != null,Dish::getCategoryId,dish.getCategoryId());
// // 添加条件,查询状态为1(起售状态)的菜品
// queryWrapper.eq(Dish::getStatus,1);
// // 天添加排序条件
// queryWrapper.orderByAsc(Dish::getSort).orderByDesc(Dish::getUpdateTime);
// List<Dish> list = dishService.list(queryWrapper);
// return R.success(list);
// }
//}/*** 根据条件查询对于菜品数据* @param dish* @return*/@GetMapping("/list")public R<List<DishDto>> list(Dish dish){List<DishDto> dishDtoList = null;// 动态构造keyString key = "dish_" + dish.getCategoryId() + "_" + dish.getStatus();// 先从redis获取缓存数据dishDtoList = (List<DishDto>) redisTemplate.opsForValue().get(key);if(dishDtoList != null){// 1 如果存在,直接返回,无需查询数据库return R.success(dishDtoList);}// 2 如果不存在,需要查询数据库,// 构造查询条件LambdaQueryWrapper<Dish> queryWrapper =new LambdaQueryWrapper<>();queryWrapper.eq(dish.getCategoryId() != null,Dish::getCategoryId,dish.getCategoryId());// 添加条件,查询状态为1(起售状态)的菜品queryWrapper.eq(Dish::getStatus,1);// 天添加排序条件queryWrapper.orderByAsc(Dish::getSort).orderByDesc(Dish::getUpdateTime);List<Dish> list = dishService.list(queryWrapper);dishDtoList = list.stream().map((item) -> {DishDto dishDto = new DishDto();BeanUtils.copyProperties(item,dishDto);Long categoryId = item.getCategoryId();// 分类id//根据id查询分类对象Category category = categoryService.getById(categoryId);if(category != null){String categoryName = category.getName();dishDto.setCategoryName(categoryName);}// 当前菜品的idLong dishId = item.getId();LambdaQueryWrapper<DishFlavor> lambdaQueryWrapper = new LambdaQueryWrapper<>();lambdaQueryWrapper.eq(DishFlavor::getDishId,dishId);List<DishFlavor> dishFlavorList = dishFlavorService.list(lambdaQueryWrapper);dishDto.setFlavors(dishFlavorList);return dishDto;}).collect(Collectors.toList());// 2-1 不存在,将查询到的菜品数据缓存到redisredisTemplate.opsForValue().set(key,dishDtoList,60, TimeUnit.MINUTES);return R.success(dishDtoList);}
}
4.3 功能测试
http://localhost:8080/front/page/login.htmlhttp://localhost:8080/backend/index.html
五、Spring Cache
5.1 Spring Cache介绍
5.2 Spring Cache常用注解
5.3 Spring Cache使用方式
5.3.1 pom
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><groupId>com.runa</groupId><artifactId>reggie_take_out_spuer</artifactId><version>1.0-SNAPSHOT</version><packaging>jar</packaging><parent><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-parent</artifactId><version>2.4.5</version><relativePath/> <!-- lookup parent from repository --></parent><name>reggie_take_out_spuer</name><url>http://maven.apache.org</url><properties><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding><java.version>1.8</java.version></properties><dependencies><!--阿里云短信服务--><dependency><groupId>com.aliyun</groupId><artifactId>aliyun-java-sdk-core</artifactId><version>4.5.16</version></dependency><dependency><groupId>com.aliyun</groupId><artifactId>aliyun-java-sdk-dysmsapi</artifactId><version>2.1.0</version></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter</artifactId></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-test</artifactId><scope>test</scope></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId><scope>compile</scope></dependency><dependency><groupId>com.baomidou</groupId><artifactId>mybatis-plus-boot-starter</artifactId><version>3.4.2</version></dependency><dependency><groupId>org.projectlombok</groupId><artifactId>lombok</artifactId><version>1.18.20</version></dependency><dependency><groupId>com.alibaba</groupId><artifactId>fastjson</artifactId><version>1.2.76</version></dependency><dependency><groupId>commons-lang</groupId><artifactId>commons-lang</artifactId><version>2.6</version></dependency><dependency><groupId>mysql</groupId><artifactId>mysql-connector-java</artifactId><scope>runtime</scope></dependency><dependency><groupId>com.alibaba</groupId><artifactId>druid-spring-boot-starter</artifactId><version>1.1.23</version></dependency><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>3.8.1</version><scope>test</scope></dependency><!--redis--><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-redis</artifactId></dependency><!-- spring Cache --><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-cache</artifactId></dependency></dependencies><build><plugins><plugin><groupId>org.springframework.boot</groupId><artifactId>spring-boot-maven-plugin</artifactId><version>2.4.5</version></plugin></plugins></build>
</project>
六、缓存套餐数据
6.1 实现思路
6.2 代码改造
6.2.1 pom 坐标
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><groupId>com.runa</groupId><artifactId>reggie_take_out_spuer</artifactId><version>1.0-SNAPSHOT</version><packaging>jar</packaging><parent><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-parent</artifactId><version>2.4.5</version><relativePath/> <!-- lookup parent from repository --></parent><name>reggie_take_out_spuer</name><url>http://maven.apache.org</url><properties><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding><java.version>1.8</java.version></properties><dependencies><!--阿里云短信服务--><dependency><groupId>com.aliyun</groupId><artifactId>aliyun-java-sdk-core</artifactId><version>4.5.16</version></dependency><dependency><groupId>com.aliyun</groupId><artifactId>aliyun-java-sdk-dysmsapi</artifactId><version>2.1.0</version></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter</artifactId></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-test</artifactId><scope>test</scope></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId><scope>compile</scope></dependency><dependency><groupId>com.baomidou</groupId><artifactId>mybatis-plus-boot-starter</artifactId><version>3.4.2</version></dependency><dependency><groupId>org.projectlombok</groupId><artifactId>lombok</artifactId><version>1.18.20</version></dependency><dependency><groupId>com.alibaba</groupId><artifactId>fastjson</artifactId><version>1.2.76</version></dependency><dependency><groupId>commons-lang</groupId><artifactId>commons-lang</artifactId><version>2.6</version></dependency><dependency><groupId>mysql</groupId><artifactId>mysql-connector-java</artifactId><scope>runtime</scope></dependency><dependency><groupId>com.alibaba</groupId><artifactId>druid-spring-boot-starter</artifactId><version>1.1.23</version></dependency><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>3.8.1</version><scope>test</scope></dependency><!--redis--><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-redis</artifactId></dependency><!-- spring Cache --><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-cache</artifactId></dependency></dependencies><build><plugins><plugin><groupId>org.springframework.boot</groupId><artifactId>spring-boot-maven-plugin</artifactId><version>2.4.5</version></plugin></plugins></build>
</project>
6.2.2 application.yml配置缓存数据过期时间
server:port: 8080
spring:application:# 应用名称 可选name: reggie_take_out_spuerdatasource:druid:driver-class-name: com.mysql.cj.jdbc.Driverurl: jdbc:mysql://localhost:3306/reggie?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf-8&zeroDateTimeBehavior=convertToNull&useSSL=false&allowPublicKeyRetrieval=trueusername: rootpassword: runa#2050redis:host: 127.0.0.1port: 6379password: 123456database: 0cache:redis:time-to-live: 1800000 # 设置缓存数据的过期时间
mybatis-plus:configuration:#在映射实体或者属性时,将数据库中表名和字段名中的下划线去掉,按照驼峰命名法映射map-underscore-to-camel-case: truelog-impl: org.apache.ibatis.logging.stdout.StdOutImplglobal-config:db-config:id-type: ASSIGN_ID
# 文件上传目录
reggie:path: D:\images\
6.2.3 开启缓存注解功能 ReggieApplication
package com.runa.reggie;import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletComponentScan;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.transaction.annotation.EnableTransactionManagement;@Slf4j
@SpringBootApplication
@ServletComponentScan
@EnableTransactionManagement
@EnableCaching // 开启spring Cache注解方式的缓存功能
public class ReggieApplication {public static void main(String[] args) {SpringApplication.run(ReggieApplication.class,args);log.info("项目启动成功~~~");}
}
6.2.4 R实现 Serializable 接口
package com.runa.reggie.common;import lombok.Data;import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;/*** 通用返回结果,服务端响应的数据最终都会封装成此对象* @param <T>*/
@Data
public class R<T> implements Serializable {private Integer code; //编码:1成功,0和其它数字为失败private String msg; //错误信息private T data; //数据private Map map = new HashMap(); //动态数据public static <T> R<T> success(T object) {R<T> r = new R<T>();r.data = object;r.code = 1;return r;}public static <T> R<T> error(String msg) {R r = new R();r.msg = msg;r.code = 0;return r;}public R<T> add(String key, Object value) {this.map.put(key, value);return this;}}
6.2.5 在SetmealController的list方法加入@Cacheable注解
package com.runa.reggie.controller;import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.runa.reggie.common.R;
import com.runa.reggie.dto.SetmealDto;
import com.runa.reggie.entity.Category;
import com.runa.reggie.entity.Setmeal;
import com.runa.reggie.service.CategoryService;
import com.runa.reggie.service.SetmealDishService;
import com.runa.reggie.service.SetmealService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.web.bind.annotation.*;import java.util.List;
import java.util.stream.Collectors;@RestController
@RequestMapping("/setmeal")
@Slf4j
public class SetmealController {@Autowiredprivate SetmealService setmealService;@Autowiredprivate CategoryService categoryService;@Autowiredprivate SetmealDishService setmealDishService;/*** 新增套餐* @param setmealDto* @return*/@PostMappingpublic R<String> save(@RequestBody SetmealDto setmealDto){log.info("套餐信息:{}",setmealDto);setmealService.saveWithDish(setmealDto);return R.success("新增套餐成功");}/*** 套餐分页查询* @param page* @param pageSize* @param name* @return*/@GetMapping("/page")public R<Page> page(int page, int pageSize, String name){//分页构造器对象Page<Setmeal> pageInfo = new Page<>(page,pageSize);Page<SetmealDto> dtoPage = new Page<>();LambdaQueryWrapper<Setmeal> queryWrapper = new LambdaQueryWrapper<>();//添加查询条件,根据name进行like模糊查询queryWrapper.like(name != null,Setmeal::getName,name);//添加排序条件,根据更新时间降序排列queryWrapper.orderByDesc(Setmeal::getUpdateTime);setmealService.page(pageInfo,queryWrapper);//对象拷贝BeanUtils.copyProperties(pageInfo,dtoPage,"records");List<Setmeal> records = pageInfo.getRecords();List<SetmealDto> list = records.stream().map((item) -> {SetmealDto setmealDto = new SetmealDto();//对象拷贝BeanUtils.copyProperties(item,setmealDto);//分类idLong categoryId = item.getCategoryId();//根据分类id查询分类对象Category category = categoryService.getById(categoryId);if(category != null){//分类名称String categoryName = category.getName();setmealDto.setCategoryName(categoryName);}return setmealDto;}).collect(Collectors.toList());dtoPage.setRecords(list);return R.success(dtoPage);}/*** 删除套餐* @param ids* @return*/@DeleteMappingpublic R<String> delete(@RequestParam List<Long> ids){log.info("ids:{}",ids);setmealService.removeWithDish(ids);return R.success("套餐数据删除成功");}/*** 根据条件查询套餐数据* @param setmeal* @return*/@GetMapping("/list")@Cacheable(value = "setmealCache", key = "#setmeal.categoryId + '_' + #setmeal.status")public R<List<Setmeal>> list(Setmeal setmeal){LambdaQueryWrapper<Setmeal> queryWrapper = new LambdaQueryWrapper<>();queryWrapper.eq(setmeal.getCategoryId() != null,Setmeal::getCategoryId,setmeal.getCategoryId());queryWrapper.eq(setmeal.getStatus() != null,Setmeal::getStatus,setmeal.getStatus());queryWrapper.orderByDesc(Setmeal::getUpdateTime);List<Setmeal> list = setmealService.list(queryWrapper);return R.success(list);}}
6.2.6 在SetmealController的delete方法加入@CacheEvict注解
package com.runa.reggie.controller;import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.runa.reggie.common.R;
import com.runa.reggie.dto.SetmealDto;
import com.runa.reggie.entity.Category;
import com.runa.reggie.entity.Setmeal;
import com.runa.reggie.service.CategoryService;
import com.runa.reggie.service.SetmealDishService;
import com.runa.reggie.service.SetmealService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.web.bind.annotation.*;import java.util.List;
import java.util.stream.Collectors;@RestController
@RequestMapping("/setmeal")
@Slf4j
public class SetmealController {@Autowiredprivate SetmealService setmealService;@Autowiredprivate CategoryService categoryService;@Autowiredprivate SetmealDishService setmealDishService;/*** 新增套餐* @param setmealDto* @return*/@PostMappingpublic R<String> save(@RequestBody SetmealDto setmealDto){log.info("套餐信息:{}",setmealDto);setmealService.saveWithDish(setmealDto);return R.success("新增套餐成功");}/*** 套餐分页查询* @param page* @param pageSize* @param name* @return*/@GetMapping("/page")public R<Page> page(int page, int pageSize, String name){//分页构造器对象Page<Setmeal> pageInfo = new Page<>(page,pageSize);Page<SetmealDto> dtoPage = new Page<>();LambdaQueryWrapper<Setmeal> queryWrapper = new LambdaQueryWrapper<>();//添加查询条件,根据name进行like模糊查询queryWrapper.like(name != null,Setmeal::getName,name);//添加排序条件,根据更新时间降序排列queryWrapper.orderByDesc(Setmeal::getUpdateTime);setmealService.page(pageInfo,queryWrapper);//对象拷贝BeanUtils.copyProperties(pageInfo,dtoPage,"records");List<Setmeal> records = pageInfo.getRecords();List<SetmealDto> list = records.stream().map((item) -> {SetmealDto setmealDto = new SetmealDto();//对象拷贝BeanUtils.copyProperties(item,setmealDto);//分类idLong categoryId = item.getCategoryId();//根据分类id查询分类对象Category category = categoryService.getById(categoryId);if(category != null){//分类名称String categoryName = category.getName();setmealDto.setCategoryName(categoryName);}return setmealDto;}).collect(Collectors.toList());dtoPage.setRecords(list);return R.success(dtoPage);}/*** 删除套餐* @param ids* @return*/@DeleteMapping@CacheEvict(value = "setmealCache" , allEntries = true)public R<String> delete(@RequestParam List<Long> ids){log.info("ids:{}",ids);setmealService.removeWithDish(ids);return R.success("套餐数据删除成功");}/*** 根据条件查询套餐数据* @param setmeal* @return*/@GetMapping("/list")@Cacheable(value = "setmealCache", key = "#setmeal.categoryId + '_' + #setmeal.status")public R<List<Setmeal>> list(Setmeal setmeal){LambdaQueryWrapper<Setmeal> queryWrapper = new LambdaQueryWrapper<>();queryWrapper.eq(setmeal.getCategoryId() != null,Setmeal::getCategoryId,setmeal.getCategoryId());queryWrapper.eq(setmeal.getStatus() != null,Setmeal::getStatus,setmeal.getStatus());queryWrapper.orderByDesc(Setmeal::getUpdateTime);List<Setmeal> list = setmealService.list(queryWrapper);return R.success(list);}}
6.2.7 在SetmealController的save方法加入@CacheEvict注解
package com.runa.reggie.controller;import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.runa.reggie.common.R;
import com.runa.reggie.dto.SetmealDto;
import com.runa.reggie.entity.Category;
import com.runa.reggie.entity.Setmeal;
import com.runa.reggie.service.CategoryService;
import com.runa.reggie.service.SetmealDishService;
import com.runa.reggie.service.SetmealService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.web.bind.annotation.*;import java.util.List;
import java.util.stream.Collectors;@RestController
@RequestMapping("/setmeal")
@Slf4j
public class SetmealController {@Autowiredprivate SetmealService setmealService;@Autowiredprivate CategoryService categoryService;@Autowiredprivate SetmealDishService setmealDishService;/*** 新增套餐* @param setmealDto* @return*/@PostMapping@CacheEvict(value = "setmealCache" , allEntries = true)public R<String> save(@RequestBody SetmealDto setmealDto){log.info("套餐信息:{}",setmealDto);setmealService.saveWithDish(setmealDto);return R.success("新增套餐成功");}/*** 套餐分页查询* @param page* @param pageSize* @param name* @return*/@GetMapping("/page")public R<Page> page(int page, int pageSize, String name){//分页构造器对象Page<Setmeal> pageInfo = new Page<>(page,pageSize);Page<SetmealDto> dtoPage = new Page<>();LambdaQueryWrapper<Setmeal> queryWrapper = new LambdaQueryWrapper<>();//添加查询条件,根据name进行like模糊查询queryWrapper.like(name != null,Setmeal::getName,name);//添加排序条件,根据更新时间降序排列queryWrapper.orderByDesc(Setmeal::getUpdateTime);setmealService.page(pageInfo,queryWrapper);//对象拷贝BeanUtils.copyProperties(pageInfo,dtoPage,"records");List<Setmeal> records = pageInfo.getRecords();List<SetmealDto> list = records.stream().map((item) -> {SetmealDto setmealDto = new SetmealDto();//对象拷贝BeanUtils.copyProperties(item,setmealDto);//分类idLong categoryId = item.getCategoryId();//根据分类id查询分类对象Category category = categoryService.getById(categoryId);if(category != null){//分类名称String categoryName = category.getName();setmealDto.setCategoryName(categoryName);}return setmealDto;}).collect(Collectors.toList());dtoPage.setRecords(list);return R.success(dtoPage);}/*** 删除套餐* @param ids* @return*/@DeleteMapping@CacheEvict(value = "setmealCache" , allEntries = true)public R<String> delete(@RequestParam List<Long> ids){log.info("ids:{}",ids);setmealService.removeWithDish(ids);return R.success("套餐数据删除成功");}/*** 根据条件查询套餐数据* @param setmeal* @return*/@GetMapping("/list")@Cacheable(value = "setmealCache", key = "#setmeal.categoryId + '_' + #setmeal.status")public R<List<Setmeal>> list(Setmeal setmeal){LambdaQueryWrapper<Setmeal> queryWrapper = new LambdaQueryWrapper<>();queryWrapper.eq(setmeal.getCategoryId() != null,Setmeal::getCategoryId,setmeal.getCategoryId());queryWrapper.eq(setmeal.getStatus() != null,Setmeal::getStatus,setmeal.getStatus());queryWrapper.orderByDesc(Setmeal::getUpdateTime);List<Setmeal> list = setmealService.list(queryWrapper);return R.success(list);}}
6.3 功能测试
http://localhost:8080/front/page/login.htmlhttp://localhost:8080/backend/index.html
相关文章:
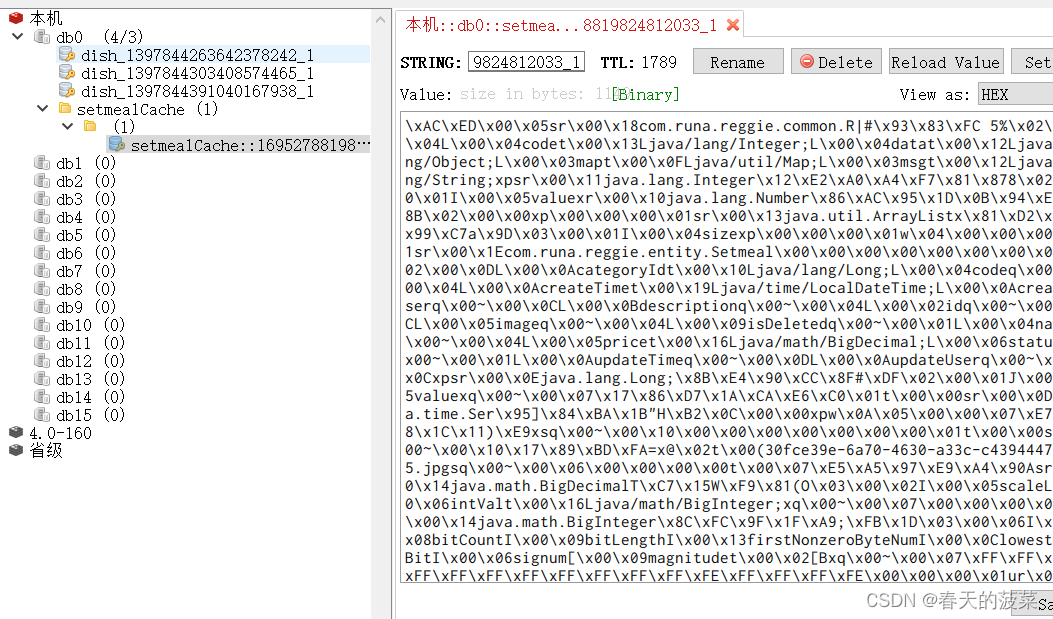
【java】【项目实战】[外卖九]项目优化(缓存)
目录 一、问题说明 二、环境搭建 2.1 Git管理代码 2.1.1 创建本地仓库 2.1.2 创建远程仓库 2.1.3 创建分支--》推送到远程仓库 2.2 maven坐标 2.3 配置文件application.yml 2.4 配置类RedisConfig 三、缓存短信验证码 3.1 实现思路 3.2 代码改造 3.2.1 UserContro…...

Scala集合常用函数与集合计算简单函数,高级计算函数Map和Reduce等
Scala集合常用函数与集合计算简单函数 1.Scala集合常用函数 基本属性和常用操作 1.常用函数: (1) 获取集合长度(2) 获取集合大小(3) 循环遍历(4) 迭代器(…...
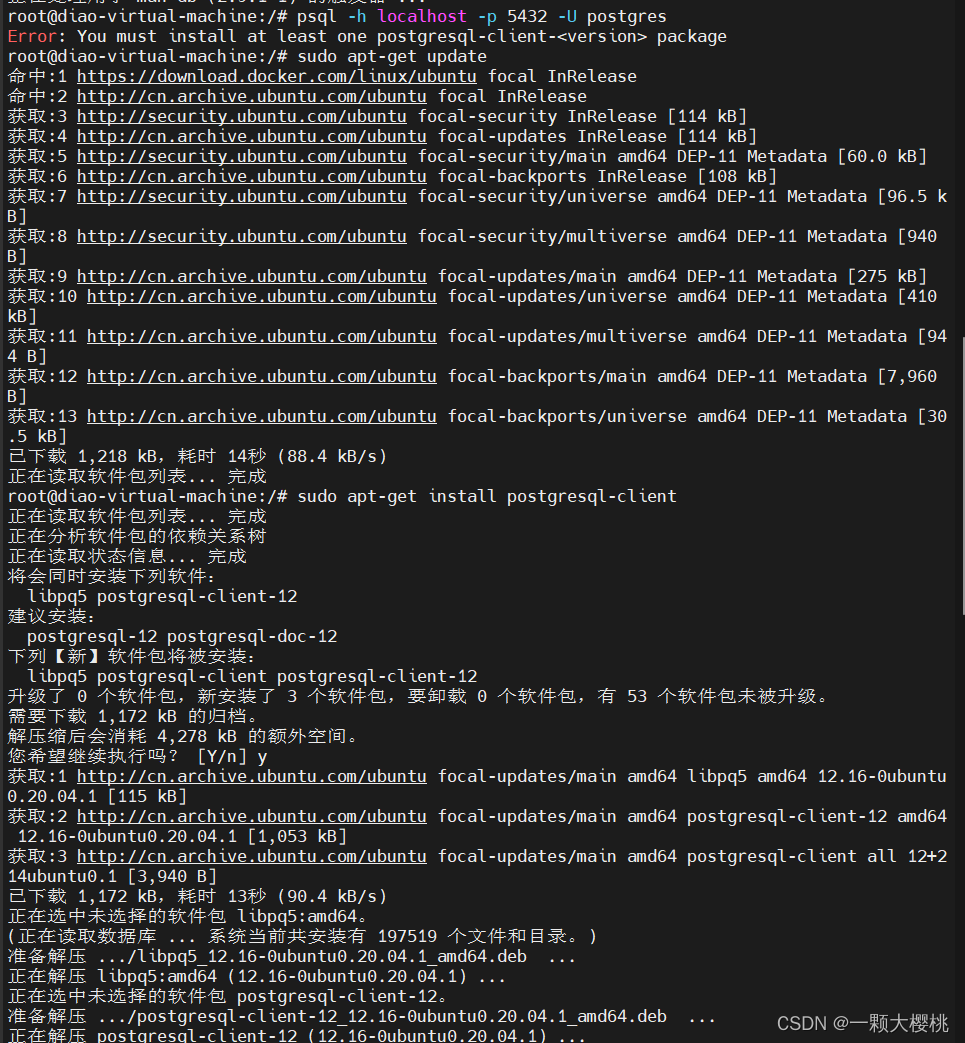
You must install at least one postgresql-client-<version> package
使用主机上的映射端口来连接到 PostgreSQL 数据库。例如,使用以下命令连接到数据库: psql -h localhost -p 5432 -U postgres出现下面的问题: 分析: 如果您在运行 psql 命令时遇到错误消息 You must install at least one pos…...
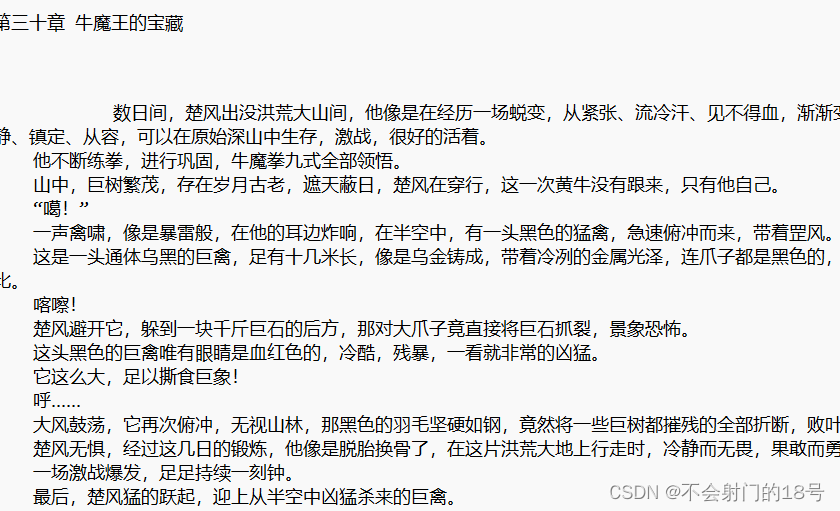
爬虫源码---爬取自己想要看的小说
前言: 小说作为在自己空闲时间下的消遣工具,对我们打发空闲时间很有帮助,而我们在网站上面浏览小说时会被广告和其他一些东西影响我们的观看体验,而这时我们就可以利用爬虫将我们想要观看的小说下载下来,这样就不会担…...

【AGC】云数据库API9开发问题汇总
【问题描述】 云数据库HarmonyOS API9 SDK已经推出了一段时间了,下面为大家汇总一些在集成使用中遇到的问题和解决方案。 【问题分析】 1. 报错信息:数据库初始化失败:{“message”:“The object type list and permission …...
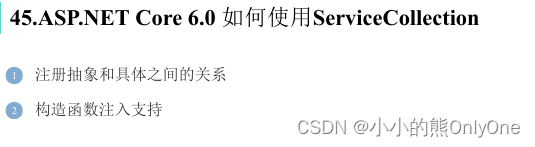
ASP.NET Core IOC容器
//IOC容器支持依赖注入{ServiceCollection serviceDescriptors new ServiceCollection();serviceDescriptors.AddTransient<IMicrophone, Microphone>();serviceDescriptors.AddTransient<IPower, Power>();serviceDescriptors.AddTransient<IHeadphone, Headp…...
入门力扣自学笔记277 C++ (题目编号:42)(动态规划)
42. 接雨水 题目: 给定 n 个非负整数表示每个宽度为 1 的柱子的高度图,计算按此排列的柱子,下雨之后能接多少雨水。 示例 1: 输入:height [0,1,0,2,1,0,1,3,2,1,2,1] 输出:6 解释:上面是由数组…...
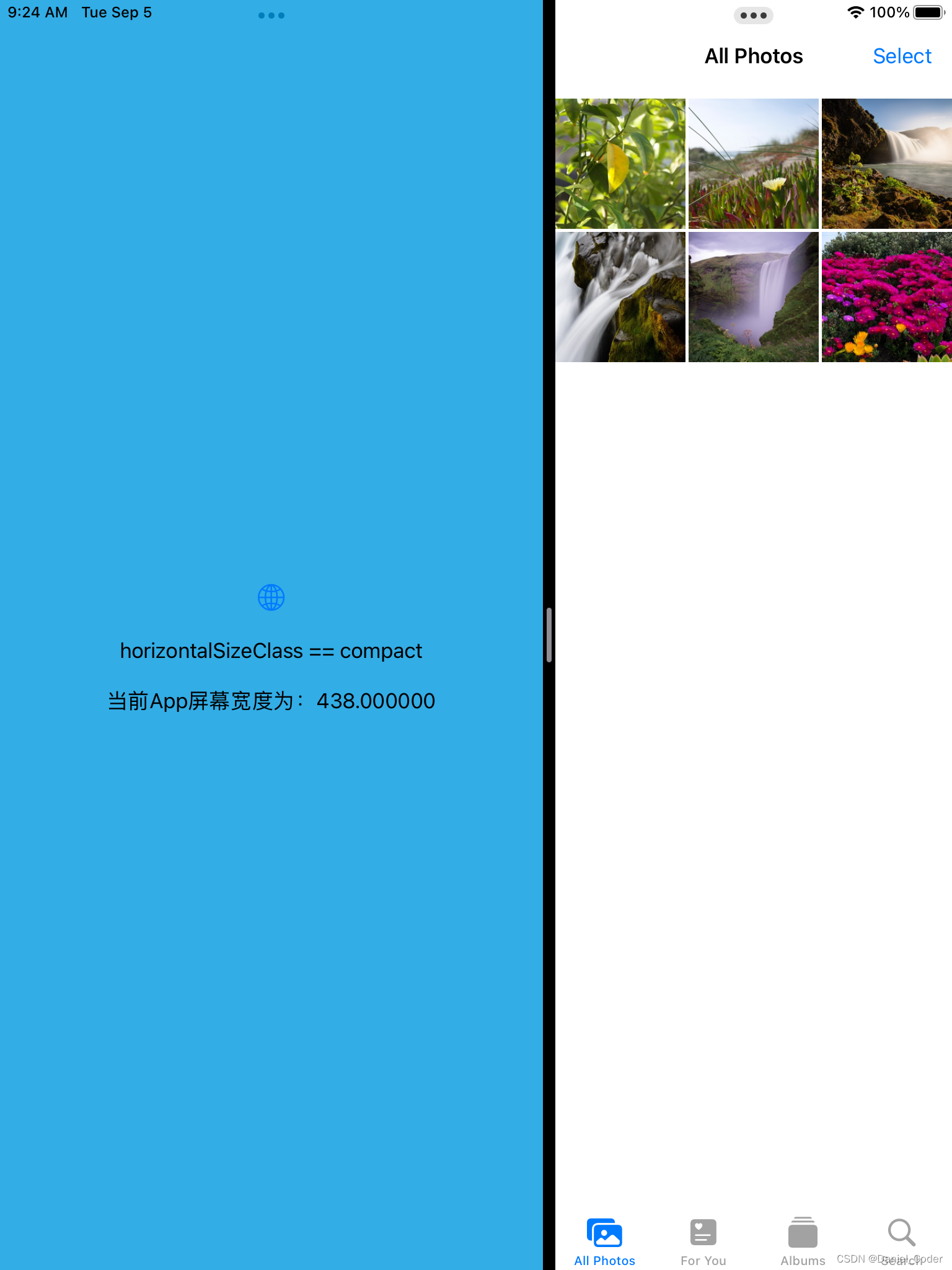
SwiftUI实现iPad多任务分屏
1. 概述 iPadOS引入了多任务分屏功能,使用户能够同时在一个屏幕上使用多个应用程序。这为用户提供了更高效的工作环境,可以在同一时间处理多个任务。 iPad多任务分屏有两种常见的模式:1/2分屏和Slide Over(滑动覆盖)…...

maven依赖,继承
依赖的范围 compile引入的依赖 对main目录下的代码有没有效,main目录下的代码能不能用compile引入的依赖中的类等 以test引入的依赖,在main中是否可以使用 provided(已提供),有了就不要带到服务器上,打包…...
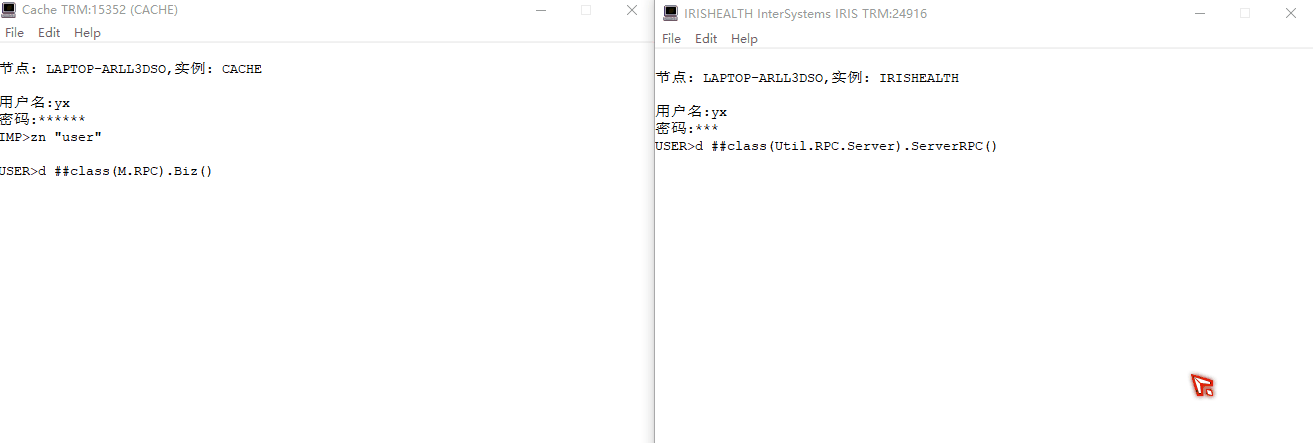
仿`gRPC`功能实现像调用本地方法一样调用其他服务器方法
文章目录 仿gRPC功能实现像调用本地方法一样调用其他服务器方法 简介单体架构微服务架构RPCgPRC gRPC交互逻辑服务端逻辑客户端逻辑示例图 原生实现仿gRPC框架编写客户端方法编写服务端方法综合演示 仿 gRPC功能实现像调用本地方法一样调用其他服务器方法 简介 在介绍gRPC简介…...
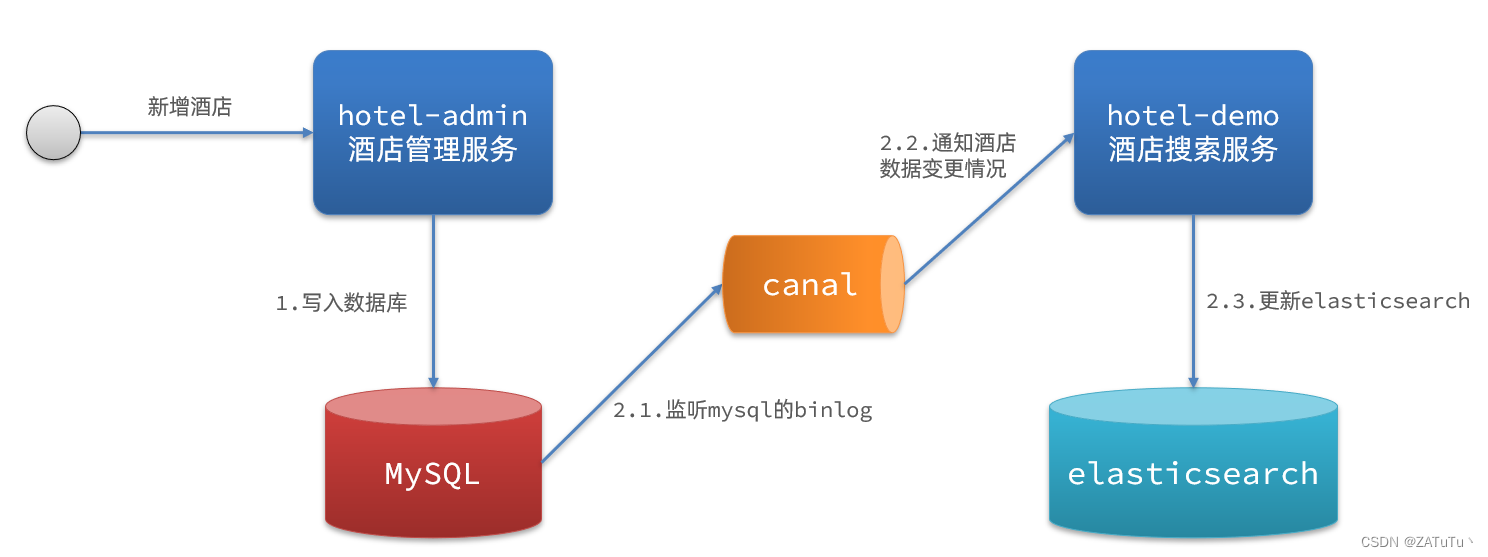
分布式环境下的数据同步
一般而言elasticsearch负责搜索(查询),而sql数据负责记录(增删改),elasticsearch中的数据来自于sql数据库,因此sql数据发生改变时,elasticsearch也必须跟着改变,这个就是…...
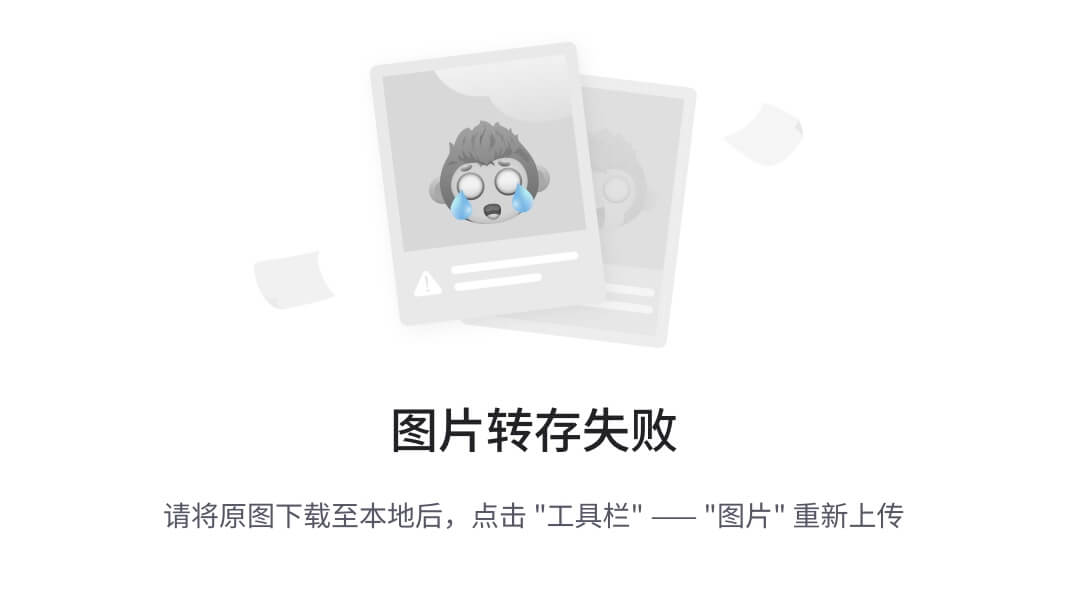
无涯教程-Flutter - 数据库
SQLite" class"css-1occaib">SQLite数据库是基于事实和标准SQL的嵌入式数据库引擎,它是小型且经过时间考验的数据库引擎,sqflite软件包提供了许多函数,可以有效地与SQLite数据库一起使用,它提供了操作SQLite数据…...
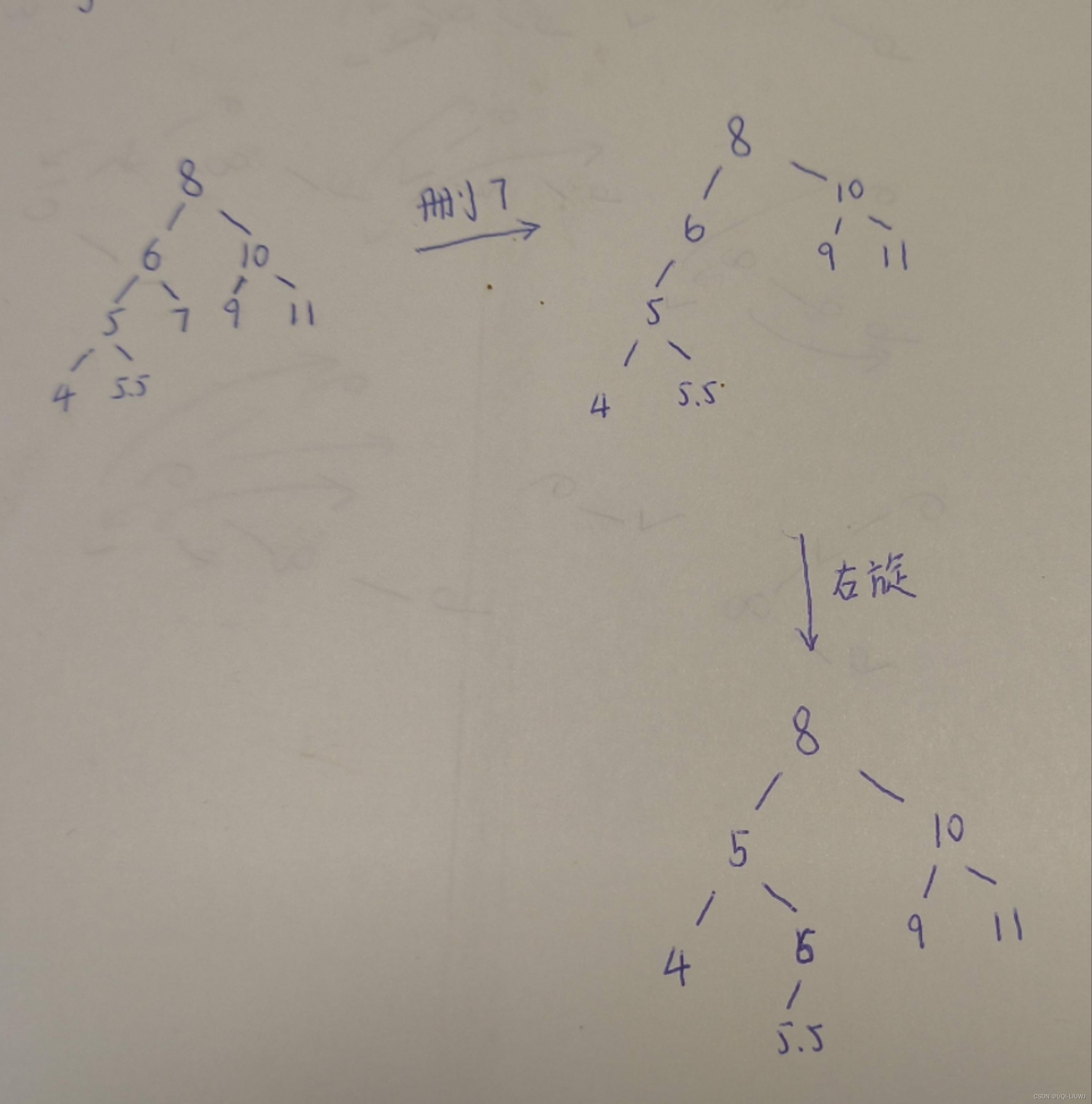
算法笔记:平衡二叉树
1 介绍 平衡二叉树(AVL树)是一种特殊的二叉搜索树(BST),它自动确保树保持低高度,以便实现各种基本操作(如添加、删除和查找)的高效性能。 ——>时间都维持在了O(logN)它是一棵空…...

redis 通用命令
目录 通用命令是什么 SET & GET keys EXISTS DEL EXPIRE TTL redis 的过期策略 定时器策略 基于优先级队列定时器 基于时间轮的定时器 TYPE 通过 redis 客户端和 redis 服务器交互。 所以需要使用 redis 的命令,但是 redis 的命令非常多。 通用命令…...
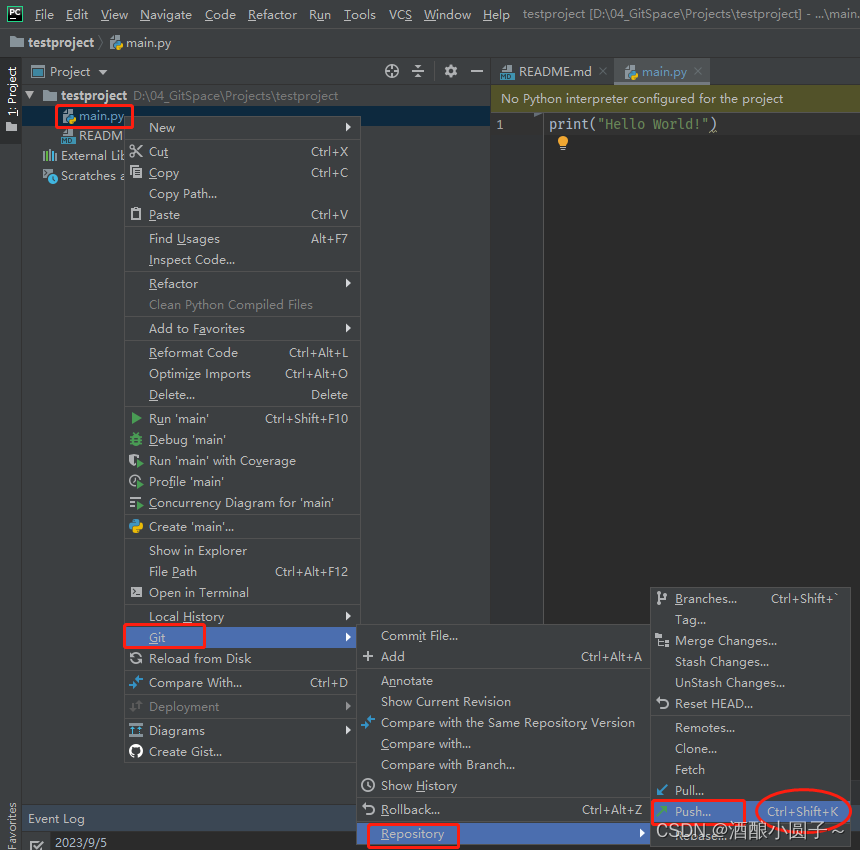
Pycharm配置及使用Git教程
文章目录 1. 安装PyCharm2. 安装Git3. 在PyCharm中配置Git插件4. 连接远程Gtilab仓库5. Clone项目代码6. 将本地文件提交到远程仓库6.1 git add6.2 git commit6.3 git push6.4 git pull 平时习惯在windows下开发,但是我们又需要实时将远方仓库的代码clone到本地&…...

CSS transition 过渡
1 前言 水平居中、垂直居中是前端面试百问不厌的问题。 其实现方案也是多种多样,常叫人头昏眼花。 水平方向可以认为是内联方向,垂直方向认为是块级方向。 下面介绍一些常见的方法。 2 内联元素的水平垂直居中 首先,常见内联元素有&…...
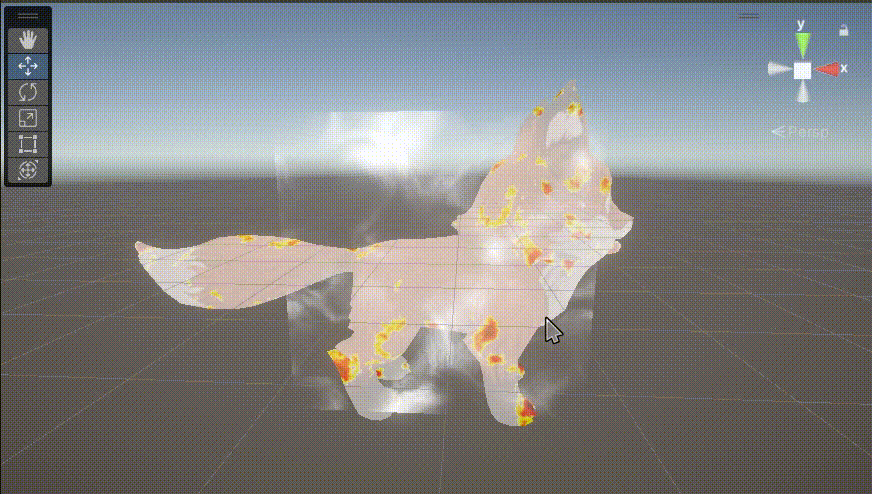
Unity中Shader的UV扭曲效果的实现
文章目录 前言一、实现的思路1、在属性面板暴露一个 扭曲贴图的属性2、在片元结构体中,新增一个float2类型的变量,用于独立存储将用于扭曲的纹理的信息3、在顶点着色器中,根据需要使用TRANSFORM_TEX对Tilling 和 Offset 插值;以及…...
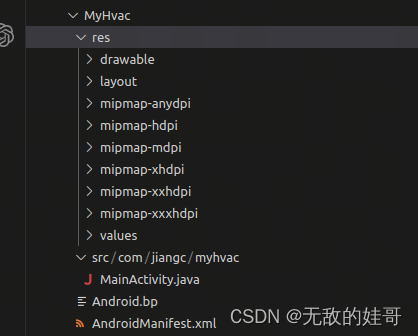
Automotive 添加一个特权APP
Automotive 添加一个特权APP platform: android-13.0.0_r32 一. 添加一个自定义空调的app为例 路径:packages/apps/Car/MyHvac app内容可以自己定义,目录结构如下: 1.1 Android.bp package {default_applicable_licenses: ["Andr…...

自定义TimeLine
自定义TimeLine 什么是TimeLineData(数据)Clip(片段)Track(轨道)Mixer(混合) 什么是TimeLine 在 Unity 中,TimeLine(时间轴)是一种用于创建和管理…...

如何使用SQL系列 之 如何在SQL中使用WHERE条件语句
引言 在结构化查询语言 (SQL)语句中,WHERE子句限制了给定操作会影响哪些行。它们通过定义特定的条件(称为搜索条件)来实现这一点,每一行都必须满足这些条件才能受到操作的影响。 本指南将介绍WHERE子句中使用的通用语法。它还将概述如何在单个WHERE子句…...
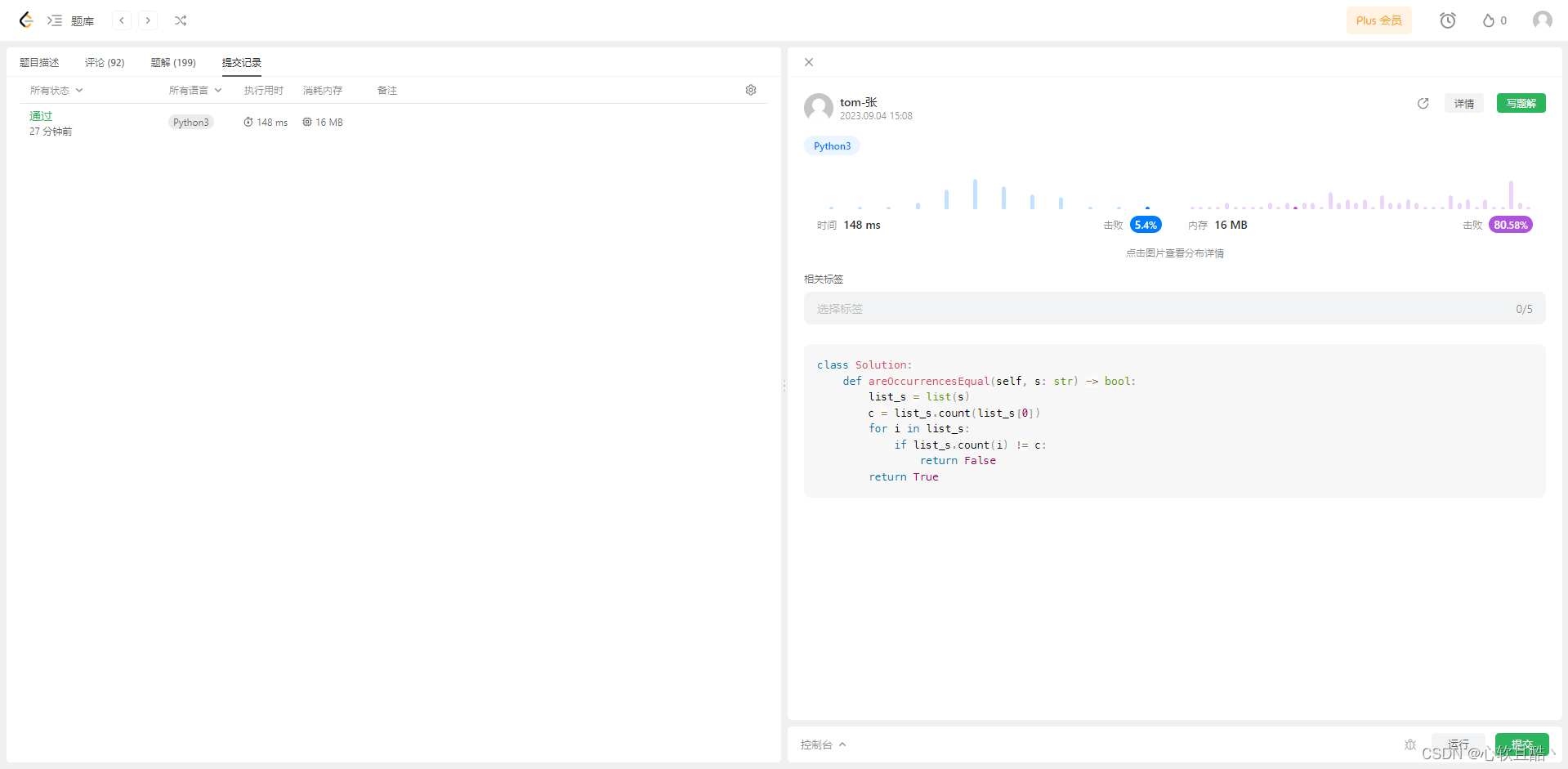
leetcode:1941. 检查是否所有字符出现次数相同(python3解法)
难度:简单 给你一个字符串 s ,如果 s 是一个 好 字符串,请你返回 true ,否则请返回 false 。 如果 s 中出现过的 所有 字符的出现次数 相同 ,那么我们称字符串 s 是 好 字符串。 示例 1: 输入:s…...

Echarts 各种点击事件监听
目录 一、鼠标事件1.1、左击1.2、双击1.3、右击1.4、右键双击1.5、中轴滚动二、时间轴2.1、时间轴监听三、拖动3.1、拖动事件一、鼠标事件 1.1、左击 chart.on(click, function(params)...
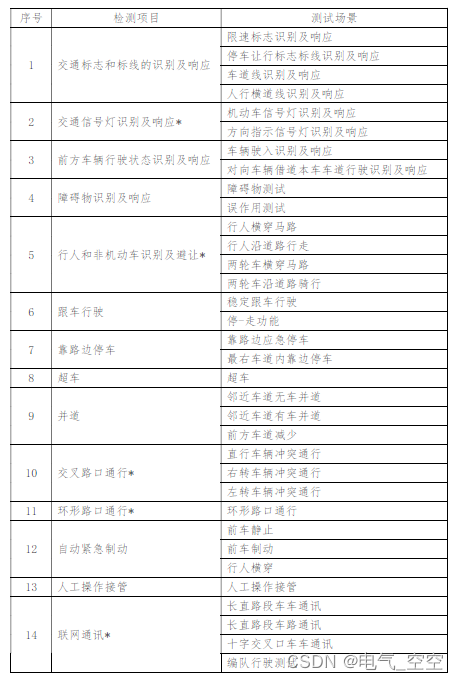
《智能网联汽车自动驾驶功能测试规程》
一、 编制背景 2018 年4 月12 日,工业和信息化部、公安部、交通运输部联合发布《智能网联汽车道路测试管理规范(试行)》(以下简称《管理规范》),对智能网联汽车道路测试申请、审核、管理以及测试主体、测试驾驶人和测试车辆要求等…...
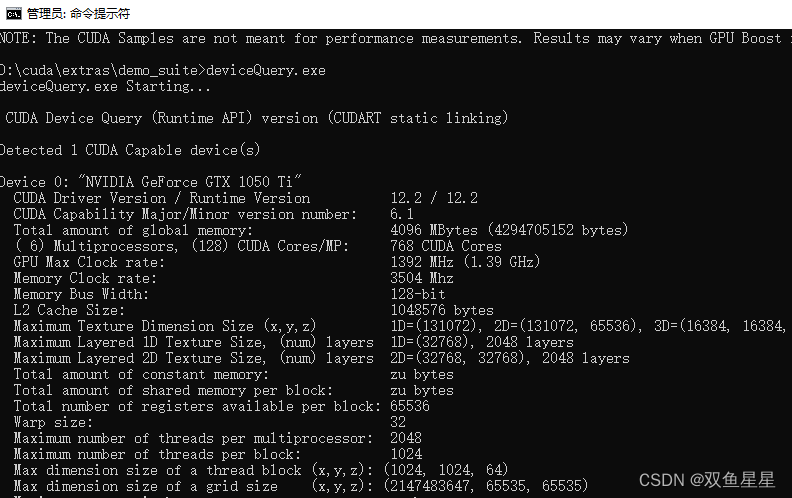
NVIDIA CUDA Win10安装步骤
前言 windows10 版本安装 CUDA ,首先需要下载两个安装包 CUDA toolkit(toolkit就是指工具包)cuDNN 1. 安装前准备 在安装CUDA之前,需要完成以下准备工作: 确认你的显卡已经正确安装,在设备管理器中可以看…...
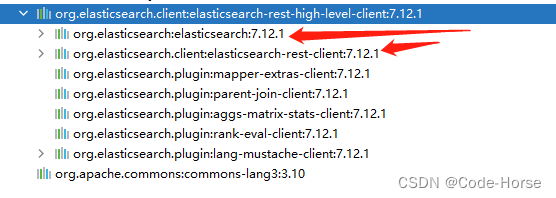
Elasticsearch、Kibana以及Java操作ES 的快速使用
docker 安装elastic search 、 kibana(可视化管理elastic search) docker pull elasticsearch:7.12.1 docker pull kibana:7.12.1创建docker自定义网络 docker自定义网络可以使得容器之间使用容器名网络互连,默认的网络不会有这功能。 一定…...

逐鹿人形机器人,百度、腾讯、小米卷起来
长期不温不火的人形机器人产业迎来新风口,技术显著提升、新品层出不穷、资本投资态度也逐渐好转。 8月18日,2023世界机器人大会博览会正式开放,全面展示了机器人行业的新技术、新产品和新应用。据悉,此次展会展览总面积达4.5万平…...

AndroidStudio推荐下载和配置
1、推荐下载链接 Download Android Studio & App Tools - Android Developers 2、gradle配置案例 // Top-level build file where you can add configuration options common to all sub-projects/modules.buildscript {repositories {maven { url https://maven.aliyun.…...

mysql异常占用资源排查
通过执行日志与连接信息排查 查看是否开启日志记录 mysql> show global variables like %general%; --------------------------------- | Variable_name | Value | --------------------------------- | general_log | OFF | | general_log_file…...

requests 库:发送 form-data 格式的 http 请求 (python)
安装 requests-toolbelt !pip install requests-toolbeltdemo from requests_toolbelt import MultipartEncoder import requestsm MultipartEncoder(fields{query: """第一,向量化匹配是有能力上限的。搜索引擎实现语义搜索已经是好几年的事情了…...
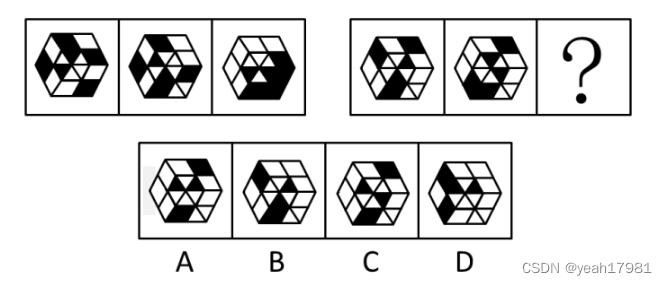
行测图形推理规律(一)元素组成
题库:粉笔网题库 (fenbi.com) 不知道和测评的行测题库是不是一样的,但是总结的规律应该是一样的。 规律并不唯一,题库的答案也只是参考答案,切勿当杠精,你觉得你的规律更合适就别管。本人所归纳的规律仅代表本人想法…...

软件外包属于什么行业/seo常用分析的专业工具
前言 本教程写了这个效果图的demo,同时总结CABasicAnimation的使用方法。 看完gif动画完,看到了什么?平移、旋转、缩放、闪烁、路径动画。 实现平移动画 实现平移动画,我们可以通过transform.translation或者水平transform.transl…...
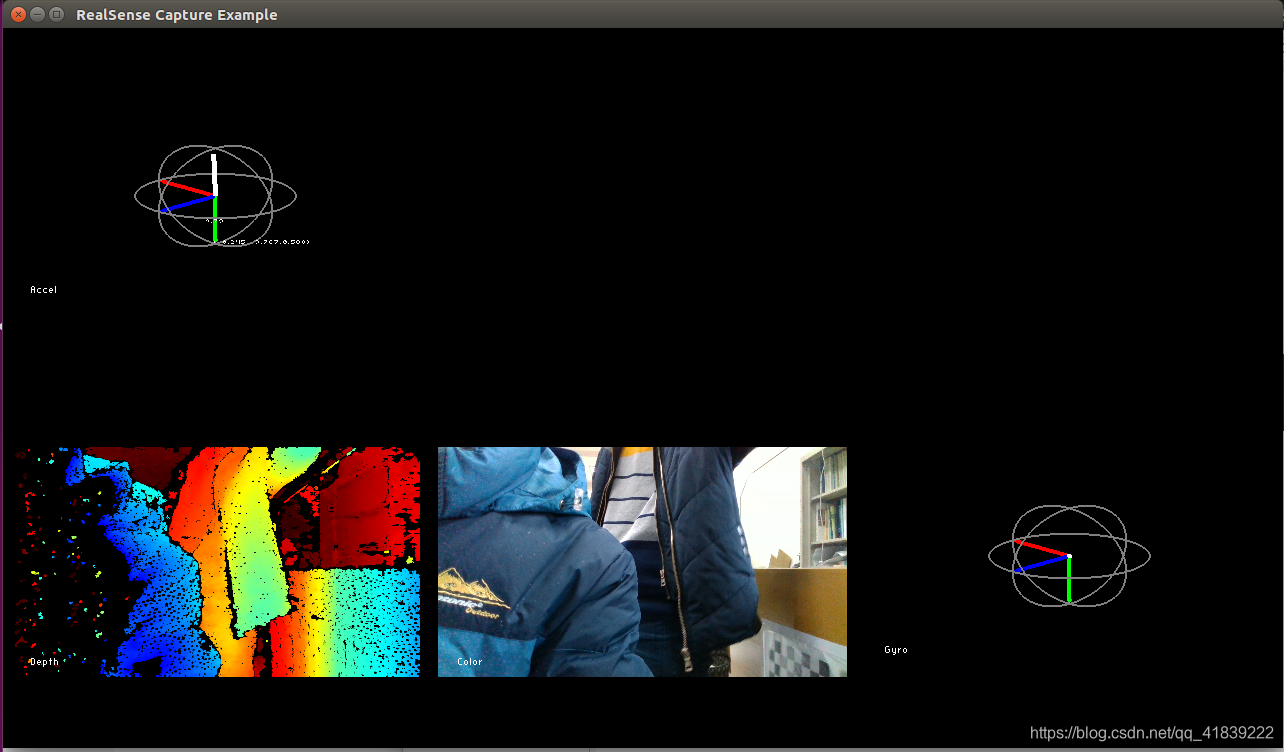
柳州网站制作/引流推广方案
转自:https://blog.csdn.net/qq_41839222/article/details/86503113 前言 等了一个月的realsense d435i终于发货了! 这款D435i(见下图)在D435的基础上,另外搭载了博世的惯性测量单元(IMU)&…...
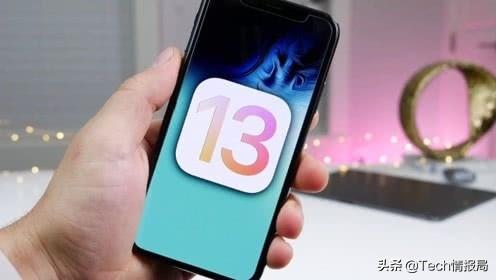
医疗网站建设公司/关键词优化快速排名
随着时代和科技的快速发展和进步,智能手机已成为日常生活中的一件必需品。而今的智能手机除了外观设计、性价比,最重要的还有处理器和系统。目前主要的系统还是安卓,以及苹果的iOS,而手机在使用一段时间后,都会收到系统…...

wordpress数据库设计优缺点/怎么优化标题和关键词排名
2019独角兽企业重金招聘Python工程师标准>>> 在 输入/输出流体系中,有两个特殊的流 PushbackInputStreamPushbackReader方法: void unread(byte[]/char[] buf) : 将一个字节或者字符数组内容推回到推回缓冲区中,从而可以重复读取刚…...

wordpress菜单栏设置/百度电脑版下载
IntelliJ IDEA 设置代码提示或自动补全的快捷键 (附IntelliJ IDEA常用快捷键) 转载博客园:https://www.cnblogs.com/jx17/p/6244491.html 修改方法如下: 点击 文件菜单(File) –> 点击 设置(Settings… CtrlAltS), –> 打开设置对话框。 在左侧…...
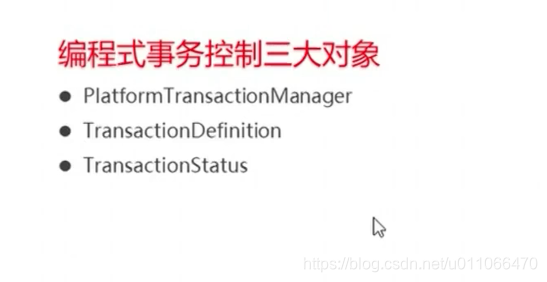
wordpress手机版如何设置密码/八八网
一.基本信息 1.1 基本常识 1.2 事务隔离级别 1.3 事务的传播行为 A业务方法调用B业务方法,如果B看A没有业务方法,则新建一个业务方法,如果B看A业务方法,则B就加入到A的事务方法中。 1.4 事务的状态 1.5 总结...