【Langchain大语言模型开发教程】模型、提示和解析
🔗 LangChain for LLM Application Development - DeepLearning.AI
学习目标
1、使用Langchain实例化一个LLM的接口
2、 使用Langchain的模板功能,将需要改动的部分抽象成变量,在具体的情况下替换成需要的内容,来达到模板复用效果。
3、使用Langchain提供的解析功能,将LLM的输出解析成你需要的格式,如字典。
模型实例化
import os
from dotenv import load_dotenv ,find_dotenv
from langchain.chat_models import ChatOpenAI
from langchain.prompts import ChatPromptTemplate
_ = load_dotenv((find_dotenv())) //使用dotenv来管理你的环境变量
我们选用智谱的API【智谱AI开放平台】来作为我们的基座大模型,通过langchain的chatOpenAI接口来实例化我们的模型。
chat = ChatOpenAI(api_key=os.environ.get('ZHIPUAI_API_KEY'),base_url=os.environ.get('ZHIPUAI_API_URL'),model="glm-4",temperature=0.98)
这里我们选用的一个例子:通过prompt来转换表达的风格
提示模板化
我们定义一个prompt
template_string = """Translate the text \
that is delimited by triple backticks \
into a style that is {style}.\
text:```{text}```
"""
使用langchain的模板功能函数实例化一个模板(从输出可以看到这里是需要两个参数style和text)
prompt_template = ChatPromptTemplate.from_template(template_string)'''
ChatPromptTemplate(input_variables=['style', 'text'],
messages=[HumanMessagePromptTemplate(prompt=PromptTemplate(
input_variables=['style', 'text'],
template='Translate the text that is delimited
by triple backticks into a style that is {style}.text:```{text}```\n'))])
'''
设置我们想要转化的风格和想要转化的内容
#style
customer_style = """American English in a clam and respectful tone"""
#text
customer_email = """
Arrr,I be fuming that me blender lid \
flew off and splattered me kitchen walls \
with smoothie! And to make matters worse, \
the warranty don't cover the cost of \
cleaning up me kitchen. I need yer help \
right now,matey!
"""
这里我们实例化出我们的prompt
customer_messages = prompt_template.format_messages(style = customer_style,text= customer_email)'''
[HumanMessage(content="Translate the text that is delimited
by triple backticks into a style
that is American English in a clam and respectful tone.
text:
```\n
Arrr,I be fuming that me blender lid flew off and
splattered me kitchen walls with smoothie!
And to make matters worse,
the warranty don't cover the cost of cleaning up me kitchen.
I need yer help right now,matey!
\n```\n")]
'''
这里我们给出一个回复的内容和转化的格式
service_reply=
"""
Hey there customer,the warranty does
not cover cleaning expenses for your kitchen
because it's your fault that you misused your blender
by forgetting to put the lid on before starting the blender.
Tough luck! see ya!
"""service_style = """
a polite tone that speaks in English pirate
"""
实例化
service_messages = prompt_template.format_messages(style = service_style , text = service_reply)
调用LLM查看结果
service_response = chat(service_messages)
print(service_response.content)'''
Avast there, dear customer! Ye be knowin' that the warranty
be not stretchin' to cover the cleanin' costs of yer kitchen,
for 'tis a matter of misadventure on yer part.
Ye did forget to secure the lid upon the blender before engagement,
leading to a spot o' trouble. Aar,
such be the ways of the sea!
No hard feelings, and may the wind be at yer back on the next journey.
Fare thee well!
'''
回复结构化
我们现在获得了某个商品的用户评价,我们想要提取其中的关键信息(下面这种形式)
customer_review = """\
This leaf blower is pretty amazing. It has four settings:\
candle blower, gentle breeze, windy city, and tornado. \
It arrived in two days, just in time for my wife's \
anniversary present. \
I think my wife liked it so much she was speechless. \
So far I've been the only one using it, and I've been \
using it every other morning to clear the leaves on our lawn. \
It's slightly more expensive than the other leaf blowers \
out there, but I think it's worth it for the extra features.
"""{"gift": False,"delivery_days": 5,"price_value": "pretty affordable!"
}
构建一个prompt 模板
review_template = """\
For the following text, extract the following information:gift: Was the item purchased as a gift for someone else? \
Answer True if yes, False if not or unknown.delivery_days: How many days did it take for the product \
to arrive? If this information is not found, output -1.price_value: Extract any sentences about the value or price,\
and output them as a comma separated Python list.Format the output as JSON with the following keys:
gift
delivery_days
price_valuetext: {text}
"""
prompt_template = ChatPromptTemplate.from_template(review_template)
message = prompt_template.format_messages(text = customer_review)
reponse = chat(message)
下面是模型的回复看起来好像一样
{"gift": true,"delivery_days": 2,"price_value": ["It's slightly more expensive than the other leaf blowers out there, but I think it's worth it for the extra features."]
}
我们打印他的类型的时候,发现这其实是一个字符串类型,这是不能根据key来获取value值的。
引入Langchain的ResponseSchema
from langchain.output_parsers import ResponseSchema
from langchain.output_parsers import StructuredOutputParsergift_schema = ResponseSchema(name="gift",description="Was the item purchased as a gift for someone else? Answer True if yes,False if not or unknown.")
delivery_days_schema = ResponseSchema(name="delivery_days", description="How many days did it take for the product to arrive? If this information is not found,output -1.")
price_value_schema = ResponseSchema(name="price_value", description="Extract any sentences about the value or price, and output them as a comma separated Python list.")
response_schemas = [gift_schema,delivery_days_schema,price_value_schema]
output_parser = StructuredOutputParser.from_response_schemas(response_schemas)
format_instructions = output_parser.get_format_instructions()
查看一下我们构建的这个结构
重新构建prompt模板,并进行实例
review_template_2 = """\
For the following text, extract the following information:gift: Was the item purchased as a gift for someone else? \
Answer True if yes, False if not or unknown.delivery_days: How many days did it take for the product\
to arrive? If this information is not found, output -1.price_value: Extract any sentences about the value or price,\
and output them as a comma separated Python list.text: {text}{format_instructions}
"""prompt = ChatPromptTemplate.from_template(template=review_template_2)messages = prompt.format_messages(text=customer_review,format_instructions=format_instructions)
我们将结果进行解析
output_dict = output_parser.parse(reponse.content){'gift': 'True','delivery_days': '2','price_value': "It's slightly more expensive than the other leaf blowers out there, but I think it's worth it for the extra features."
}
我们再次查看其类型,发现已经变成了字典类型,并可以通过key去获取value值。
相关文章:

【Langchain大语言模型开发教程】模型、提示和解析
🔗 LangChain for LLM Application Development - DeepLearning.AI 学习目标 1、使用Langchain实例化一个LLM的接口 2、 使用Langchain的模板功能,将需要改动的部分抽象成变量,在具体的情况下替换成需要的内容,来达到模板复用效…...

Flutter 中的基本数据类型:num、int 和 double
在 Dart 编程语言中,数值类型的基础是 num,而 int 和 double 则是 num 的子类型。在开发 Flutter 应用时,理解这三者的区别和使用场景是非常重要的。本文将详细介绍 num、int 和 double 的定义及其使用区别。 num num 是 Dart 中的数值类型…...
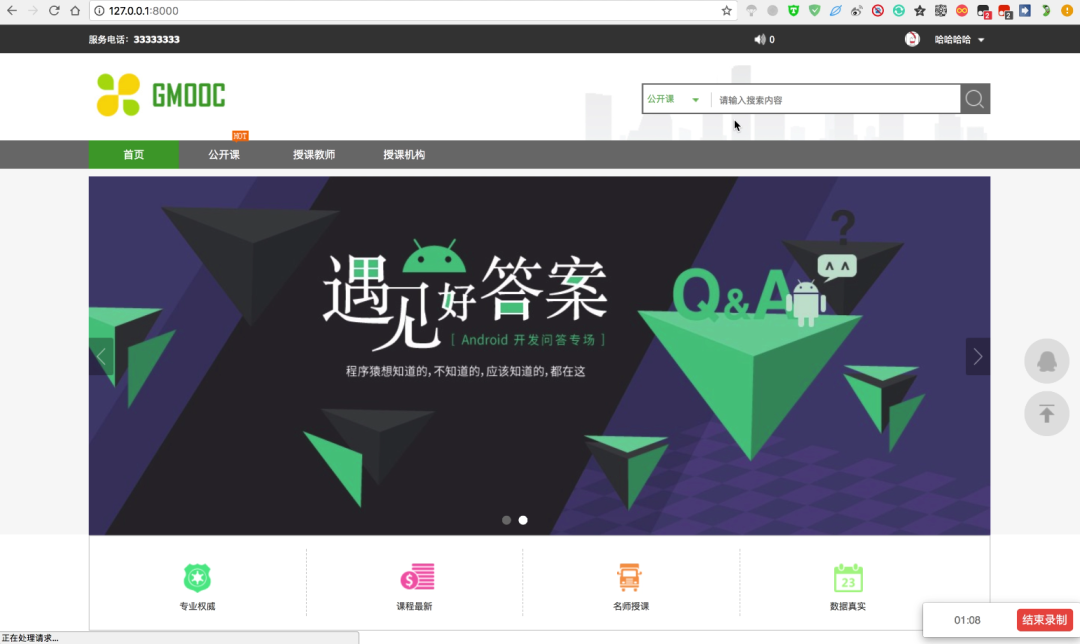
基于Python+Django,开发的一个在线教育系统
一、项目简介 使用Python的web框架Django进行开发的一个在线教育系统! 二、所需要的环境与组件 Python3.6 Django1.11.7 Pymysql Mysql pure_pagination DjangoUeditor captcha xadmin crispy_forms 三、安装 1. 下载项目后进入项目目录cd Online-educ…...

密码学原理精解【9】
这里写目录标题 迭代密码概述SPN具体算法过程SPN算法基本步骤举例说明注意 轮换-置换网络一、定义与概述二、核心组件三、加密过程四、应用实例五、总结 轮函数理论定义与作用特点与性质应用实例总结 迭代密码理论定义与原理特点与优势应用场景示例发展趋势 AES特点概述一、算法…...
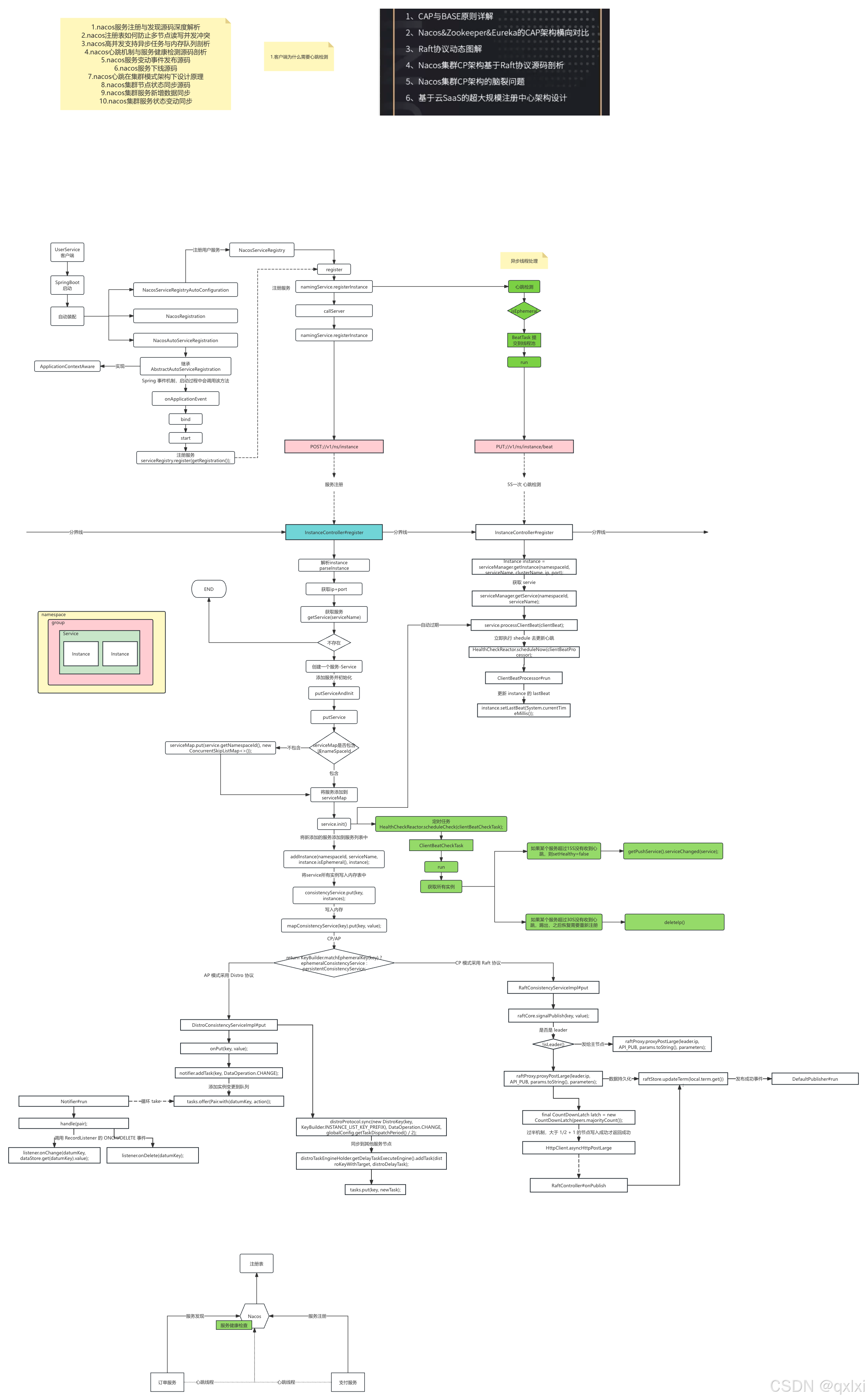
【Nacos】Nacos服务注册与发现 心跳检测机制源码解析
在前两篇文章,介绍了springboot的自动配置原理,而nacos的服务注册就依赖自动配置原理。 Nacos Nacos核心功能点 服务注册 :Nacos Client会通过发送REST请求的方式向Nacos Server注册自己的服务,提供自身的元数据,比如ip地址、端…...

python 66 个冷知识 0720
66个有趣的Python冷知识 一行反转列表 使用切片一行反转列表:reversed_list my_list[::-1] 统计文件单词数量 使用 collections.Counter 统计文件中每个单词的数量:from collections import Counter; with open(file.txt) as f: word_count Counter(f…...
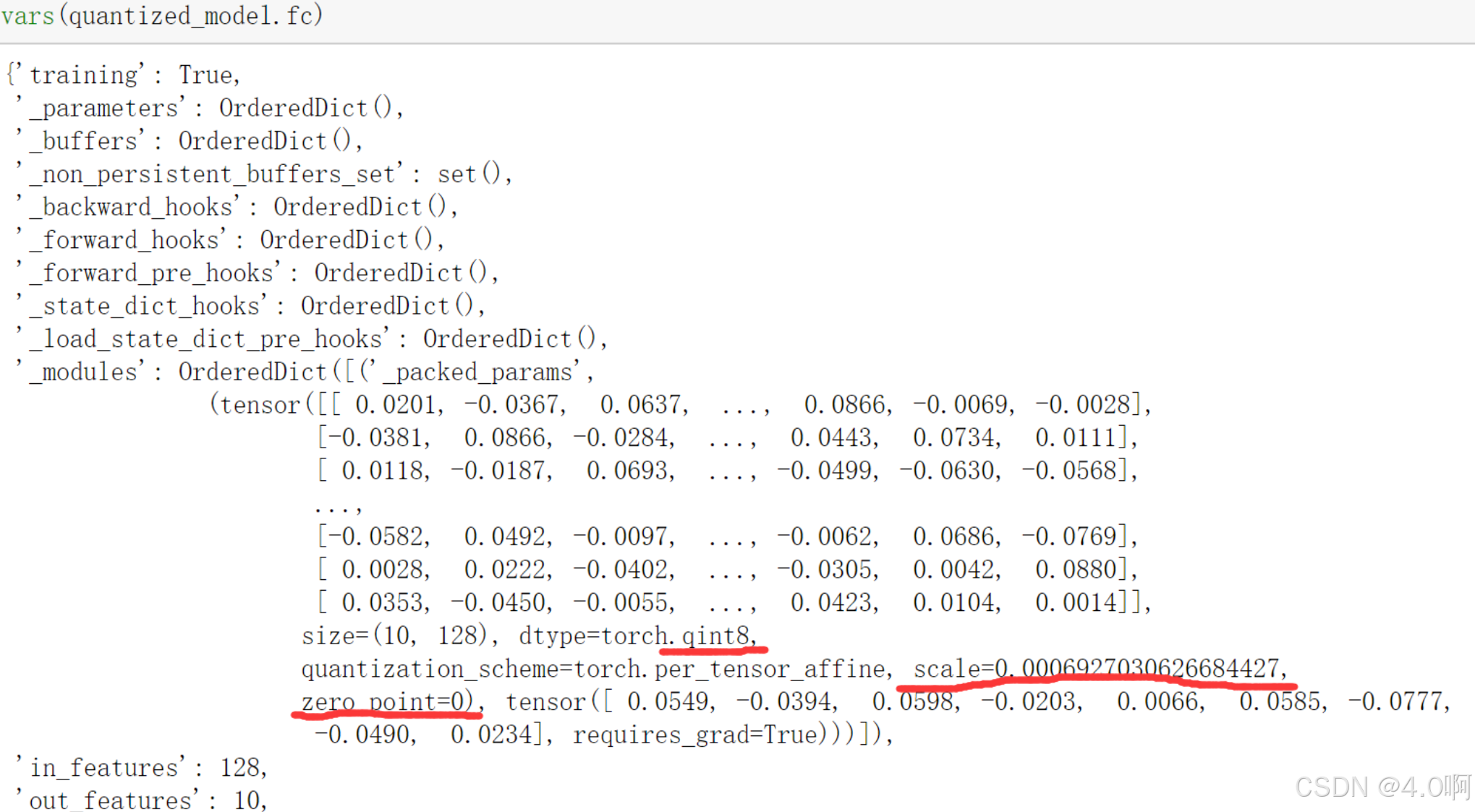
利用PyTorch进行模型量化
利用PyTorch进行模型量化 目录 利用PyTorch进行模型量化 一、模型量化概述 1.为什么需要模型量化? 2.模型量化的挑战 二、使用PyTorch进行模型量化 1.PyTorch的量化优势 2.准备工作 3.选择要量化的模型 4.量化前的准备工作 三、PyTorch的量化工具包 1.介…...
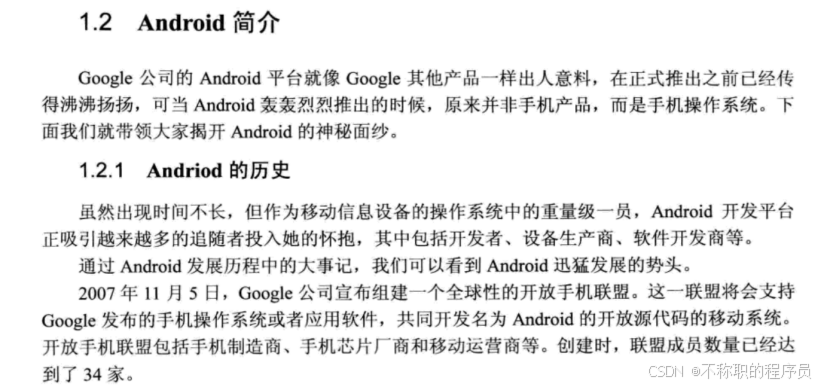
Android 小白菜鸟从入门到精通教程
前言 Android一词最早出现于法国作家利尔亚当(Auguste Villiers de l’Isle-Adam)在1886年发表的科幻小说《未来的夏娃》(L’ve future)中。他将外表像人的机器起名为Android。从初学者的角度出发,通过通俗易懂的语言…...
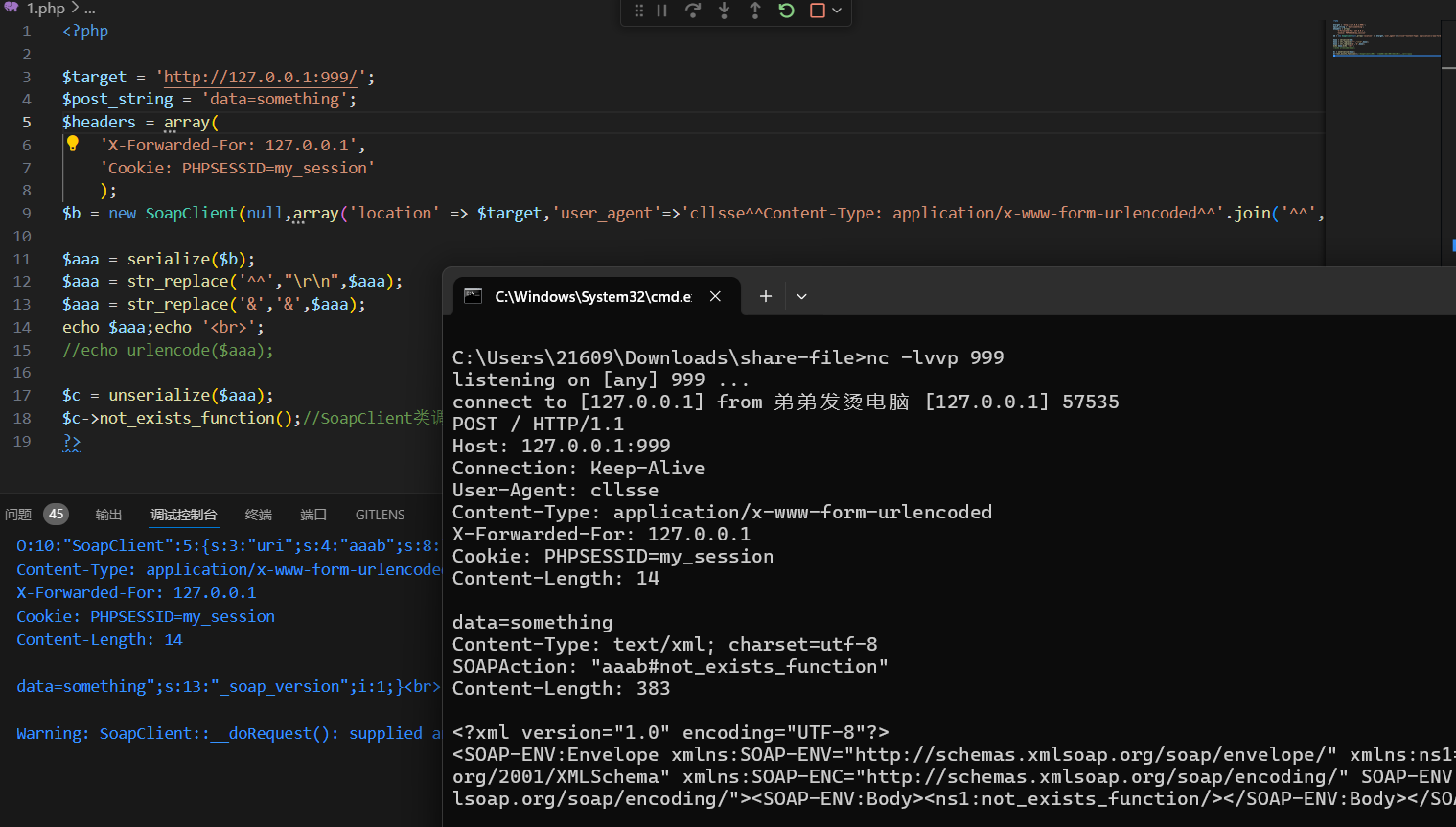
php相关
php相关 借鉴了小迪安全以及各位大佬的博客,如果一切顺利,会不定期更新。 如果感觉不妥,可以私信删除。 默认有php基础。 文章目录 php相关1. php 缺陷函数1. 与2. MD53. intval()4. preg_match() 2. php特性1. php字符串解析特性2. 杂…...

uniapp上传功能用uni-file-picker实现
文章目录 html代码功能实现css样式代码 html代码 <uni-file-pickerselect"onFileSelected"cancel"onFilePickerCancel"limit"1"class"weightPage-upload-but"file-mediatype"image"></uni-file-picker><imag…...
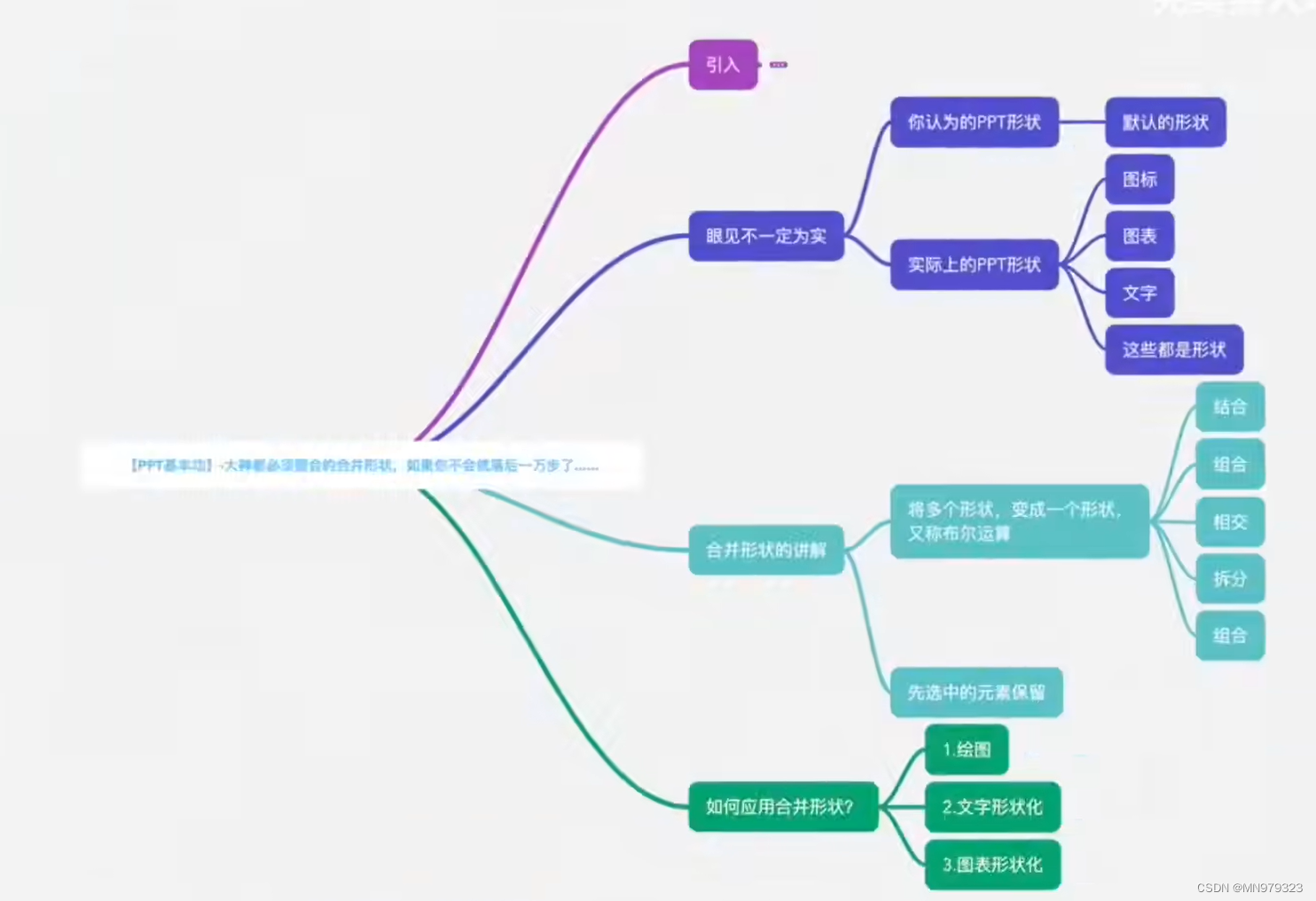
【PPT笔记】1-3节 | 默认设置/快捷键/合并形状
文章目录 说明笔记1 默认设置1.1 OFFICE版本选择1.1.1 Office某某数字专属系列1.1.2 Office3651.1.3 产品信息怎么看 1.2 默认设置1.2.1 暗夜模式1.2.2 无限撤回1.2.3 自动保存(Office2013版本及以上)1.2.4 图片压缩1.2.5 字体嵌入1.2.6 多格式导出1.2.7…...
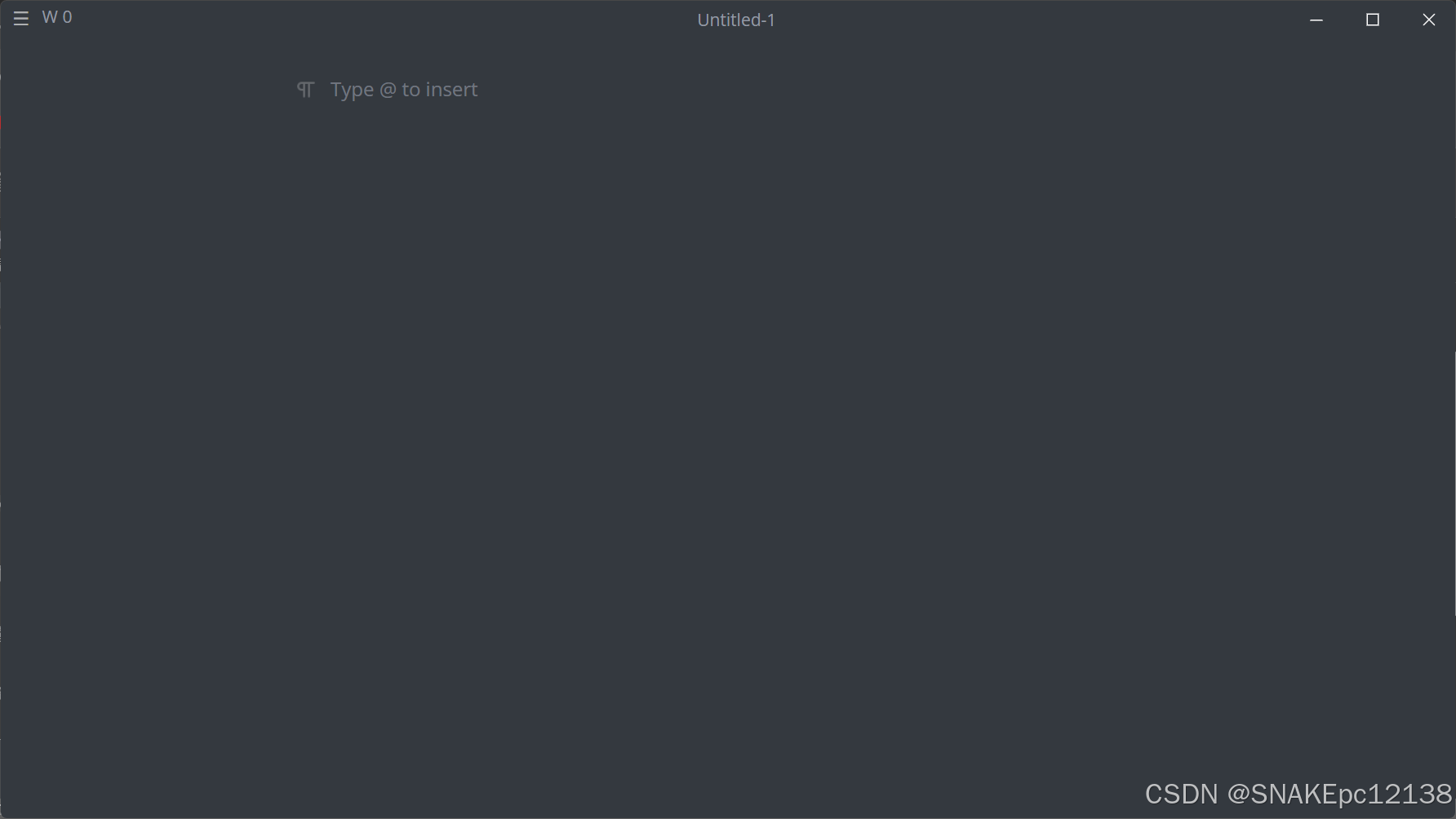
Qt中的高分辨率及缩放处理
写在前面 使用Qt开发界面客户端,需要考虑不同分辨率及缩放对UI界面的影响,否则会影响整体的交互使用。 问题 高分辨率/缩放设备上图片/图标模糊 若不考虑高分辨及缩放处理,在高分辨率/缩放设备上,软件中的图片、图标可能会出现…...
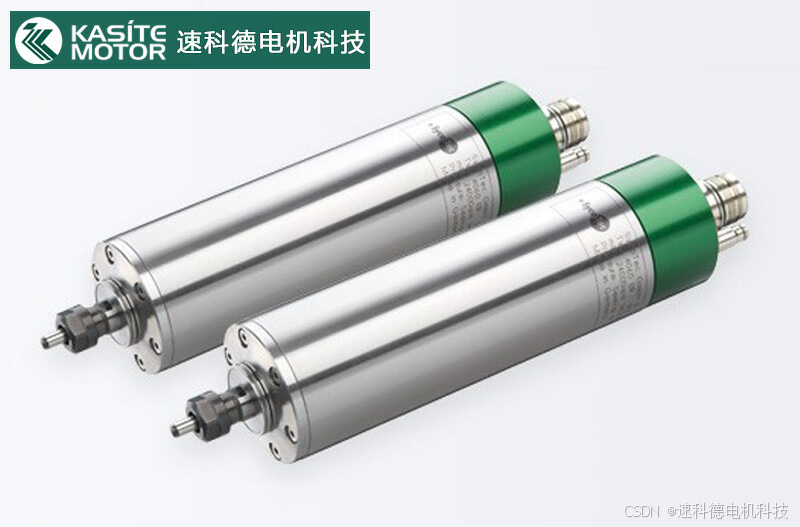
电机泵盖机器人打磨去毛刺,选德国进口高精度主轴
机器人打磨去毛刺该如何选择主轴呢?首先我们需要考虑的是工件的材质,电机泵盖通常使用铸铁、不锈钢、合金钢等金属材质,因此这类保持的硬度较高,一般会选择功率、扭矩较大的德国进口高精度主轴Kasite 4060 ER-S。 Kasite 4060 ER-…...

Android init.rc各阶段的定义和功能
Android开机优化系列文档-CSDN博客 Android 14 开机时间优化措施汇总-CSDN博客Android 14 开机时间优化措施-CSDN博客根据systrace报告优化系统时需要关注的指标和优化策略-CSDN博客Android系统上常见的性能优化工具-CSDN博客Android上如何使用perfetto分析systrace-CSDN博客A…...
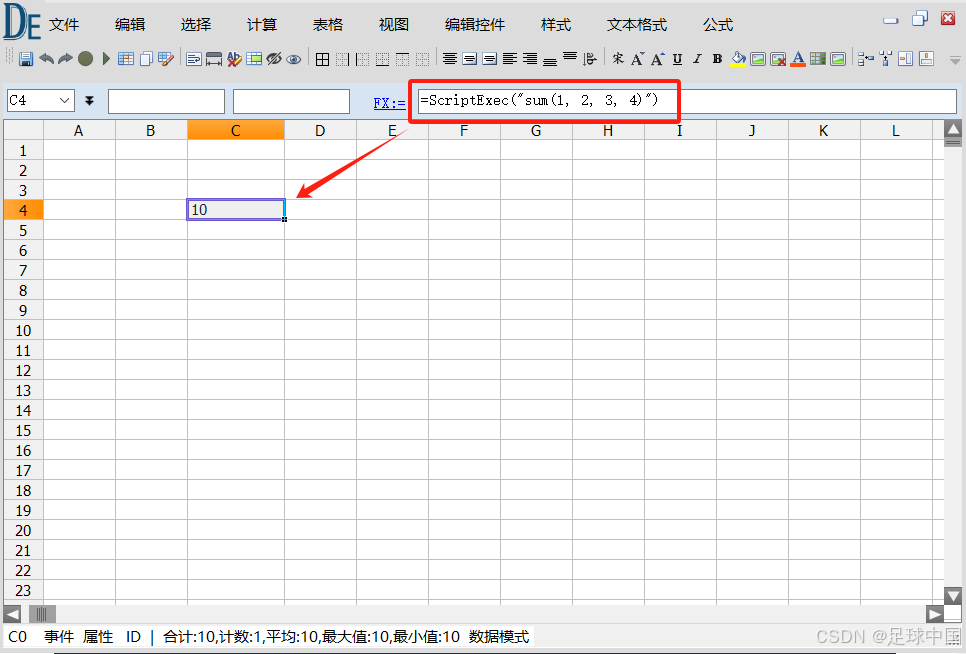
.net dataexcel 脚本公式 函数源码
示例如: ScriptExec(""sum(1, 2, 3, 4)"") 结果等于10 using Feng.Excel.Builder; using Feng.Excel.Collections; using Feng.Excel.Interfaces; using Feng.Script.CBEexpress; using Feng.Script.Method; using System; using System.Collections.Gen…...
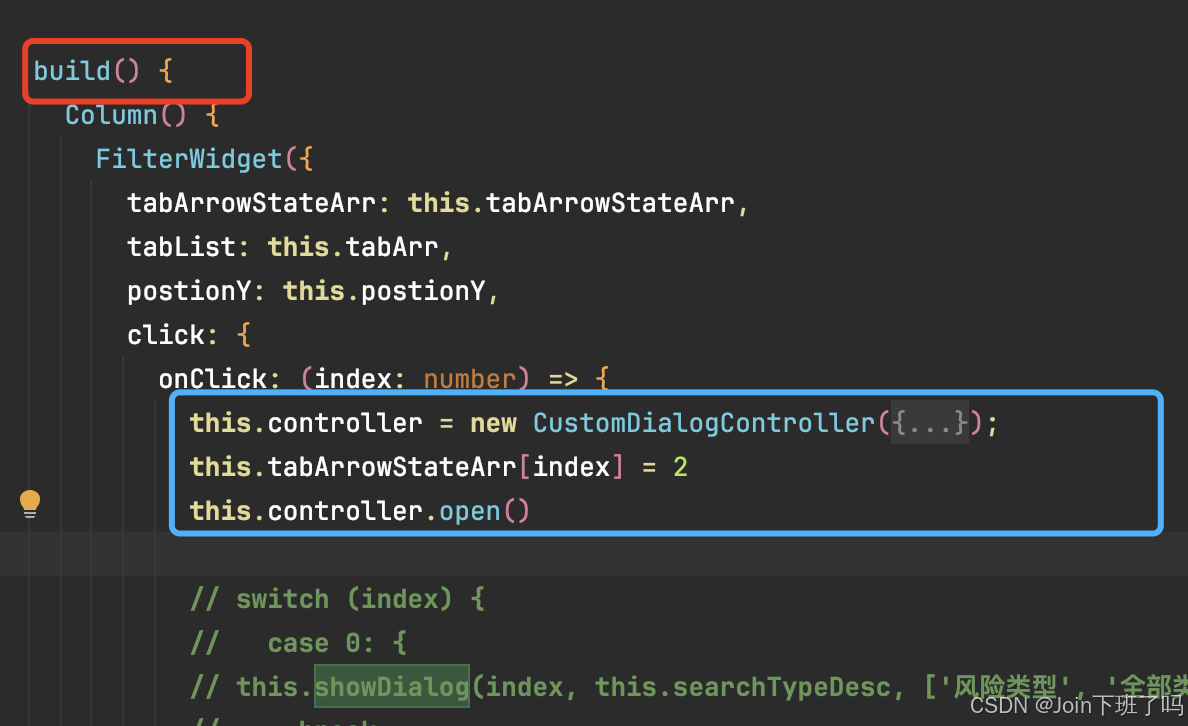
HarmonyOS ArkUi @CustomDialog 和promptAction.openCustomDialog踩坑以及如何选择
CustomDialog 内使用Link,如何正常使用 错误使用方式: 定义一个函数,在函数内使用弹窗,如下面代码showDialog: 这种使用方式,无法在自定义的CustomDialog内使用 Link,进行父子双向绑定&#x…...

Python面试题:详细讲解Python的多线程与多进程编程问题
在 Python 中,多线程和多进程编程是并发编程的两种主要方式,用于提高程序的执行效率和响应性。虽然它们都可以实现并发执行,但它们的工作原理和适用场景有所不同。以下是对 Python 多线程和多进程编程的详细讲解,包括它们的工作原…...
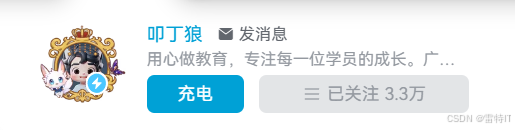
前端Canvas入门——用canvas写五子棋?
前言 五子棋的实现其实不难,因为本身就是一个很小的游戏。 至于画线什么的,其实很简单,都是lineTo(),moveTo()就行了。 难的在于——怎么让棋子落入到指定的格子上,怎么判断连子胜利。 当然啦,这部分是…...

[PaddlePaddle飞桨] PaddleDetection-通用目标检测-小模型部署
PaddleDetection的GitHub项目地址 推荐环境: PaddlePaddle > 2.3.2 OS 64位操作系统 Python 3(3.5.1/3.6/3.7/3.8/3.9/3.10),64位版本 pip/pip3(9.0.1),64位版本 CUDA > 10.2 cuDNN > 7.6pip下载指令: python -m pip i…...
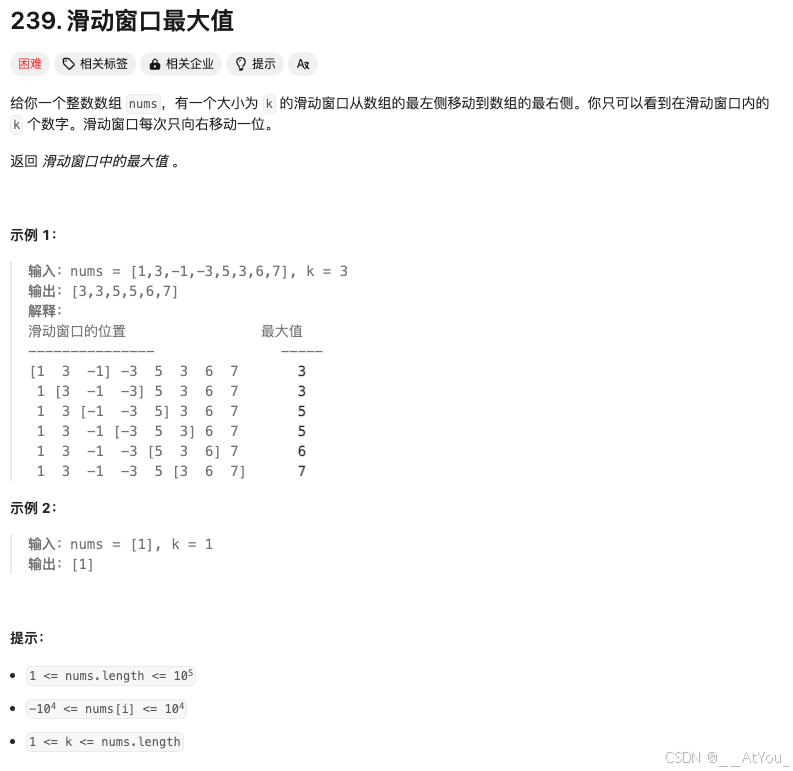
Golang | Leetcode Golang题解之第239题滑动窗口最大值
题目: 题解: func maxSlidingWindow(nums []int, k int) []int {n : len(nums)prefixMax : make([]int, n)suffixMax : make([]int, n)for i, v : range nums {if i%k 0 {prefixMax[i] v} else {prefixMax[i] max(prefixMax[i-1], v)}}for i : n - 1…...

深度解析:在 React 中实现类似 Vue 的 KeepAlive 组件
在前端开发中,Vue 的 keep-alive 组件是一个非常强大的工具,它可以在组件切换时缓存组件的状态,避免重新渲染,从而提升性能。那么,如何在 React 中实现类似的功能呢?本文将带你深入探讨,并通过代…...

2024-7-20 IT新闻
目录 微软全球IT系统故障 中国量子计算产业峰会召开 其他IT相关动态 微软全球IT系统故障 后续处理: 微软和CrowdStrike均迅速响应,发布了相关声明并部署了修复程序。CrowdStrike撤销了有问题的软件更新,以帮助用户恢复系统正常运作。微软也…...
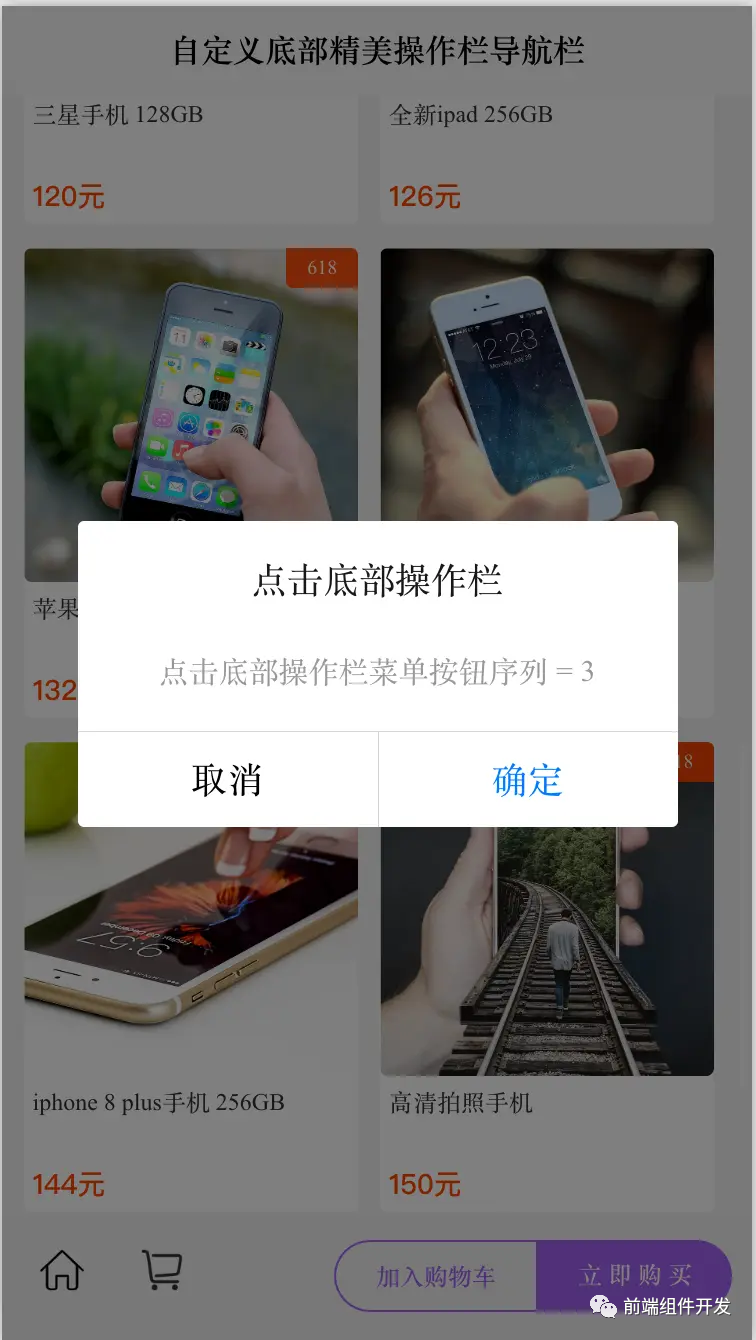
前端组件化开发:以Vue自定义底部操作栏组件为例
摘要 随着前端技术的不断演进,组件化开发逐渐成为提升前端开发效率和代码可维护性的关键手段。本文将通过介绍一款Vue自定义的底部操作栏组件,探讨前端组件化开发的重要性、实践过程及其带来的优势。 一、引言 随着Web应用的日益复杂,传统的…...
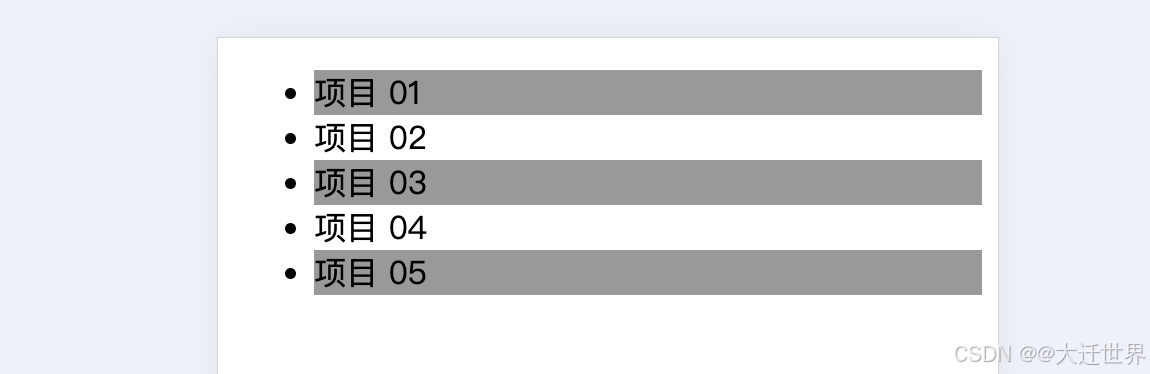
11.斑马纹列表 为没有文本的链接设置样式
斑马纹列表 创建一个背景色交替的条纹列表。 使用 :nth-child(odd) 或 :nth-child(even) 伪类选择器,根据元素在一组兄弟元素中的位置,对匹配的元素应用不同的 background-color。 💡 提示:你可以用它对其他 HTML 元素应用不同的样式,如 <div>、<tr>、<p&g…...

【算法】跳跃游戏II
难度:中等 题目: 给定一个长度为 n 的 0 索引整数数组 nums。初始位置为 nums[0]。 每个元素 nums[i] 表示从索引 i 向前跳转的最大长度。换句话说,如果你在 nums[i] 处,你可以跳转到任意 nums[i j] 处: 0 < j < nums[…...
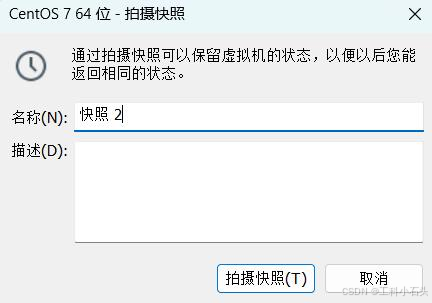
学习大数据DAY20 Linux环境配置与Linux基本指令
目录 Linux 介绍 Linux 发行版 Linux 和 Windows 比较 Linux 就业方向: 下载 CentOS Linux 目录树 Linux 目录结构 作业 1 常用命令分类 文件目录类 作业 2 vim 编辑文件 作业 3 你问我第 19 天去哪了?第 19 天在汇报第一阶段的知识总结,没什…...

达梦+flowable改造
原项目springbootflowablemysql模式现需改造springbootflowable达梦, 1.在项目中引入达梦jpa包 引入高版本包已兼容flowable(6.4.2)liquibase(3.6.2) 我没有像网上做覆盖及达梦配置 <dependency> …...

【乐吾乐2D可视化组态编辑器】消息
消息 乐吾乐2D可视化组态编辑器demo:https://2d.le5le.com/ 监听消息 const fn (event, data) > {}; meta2d.on(event, fn);// 监听全部消息 meta2d.on(*, fn);// 取消监听 meta2d.off(event, fn); meta2d.off(*, fn); Copy 系统消息 event(…...
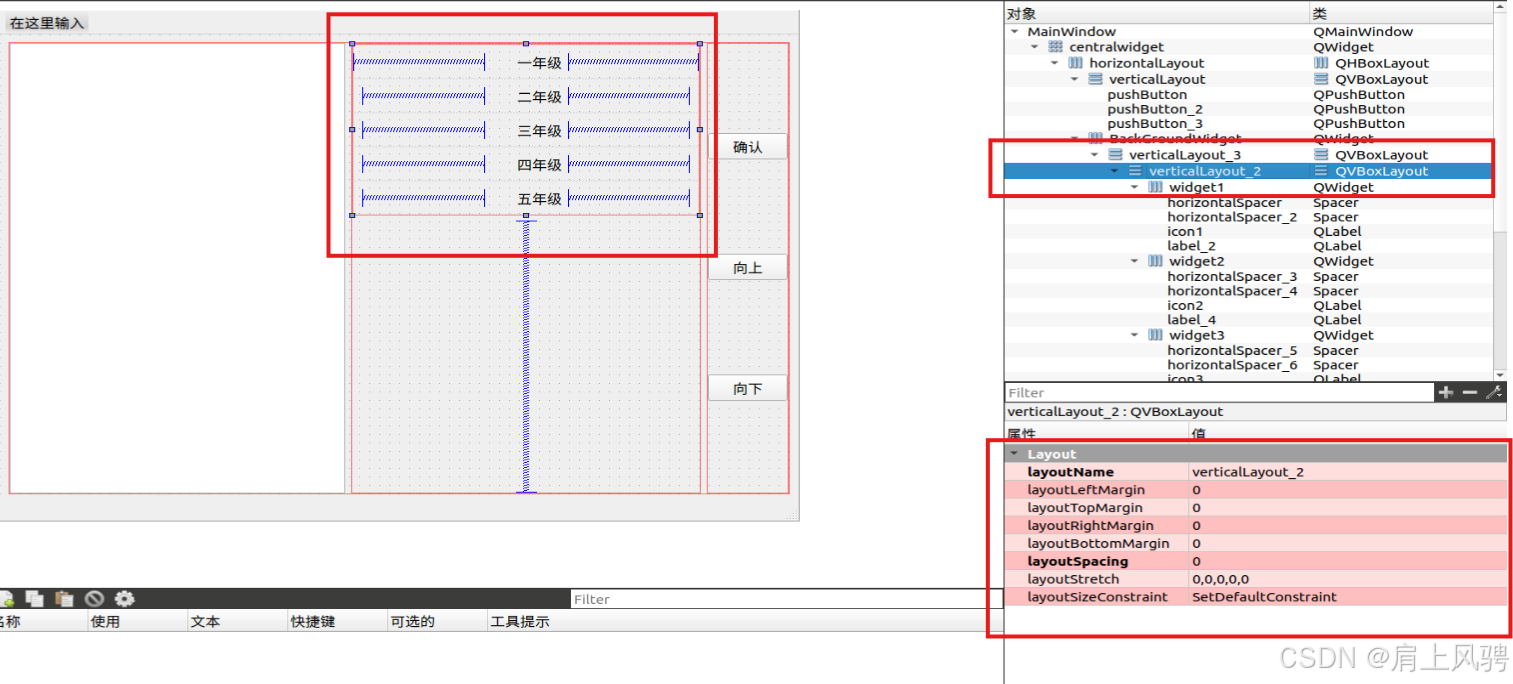
Qt创建列表,通过外部按钮控制列表的选中下移、上移以及左侧图标的显现
引言 项目中需要使用列表QListWidget,但是不能直接拿来使用。需要创建一个列表,通过向上和向下的按钮来向上或者向下移动选中列表项,当当前项背选中再去点击确认按钮,会在列表项的前面出现一个图标。 实现效果 本实例实现的效果如下: 实现思路 思路一 直接采用QLis…...

svn不能记住密码,反复弹出GNOME,自动重置svn.simple文件
1. 修改文件 打开 ~/.subversion/auth/svn.simple/xxx 更新前 K 15 svn:realmstring V 32 xxxxx //svn 地址,库的地址 K 8 username V 4 xxx //用户名 END在顶部插入下面内容, 注意,如果密码不对,则文件文法正常生效 更新后…...
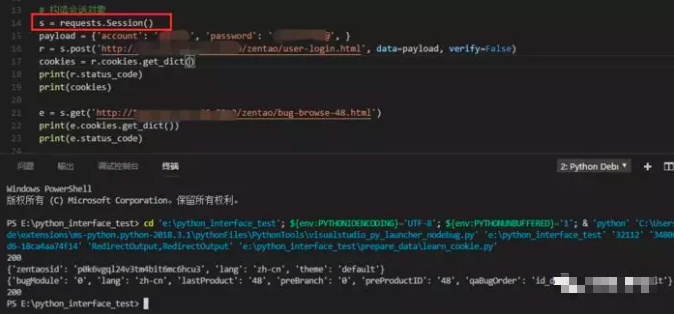
个人网站域名后缀/seo专业培训seo专业培训
Cookie和Session的简单理解 由于Http协议是无状态的,所以产生了cookie和session进行状态的管理。 从哪里来,在哪里,到哪里去: --> Cookie是由服务端生成,存储在响应头中,返回给客户端,客…...
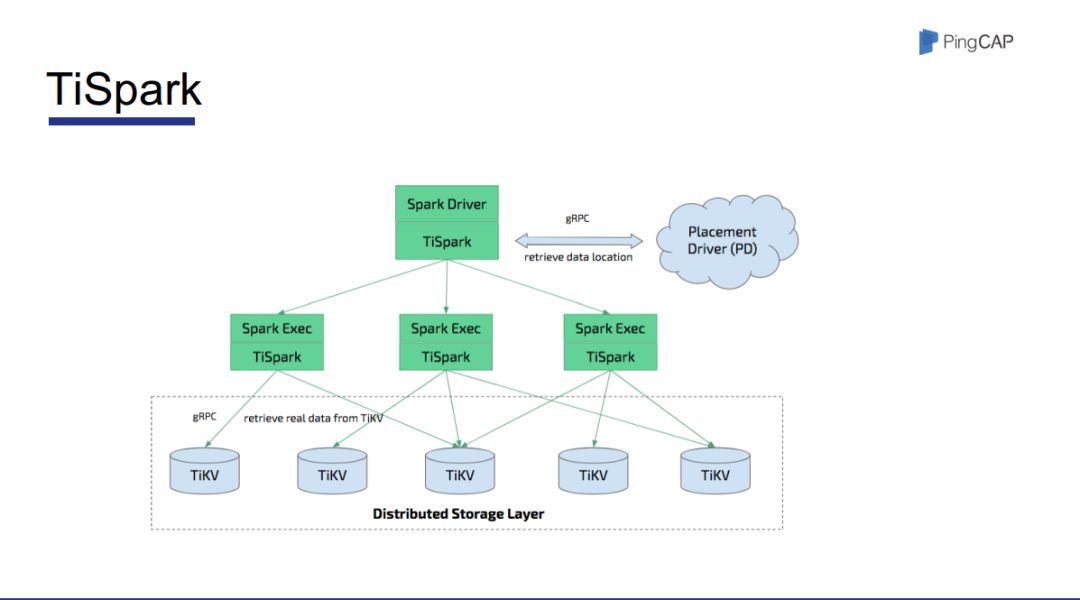
wordpress精选主题/无锡seo网站管理
嘉宾介绍姚维,现PingCAP TiDB内核专家,曾就职于360基础架构部门、UC。为什么我会加入PingCAP呢?在360的时候,我负责Atlas的Sharding(切片技术)的实现。在这个过程中,我发现中间件这个数据库方案存在了诸多限制。比如说…...

做淘宝客的的网站有什么要求吗/seo教学平台
刚开始接触java的时候感觉要学习太多的东西了,而且听别人说很难,就有点畏惧感,可是慢慢的就感觉并没有那么难,和c语言有很多类似的地方,也有很多互通的知识,感觉慢慢去深入的学习java,还是可以一…...
深圳汇网网站建设/搜索引擎哪个最好用
转载“共享博客” 原文 http://www.sharedblog.cn/?post120 容器溢出 语法: overflow: visible | hidden | scroll | auto | inherit; visible: 默认值,容器溢出不裁剪,正常显示; hidden: 溢出部分隐藏不可见; scroll: 当容器没有溢…...

哪些网站可以做养殖的广告/永久免费域名注册
类型化数组是javascript操作(内存)二进制数据的一个接口。类型化数组是建立在ArrayBuffer对象的基础上的。它的作用是,分配一段可以存放数据的连续内存区域。var buf new ArrayBuffer(32); //生成一段32字节的内存区域,即变量buf在内存中占了32字节大小…...

广州seo网站推广优化/今日头条新闻在线看
什么是索引覆盖就是select的数据列只用从索引中就能够取得,不必读取数据行,换句话说查询列要被所建的索引覆盖。那么显然select * from ...是一种拙劣的查询,除非你建立了包含所有列的索引(这样建索引脑子进水)。对 于…...