C# Onnx E2Pose人体关键点检测
C# Onnx E2Pose人体关键点检测
目录
效果
模型信息
项目
代码
下载
效果
模型信息
Inputs
-------------------------
name:inputimg
tensor:Float[1, 3, 512, 512]
---------------------------------------------------------------
Outputs
-------------------------
name:kvxy/concat
tensor:Float[1, 341, 17, 3]
name:pv/concat
tensor:Float[1, 341, 1, 1]
---------------------------------------------------------------
项目
代码
using Microsoft.ML.OnnxRuntime;
using Microsoft.ML.OnnxRuntime.Tensors;
using OpenCvSharp;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Imaging;
using System.Linq;
using System.Windows.Forms;
namespace Onnx_Demo
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
string fileFilter = "*.*|*.bmp;*.jpg;*.jpeg;*.tiff;*.tiff;*.png";
string image_path = "";
string startupPath;
DateTime dt1 = DateTime.Now;
DateTime dt2 = DateTime.Now;
string model_path;
Mat image;
Mat result_image;
SessionOptions options;
InferenceSession onnx_session;
Tensor<float> input_tensor;
List<NamedOnnxValue> input_container;
IDisposableReadOnlyCollection<DisposableNamedOnnxValue> result_infer;
DisposableNamedOnnxValue[] results_onnxvalue;
Tensor<float> result_tensors;
int inpHeight, inpWidth;
float confThreshold;
int[] connect_list = { 0, 1, 0, 2, 1, 3, 2, 4, 3, 5, 4, 6, 5, 6, 5, 7, 7, 9, 6, 8, 8, 10, 5, 11, 6, 12, 11, 12, 11, 13, 13, 15, 12, 14, 14, 16 };
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog ofd = new OpenFileDialog();
ofd.Filter = fileFilter;
if (ofd.ShowDialog() != DialogResult.OK) return;
pictureBox1.Image = null;
image_path = ofd.FileName;
pictureBox1.Image = new Bitmap(image_path);
textBox1.Text = "";
image = new Mat(image_path);
pictureBox2.Image = null;
}
unsafe private void button2_Click(object sender, EventArgs e)
{
if (image_path == "")
{
return;
}
button2.Enabled = false;
pictureBox2.Image = null;
textBox1.Text = "";
Application.DoEvents();
//读图片
image = new Mat(image_path);
//将图片转为RGB通道
Mat image_rgb = new Mat();
Cv2.CvtColor(image, image_rgb, ColorConversionCodes.BGR2RGB);
Cv2.Resize(image_rgb, image_rgb, new OpenCvSharp.Size(inpHeight, inpWidth));
//输入Tensor
input_tensor = new DenseTensor<float>(new[] { 1, 3, inpHeight, inpWidth });
for (int y = 0; y < image_rgb.Height; y++)
{
for (int x = 0; x < image_rgb.Width; x++)
{
input_tensor[0, 0, y, x] = image_rgb.At<Vec3b>(y, x)[0];
input_tensor[0, 1, y, x] = image_rgb.At<Vec3b>(y, x)[1];
input_tensor[0, 2, y, x] = image_rgb.At<Vec3b>(y, x)[2];
}
}
//将 input_tensor 放入一个输入参数的容器,并指定名称
input_container.Add(NamedOnnxValue.CreateFromTensor("inputimg", input_tensor));
dt1 = DateTime.Now;
//运行 Inference 并获取结果
result_infer = onnx_session.Run(input_container);
dt2 = DateTime.Now;
// 将输出结果转为DisposableNamedOnnxValue数组
results_onnxvalue = result_infer.ToArray();
float[] kpt = results_onnxvalue[0].AsTensor<float>().ToArray();
float[] pv = results_onnxvalue[1].AsTensor<float>().ToArray();
float[] temp = new float[51];
int num_proposal = 341;
int num_pts = 17;
int len = num_pts * 3;
List<List<int>> results = new List<List<int>>();
for (int i = 0; i < num_proposal; i++)
{
Array.Copy(kpt, i * 51, temp, 0, 51);
if (pv[i] >= confThreshold)
{
List<int> human_pts = new List<int>();
for (int ii = 0; ii < num_pts * 2; ii++)
{
human_pts.Add(0);
}
for (int j = 0; j < num_pts; j++)
{
float score = temp[j * 3] * 2;
if (score >= confThreshold)
{
float x = temp[j * 3 + 1] * image.Cols;
float y = temp[j * 3 + 2] * image.Rows;
human_pts[j * 2] = (int)x;
human_pts[j * 2 + 1] = (int)y;
}
}
results.Add(human_pts);
}
}
result_image = image.Clone();
int start_x = 0;
int start_y = 0;
int end_x = 0;
int end_y = 0;
for (int i = 0; i < results.Count; ++i)
{
for (int j = 0; j < num_pts; j++)
{
int cx = results[i][j * 2];
int cy = results[i][j * 2 + 1];
if (cx > 0 && cy > 0)
{
Cv2.Circle(result_image, new OpenCvSharp.Point(cx, cy), 3, new Scalar(0, 0, 255), -1, LineTypes.AntiAlias);
}
start_x = results[i][connect_list[j * 2] * 2];
start_y = results[i][connect_list[j * 2] * 2 + 1];
end_x = results[i][connect_list[j * 2 + 1] * 2];
end_y = results[i][connect_list[j * 2 + 1] * 2 + 1];
if (start_x > 0 && start_y > 0 && end_x > 0 && end_y > 0)
{
Cv2.Line(result_image, new OpenCvSharp.Point(start_x, start_y), new OpenCvSharp.Point(end_x, end_y), new Scalar(0, 255, 0), 2, LineTypes.AntiAlias);
}
}
start_x = results[i][connect_list[num_pts * 2] * 2];
start_y = results[i][connect_list[num_pts * 2] * 2 + 1];
end_x = results[i][connect_list[num_pts * 2 + 1] * 2];
end_y = results[i][connect_list[num_pts * 2 + 1] * 2 + 1];
if (start_x > 0 && start_y > 0 && end_x > 0 && end_y > 0)
{
Cv2.Line(result_image, new OpenCvSharp.Point(start_x, start_y), new OpenCvSharp.Point(end_x, end_y), new Scalar(0, 255, 0), 2, LineTypes.AntiAlias);
}
}
pictureBox2.Image = new Bitmap(result_image.ToMemoryStream());
textBox1.Text = "推理耗时:" + (dt2 - dt1).TotalMilliseconds + "ms";
button2.Enabled = true;
}
private void Form1_Load(object sender, EventArgs e)
{
startupPath = System.Windows.Forms.Application.StartupPath;
model_path = "model/e2epose_resnet50_1x3x512x512.onnx";
// 创建输出会话,用于输出模型读取信息
options = new SessionOptions();
options.LogSeverityLevel = OrtLoggingLevel.ORT_LOGGING_LEVEL_INFO;
options.AppendExecutionProvider_CPU(0);// 设置为CPU上运行
// 创建推理模型类,读取本地模型文件
onnx_session = new InferenceSession(model_path, options);//model_path 为onnx模型文件的路径
// 创建输入容器
input_container = new List<NamedOnnxValue>();
image_path = "test_img/1.jpg";
pictureBox1.Image = new Bitmap(image_path);
image = new Mat(image_path);
inpWidth = 512;
inpHeight = 512;
confThreshold = 0.5f;
}
private void pictureBox1_DoubleClick(object sender, EventArgs e)
{
Common.ShowNormalImg(pictureBox1.Image);
}
private void pictureBox2_DoubleClick(object sender, EventArgs e)
{
Common.ShowNormalImg(pictureBox2.Image);
}
SaveFileDialog sdf = new SaveFileDialog();
private void button3_Click(object sender, EventArgs e)
{
if (pictureBox2.Image == null)
{
return;
}
Bitmap output = new Bitmap(pictureBox2.Image);
sdf.Title = "保存";
sdf.Filter = "Images (*.jpg)|*.jpg|Images (*.png)|*.png|Images (*.bmp)|*.bmp";
if (sdf.ShowDialog() == DialogResult.OK)
{
switch (sdf.FilterIndex)
{
case 1:
{
output.Save(sdf.FileName, ImageFormat.Jpeg);
break;
}
case 2:
{
output.Save(sdf.FileName, ImageFormat.Png);
break;
}
case 3:
{
output.Save(sdf.FileName, ImageFormat.Bmp);
break;
}
}
MessageBox.Show("保存成功,位置:" + sdf.FileName);
}
}
}
}
using Microsoft.ML.OnnxRuntime;
using Microsoft.ML.OnnxRuntime.Tensors;
using OpenCvSharp;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Imaging;
using System.Linq;
using System.Windows.Forms;namespace Onnx_Demo
{public partial class Form1 : Form{public Form1(){InitializeComponent();}string fileFilter = "*.*|*.bmp;*.jpg;*.jpeg;*.tiff;*.tiff;*.png";string image_path = "";string startupPath;DateTime dt1 = DateTime.Now;DateTime dt2 = DateTime.Now;string model_path;Mat image;Mat result_image;SessionOptions options;InferenceSession onnx_session;Tensor<float> input_tensor;List<NamedOnnxValue> input_container;IDisposableReadOnlyCollection<DisposableNamedOnnxValue> result_infer;DisposableNamedOnnxValue[] results_onnxvalue;Tensor<float> result_tensors;int inpHeight, inpWidth;float confThreshold;int[] connect_list = { 0, 1, 0, 2, 1, 3, 2, 4, 3, 5, 4, 6, 5, 6, 5, 7, 7, 9, 6, 8, 8, 10, 5, 11, 6, 12, 11, 12, 11, 13, 13, 15, 12, 14, 14, 16 };private void button1_Click(object sender, EventArgs e){OpenFileDialog ofd = new OpenFileDialog();ofd.Filter = fileFilter;if (ofd.ShowDialog() != DialogResult.OK) return;pictureBox1.Image = null;image_path = ofd.FileName;pictureBox1.Image = new Bitmap(image_path);textBox1.Text = "";image = new Mat(image_path);pictureBox2.Image = null;}unsafe private void button2_Click(object sender, EventArgs e){if (image_path == ""){return;}button2.Enabled = false;pictureBox2.Image = null;textBox1.Text = "";Application.DoEvents();//读图片image = new Mat(image_path);//将图片转为RGB通道Mat image_rgb = new Mat();Cv2.CvtColor(image, image_rgb, ColorConversionCodes.BGR2RGB);Cv2.Resize(image_rgb, image_rgb, new OpenCvSharp.Size(inpHeight, inpWidth));//输入Tensorinput_tensor = new DenseTensor<float>(new[] { 1, 3, inpHeight, inpWidth });for (int y = 0; y < image_rgb.Height; y++){for (int x = 0; x < image_rgb.Width; x++){input_tensor[0, 0, y, x] = image_rgb.At<Vec3b>(y, x)[0];input_tensor[0, 1, y, x] = image_rgb.At<Vec3b>(y, x)[1];input_tensor[0, 2, y, x] = image_rgb.At<Vec3b>(y, x)[2];}}//将 input_tensor 放入一个输入参数的容器,并指定名称input_container.Add(NamedOnnxValue.CreateFromTensor("inputimg", input_tensor));dt1 = DateTime.Now;//运行 Inference 并获取结果result_infer = onnx_session.Run(input_container);dt2 = DateTime.Now;// 将输出结果转为DisposableNamedOnnxValue数组results_onnxvalue = result_infer.ToArray();float[] kpt = results_onnxvalue[0].AsTensor<float>().ToArray();float[] pv = results_onnxvalue[1].AsTensor<float>().ToArray();float[] temp = new float[51];int num_proposal = 341;int num_pts = 17;int len = num_pts * 3;List<List<int>> results = new List<List<int>>();for (int i = 0; i < num_proposal; i++){Array.Copy(kpt, i * 51, temp, 0, 51);if (pv[i] >= confThreshold){List<int> human_pts = new List<int>();for (int ii = 0; ii < num_pts * 2; ii++){human_pts.Add(0);}for (int j = 0; j < num_pts; j++){float score = temp[j * 3] * 2;if (score >= confThreshold){float x = temp[j * 3 + 1] * image.Cols;float y = temp[j * 3 + 2] * image.Rows;human_pts[j * 2] = (int)x;human_pts[j * 2 + 1] = (int)y;}}results.Add(human_pts);}}result_image = image.Clone();int start_x = 0;int start_y = 0;int end_x = 0;int end_y = 0;for (int i = 0; i < results.Count; ++i){for (int j = 0; j < num_pts; j++){int cx = results[i][j * 2];int cy = results[i][j * 2 + 1];if (cx > 0 && cy > 0){Cv2.Circle(result_image, new OpenCvSharp.Point(cx, cy), 3, new Scalar(0, 0, 255), -1, LineTypes.AntiAlias);}start_x = results[i][connect_list[j * 2] * 2];start_y = results[i][connect_list[j * 2] * 2 + 1];end_x = results[i][connect_list[j * 2 + 1] * 2];end_y = results[i][connect_list[j * 2 + 1] * 2 + 1];if (start_x > 0 && start_y > 0 && end_x > 0 && end_y > 0){Cv2.Line(result_image, new OpenCvSharp.Point(start_x, start_y), new OpenCvSharp.Point(end_x, end_y), new Scalar(0, 255, 0), 2, LineTypes.AntiAlias);}}start_x = results[i][connect_list[num_pts * 2] * 2];start_y = results[i][connect_list[num_pts * 2] * 2 + 1];end_x = results[i][connect_list[num_pts * 2 + 1] * 2];end_y = results[i][connect_list[num_pts * 2 + 1] * 2 + 1];if (start_x > 0 && start_y > 0 && end_x > 0 && end_y > 0){Cv2.Line(result_image, new OpenCvSharp.Point(start_x, start_y), new OpenCvSharp.Point(end_x, end_y), new Scalar(0, 255, 0), 2, LineTypes.AntiAlias);}}pictureBox2.Image = new Bitmap(result_image.ToMemoryStream());textBox1.Text = "推理耗时:" + (dt2 - dt1).TotalMilliseconds + "ms";button2.Enabled = true;}private void Form1_Load(object sender, EventArgs e){startupPath = System.Windows.Forms.Application.StartupPath;model_path = "model/e2epose_resnet50_1x3x512x512.onnx";// 创建输出会话,用于输出模型读取信息options = new SessionOptions();options.LogSeverityLevel = OrtLoggingLevel.ORT_LOGGING_LEVEL_INFO;options.AppendExecutionProvider_CPU(0);// 设置为CPU上运行// 创建推理模型类,读取本地模型文件onnx_session = new InferenceSession(model_path, options);//model_path 为onnx模型文件的路径// 创建输入容器input_container = new List<NamedOnnxValue>();image_path = "test_img/1.jpg";pictureBox1.Image = new Bitmap(image_path);image = new Mat(image_path);inpWidth = 512;inpHeight = 512;confThreshold = 0.5f;}private void pictureBox1_DoubleClick(object sender, EventArgs e){Common.ShowNormalImg(pictureBox1.Image);}private void pictureBox2_DoubleClick(object sender, EventArgs e){Common.ShowNormalImg(pictureBox2.Image);}SaveFileDialog sdf = new SaveFileDialog();private void button3_Click(object sender, EventArgs e){if (pictureBox2.Image == null){return;}Bitmap output = new Bitmap(pictureBox2.Image);sdf.Title = "保存";sdf.Filter = "Images (*.jpg)|*.jpg|Images (*.png)|*.png|Images (*.bmp)|*.bmp";if (sdf.ShowDialog() == DialogResult.OK){switch (sdf.FilterIndex){case 1:{output.Save(sdf.FileName, ImageFormat.Jpeg);break;}case 2:{output.Save(sdf.FileName, ImageFormat.Png);break;}case 3:{output.Save(sdf.FileName, ImageFormat.Bmp);break;}}MessageBox.Show("保存成功,位置:" + sdf.FileName);}}}
}
下载
源码下载
相关文章:
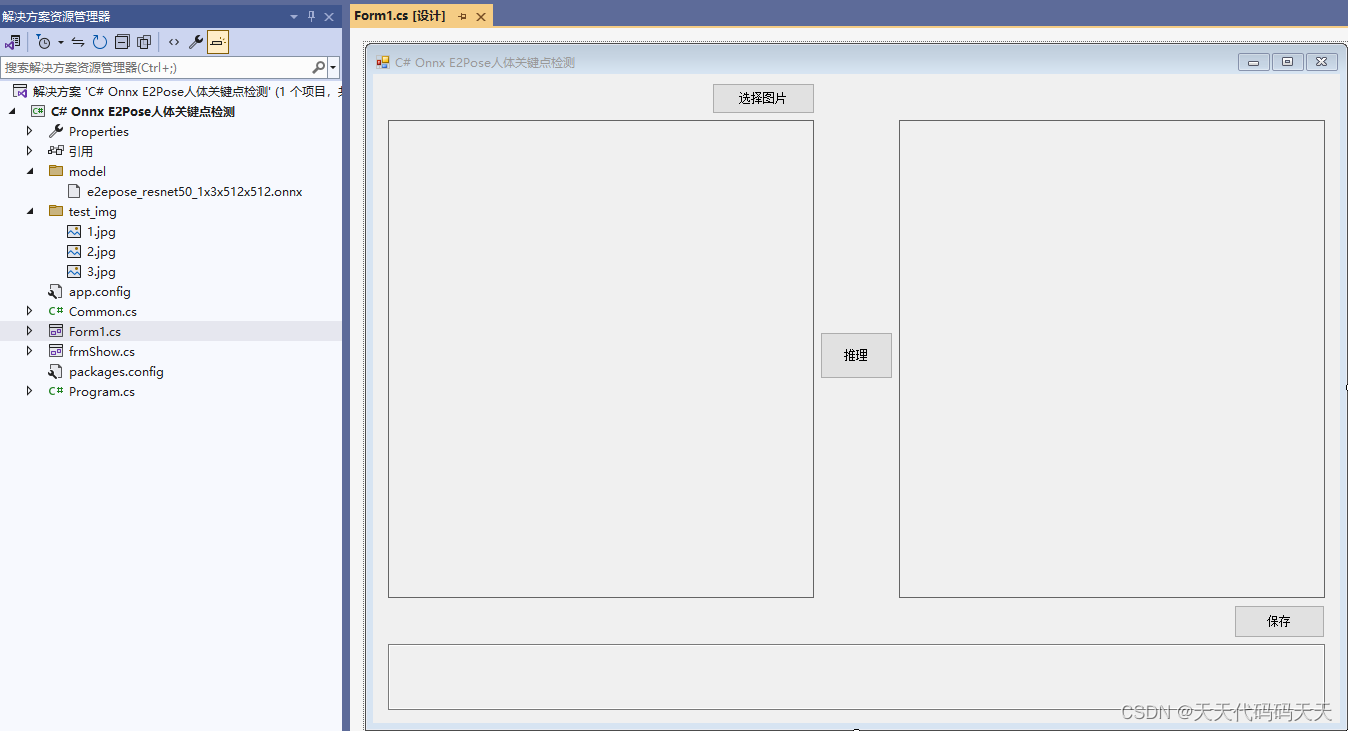
C# Onnx E2Pose人体关键点检测
C# Onnx E2Pose人体关键点检测 目录 效果 模型信息 项目 代码 下载 效果 模型信息 Inputs ------------------------- name:inputimg tensor:Float[1, 3, 512, 512] --------------------------------------------------------------- Outputs ---…...
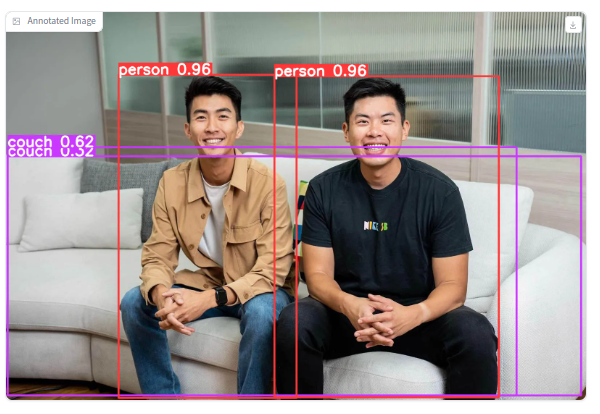
YOLO10:手把手安装教程与使用说明
目录 前言一、YOLO10检测模型二、YOLO安装过程1.新建conda的环境 yolo10安装依赖包测试 总结 前言 v9还没整明白,v10又来了。而且还是打败天下无敌手的存在,连最近很火的RT-DETR都被打败了。那么,笑傲目标检测之林的v10又能持续多久呢&#…...
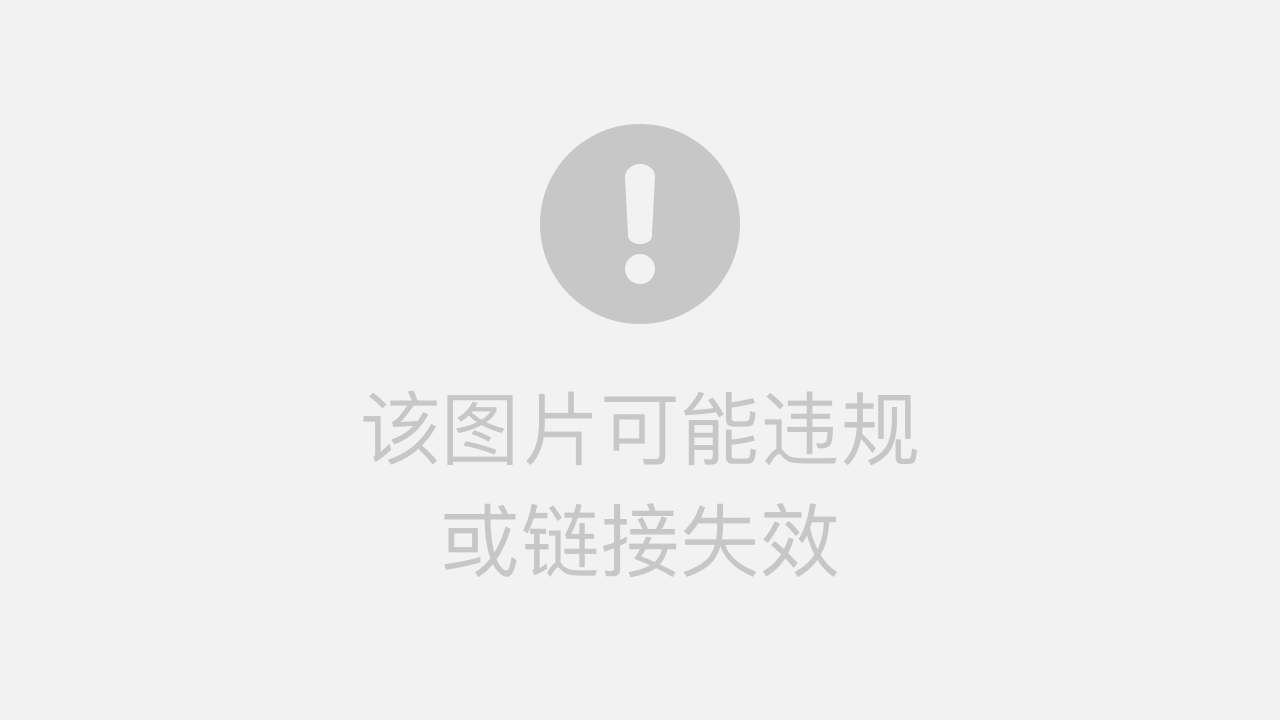
EasyRecovery2024永久免费crack激活码注册码
在数字化时代,数据已经成为我们生活和工作中不可或缺的一部分。无论是个人用户还是企业用户,都面临着数据丢失的风险。一旦数据丢失,可能会给我们的工作带来极大的不便,甚至可能对企业造成重大损失。因此,数据安全和恢…...

Linux Centos内网环境中安装mysql5.7详细安装过程
一、下载安装包 下载地址(可下载历史版本): https://downloads.mysql.com/archives/community 二、解压到安装路径 tar -zxvf mysql-5.7.20-linux-glibc2.12-x86_64.tar.gz三、重命名 mv /usr/local/mysql-5.7.20-linux-glibc2.12-x86_64 …...

新字符设备驱动实验学习
register_chrdev 和 unregister_chrdev 这两个函数是老版本驱动使用的函数,现在新的字符设备驱动已经不再使用这两个函数,而是使用Linux内核推荐的新字符设备驱动API函数。新字符设别驱动API函数在驱动模块加载的时候自动创建设备节点文件。 分配和释放…...

篇1:Mapbox Style Specification
目录 引言 地图创建与样式加载 Spec Reference Root sources type:vector矢量瓦片...
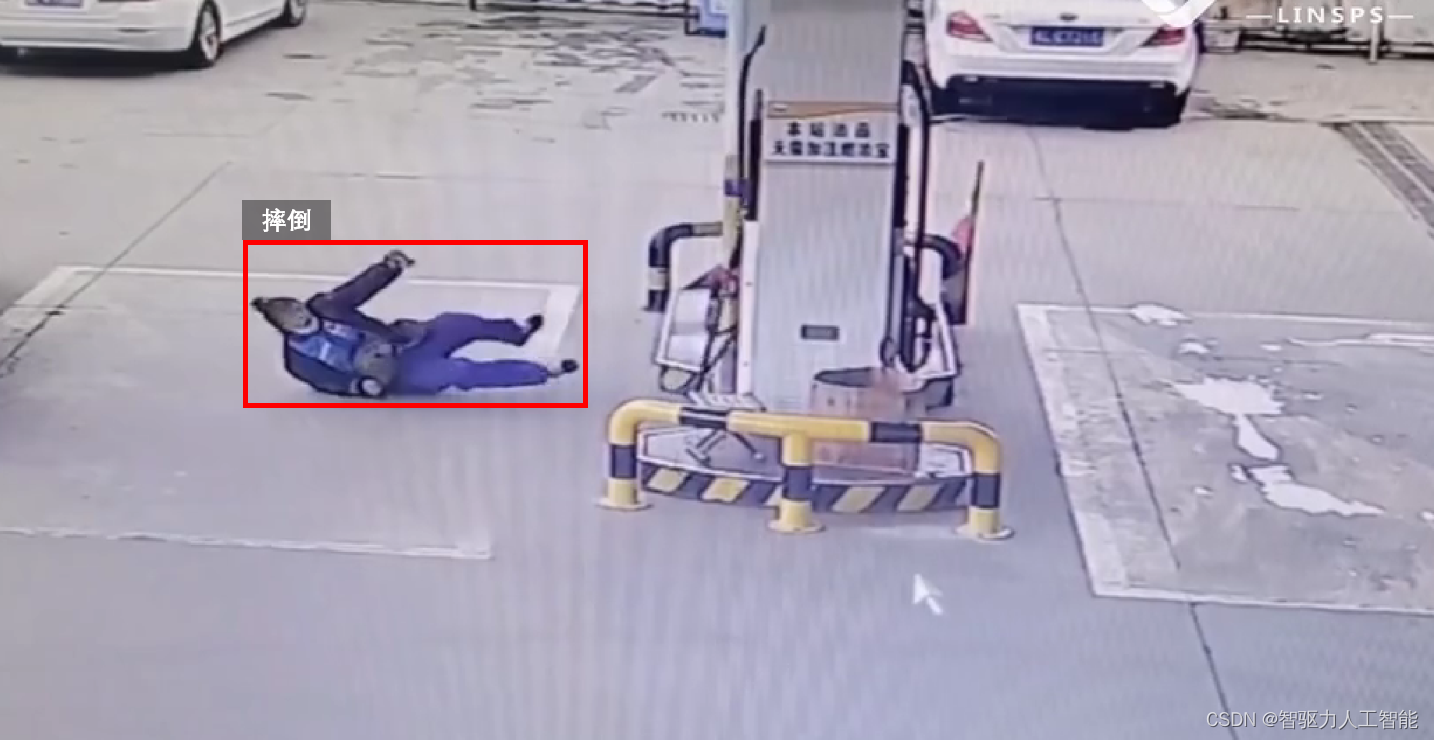
实时监控与报警:人员跌倒检测算法的实践
在全球范围内,跌倒事件对老年人和儿童的健康与安全构成了重大威胁。据统计,跌倒是老年人意外伤害和死亡的主要原因之一。开发人员跌倒检测算法的目的是通过技术手段及时发现和响应跌倒事件,减少因延迟救助而造成的严重后果。这不仅对老年人群…...

LeetCode25_K个一组翻转链表
. - 力扣(LeetCode) 一、题目描述 二、过程模拟 1. 第一步 2. 第二步:子链表分组 3. 第三步:断开前后两组 4. 第四步:翻转start到end的部分 5. 第五步:连接翻转好的前半部分和未翻转的后半部分ÿ…...
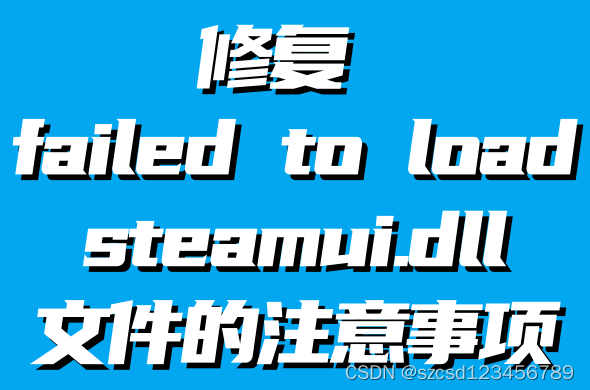
电脑突然提示:“failed to load steamui.dll”是什么情况?分享几种解决steamui.dll丢失的方法
相信有一些用户正在面临一个叫做“failed to load steamui.dll”的问题,这种情况多半发生在试图运行某个程序时,系统会提示一条错误消息:“failed to load steamui.dll”。那么,为何steamui.dll文件会丢失,又应该如何解…...
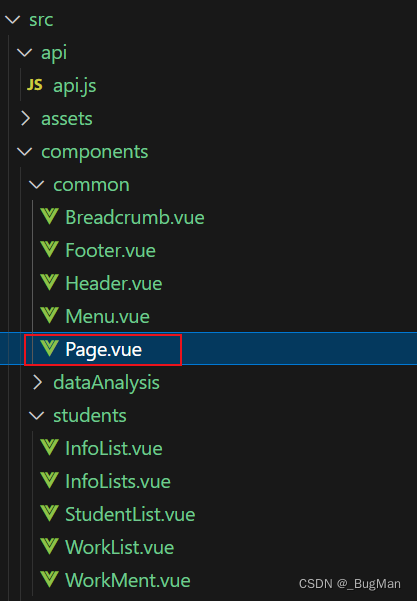
【vue实战项目】通用管理系统:作业列表
目录 目录 1.前言 2.后端API 3.前端API 4.组件 5.分页 6.封装组件 1.前言 本文是博主前端Vue实战系列中的一篇文章,本系列将会带大家一起从0开始一步步完整的做完一个小项目,让你找到Vue实战的技巧和感觉。 专栏地址: https://blog…...
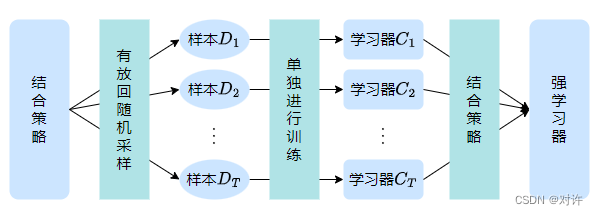
Scikit-Learn随机森林回归
Scikit-Learn随机森林回归 1、随机森林1.1、集成学习1.2、Bagging方法1.3、随机森林算法1.4、随机森林的优缺点2、Scikit-Learn随机森林回归2.1、Scikit-Learn随机森林回归API2.2、随机森林回归实践(加州房价预测)1、随机森林 随机森林是一种由决策树构成的集成算法,它在大多…...

Vue Router 教程
Vue Router 是 Vue.js 的官方路由管理器,它提供了一种方便的方式来管理应用的路由。在本教程中,我们将介绍 Vue Router 的一些常见用法和示例。 一、安装 Vue Router 使用 Vue Router 之前,需要先安装它。可以使用以下命令通过 npm 安装&am…...
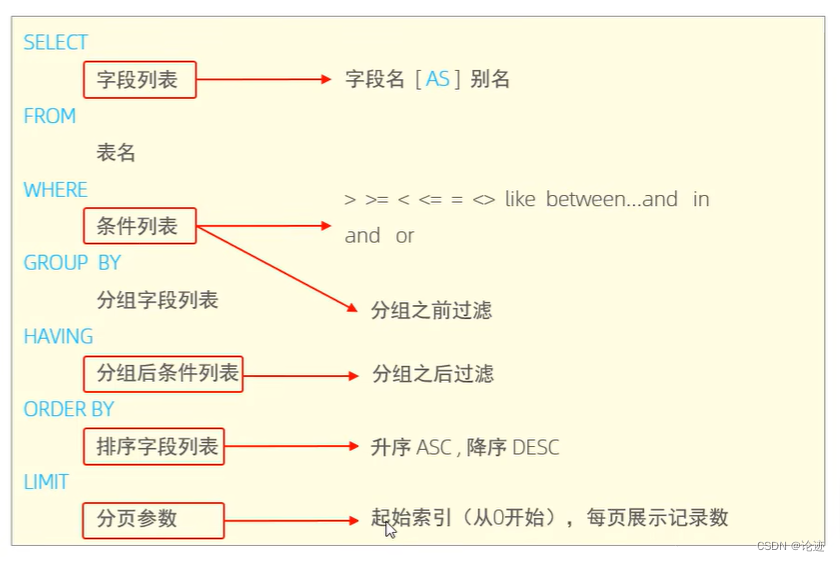
【数据库】SQL--DQL(初阶)
文章目录 DCL1. 基本介绍2. 语法2.1 基础查询2.2 条件查询2.3 聚合函数2.4 聚合查询2.5 分组查询2.6 排序查询2.7 分页查询2.8 综合案例练习2.9 执行顺序 3. DQL总结 DCL 更多数据库MySQL系统内容就在以下专栏: 专栏链接:数据库MySQL 1. 基本介绍 DQL英…...
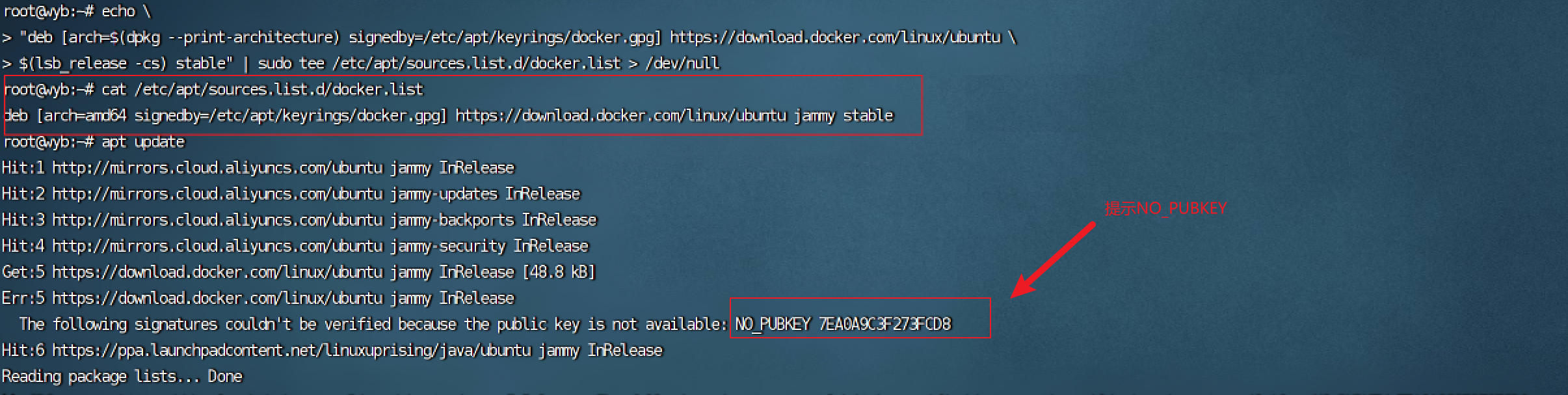
【docker】docker的安装
如果之前安装了旧版本的docker我们需要进行卸载: 卸载之前的旧版本 卸载 # 卸载旧版本 sudo apt-get remove docker docker-engine docker.io containerd runc # 卸载历史版本 apt-get purge docker-ce docker-ce-cli containerd.io docker-buildx-plugin docker…...
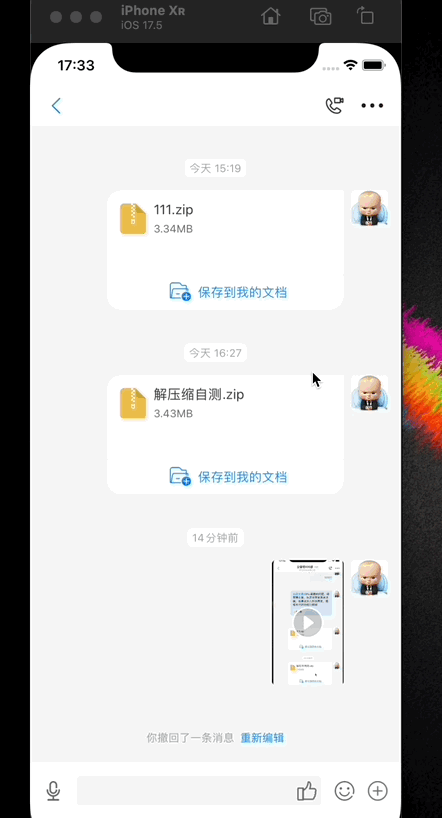
OC IOS 文件解压缩预览
热很。。热很。。。。夏天的城市只有热浪没有情怀。。。 来吧,come on。。。 引用第三方库: pod SSZipArchive 开发实现: 一、控制器实现 头文件控制器定义: // // ZipRarViewController.h // // Created by carbonzhao on 2…...

python-web应用程序-Django-From组件
python-web应用程序-Django-From组件 添加用户时 原始方法(本质)【麻烦】 def user_add(req):if req.method GET:return render(req,XXX.html)#POST请求处理:XXXXX-用户数据没有校验 -出现错误提示 -页面上的每一个字段都需要我们重新写一遍 -关联数…...
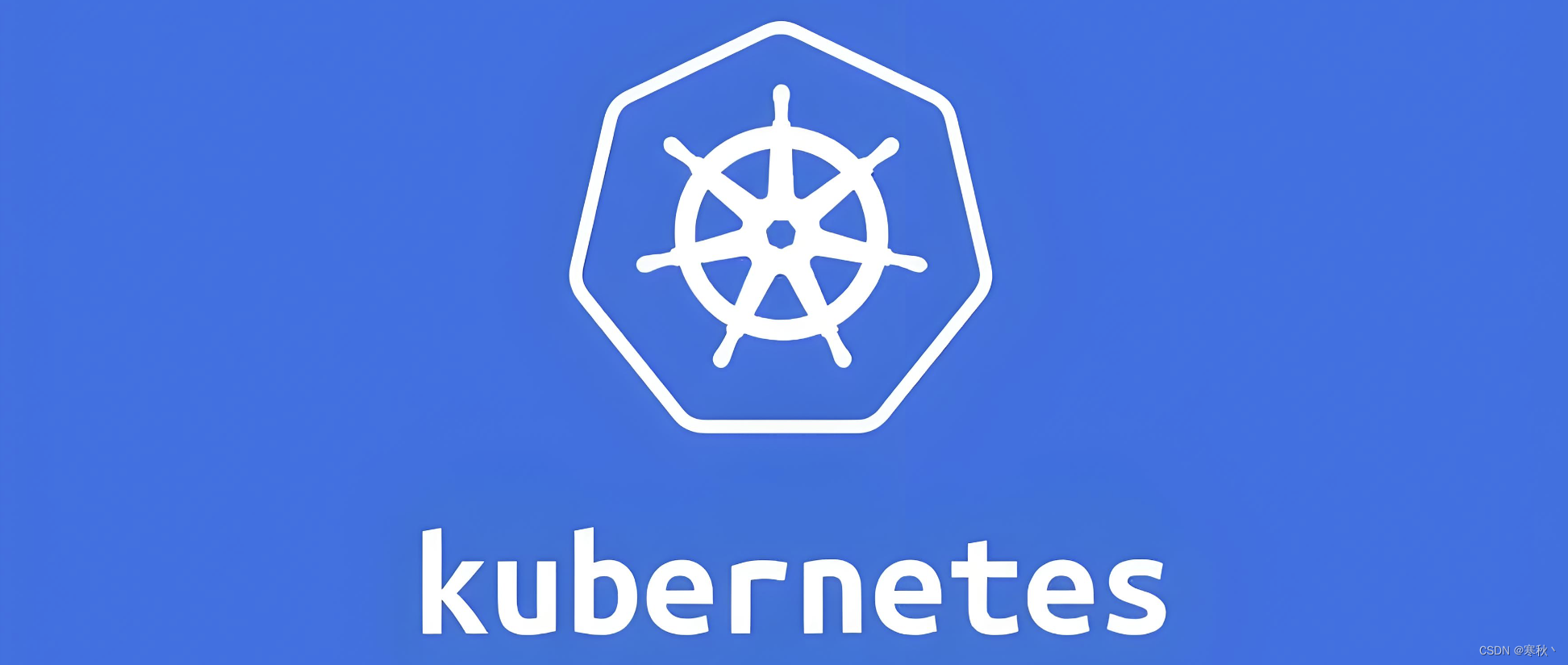
K8s(Kubernetes)常用命令
大家好,当谈及容器编排工具时,Kubernetes(常简称为K8s)无疑是当今最受欢迎和广泛使用的解决方案之一。作为一个开源的容器编排平台,Kubernetes 提供了丰富的功能,可以帮助开发人员和运维团队管理、部署和扩…...

C#-for循环语句
for循环语句 语法: for(初始化变量; 判断条件; 增量表达式) { // 内部代码 } 第一个空(初始表达式): 一般用来声明一个临时的局部变量 用来计数第二个空(条件表达式): 表明进入循环的条件 一个bool类型的值(bool类型 条件表达式 逻辑运算符)第三个空(增量表达式): 使用第一个空…...
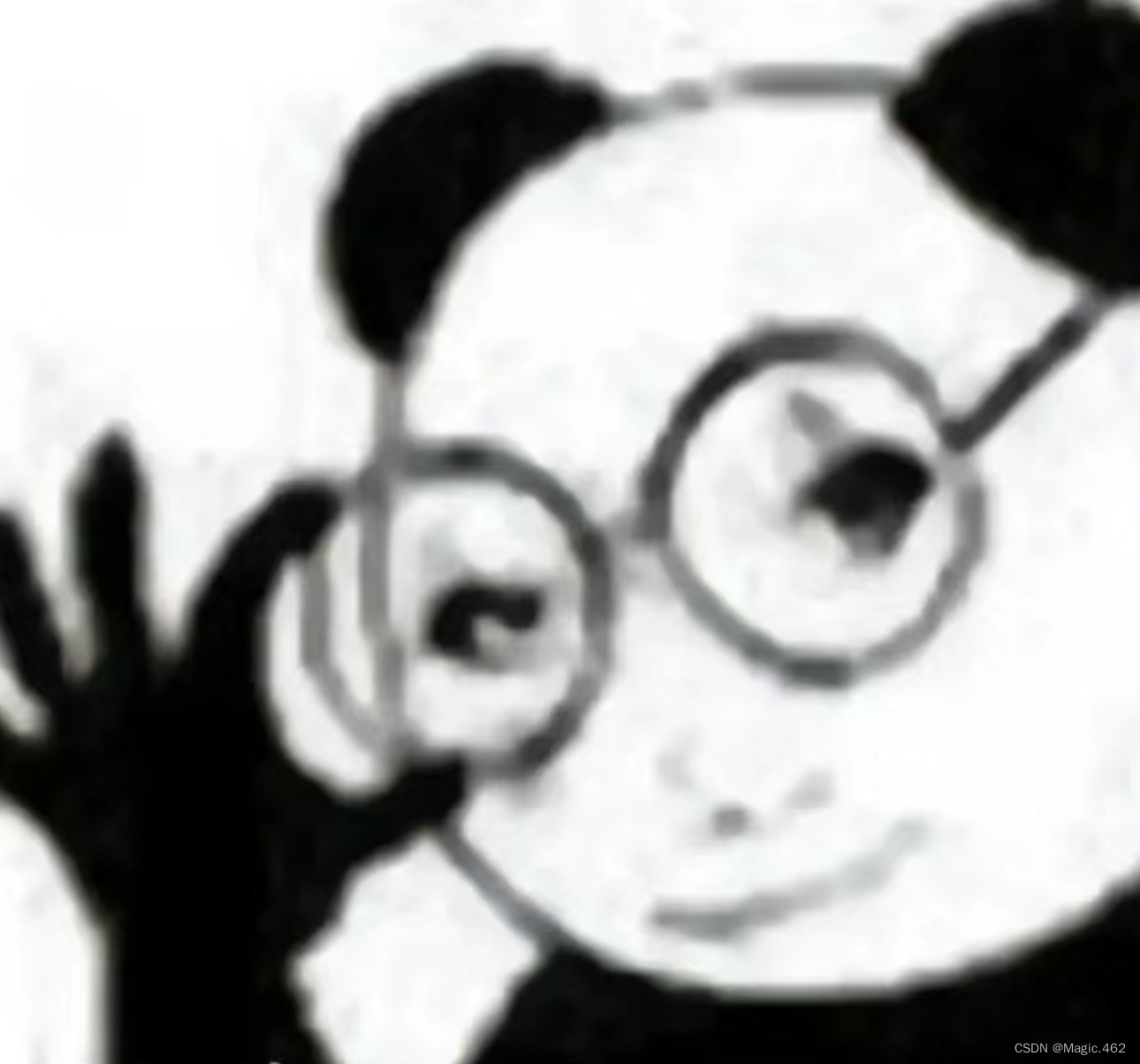
css动画案例练习之会展开的魔方和交错的小块
这里写目录标题 一级目录二级目录三级目录 下面开始案例的练习,建议第一个动手操作好了再进行下一个一、交错的小块效果展示1.大致思路1.基本结构2.实现动态移动 2.最终版代码 二、会展开的魔方1.大致思路1.基本结构;2.静态魔方的构建3.让静态的魔方动起来 2.最终版…...
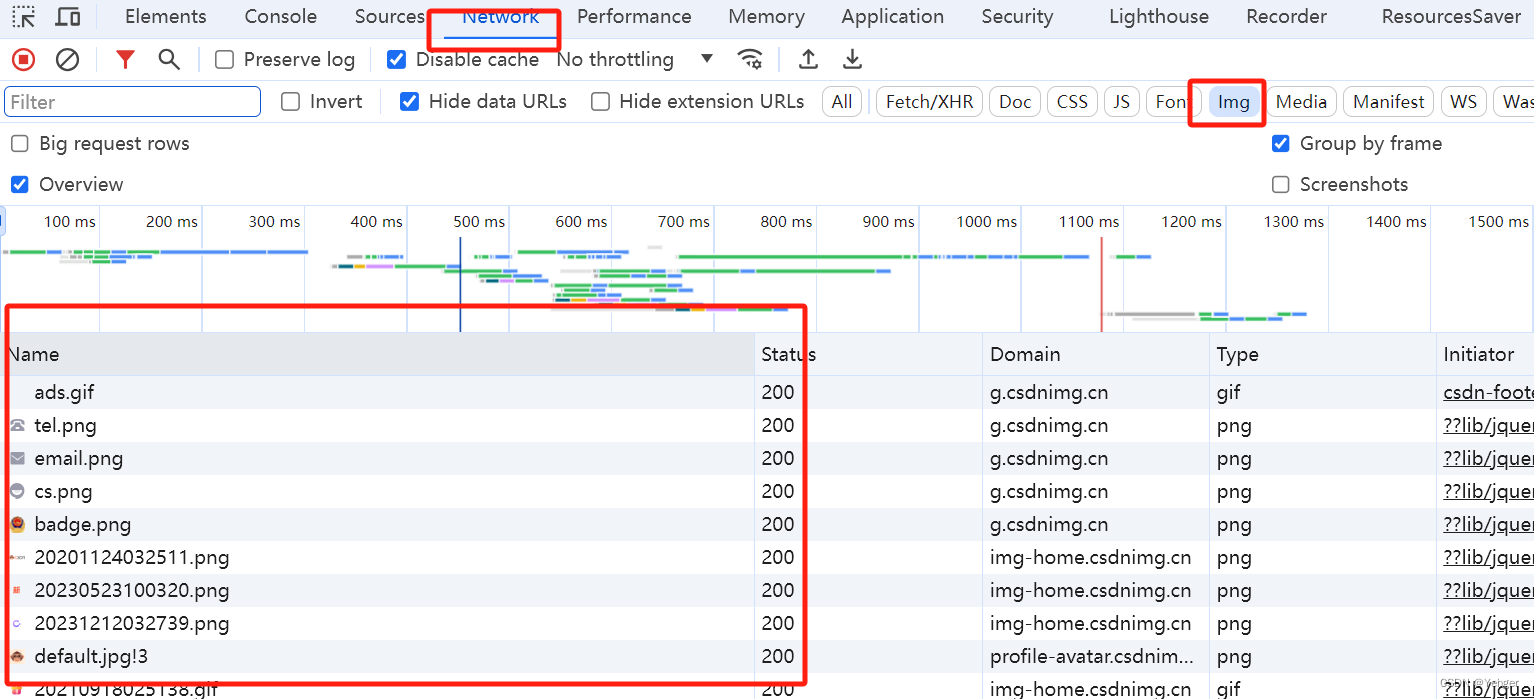
前端逆向之下载canvas引用的图片
前端逆向之下载canvas引用的图片 一、来源二、解决三、如果在Network这里也找不到呢? 一、来源 当我们用dom检查器的时候无法选中想要扒下来的图片,只能选中canvas,这种时候该怎么办呢? 二、解决 这个时候应该换个脑子…...

深度学习手撕代码题
目录: PyTorch实现注意力机制、多头注意力与自注意力Numpy广播机制实现矩阵间L2距离的计算Conv2D卷积的Python和C++实现Numpy实现bbox_iou的计算Numpy实现FocallossPython实现nms、softnmsPython实现BN批量归一化PyTorch卷积与BatchNorm的融合分割网络损失函数Dice Loss代码实…...
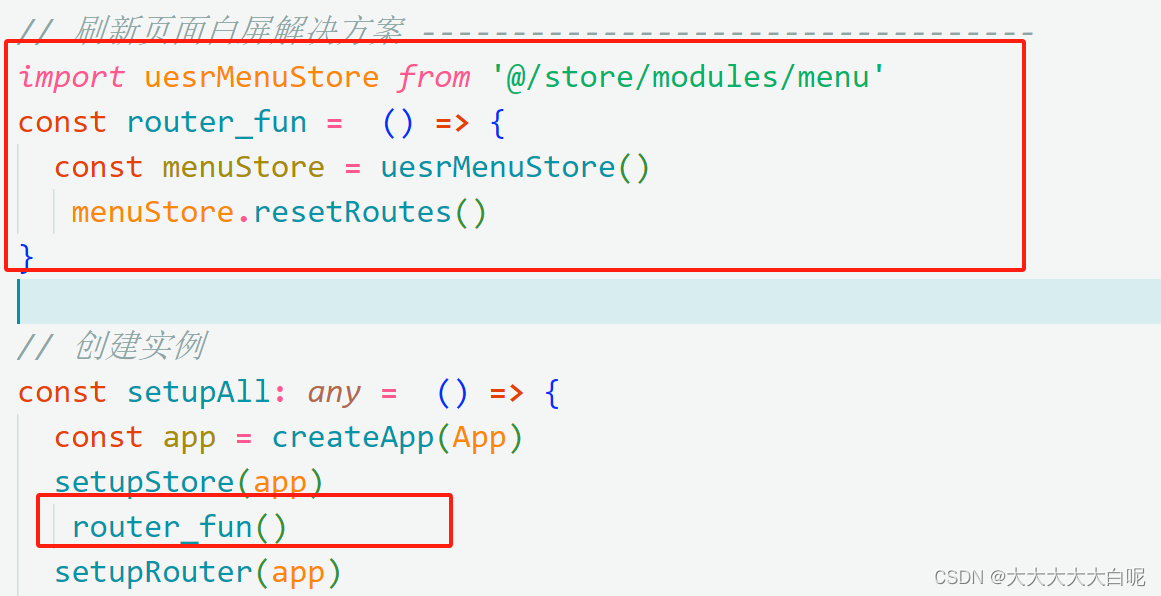
vue3 + ts 动态添加路由,刷新页面白屏问题解决方案
1、store 中添加路由的方法 2、main.ts中使用该方法 然后就可以任意刷新页面了,有问题可以随时滴我...........

【Kubernetes】k8s的调度约束(亲和与反亲和)
一、调度约束 list-watch 组件 Kubernetes 是通过 List-Watch 的机制进行每个组件的协作,保持数据同步的,每个组件之间的设计实现了解耦。 用户是通过 kubectl 根据配置文件,向 APIServer 发送命令,在 Node 节点上面建立 Pod 和…...
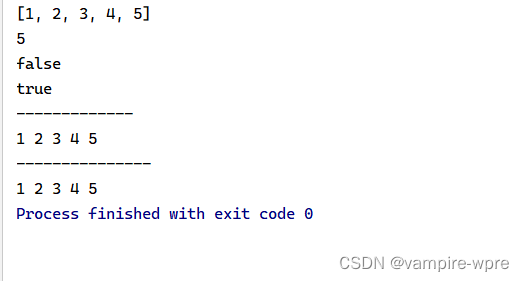
Java数据结构- Map和Set
目录 1. Map和Set2. Map的使用3. Set的使用 1. Map和Set Java中,Map和Set是两个接口,TreeSet、HashSet这两个类实现了Set接口,TreeMap、HashMap这两个类实现了Map接口。 带Tree的这两个类(TreeSet、TreeMap)底层的数…...
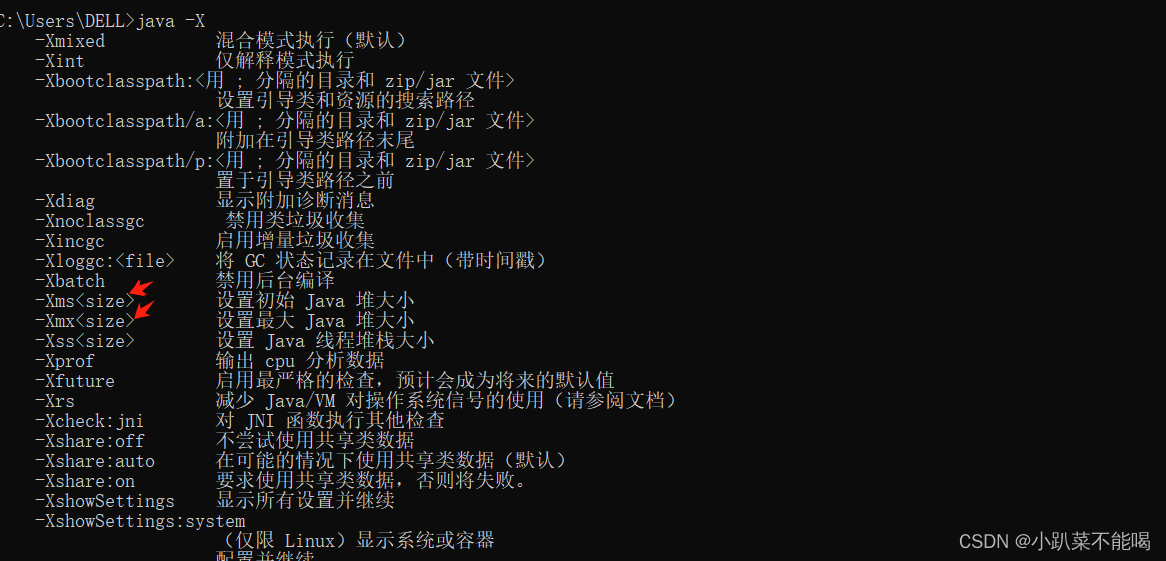
JVM参数配置
JVM参数的三种表示方法 在jvm中,jvm虚拟机参数有以下三种表示方法: 标准参数(-)所有的JVM实现都必须实现这些参数的功能,而且向后兼容非标准参数(-X),默认jvm实现这些参数的功能&…...

Vue 实现的精彩动画效果
在 Vue 开发中,我们可以利用<transition>组件来打造各种令人惊艳的动画效果。下面来详细看看这些有趣的动画效果及其实现代码。 一、缩放类效果 zoom-in(整体放大进入) <template><div><button click"isShow ! …...
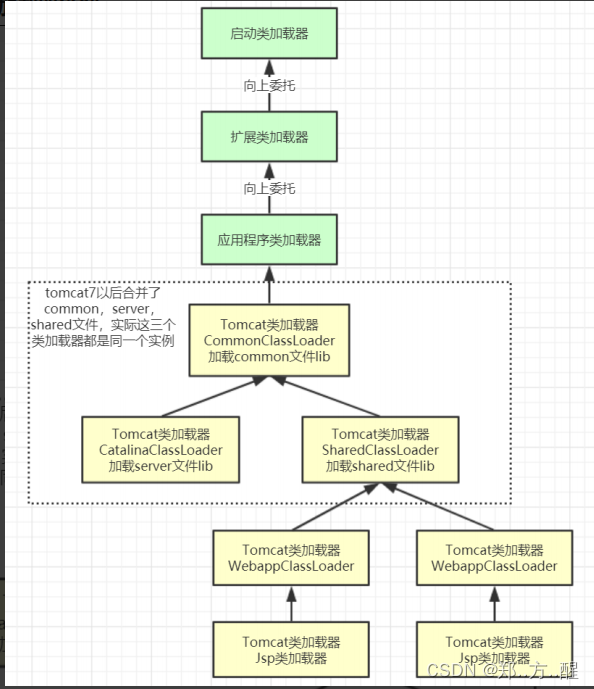
JVM类加载机制详解(JDK源码级别)
提示:从JDK源码级别彻底剖析JVM类加载机制、双亲委派机制、全盘负责委托机制、打破双亲委派机制的程序、Tomcat打破双亲委派机制、tomcat自定义类加载器详解、tomcat的几个主要类加载器、手写tomcat类加载器 文章目录 前言一、loadClass的类加载大概有如下步骤二、j…...
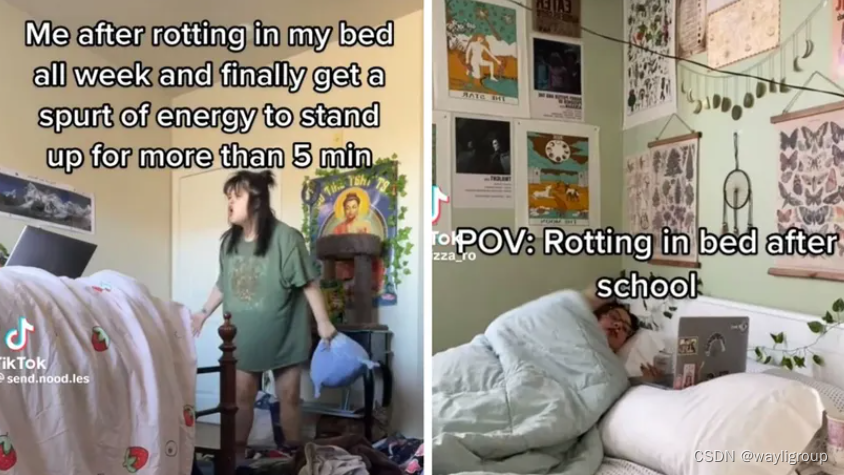
美国年轻人热衷床上“摆烂”,沃尔玛发掘床上用品新商机!
美国年轻人近年来热衷于床上“摆烂”生活方式,这反映了他们对舒适放松的追求和现代生活的压力。沃尔玛作为零售业巨头,敏锐地捕捉到这一市场变化,发现了床上用品的新商机。 美国年轻人忙碌中渴望宁静空间。床成为他们放松、逃离现实压力的理想…...

3168. 候诊室中的最少椅子数
给你一个字符串 s,模拟每秒钟的事件 i: 如果 s[i] E,表示有一位顾客进入候诊室并占用一把椅子。如果 s[i] L,表示有一位顾客离开候诊室,从而释放一把椅子。 返回保证每位进入候诊室的顾客都能有椅子坐的 最少 椅子…...
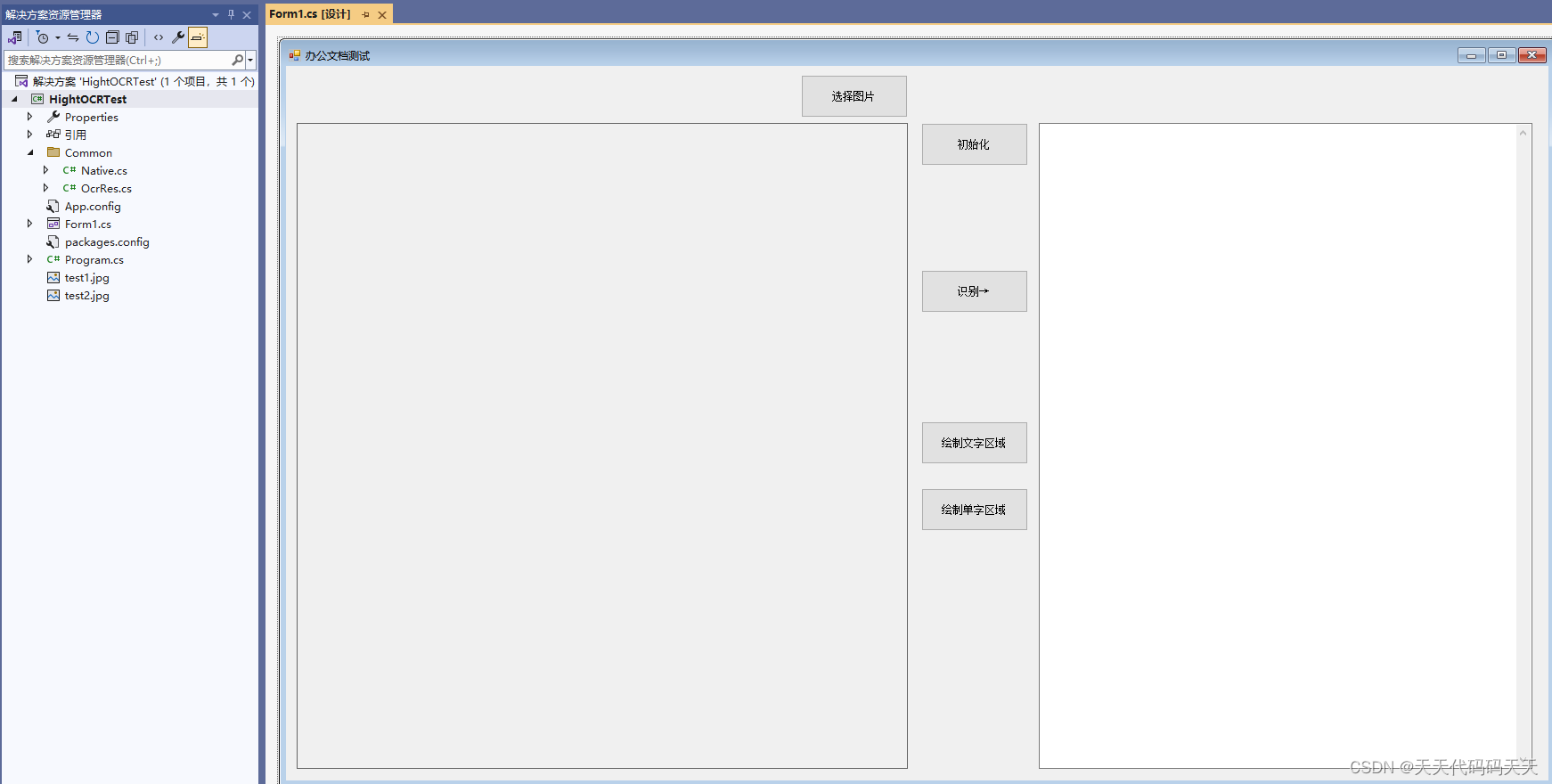
C# PaddleOCR 单字识别效果
C# PaddleOCR 单字识别效果 效果 说明 根据《百度办公文档识别C离线SDKV1.2用户接入文档.pdf》,使用C封装DLL,C#调用。 背景 为使客户、第三方开发者等能够更快速、方便的接入使用百度办公文档识别 SDK、促进百度 OCR产品赋能更多客户,特设…...
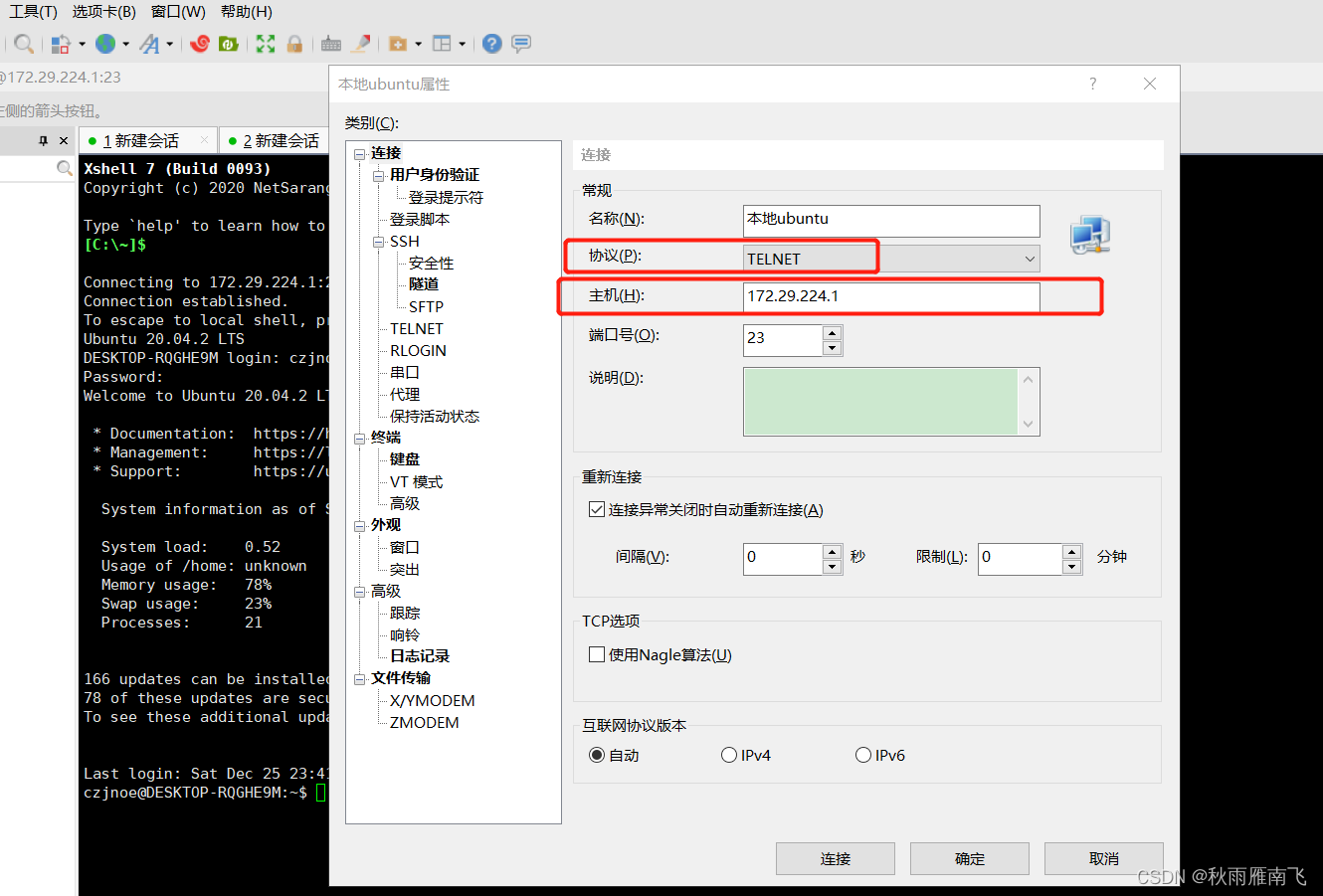
厦门网站建设价格/网站建设公司大型
一、安装telnet客户端 sudo apt-get install telnet 二、安装telnet服务端 sudo apt-get install telnetd 三、安装网络守护进程 sudo apt-get install openbsd-inetd 四、安装xinetd sudo apt-get install xinetd 五、重启telnet服务 sudo /etc/init.d/…...

桂林做旅游网站失败的网站/免费下载百度到桌面
/*************************************************************************** I.MX6 Android i2c-tools porting* 说明:* 判断I2C总线设备是否存在,每次都去查dmesg,实在是烦了,于是还是移植* 一个i2c-…...

dede做电影网站/合肥网站快速排名提升
在为程序加背景时,发现在拖动List或Grid列表时一片漆黑,很是难看android提供了一种方法,在用户拖动列表时不总是一片漆黑或者说透明。其实只是View的属性而已,ListView、GridView都有这个属性,两种设置如 下࿱…...

做电影网站如何规避版权/方象科技服务案例
先膜拜一波神仙yww 给定一个矩阵(没有任何特殊性质),如何求它的特征多项式? 算法一 直接把\(\lambda\)代入\((n1)\)个点值,求完行列式之后插值即可。 时间复杂度\(O(n^4)\) 算法二 下面介绍一个更快的做法。定义 对于矩阵\(\bm A,\bm B\), 若…...

开奖网站怎么做/有利于seo优化的是
自从2011年KlayGE在代码库中包含所有第三方库以来,Python一直都是基于3.2的版本,这几年来Python本身的发展都没跟上。最近打算把Python移植到WinRT平台,所以干脆先一鼓作气,更新到最新的3.4.1上。升级3.4.1把代码更新到3.4.1&…...

wordpress千万级访问/石家庄最新疫情
1、在windows下安装3-windows-installer.exe软件,默认安装。手动指定安装位置。比如:d:/crm2、访问一下看看是否好用。3、进入d:/crm,拷贝D:\crm\htdocs文件夹,到linux服务器下的网站默认文件夹下:/usr/local/apache2/htdocs,改名…...