C/C++ - 编码规范(USNA版)
[IC210] Resources/C++ Programming Guide and Tips
所有提交的评分作业(作业、项目、实验、考试)都必须使用本风格指南。本指南的目的不是限制你的编程,而是为你的程序建立统一的风格格式。
* 这将有助于你调试和维护程序。
* 有助于他人(包括指导教师)理解您的程序。
This style guide is mandatory for all submitted work for grading (homework, projects, labs, exams). The purpose of the guide is not to restrict your programming but rather to establish a consistent style format for your programs.
* This will help you debug and maintain your programs.
* It will help others (including your instructor) to understand them.
Whitespace and Visual Layout
"空白 "指换行符、空格字符和制表符。在源代码中,我们一般不使用制表符。事实上,emacs 会用适当数量的空格字符替换制表符。
"Whitespace" refers to newlines, space characters and tabs. In source code we do not generally use tabs. In fact, emacs replaces tab characters with an appropriate number of space characters.
在很多情况下,为了让编译器正确编译源代码,我们并不需要空格,尽管编译出来的结果我们无法阅读。我们主要通过三种方式使用空格来格式化代码,以达到最清晰的效果:
In many circumstances whitespace is not required in order for compilers to correctly compile source code, even though the result is unreadable to us. There are three major ways we use whitespace to format code for maximum clarity:
* 缩进: 每一级块嵌套必须缩进一致的空格数(通常为两空格)。
* 空行: 在源代码中,使用空行来分隔完成不同任务的代码块。虽然在某种程度上这是一种品味和艺术,但你应该期望用空行来分隔不同的函数,而一个长的函数体通常也应该用空行来分割成若干块。
* 用空格分隔代码元素: 我们建议在运算符和操作数之间使用空格,除非通过省略空格来突出优先级。
* Indentation: Each level of block nesting must be indented by a consistent number of spaces (usually two spaces).
* Blank Lines: In source code, use blank lines to separate chunks of code that accomplish distinct tasks. While to some extent this is taste and art, you should expect distinct functions to be separated by blank lines, and a long function body should usually be broken into chunks by blank lines as well.
* Whitespace separating elements of code: We recommend using whitespace between operators and operands, except where precedence is highlighted by leaving out spaces.
Comments
注释的缩进应与其描述的代码块一致。注释可分为战略和战术两大类。
-
应在代码开头给出文件名和作者。
-
策略性注释用于向读者概述正在发生的事情。这些注释出现在文件开头、重要函数之前和重要代码块之上。策略性注释通常以句子的形式编写,重点是解释代码块设计的全貌。
-
战术性注释旨在解释代码中棘手的地方、参数的作用以及有关程序控制流程的提示。这些注释应在需要时混合在代码中。这些注释应比策略性注释短,采用项目符号格式,并可采用内联格式。例如,应在给变量分配固定的、非显而易见的值的语句旁边加上战术性注释。
下面的示例展示了良好的注释。注意战术性注释,说明为什么使用 "9.0 "而不是 "9"。
Comments should be indented consistently with the block of code they describe. Comments can be divided into two general categories, strategic and tactical.
* Filename and Author should be given in the beginning of your code.
* Strategic comments are used to give the reader a general overview of what is going on. These comments appear at the beginning of files, before important functions, and above important blocks of code. Strategic comments tend to be written in sentences with an emphasis on explaining the big picture of what the block of code is designed to do.
* Tactical comments are designed to explain tricky areas of code, what parameters do, and hints about the control flow of the program. These comments should be intermixed in the code when needed. They should be shorter than strategic comments, in bullet format, and may be in inline format. One should, for instance, place a tactical comment next to the statement that assigns a fixed, non-obvious value to a variable.
The following example shows good commenting. Note the tactical comment about why "9.0" is used instead of "9".
Naming
* 变量应有有意义的名称。
例如,在一个程序中使用一个变量来跟踪足球比赛中的每次带球码数。为了程序的可读性,这样的变量应该叫做 yardsPerCarry,而不是仅仅 x。这必须与使用过长的名称相平衡。(15 个字符左右已接近 "过长 "限制)。
* Variables should have meaningful names.
For example, consider a program that uses a variable to track the yards per carry of a football game. Such a variable should be called yardsPerCarry rather than just x for program readability. This must be balanced against using names which are too long. (Fifteen or so characters approaches the "too long" limit.)
Curly Braces, i.e. { }'s
在 C++ 中,大括号(即 { })用于从一个或多个语句中创建一个 "块"。它用于划分函数体、循环和switch语句、结构体定义以及 if 语句中的 then 和 else 块。
-
一般情况下,要么始终将开头的大括号放在新的一行(本课程的首选),要么始终将其放在上一行的最后。一致性再次成为关键。
-
如果将开头的大括号放在新行,本课程的首选是保留上一行的缩进,即不缩进大括号。一般来说,除了极少数情况下将整个代码块放在一行外,任何一种大括号都会占用自己的行。例外情况是,注释可以跟在开大括号或闭大括号后面。
-
新建代码块内的代码块应缩进。
Curly Braces, i.e. { }'s in C++ are used to create a "block" from one or more statements. The delineate the bodies of functions, loops and switch statements, struct definitions, and the then and else blocks of if statments.
* Generally, one should either always put the opening curly brace on a new line (preferred for this course) or always but it last on the previous line. Once again consistency is key.
* If the opening brace goes on a new line, the preference for this course is to keep the previous line's indentation — i.e. do not indent the curly brace. Generally, curly braces of either kind will occupy their own line, except for rare occasions when an entire block is put on one line. The exception is that comments may follow either opening or closing braces.
* The chunk of code inside the newly created block should be indented.
Other Tips on Naming
-
不应使用单字母变量或常量。
但在通常情况下,用单个字母来标识某些变量或常量是例外情况。坐标系(x、y 和 z)就是一个例子。第二个例外情况出现在循环计数器变量的使用上,通常的做法是在 for 循环中使用 i 和 j 这样的变量作为计数器。
-
函数名和变量名应以小写字母开头。
由多个名称组成的标识符应将每个名称的第一个字母(在第一个字母之后)改为大写(如 yardsPerCarry)或使用下划线(yards_per_carry)。强烈建议使用前一种方法。
-
常量的命名应全部使用大写字母。
例如
const int PI = 3.14;
一般来说,除非是正常单词的一部分,否则最好避免在名称中使用小写字母 "L "或字母 "O"。例如,"cell" 是 c- e - 1- ONE 还是 c- e- 1-1?
-
避免使用大小写不同、外观相似或仅有细微差别的名称。
例如,如果在同一个程序中使用 InputData、InData 和 DataInput,肯定会引起混淆。
-
函数名称应反映函数的作用(printArray)或返回值(getAge)。
-
布尔变量名应该听起来像 "是/否"类的意思,例如 "isEmpty "和 "isFinished"。
* Single letter variables or constants should not be used.
An exception to this rule is when it is common practice to identify something with a single letter. An example of this is the coordinate system (x, y, and z). A second exception occurs in the use of loop counter variables where it is common practice to use variables like i and j as counters in for loops.
* Function names and variable names should begin with a lower case letter.
An identifier consisting of multiple names SHALL have each name distinguished by making the first letter of each name part (after the first) upper case (e.g. yardsPerCarry) or by using underscores (yards_per_carry). The former method is strongly encouraged.
* Constants should be named in all upper case letters.
Example:
const int PI = 3.14;
It's generally good to avoid lower case letter 'L', or the letter 'O' in names unless they are part of normal words. This is to avoid confusion with the numbers 1 and 0. For example, is "cell" c- e - 1- ONE or c- e- 1-1?
* Avoid names that differ only in case, look similar, or differ only slightly.
For example, InputData, InData and DataInput will certainly be confusing if used in the same program.
* Names of functions should reflect what they do (printArray), or what they return (getAge).
* Boolean variable names should sound like Yes/No things — "isEmpty" and "isFinished" are possible examples.
Miscellaneous but Important Stuff
-
任何一行代码都不得超出右边距。如果一行代码过长,应将其分割成若干段。请记住,编译器在很大程度上会忽略程序中多余的空白(但在处理字符串时要小心)。
-
始终使用最合适的运算符或结构来完成你希望它完成的工作。良好的设计和清晰度比优化更重要。不要声明或使用超过需要的变量。
-
数字常量("神奇数字")不得直接编码。唯一允许的例外是 0、1 或 -1,以及那些不可能发生变化的常数;例如,确定一个数字是否为偶数的代码可以使用数字 2,因为它不可能发生变化。如果从名称中看不出数值,数字常量必须有注释来解释其值。
* No line of code should wrap beyond the right margin. If a line of code becomes too long, break it up into pieces. Remember the compiler largely ignores extra whitespace in your programs (but be careful with strings).
* Always use the most appropriate operator or construct for the work you want it to do. Good design and clarity take precedence over optimization. Do not declare or use more variables than are necessary.
* Numerical constants ("magic numbers") must not be coded directly. The only allowable exceptions are for 0, 1 or -1, and those which are not likely to change; for example code determining if a number is even can use the number 2 since it is not likely to change. Numerical constants must have a comment explaining the value if it is not evident from the name.
Practical tips for tackling programming problems
以下是一些实用技巧,可以提高你的编程技巧。
-
程序员越独立越好。当然,在编写程序时,你需要查看讲义、示例程序和其他资料。不过,要在编写代码之前进行,但尽可能避免在编写代码时看这些资料。特别是,如果你真的是编程新手,至少在前三周,尽量从头开始写代码,不要做任何复制粘贴的工作。我知道这是不可能做到的,但酌情尝试。
这种方法有一些好处:
-
你会更清楚自己遗漏了哪些概念(或不太重要的细节)。这样,你就能更容易地发现自己的误解并加以纠正。
-
你会更快地写出代码。在你的大脑中访问某些东西要比在任何其他存储器中访问快得多。记忆层次很重要。
-
你的大脑似乎会进行创造性的综合。如果在大脑中存储更多内容,你可能会获得更多关于编程的基本观点。然而,大脑之外的东西对你来说始终是不变的。
-
-
细节决定成败。不要忘记,作为程序员,你要对程序中的每个字符负责。乍一看,事情可能很简单,但通常要花费比预期更多的时间和精力才能完成。
再举个例子,在线帮助手册有时会解释技术细节。在这种情况下,阅读一个段落可能需要 5 分钟或更长时间才能在大脑中理清所有细节。不要跳过细节。细节决定成败。
-
退一步,再思考。当你完成一项任务时,花一点时间退后一步。检查你是否同时掌握了大的逻辑和实现逻辑的小细节。看看是否有一些不一致的地方,如果有,就加以修正。
-
一辆无法行驶的汽车有多大价值?没用,我不会买这辆车。同样,无论你的源代码有多少行,如果它不能运行,它就是无用的,我也不会买你的程序。试着做一个好的、能用的产品。如果你的代码不能工作,你就缺少了一些东西;是什么呢?
Here are a few practical tips that will improve your programming skills.
* The more independent a programmer is, the better. Of course, you need to look at lecture notes, sample programs, and other materials to write your program. However, do this before writing the code, but try to avoid looking at them if possible while writing your code. In particular, if you are really new to programming, try to write your code from scratch without doing any copy-and-paste, at least for the first three weeks. I know it's impossible to do so; try with your discretion.
There are some benefits to this approach:
* You will become more conscious which concepts (or less important details) you miss. This way, you can identify your misunderstandings and fix them with more ease.
* You will write a code faster. Access to something in your brain is much faster than in any other storage. Memory hierarchy matters.
* Your brain seems to do creative synthesis. You may gain more fundamental perspectives on programming if you put more in your brain. However, things outside your brain always stay the same to you.
* The devil is in the details. Don't forget that is it you as a programmer who are responsible for all the bits and pieces of your program. Things may seem simple at a first look, but usually they will take more time and effort to complete than expected.
As one more example, the online notes sometimes explain technical details. In this case, reading one paragraph may take even 5 minutes or more to work out all the details in your brain. Don't skip through the details. The devil is in the details.
* Step back, and think again. When you finish a task, take a brief moment and step back. Check if you get both the big picture logic and the small details to implement the logic. See if there are some inconsistencies and fix them if any.
* How valuable is a car that doesn't run? It's useless, and I won't buy the car. Likewise, however many lines your source code may have, if it doesn't work, it's useless, and I won't buy your program. Try to make a good, working product. If your code doesn't work, you are missing something; what is it?
参考:
https://www.usna.edu/Users/cs/choi/ic210/resources/style.html
相关文章:
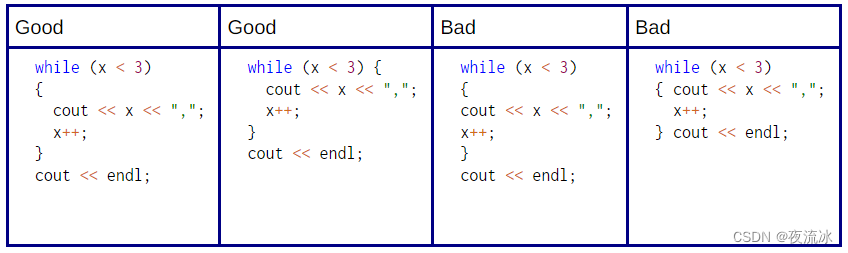
C/C++ - 编码规范(USNA版)
[IC210] Resources/C Programming Guide and Tips 所有提交的评分作业(作业、项目、实验、考试)都必须使用本风格指南。本指南的目的不是限制你的编程,而是为你的程序建立统一的风格格式。 * 这将有助于你调试和维护程序。 * 有助于他人&am…...

leetcode刷MySQL记录——sum/count里加条件判断、avg求满足条件记录数占比
leetcode题目:1934. 确认率 在刷leetcode的MySQL题中,从题目的题解知道了count和avg聚合函数的另外用法,在此记录。 count() 里加条件判断 count函数用于统计在符合搜索条件的记录中,指定的表达式expr不为NULL的行数有多少&…...

PHP的SHA256WithRSA签名和Curl POST请求函数
getCustomerEncryptionKey - 获取加密秘钥 /** * 获取加密秘钥 * param array $params * return string */ public function getCustomerEncryptionKey(array $params): string { //1.ASCII码(字典序-升序)排序…...
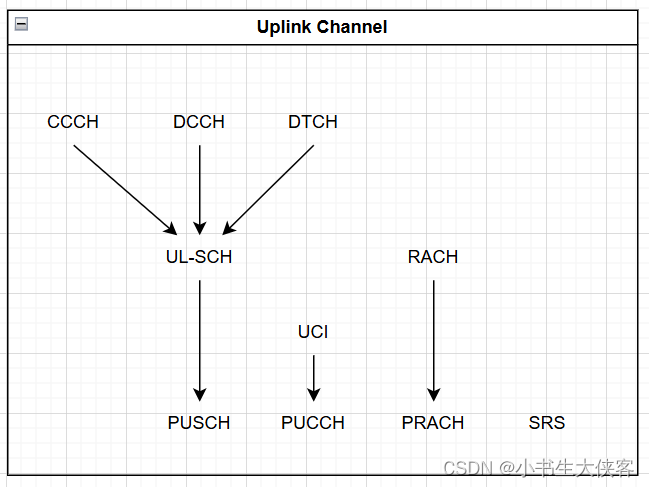
5G RAN
两个entity:NodeB、UE entity之间传输数据的东东 entity内部的流水线岗位:L3/L2/L1 岗位之间是消息交互/信令交互...
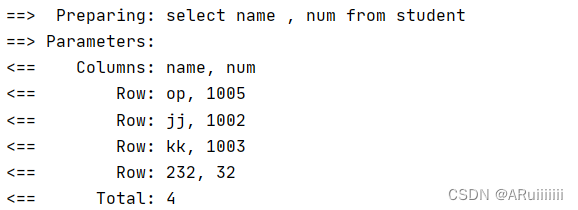
Mybatis动态sql标签
动态SQL标签简介: MyBatis的一个强大的特性之一通常是它的动态SQL能力。如果你有使用JDBC或其他相似框架的经验,你就明白条件地串联SQL字符串在一起是多么的痛苦,确保不能忘了空格或在列表的最后省略逗号。动态SQL可以彻底处理这种痛苦。 Mybatis中实现动态sql的标签有&#x…...
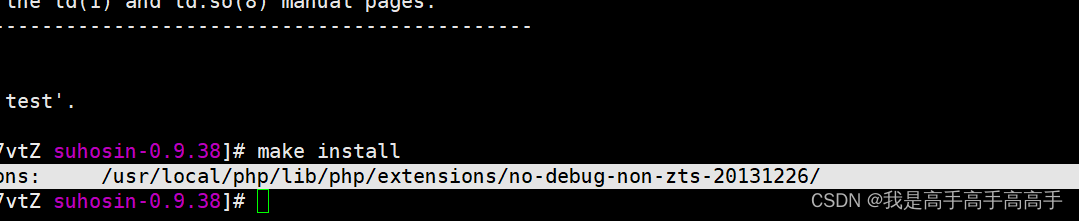
Linux CentOS Suhosin禁用php5.6版本eval函数详细图文教程
方法一:PHP_diseval_extension禁用 Linux CentOS 禁用php的eval函数详细图文教程_centos php 禁用 eval-CSDN博客 这个方法make报错,懒得费时间处理,直接用第二种 方法二:suhosin禁用 服务器只装了一个PHP5.6版本,一…...

这5招底层逆袭玄学,一个人越来越厉害的秘诀
在这个充满机遇与挑战的时代,每个人都渴望能够逆袭成功,摆脱底层的束缚,成为人生赢家。 然而,现实往往是残酷的,许多人在追逐梦想的路上遭遇挫折,甚至迷失了方向。 那么,有没有一些"…...
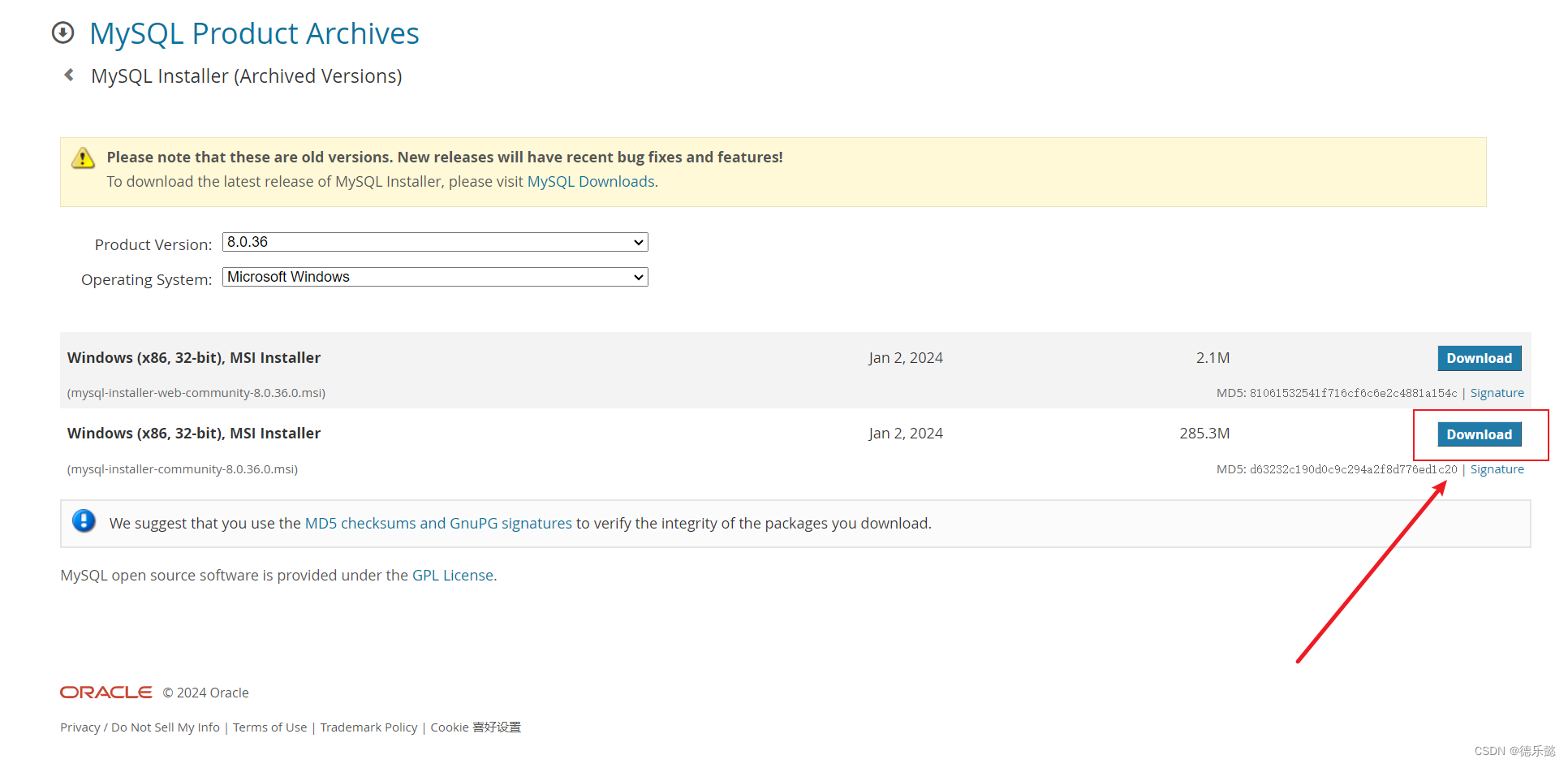
MySQL自学教程:1. MySQL简介与安装
MySQL简介与安装 一、MySQL简介二、MySQL安装(一)Windows系统上的安装(二)Linux系统上的安装(以Ubuntu为例)(三)Mac OS系统上的安装三、安装后的基本配置四、总结一、MySQL简介 MySQL是一个流行的开源关系型数据库管理系统(RDBMS),广泛应用于各种业务场景,从小型个…...

Jenkins多stage共享同一变量方式
在第一个stage中为这个变量赋值,在其它stage中使用这个变量 import java.nio.file.Files import java.nio.file.Path import java.nio.file.Paths import java.nio.file.StandardCopyOption import groovy.json.JsonOutput import groovy.json.JsonSlurper// 共享的…...

Ant design Vue 表格中显示不同的状态(多条件显示)
比如:后端一个字段有多种状态: 那么后端接口会返回:0 或者 1 或者 2 其中一个,前端需要展示的是对应的文字,像简单的只有两个状态的可以直接在列里面操作: {title: 状态,dataIndex: usable,customRender: …...
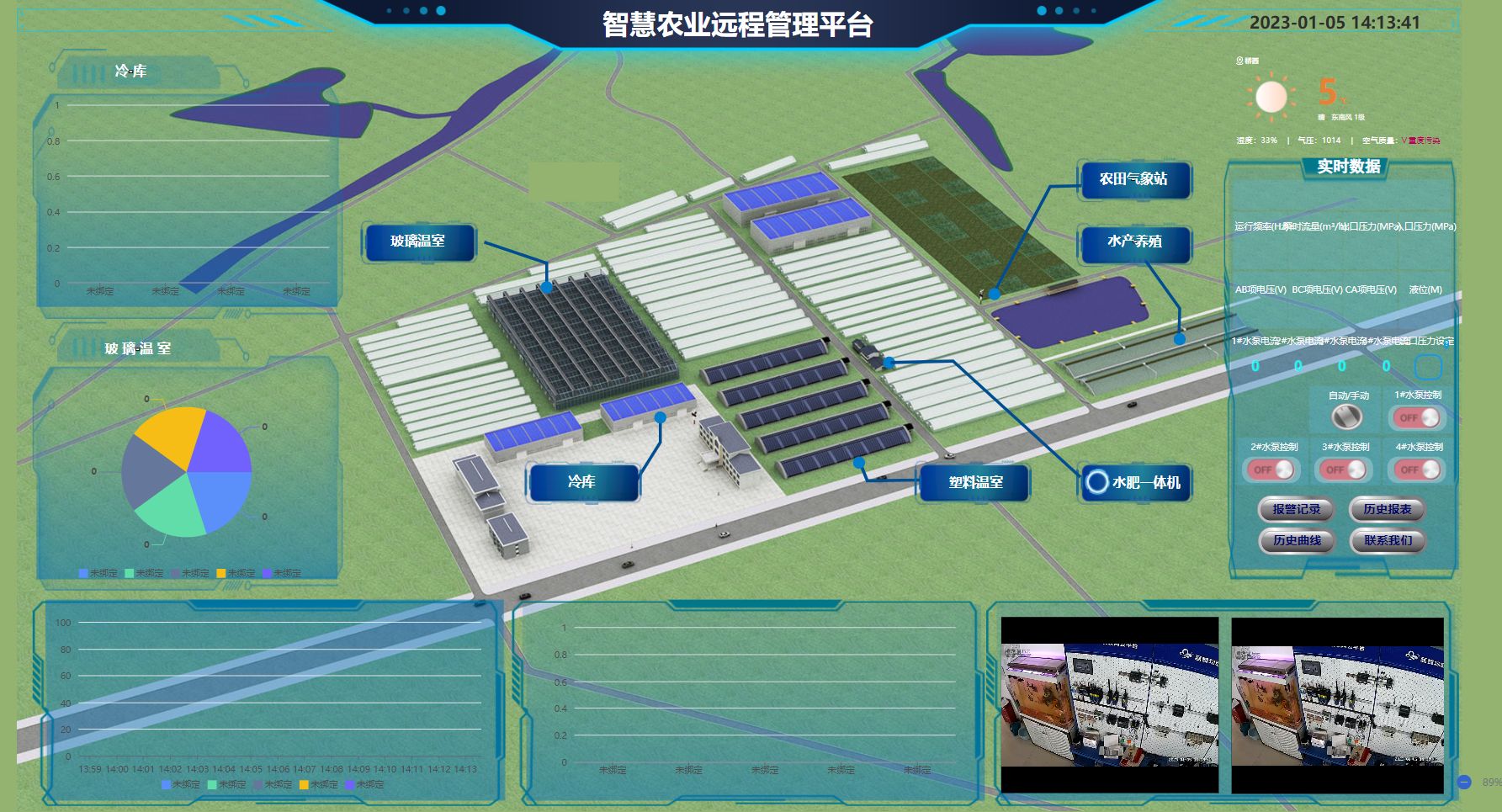
如何借助物联网实现土壤监测与保护
如何借助物联网实现土壤监测与保护 高标准农田信息化是指利用现代信息技术,如物联网、大数据、云计算等,对农田进行数字化、智能化的管理,以提高农田的生产效率和可持续发展能力。其中,土壤监测与保护是农田信息化的重要内容之一…...
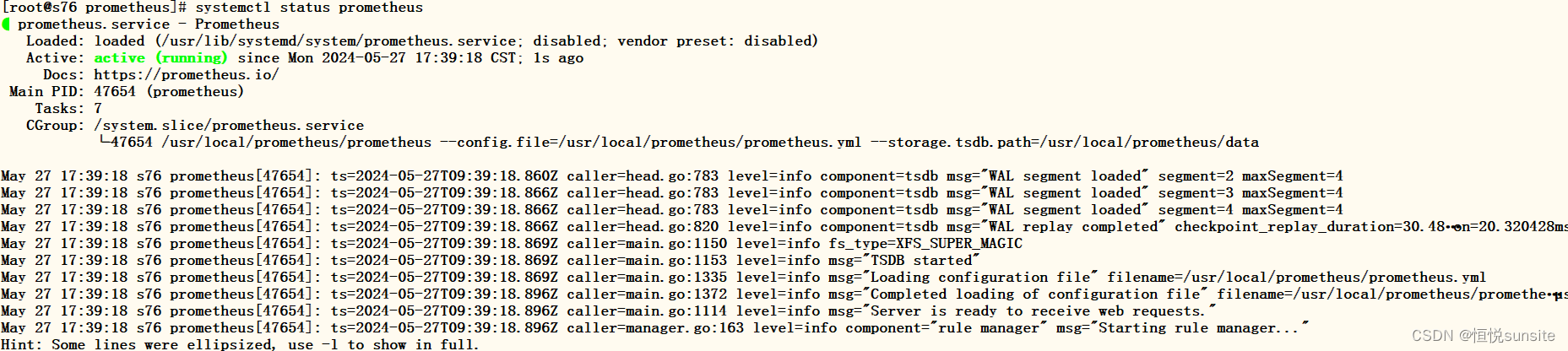
Linux之prometheus安装和使用简介(一)
一、prometheus简介 普罗米修斯Prometheus是一个开源系统监控和警报工具包,最初构建于SoundCloud。自2012年成立以来,许多公司和组织都采用了普罗米修斯,该项目拥有非常活跃的开发人员和用户社区。它现在是一个独立的开源项目,独立…...

orcle数据表空间操作sql
orcle数据表空间操作sql 1.查询表空间路径: select t1.name,t2.name from v$tablespace t1,v$datafile t2 where t1.ts#t2.ts#2.删除表空间和空间里的表: drop tablespace LTSYSDATA01 including contents and datafiles;1、表空间的路径、名称查询&…...
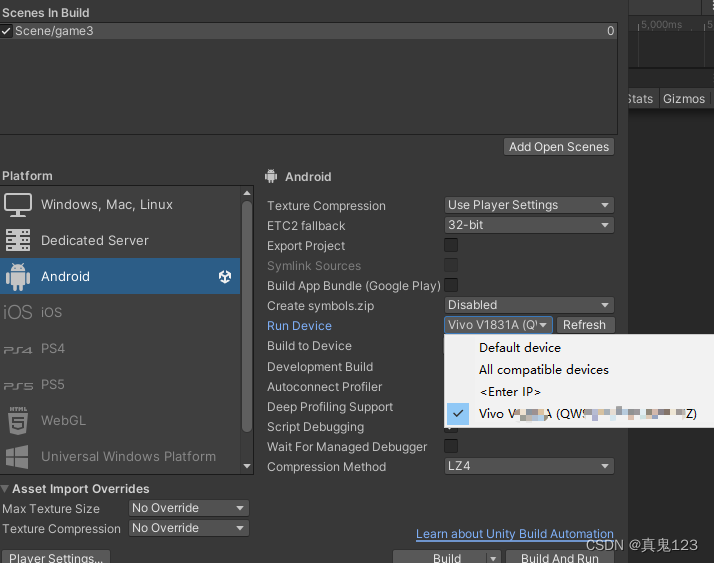
【Unity Android】Unity链接安卓手机调试
一、物理连接手机 1.USB数据线链接 2.打开开发者模式 大部分手机在手机设置->系统管理->关于手机->软件版本型号中,点击7次以上,来开启系统管理中的开发者模式选项。 3.打开USB调试 打开开发者模式后,开启USB调试 二、Unity中…...
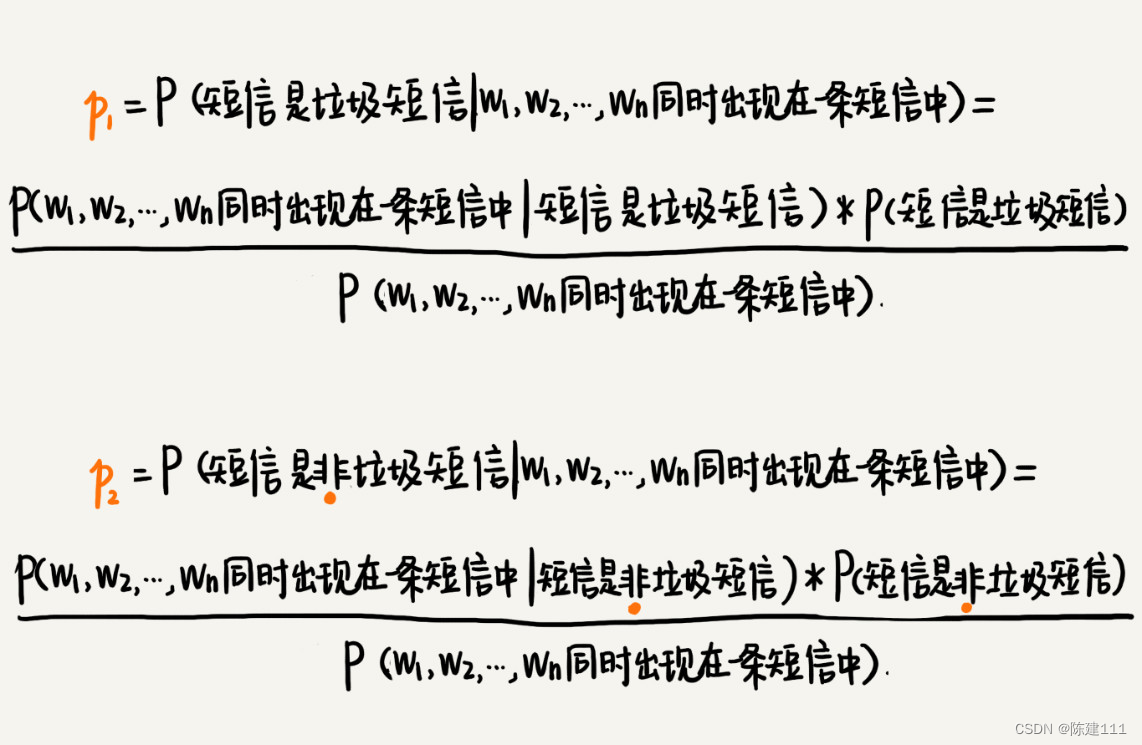
数据结构与算法笔记:高级篇 - 概率统计:如何利用朴素贝叶斯算法过滤垃圾短信?
概述 上篇文章我们讲到,如何用位图、布隆过滤器,来过滤重复数据。本章,我们再讲一个跟过滤相关的问题,如果过滤垃圾短信? 垃圾短信和骚扰电话,我想每个人都收到过吧?买房、贷款、投资理财、开…...
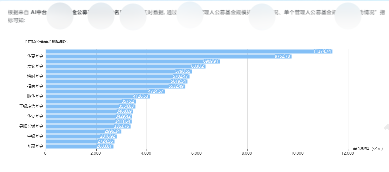
vue3中通过vditor插件实现自定义上传图片、录入echarts、脑图、markdown语法的编辑器
1、下载Vditor插件 npm i vditor 我的vditor版本是3.10.2,大家可以自行选择下载最新版本 官网:Vditor 一款浏览器端的 Markdown 编辑器,支持所见即所得(富文本)、即时渲染(类似 Typora)和分屏 …...
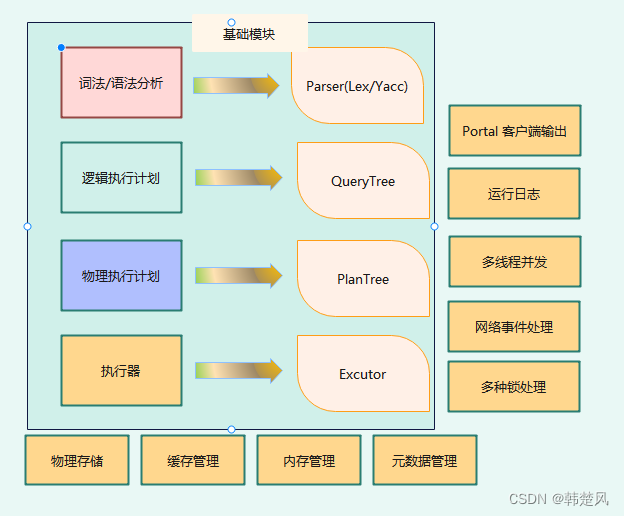
揭示数据库内核的奥秘--手写数据库toadb开源项目
揭示数据库内核的奥秘–手写数据库toadb 数据为王的时代 在信息化时代,数据已成为企业和应用不可或缺的核心,而数据库不仅是数据的仓库,更是支撑业务决策、系统运行的基石。对于求职者而言,掌握数据库知识已成为求职市场上的必考…...

Grafana调整等待时间,避免Gateway timeout报错
使用Grafana的HTTP时,有些即时数据需要运算量与时间,而grafana的默认timeout是30秒,因此需要通过修改配置文件,避免grafana提前中断连接 修改原始配置文件: 删除;调整timeout30为timeout60 # This setting also applies to cor…...

MetaGPT全面指南:多代理协作框架的深入解析与应用
文章目录 理解MetaGPT1.1 MetaGPT的基础1.2 MetaGPT的独特之处1.3 MetaGPT在AI领域的应用 MetaGPT的工作原理2.1 训练2.2 微调2.3 推理2.4 多代理协作的概念2.5 如何分配角色给GPTs2.6 复杂任务的完成过程 实际应用3.1 客户支持3.2 内容创作3.3 教育3.4 医疗保健3.5 在企业中的…...

图的关键路径算法
关键路径算法(Critical Path Method, CPM)是一种用于项目管理和调度的技术,通过分析项目任务的最早开始时间、最晚完成时间和总时差,找出项目中关键的任务路径。这条关键路径决定了项目的最短完成时间,因为关键路径上的…...
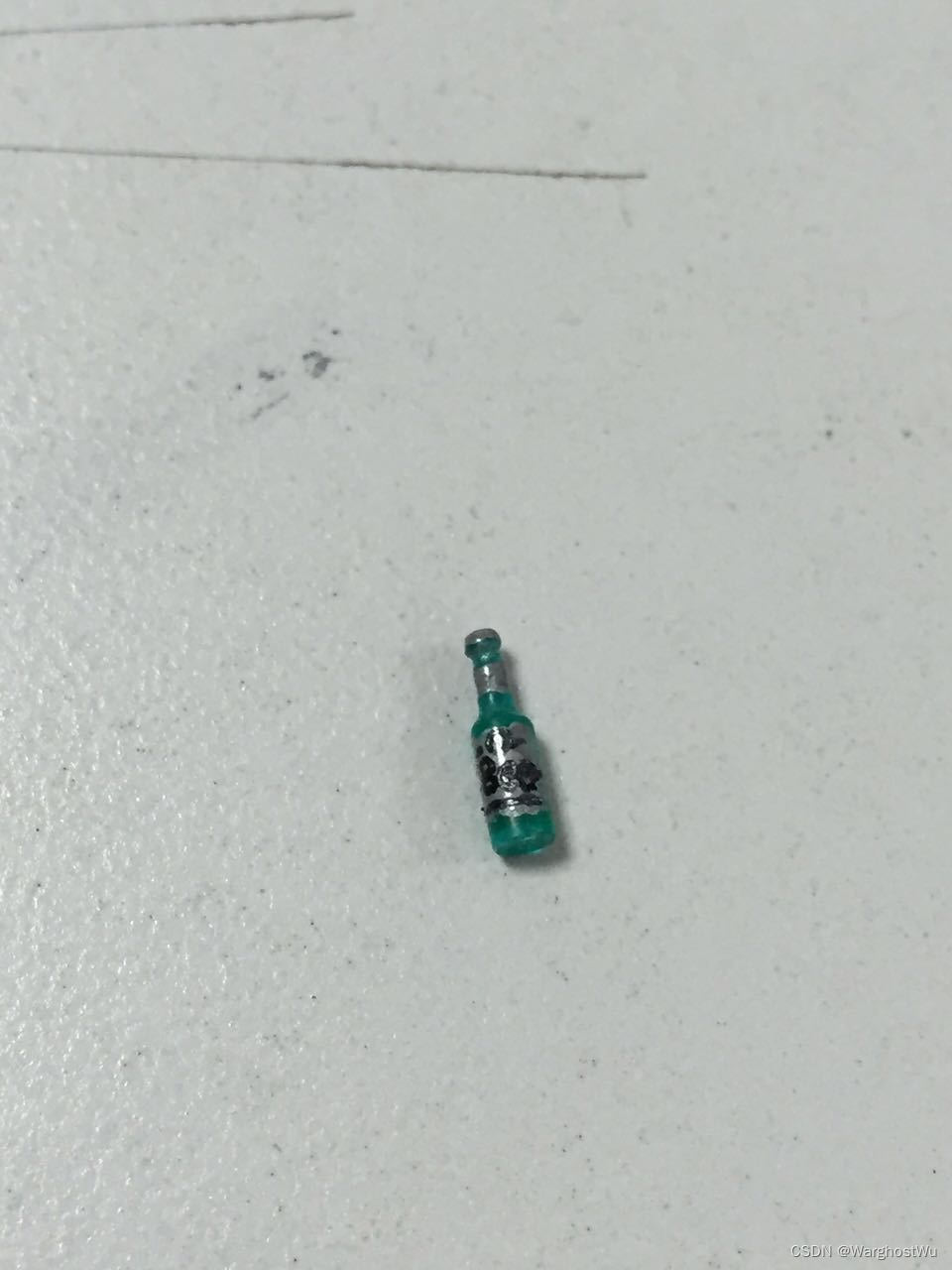
模型情景制作-冰镇啤酒
夏日炎炎,当我们在真实世界中开一瓶冰镇啤酒的时候,我们也可以为模型世界中的人物添加一些冰镇啤酒。 下面介绍一种快速酒瓶制造方法,您只需要很少工具: 截取尽量直的流道(传说中的板件零件架),将其夹在您的…...

网页实现黑暗模式的几种方式
## 实现暗黑模式的最佳方式 在现代网页设计中,暗黑模式已成为提高用户体验的重要功能。实现暗黑模式不仅可以减少用户眼睛的疲劳,还能在某些情况下节省设备电量。本文将介绍实现暗黑模式的几种最佳方式。 ### 使用 CSS 变量 (CSS Custom Properties) …...
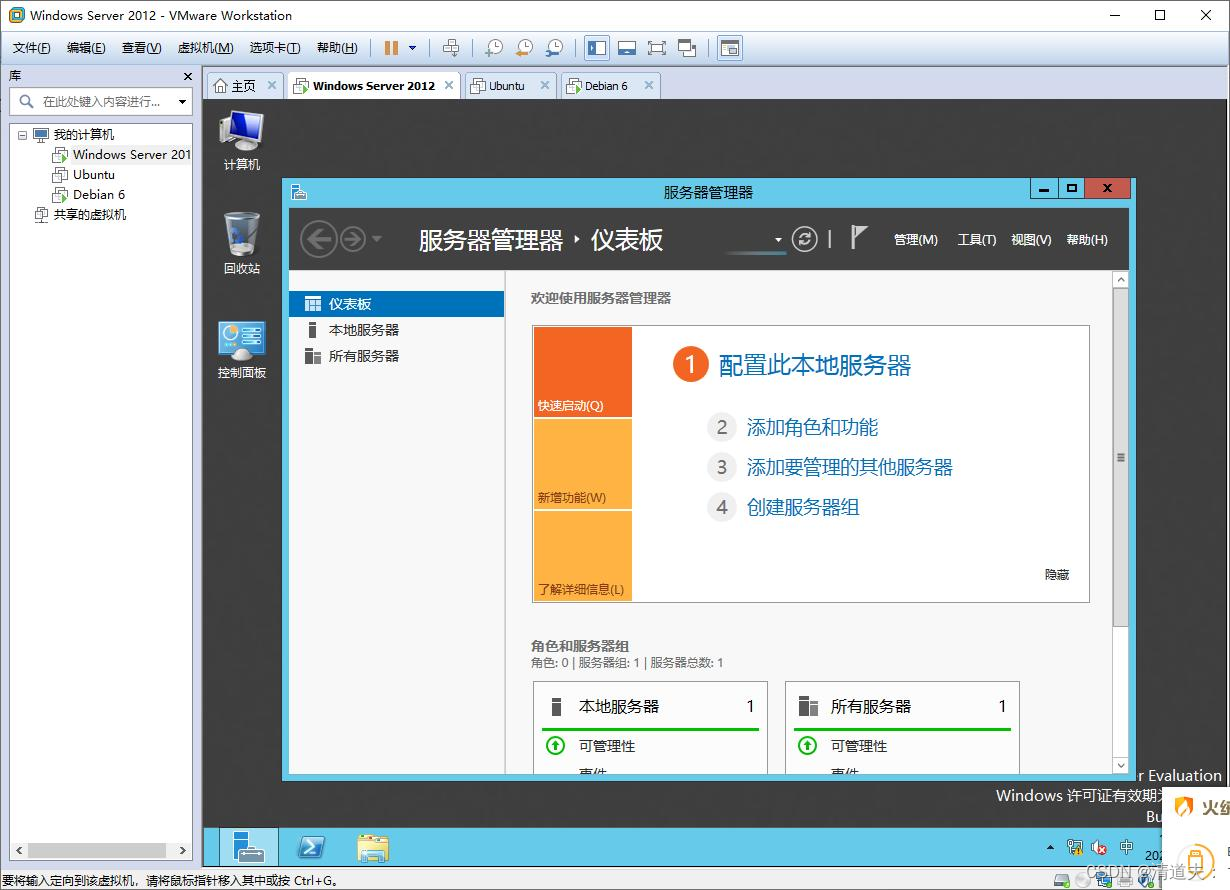
VMware Workstation环境下,邮件(E-Mail)服务的安装配置,并用Windows7来验证测试
需求说明: 某企业信息中心计划使用IP地址17216.11.0用于虚拟网络测试,注册域名为xyz.net.cn.并将172.16.11.2作为主域名的服务器(DNS服务器)的IP地址,将172.16.11.3分配给虚拟网络测试的DHCP服务器,将172.16.11.4分配给虚拟网络测试的web服务器,将172.16.11.5分配给FTP服务器…...

《信号与系统》复试建议
目录 第一章 绪论 第二章 连续时间系统的时域分析 第三章 傅立叶变换(重点) 第四章 拉普拉斯变换(重点) 第五章 傅立叶变换在通信系统中的应用 第六章 信号的矢量空间分析 第七章 离散时间系统的时域分析 第八章 Z变换与离…...

代码随想录训练营Day45
提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档 文章目录 前言一、打家劫舍二、打家劫舍2三、打家劫舍3 前言 提示:这里可以添加本文要记录的大概内容: 今天是跟着代码随想录刷题的第45天ÿ…...
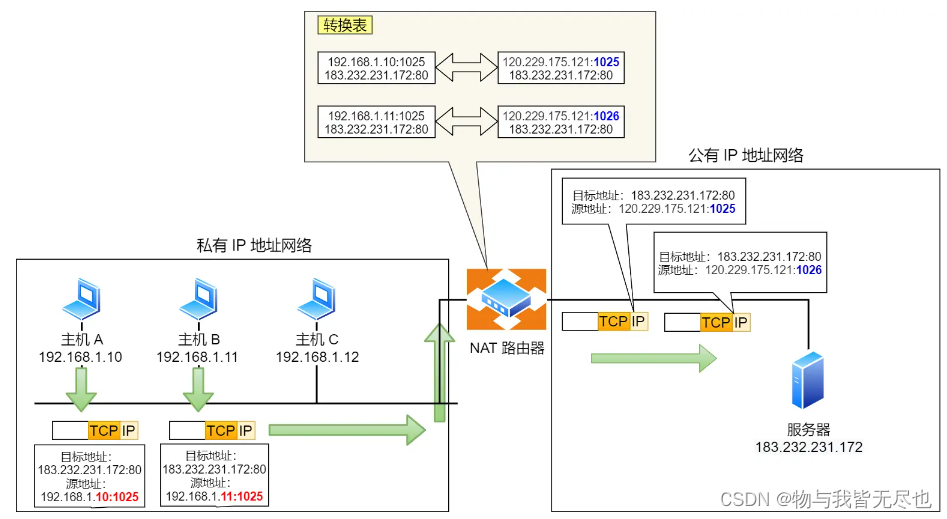
NAT和内网穿透
NAT(Network Address Translation,网络地址转换)是一种广泛应用于计算机网络的技术,其主要目的是为了解决IPv4地址空间的短缺问题,并且增强网络安全。NAT技术允许一个私有网络内的多个设备共享一个或几个全局唯一的公共…...

android | 声明式编程!(笔记)
https://www.jianshu.com/p/c133cb7cac21 讲的不错 命令式UI (how to do) 声明式UI (what to do) what to do 也许有人会说Data Binding不是可以让XML自己"动"起来吗?没有错,Data Binding其实就是Compose诞生之前的一种声明式U方案,谷歌曾…...
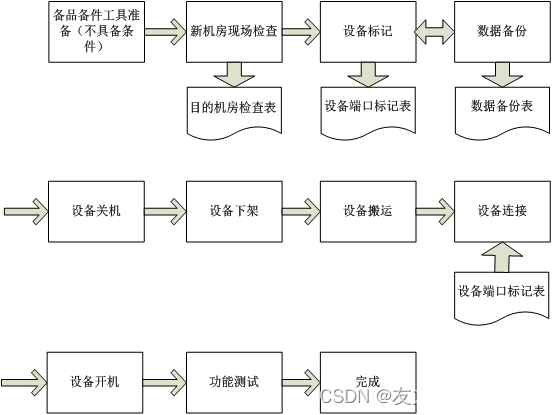
友力科技IDC机房搬迁方案流程分享
机房搬迁流程 系统搬迁实施流程包括:准备、拆卸、装运、安装、调试等五个流程,具体如下: 准备:包括相关人员和设备准备、新机房环境准备、网络环境、备份、现场所有设备打标签、模块、设备准备等准备工作。拆卸:主要只核心设备下…...
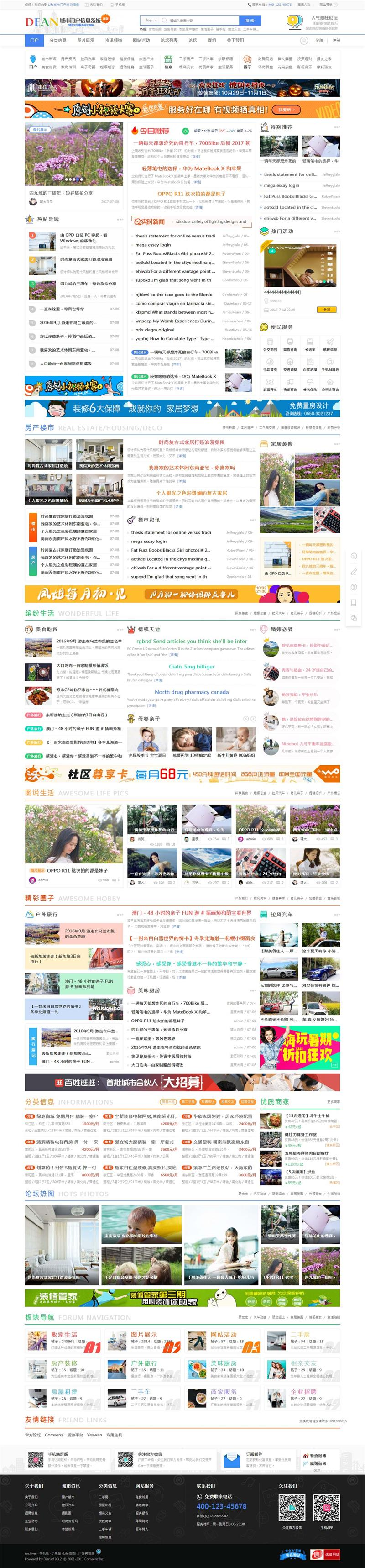
仿迪恩城市门户分类信息网discuz模板
Discuz x3.3模板 仿迪恩城市门户分类信息网 (GBK) Discuz模板 仿迪恩城市门户分类信息网(GBK)...
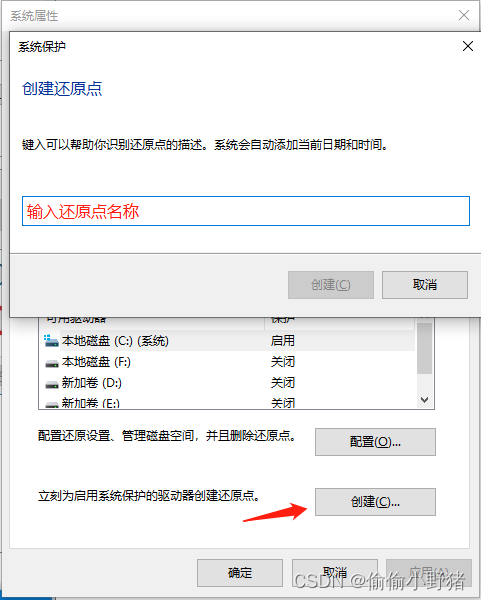
Windows 注册表是什么?如何备份注册表?
Windows注册表(Windows Registry)是微软Windows操作系统中的一个重要组件,用于存储系统和应用程序的配置信息和选项。下面就给大家详细讲解一下什么是注册表。 注册表的概念 Windows 注册表是一个集中管理的数据库,存储了系统、…...