使用 Python 进行测试(8)纯净测试
原文:Testing with Python (part 8): purity test
总结
如果你要使用综合测试(integrated tests):
def test_add_new_item_to_cart(product, cart):new_product = Product.objects.create(name='New Product', price=15.00)new_cart_item = add_item_to_cart(cart, new_product, 1)assert new_cart_item.cart == cartassert new_cart_item.product == new_productassert new_cart_item.quantity == 1
或者你要隔离地测试,通过mock
摆脱组合爆炸:
def test_add_to_cart_endpoint():product_mock = mock()cart_mock = mock()cart_item_mock = mock(quantity=1)user_mock = mock(cart=cart_mock)request_mock = mock(user=user_mock)(when(get_object_or_404).called_with(Product, id=1).thenReturn(product_mock))(when(add_item_to_cart).called_with(cart_mock, product_mock, 1) .thenReturn(cart_item_mock))response = add_to_cart(request_mock, CartSchema(id=1, quantity=1))assert response == {"id": 1, "quantity": 1}
两者都可以。
第一个选项容易编写和阅读,但时间长了会有很多累赘。
第二个可以构建一个假设所有依赖都已测试且正常运作的信任金字塔。如果你从测试基础开始,通过归纳可知,每个测试都是洁净的、独立的、快速的。但是,你真的确定你在测试这个功能,而不是被你的模拟数据一直返回“是”所欺骗了吗?毕竟,这些测试更难读写。
蓝队还是红队?
红蓝对决
IT领域也有不同的流派,通常有红队和蓝队,例如Mac VS Windows,Python VS Ruby, Vim VS Emacs,…
每个层次都有对立,制表符 VS 空格, 方法 VS函数,是否应该使用 early return,…
对于测试来说, 隔离测试 还是 综合测试(interated test)?
措辞
网上有时将“integration tests”和“integrated tests”混用,这会让人困惑。为了避免歧义,本文约定:
- Integrated test(综合测试):对非隔离代码的测试
- Integration test(集成测试):测试部件是否配合,Integration test 是 Integrated test,但反之不成立。
- e2e test(端到端)
- 持续集成:提交代码后, 打包并安装整个系统并进行测试。
什么隔离测试?隔离测试指测试代码的某一部分时,通过mock将其与依赖项隔离。
API 端点的测试
假设我们有一个使用友好的 django-ninja
库的 HTTP API 端点,用于在购物网站上将产品添加到购物车:
# WARNING: Since the project doesn't actually exist, I write
# this code and the associated tests without running it, so read
# it to get the general idea, don't take it as exactly what
# you should writeimport loggingfrom django.shortcuts import get_object_or_404
from django.db import transaction
from .models import CartSchema, CartItem, Product
from .endpoints import apilog = logging.getLogger(__name__)@api.post("/cart")
def add_to_cart(request, payload: CartSchema):product = get_object_or_404(Product, id=payload.product_id)# Add an item to the cart if it doesn't exist, or update # the quantity if it does. We do that with locked rows in # a transaction to deal with the potential race condition.with transaction.atomic():locked_qs = CartItem.objects.select_for_update()cart_item = locked_qs.filter(cart=request.user.cart, product=product).first()if cart_item:cart_item.quantity += payload.quantitycart_item.save()else:cart_item = CartItem.objects.create(cart=request.user.cart,product=product,quantity=payload.quantity)log.info('Product %s added to user %s cart',product.id, request.user.id)return {"id": product_id, "quantity": cart_item.quantity}
将使用 Django 测试客户端进行综合测试。由于它将访问测试数据库,因此我们需要fixture:
import pytest
from django.contrib.auth.models import User
from ninja.testing import TestClient
from .models import Product, CartItem, Cart
from .endpoints import api@pytest.fixture
def user(db): # db fixture assumed from pytest-djangouser = User.objects.create_user(username='testuser', password='testpass')Cart.objects.create(user=user)return user@pytest.fixture
def client(user):return TestClient(api)@pytest.fixture
def auth_client(client, user):client.authenticate(user)return client@pytest.fixture
def product(db):return Product.objects.create(name='Test Product', price=10.0)@pytest.fixture
def cart_item(db, user, product):return CartItem.objects.create(cart=user.cart, product=product, quantity=1)
然后我们需要测试:
- 认证
- 不存在的产品 ID 返回 404。
- 创建了一个不存在的商品购物车。
- 任何现有的商品购物车数量都会增加。
- API 返回格式符合我们的期望。
API端点的集成测试
def test_user_not_authenticated(product, client):payload = {'product_id': product.id, 'quantity': 1}response = client.post('/cart', json=payload)assert response.status_code == 401def test_non_existing_product_raises_404(auth_client):payload = {'product_id': 9999, 'quantity': 1}response = auth_client.post('/cart', json=payload)assert response.status_code == 404def test_cart_item_quantity_incremented(auth_client, product, cart_item):payload = {'product_id': product.id, 'quantity': 1}response = auth_client.post('/cart', json=payload)assert response.status_code == 200cart_item.refresh_from_db()assert cart_item.quantity == 2def test_cart_item_created_if_not_exist(auth_client, product):payload = {'product_id': product.id, 'quantity': 1}response = auth_client.post('/cart', json=payload)cart_item = CartItem.objects.get(cart=user.cart, product=product)assert response.status_code == 200assert cart_item.quantity == 1assert response.json() == {"id": product.id, "quantity": 1}# You may want to write more tests, like if your schema rejects
# properly badly formatted requests, or to check what happens with
# congestions on locked rows but this is enough to make our point.
它们很好,可以测试功能,并且还能测试函数之间的相互配合。
另一方面:
- 由于它需要处理测试数据库,所以速度较慢。
- 每个测试都依赖于整体。如果一个部分出问题,整个测试套件就会崩溃。
- 改变代码意味着改变所有这些测试,系统的所有部分彼此依赖。
- 测试的内容并不是一眼就能看出来的,因为存在大量干扰。
我喜欢这些测试,因为它们便宜且相对容易实施。测试通常是一次性的,特别是在项目早期阶段,代码库经常变动的时候。但如果我们不想使用一次性测试呢?如果我们想要一个强大、快速且能够证明整个系统现在以及未来几年都是健全的测试套件怎么办?孤立测试可以帮助解决这个问题。
同样的端点,但是隔离测试
隔离测试推动设计,因为要创建良好的隔离,你需要清晰、明确的部分,实际上你可以隔离这些部分。在我们的例子中,如果我们稍作停顿,我们可以看到购物车项的创建可以移到一个单独的函数中:
def add_item_to_cart(cart, product, quantity):"""Create a cart item, or update the quantity an existing one.We do that with locked rows in a transaction to deal with the potential race condition."""with transaction.atomic():locked_qs = CartItem.objects.select_for_update()cart_item = locked_qs.filter(cart=cart, product=product).first()if cart_item:cart_item.quantity += quantitycart_item.save()return cart_itemreturn CartItem.objects.create(cart=request.user.cart,product=product,quantity=quantity)@api.post("/cart")
def add_to_cart(request, payload: CartSchema):product = get_object_or_404(Product, id=product_id)cart_item = add_item_to_cart(request.user.cart, product, quantity)log.info('Product %s added to user %s cart', product_id, request.user.id)return {"id": product_id, "quantity": cart_item.quantity}
add_item_to_cart
成为我们可以模拟的对象,并且隔离数据库的副作用。它还具有增强关注点分离的良好特性,代码的每个部分都更加清晰。
我们可以联合测试改写后的add_item_to_cart
,这也变得更加简洁、清晰了:
@pytest.fixture
def cart(db):user = User.objects.create_user(username='testuser', password='testpass')return Cart.objects.create(user=user)@pytest.fixture
def product(db):return Product.objects.create(name='Test Product', price=10.00)def test_add_existing_item_to_cart(cart, product, cart_item):cart_item = CartItem.objects.create(cart=cart, product=product, quantity=1)updated_cart_item = add_item_to_cart(cart, product, 2)cart_item.refresh_from_db()assert cart_item.quantity == 3assert updated_cart_item == cart_itemdef test_add_new_item_to_cart(product, cart):new_product = Product.objects.create(name='New Product', price=15.00)new_cart_item = add_item_to_cart(cart, new_product, 1)assert new_cart_item.cart == cartassert new_cart_item.product == new_productassert new_cart_item.quantity == 1
然后我们转向测试端点。这就是有趣的地方。因为我们在隔离环境中进行测试,所以我们不需要测试所有可能性的笛卡尔积,我们只需要检查它是否以正确的参数调用了正确的内容。
import pytest
from mockito import when, mock
from django.shortcuts import get_object_or_404
from .views import add_to_cart
from .models import Product, CartItem, User
from .models import CartSchema, CartItem, Productdef test_add_to_cart_endpoint():product_mock = mock()cart_mock = mock()cart_item_mock = mock(quantity=1)user_mock = mock(cart=cart_mock)request_mock = mock(user=user_mock)(when(get_object_or_404).called_with(Product, id=1).thenReturn(product_mock))(when(add_item_to_cart).called_with(cart_mock, product_mock, 1) .thenReturn(cart_item_mock))response = add_to_cart(request_mock, CartSchema(id=1, quantity=1))assert response == {"id": 1, "quantity": 1}
确实,我们不需要测试如果产品不存在,端点是否返回 404 状态码。 get_object_or_404
已经由 Django 测试套件测试过,所以我们可以假设它能正常工作。我们只需要检查我们如何使用它。同样,既然我们已经测试过add_item_to_cart
,我们就不需要检查项目是否已经正确添加。我们可以假设它有效,只需检查我们是否使用它即可。这意味着测试端点是一个单一的测试。它也独立于购物车管理、路由、身份验证或请求/响应序列化的细节。
由于 django-ninja 允许您转储 API 的模式,我们还可以通过一次性检查整个 API 签名来提高我们的弹性
import json
import pytest
from django.urls import reverse
from ninja.testing import TestClient
from .endpoints import api
from pathlib import Pathclient = TestClient(api)
schema_file = Path('api_schema.json')# This is naive and will fail even if you just add an enpoint,
# but you get the idea
def test_api_schema(get_api_schema):if not schema_file.exists():# This command doesn't really exist, but you can code it.pytest.fail(f"API schema missing. Run ./manage.py dump_schema.")current_schema = client.get(reverse("api:schema")).json()saved_schema = json.loads(schema_file.read_text())if current_schema != saved_schema:pytest.fail("API schema has changed. Follow stability policy.")
所以,红还是蓝?
光谱就是光谱,当然你可以把光标放在任何你喜欢的地方。我写这篇文章假装有两种做法,但我们都知道我们可以走得更极端,或者在中间,混合两者。我知道我知道,我用“这要看情况”来逃避做出明确结论。欢迎来到现实生活,事情很糟糕,绝对真理不存在。我在等待那一天,当我们发现物理定律在整个宇宙中并不是均匀的时候。那将会很有趣。
所以:
- 这是集成测试示例
- 这是隔离测试示例
你更喜欢哪一个?
集成测试目前较短,但这种情况不会持续下去。一旦我们添加更多功能,可能性图将增加,测试数量要么激增,要么忽略边缘情况。
But they are much easier to read because they are a collection of basic concepts.
但它们更容易阅读,因为它们是基本概念的集合。
On the other hand, the isolated tests are more correct: they are actually testing the business logic directly, and not just what the API returns. They can also already warn us when we break our public API in any way, and forever. Since only a fraction touches the DB, they are going to be fast, meaning we have a quick feedback loop.
另一方面,孤立测试更加准确:它们实际上直接测试业务逻辑,而不仅仅是 API 返回的内容。它们还可以在我们以任何方式破坏公共 API 时发出警告,而且是永久的。由于只有一小部分接触数据库,它们将会很快,这意味着我们有一个快速的反馈循环。
But the mock magic makes you stop. Do you really know what happens in there? Is it saving your butt, or are you testing your mocks instead of your code base?
但是模拟魔术让你停下来。你真的知道里面发生了什么吗?它是在帮你摆脱困境,还是你在测试模拟而不是代码库?
Plus, isolated tests are harder to write, require more experience, and more abstract thinking. They are less code in the long run but will take longer to bootstrap. You can’t delegate them to ChatGPT (or an intern), it will get them completely wrong.
另外,隔离测试更难编写,需要更丰富的经验和更抽象的思维。从长远来看,它们包含的代码更少,但需要更长的启动时间。你不能将它们委托给 ChatGPT(或实习生),否则它会完全错误地执行。
Then again, by nature, you won’t get the combinatorial explosions of all the possible things to test mixing with each other. Your code base will get exponentially more robust and more tested. You won’t lie to yourself about what correctness you cover. As much.
再说一遍,从本质上讲,你不会得到所有可能要测试的事物的组合爆炸混合在一起。你的代码库将变得指数级更加健壮和经过更多测试。你不会对自己所覆盖的正确性撒谎。就这样。
This builds trust incrementally since each test can assume the underlying functions are tested, recursively until you reach the ones actually performing the side effects. You know the ground you cover, and you can rely on this coverage progression.
这样逐步建立信任,因为每个测试都可以假设底层函数已经被测试,递归地进行,直到达到实际执行副作用的函数。你知道你所覆盖的范围,可以依赖这种覆盖进展。
However, and I’m going to repeat myself, I rarely go full isolated testing.
不过,我要重复一遍,我很少进行完全隔离的测试。
Most projects are just not that critical. For the typical app, change rates are going to be so fast that having dirty but disposable tests is more important than proving the system is very reliable. Bugs in production will happen, and the boss is likely ok with it although he won’t admit it.
大多数项目并非如此关键。对于典型的应用程序来说,变化速度会非常快,因此拥有脏但是可丢弃的测试比证明系统非常可靠更重要。生产中的错误是不可避免的,老板可能会接受,尽管他不会承认。
Or rather, his bank account is.
或者说,他的银行账户是。
Because the hard truth is very reliable software is super expensive. It takes time, it takes skill, it takes care. Plus, the typical users are quite tolerant of bugs but want features, yet probably don’t want to pay much for them.
因为残酷的事实是非常可靠的软件非常昂贵。它需要时间,需要技术,需要关怀。此外,典型的用户对错误有相当高的容忍度,但他们希望有更多功能,然而可能不愿为此支付太多费用。
If you ever wondered why software is so buggy in general, and the advertising industry so powerful, there it is.
如果你曾经 wondered 为什么软件普遍如此 buggy,广告行业如此强大,这就是原因所在。
Since this is a zero-sum game, spending resources on one thing means you don’t spend it on another. Design is expensive. Ergonomics testing is expensive, marketing is expensive.
由于这是一个零和游戏,将资源花费在一件事情上意味着你不会在另一件事情上花费。设计是昂贵的。人体工程学测试是昂贵的,市场营销也是昂贵的。
If you ever wondered why a super robust FOSS app is very ugly or hard to use, there it is.
如果你
On top of that, unlike in many industries, the consequences of user-facing issues are not as high as in the typical nerd mind. Most companies pay little price for a security breach or messages delivered to the wrong user. But don’t tweet the wrong thing, you could lose your head!
If you ever wondered why a bug has not been addressed for years, but the icon pack has been updated 3 times to reflect the current trend, there it is.
如果你曾经想过为什么一个漏洞多年来都没有得到解决,但图标包已经更新了 3 次以反映当前的趋势,那就是原因。
Anyway, a few projects reach a huge user count and have very few bugs at the same time.
无论如何,一些项目能够同时达到巨大的用户数量并且很少出现 bug。
相关文章:

使用 Python 进行测试(8)纯净测试
原文:Testing with Python (part 8): purity test 总结 如果你要使用综合测试(integrated tests): def test_add_new_item_to_cart(product, cart):new_product Product.objects.create(nameNew Product, price15.00)new_cart…...
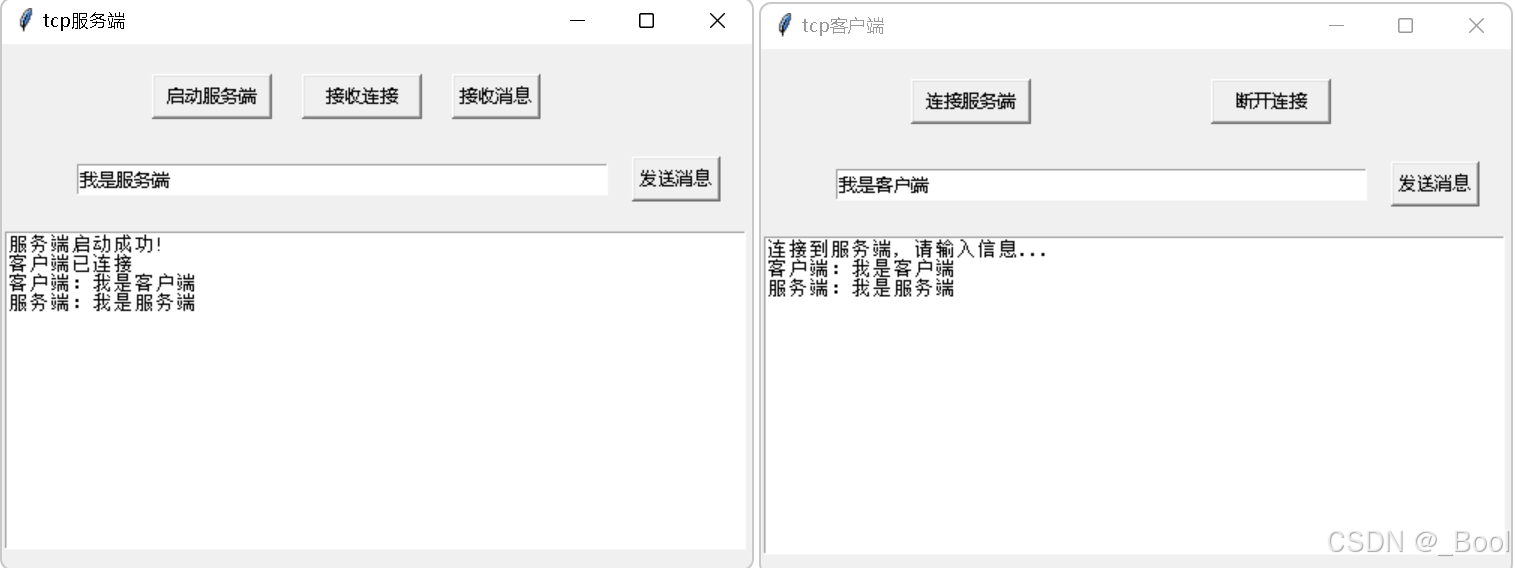
python的tkinter、socket库开发tcp的客户端和服务端
一、tcp通讯流程和开发步骤 1、tcp客户端和服务端通讯流程图 套接字是通讯的利器,连接时要经过三次握手建立连接,断开连接要经过四次挥手断开连接。 2、客户端开发流程 1)创建客户端套接字 2)和服务端器端套接字建立连接 3&#x…...

Python面试题:Python中的异步编程:详细讲解asyncio库的使用
Python 的异步编程是实现高效并发处理的一种方法,它使得程序能够在等待 I/O 操作时继续执行其他任务。在 Python 中,asyncio 库是实现异步编程的主要工具。asyncio 提供了一种机制来编写可以在单线程内并发执行的代码,适用于 I/O 密集型任务。…...
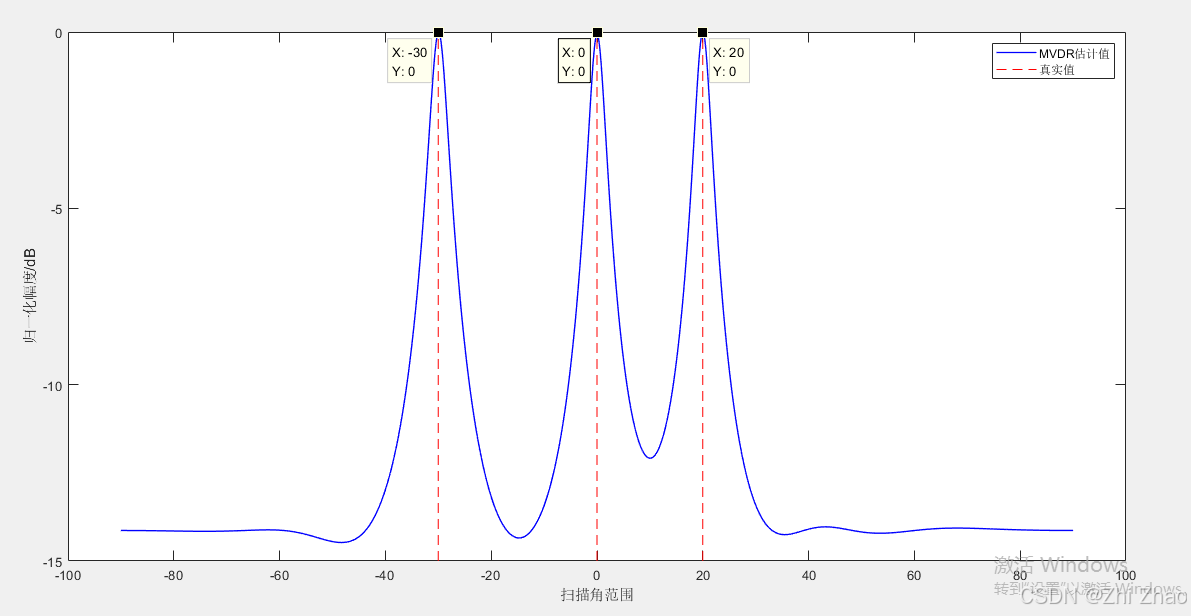
【信号频率估计】MVDR算法及MATLAB仿真
目录 一、MVDR算法1.1 简介1.2 原理1.3 特点1.3.1 优点1.3.2 缺点 二、算法应用实例2.1 信号的频率估计2.2 MATLAB仿真代码 三、参考文献 一、MVDR算法 1.1 简介 最小方差无失真响应(Mininum Variance Distortionless Response,MVDR)算法最…...
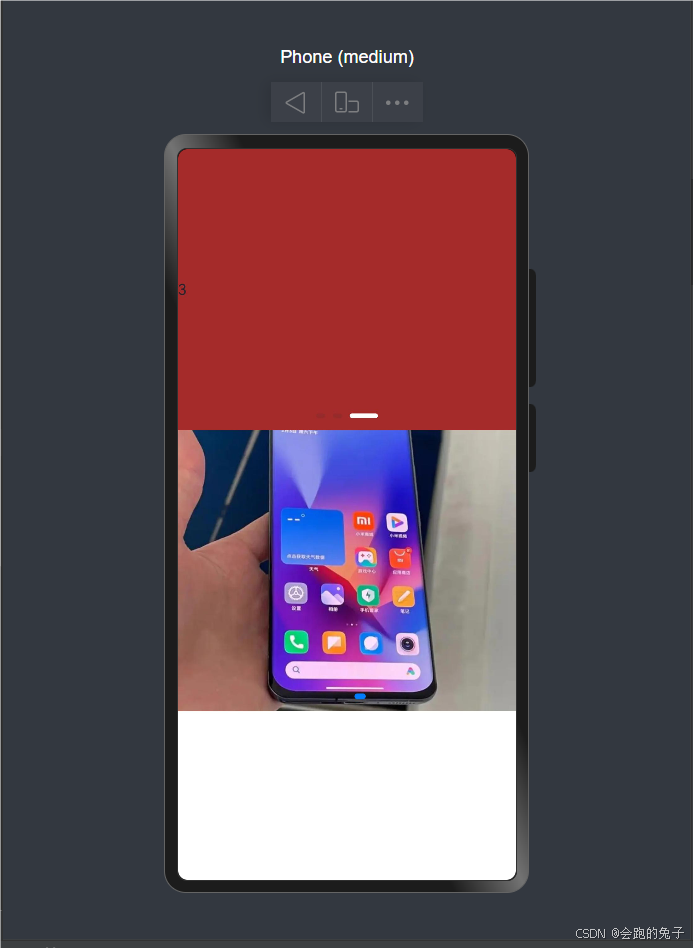
HarmonyOS NEXT零基础入门到实战-第二部分
HarmonyOS NEXT零基础入门到实战-第二部分 Swiper 轮播组件 Swiper是一个 容器 组件,当设置了多个子组件后,可以对这些 子组件 进行轮播显示。(文字、图片...) 1、Swiper基本语法 2、Swiper常见属性 3、Swiper样式自定义 4、案例&…...
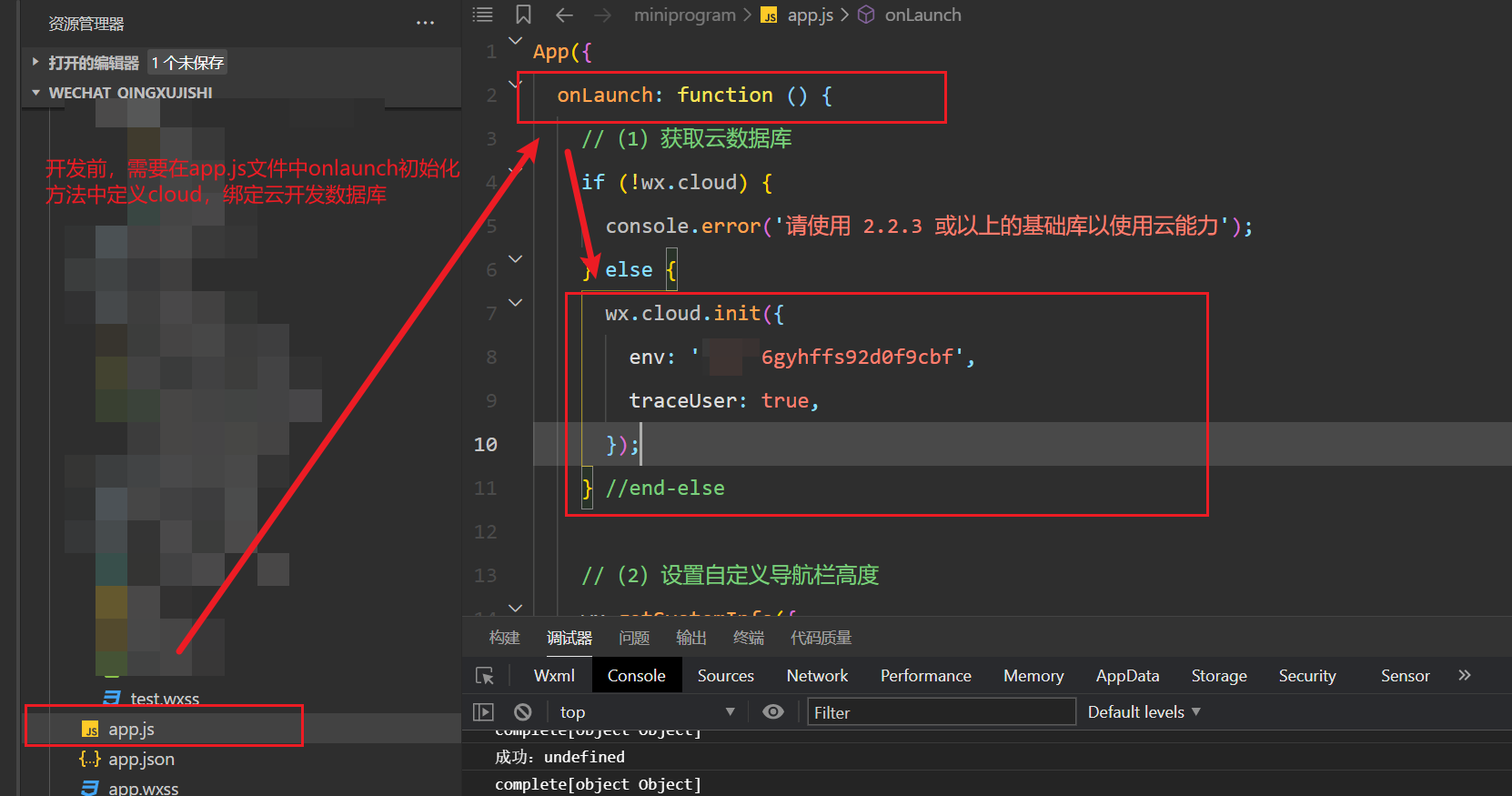
《小程序02:云开发之增删改查》
一、前置操作 // 一定要用这个符号包含里面的${}才会生效 wx.showToast({title: 获取数据成功:${colorLista}, })1.1:初始化介绍 **1、获取数据库引用:**在开始使用数据库 API 进行增删改查操作之前,需要先获取数据库的引用 cons…...
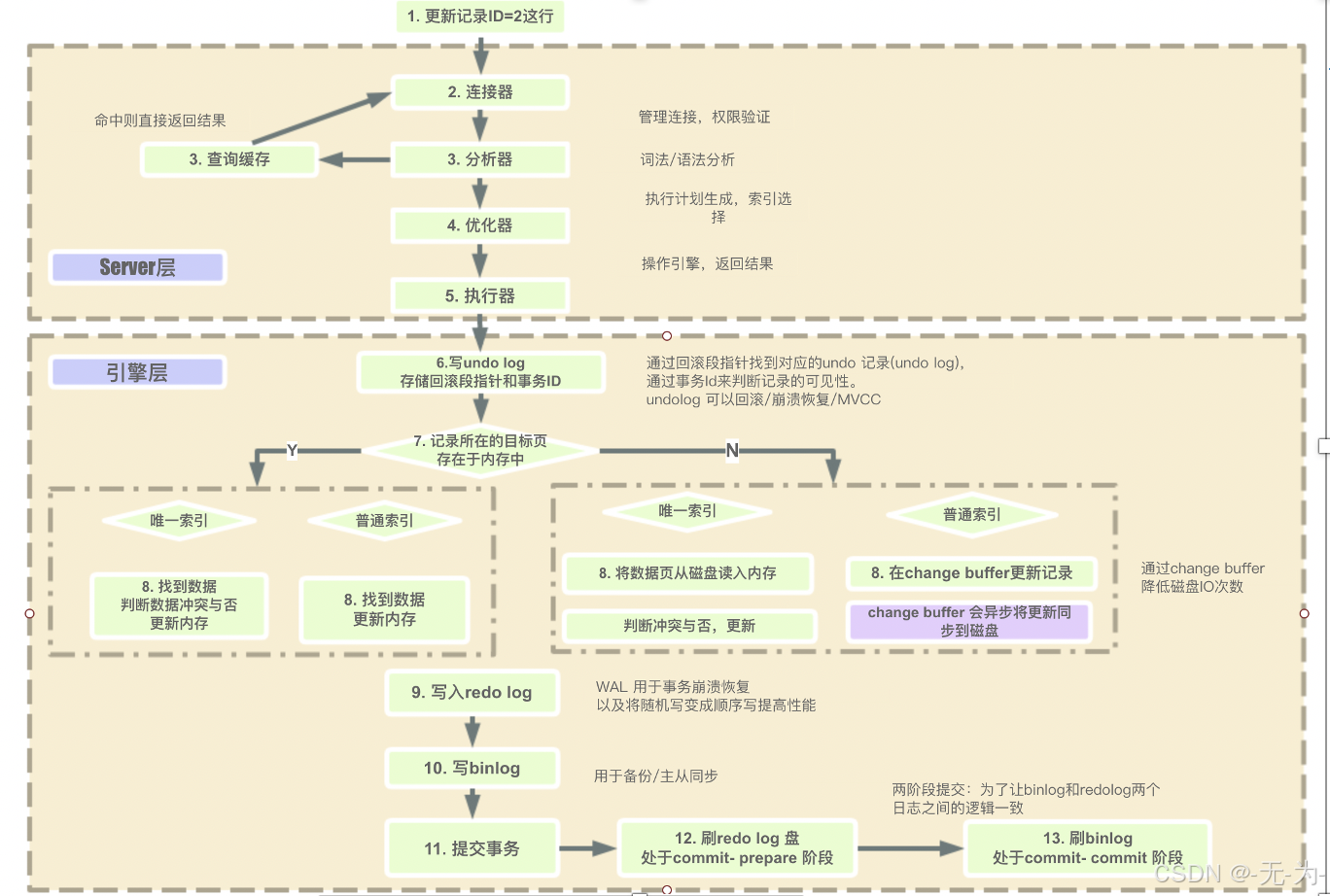
SQL执行流程、SQL执行计划、SQL优化
select查询语句 select查询语句中join连接是如何工作的? 1、INNER JOIN 返回两个表中的匹配行。 2、LEFT JOIN 返回左表中的所有记录以及右表中的匹配记录。 3、RIGHT JOIN 返回右表中的所有记录以及左表中的匹配记录。 4、FULL OUTER JOIN 返回左侧或右侧表中有匹…...

【前端】JavaScript入门及实战41-45
文章目录 41 嵌套的for循环42 for循环嵌套练习(1)43 for循环嵌套练习(2)44 break和continue45 质数练习补充 41 嵌套的for循环 <!DOCTYPE html> <html> <head> <title></title> <meta charset "utf-8"> <script type"…...
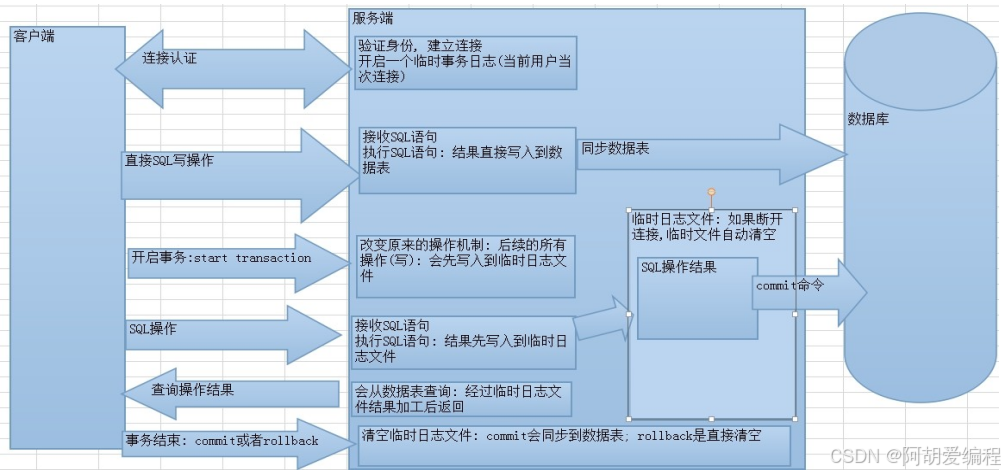
更加深入Mysql-04-MySQL 多表查询与事务的操作
文章目录 多表查询内连接隐式内连接显示内连接 外连接左外连接右外连接 子查询 事务事务隔离级别 多表查询 有时我们不仅需要一个表的数据,数据可能关联到俩个表或者三个表,这时我们就要进行夺标查询了。 数据准备: 创建一个部门表并且插入…...

基于最新版的flutter pointycastle: ^3.9.1的AES加密
基于最新版的flutter pointycastle: ^3.9.1的AES加密 自己添加pointycastle: ^3.9.1库config.dartaes_encrypt.dart 自己添加pointycastle: ^3.9.1库 config.dart import dart:convert; import dart:typed_data;class Config {static String password 成都推理计算科技; // …...

K8S内存资源配置
在 Kubernetes (k8s) 中,资源请求和限制用于管理容器的 CPU 和内存资源。配置 CPU 和内存资源时,使用特定的单位来表示资源的数量。 CPU 资源配置 CPU 单位:Kubernetes 中的 CPU 资源以 “核” (cores) 为单位。1 CPU 核心等于 1 vCPU/Core…...
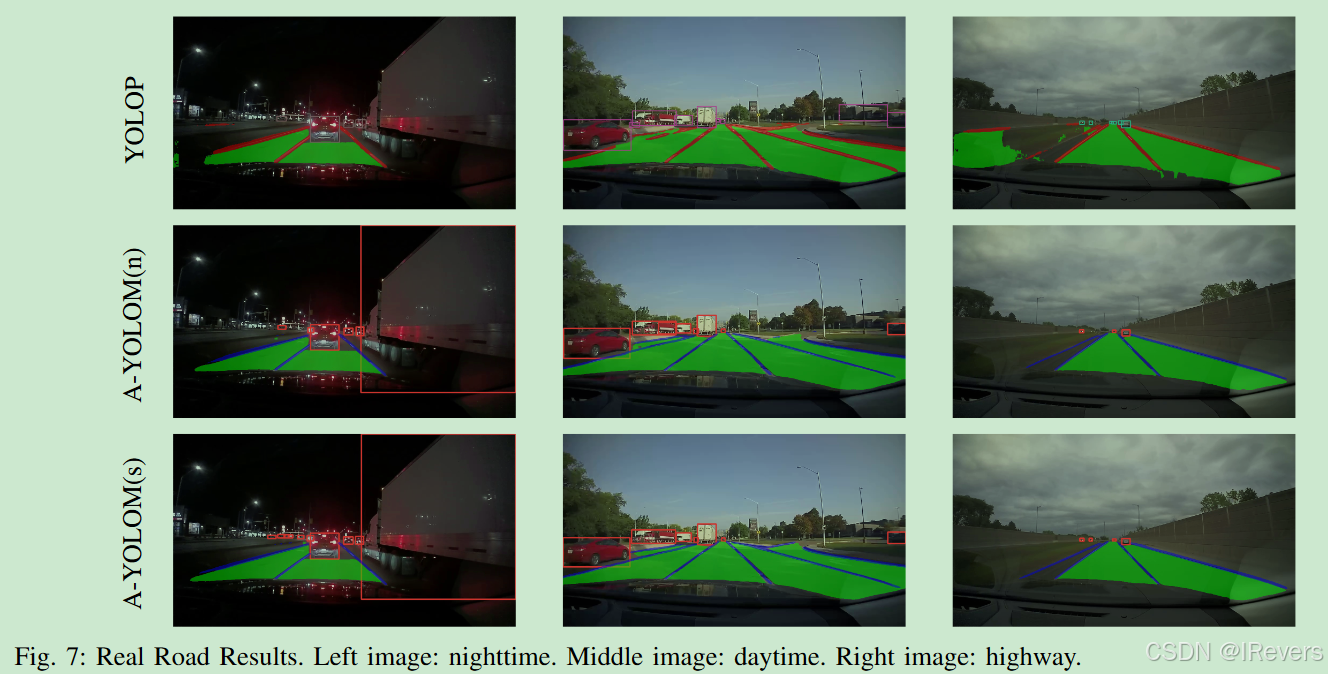
【多任务YOLO】 A-YOLOM: You Only Look at Once for Real-Time and Generic Multi-Task
You Only Look at Once for Real-Time and Generic Multi-Task 论文链接:http://arxiv.org/abs/2310.01641 代码链接:https://github.com/JiayuanWang-JW/YOLOv8-multi-task 一、摘要 高精度、轻量级和实时响应性是实现自动驾驶的三个基本要求。本研究…...

数学建模--灰色关联分析法
目录 简介 基本原理 应用场景 优缺点 优点: 缺点: 延伸 灰色关联分析法在水质评价中的具体应用案例是什么? 如何克服灰色关联分析法在主观性强时的数据处理和改进方法? 灰色关联分析法与其他系统分析方法(如A…...
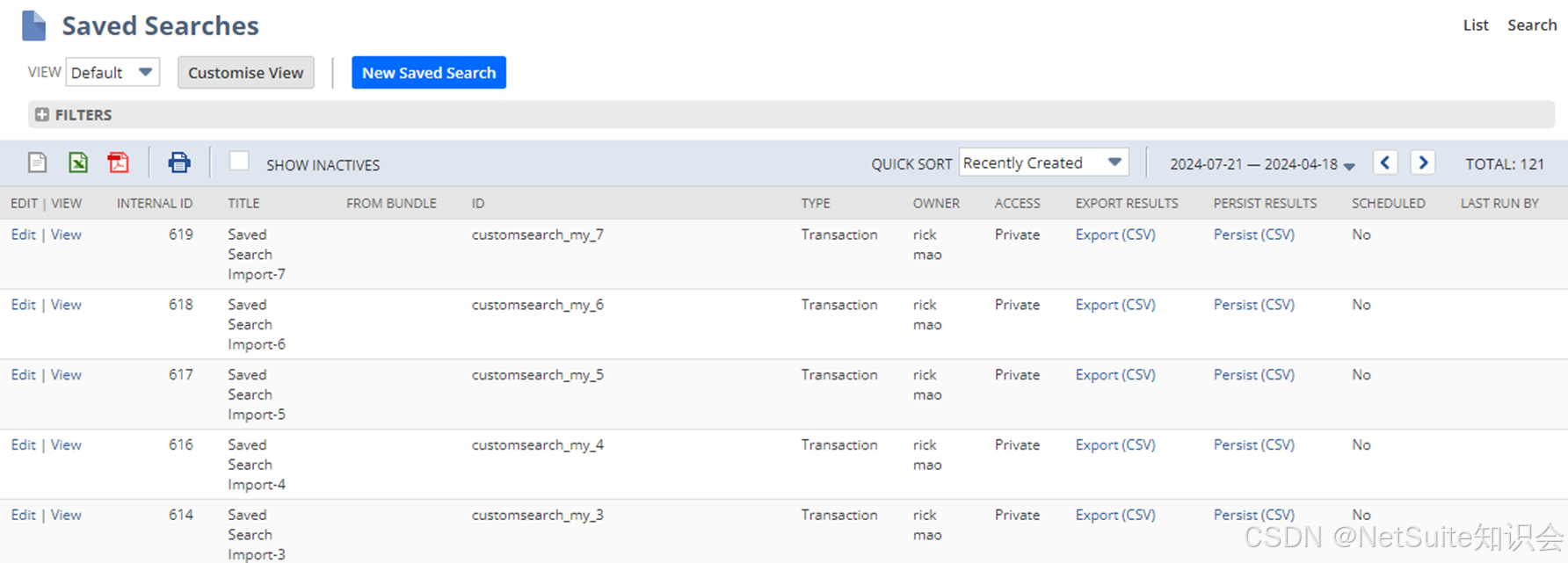
NetSuite Saved Search迁移工具
我们需要在系统间迁移Saved Search,但是采用Copy To Account或者Bundle时,会有一些Translation不能迁移,或者很多莫名其妙的Dependency,导致迁移失败。因此,我们想另辟蹊径,借助代码完成Saved Search的迁移…...
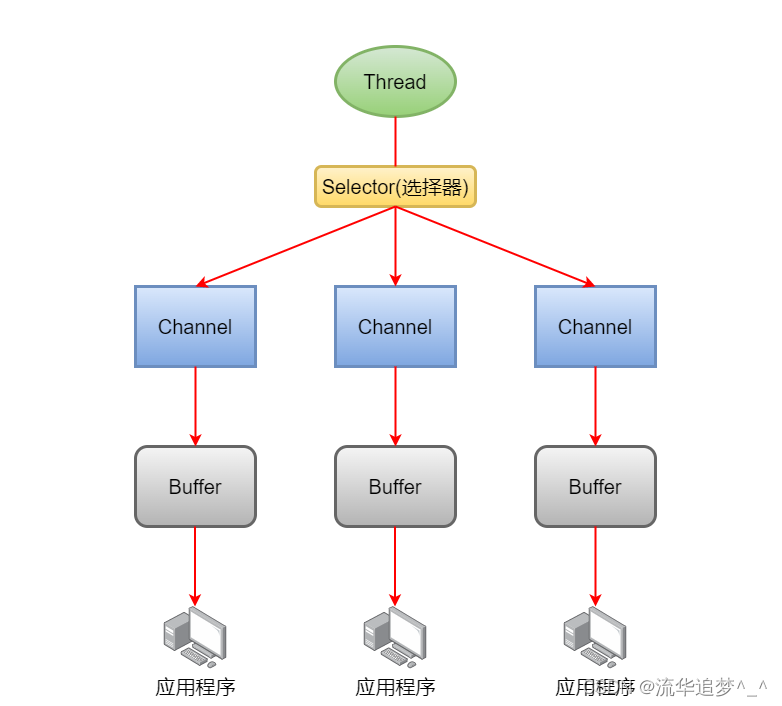
Java IO模型深入解析:BIO、NIO与AIO
Java IO模型深入解析:BIO、NIO与AIO 一. 前言 在Java编程中,IO(Input/Output)操作是不可或缺的一部分,它涉及到文件读写、网络通信等方面。Java提供了多种类和API来支持这些操作。本文将从IO的基础知识讲起ÿ…...
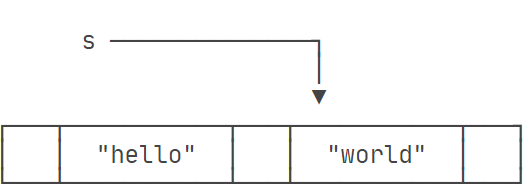
《从C/C++到Java入门指南》- 9.字符和字符串
字符和字符串 字符类型 Java 中一个字符保存一个Unicode字符,所以一个中文和一个英文字母都占用两个字节。 // 计算1 .. 100 public class Hello {public static void main(String[] args) {char a A;char b 中;System.out.println(a);System.out.println(b)…...
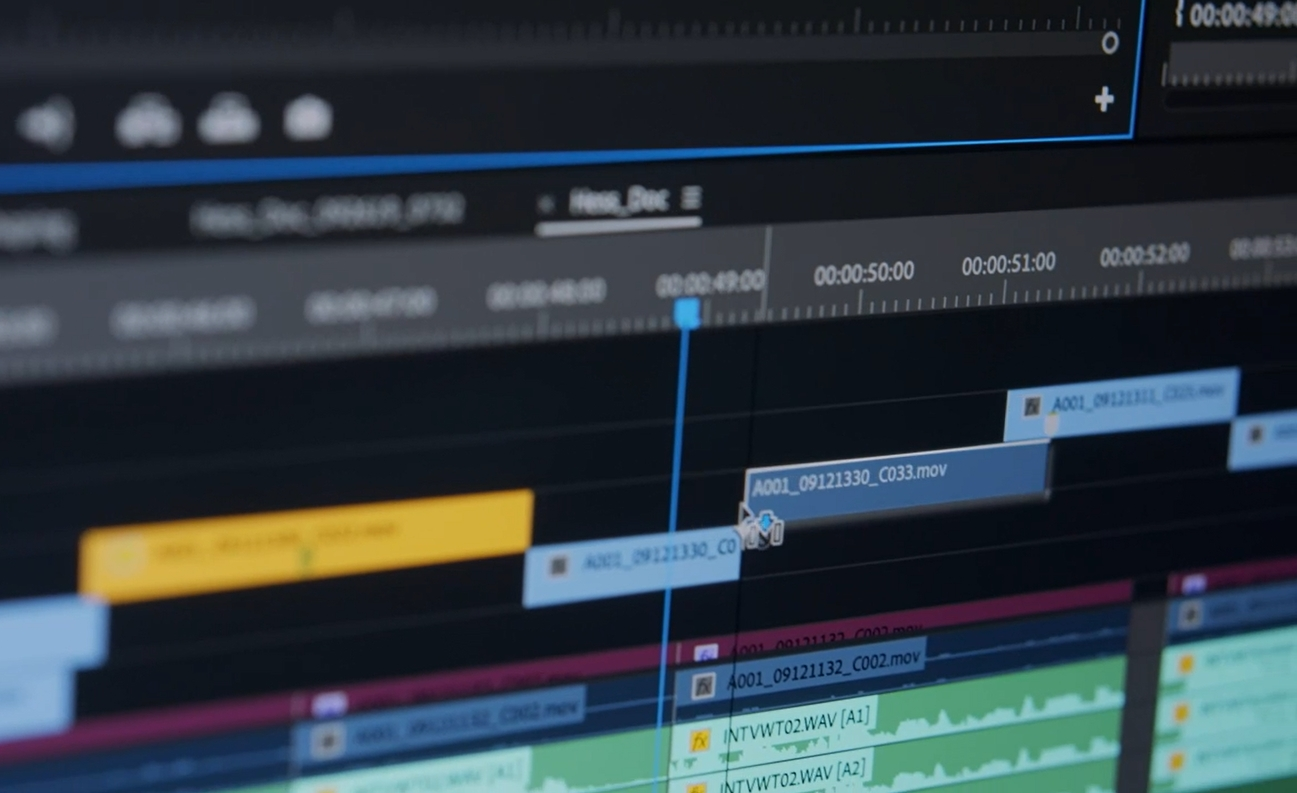
Adobe国际认证详解-视频剪辑
在数字化时代,视频剪辑已成为创意表达和视觉传播的重要手段。随着技术的不断进步,熟练掌握视频剪辑技能的专业人才需求日益增长。在这个背景下,Adobe国际认证应运而生,成为全球创意设计领域的重要标杆。 Adobe国际认证是由Adobe公…...

昇思25天学习打卡营第19天|MindNLP ChatGLM-6B StreamChat
文章目录 昇思MindSpore应用实践ChatGML-6B简介基于MindNLP的ChatGLM-6B StreamChat Reference 昇思MindSpore应用实践 本系列文章主要用于记录昇思25天学习打卡营的学习心得。 ChatGML-6B简介 ChatGLM-6B 是由清华大学和智谱AI联合研发的产品,是一个开源的、支持…...

.NET在游戏开发中有哪些成功的案例?
简述 在游戏开发的多彩世界中,技术的选择往往决定了作品的成败。.NET技术,以其跨平台的性能和强大的开发生态,逐渐成为游戏开发者的新宠。本文将带您探索那些利用.NET技术打造出的著名游戏案例,领略.NET在游戏开发中的卓越表现。 …...

搜维尔科技:我们用xsens完成了一系列高难度的运动项目并且捕获动作
我们用xsens完成了一系列高难度的运动项目并且捕获动作 搜维尔科技:我们用xsens完成了一系列高难度的运动项目并且捕获动作...

深入探讨:Node.js、Vue、SSH服务与SSH免密登录
在这篇博客中,我们将深入探讨如何在项目中使用Node.js和Vue,并配置SSH服务以及实现SSH免密登录。我们会一步步地进行讲解,并提供代码示例,确保你能轻松上手。 一、Node.js 与 Vue 的结合 1.1 Node.js 简介 Node.js 是一个基于 …...
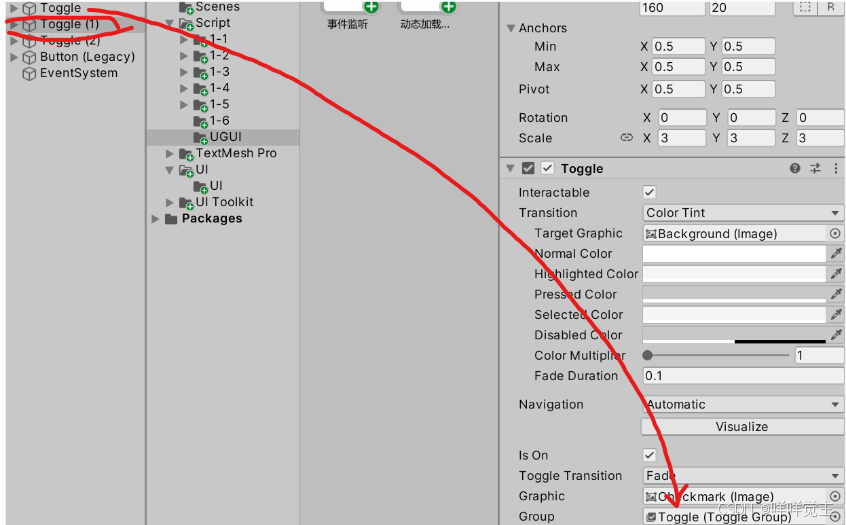
Unity UGUI 之 Toggle
本文仅作学习笔记与交流,不作任何商业用途本文包括但不限于unity官方手册,唐老狮,麦扣教程知识,引用会标记,如有不足还请斧正 1.什么是Toggle? Unity - Manual: Toggle 带复选框的开关,可…...

Git报错:error: fsmonitor--daemon failed to start处理方法
问题描述 git用了很久了,但是后面突然发现执行命令时,后面都会出现这个报错,虽然该报错好像不会影响正常的命令逻辑,但是还是感觉有天烦人,就去找了找资料。 $ git status error: fsmonitor--daemon failed to start…...
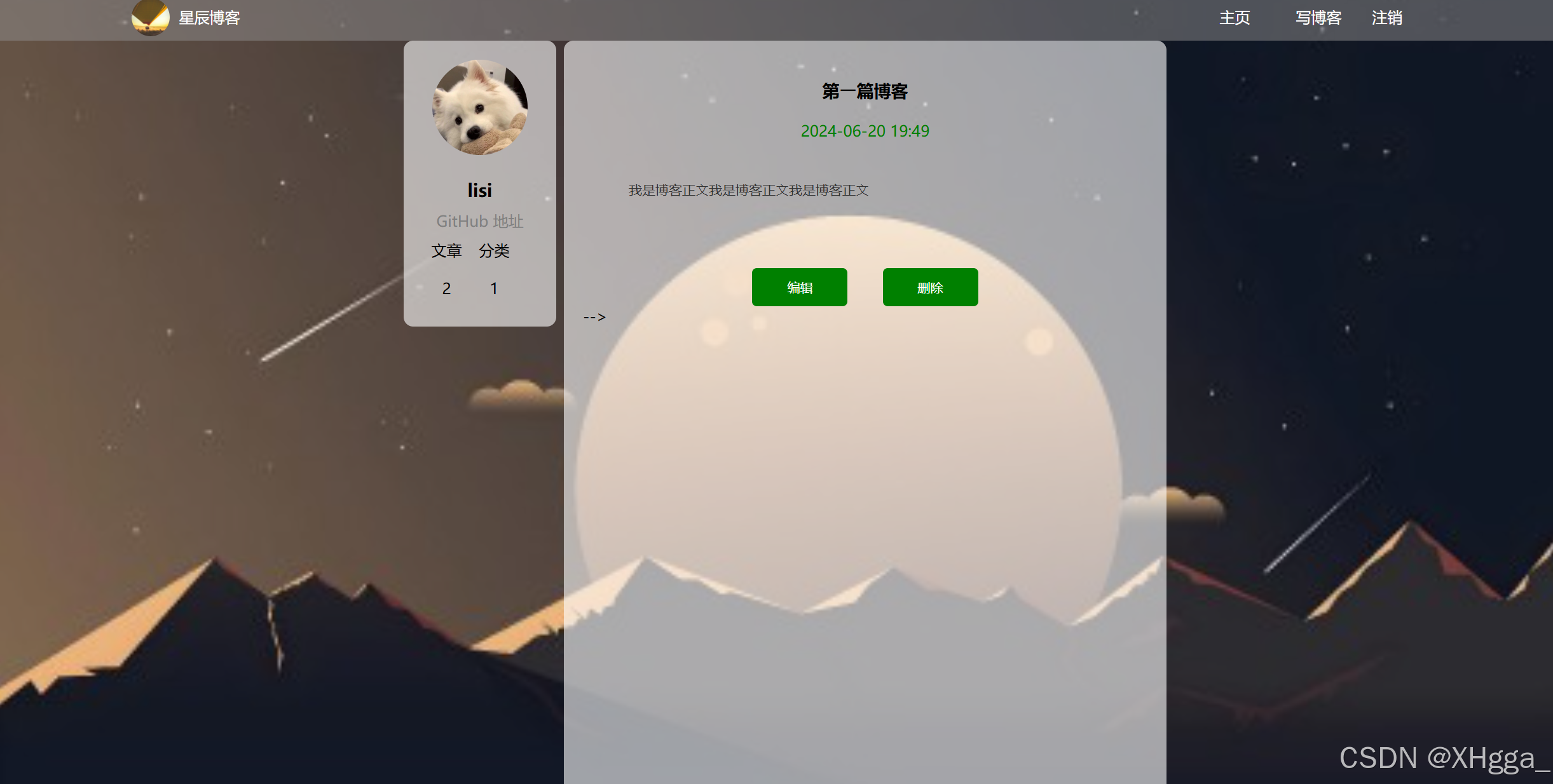
【项目】星辰博客介绍
目录 一、项目背景 二、项目功能 1. 登录功能: 2. 列表页面: 3. 详情页面: 4. 写博客: 三、技术实现 四、功能页面展示 1. 用户登录 2. 博客列表页 3. 博客编辑更新页 4.博客发表页 5. 博客详情页 五.系统亮点 1.强…...
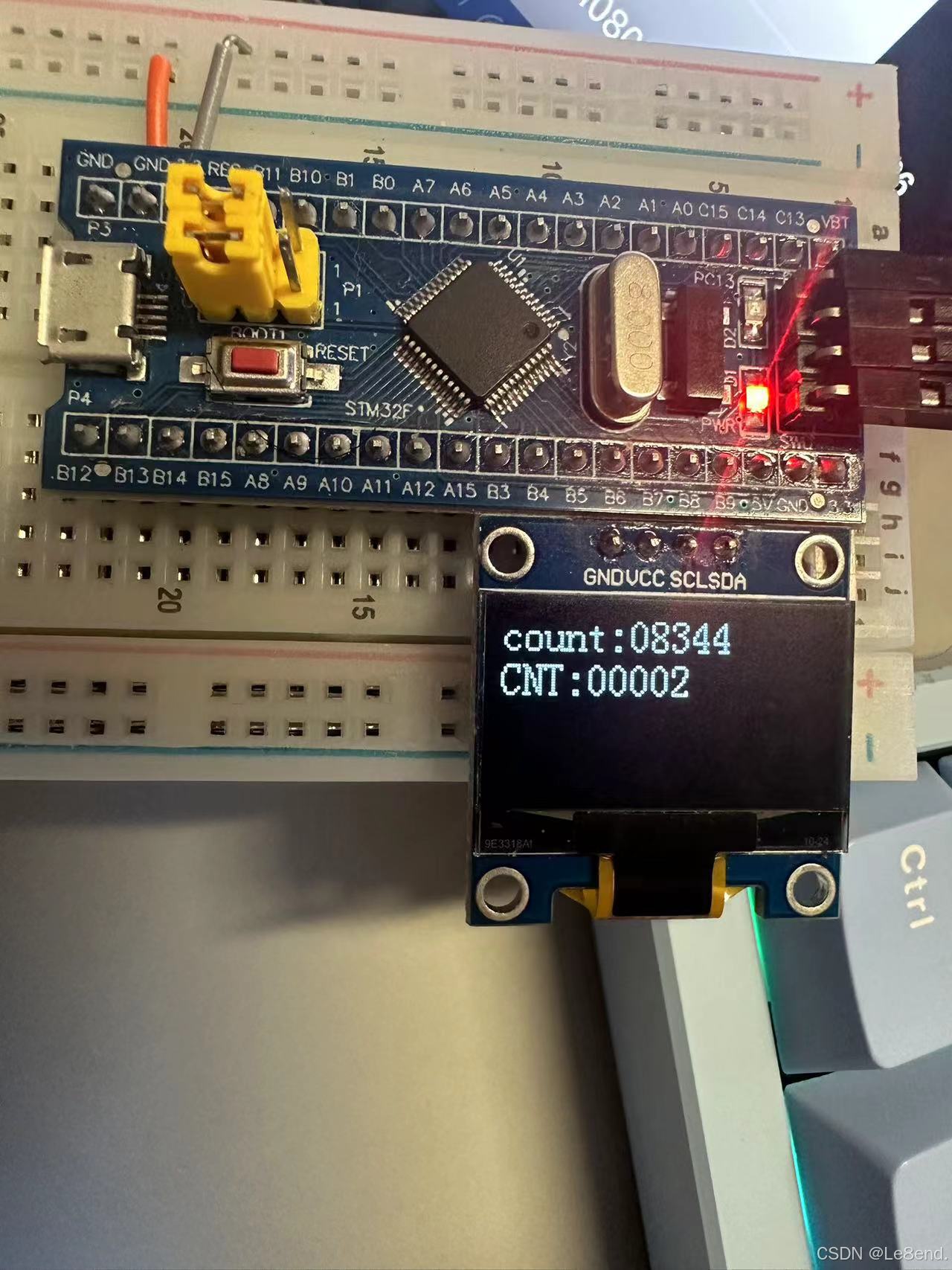
从0开始的STM32HAL库学习6
外部时钟源选择 配置环境 选择TIM2 配置红色框图中的各种配置 时钟源选择外部时钟 2 1. 预分频器 Prescaler ,下面填0,不分频 2. 计数模式 CounterModer ,计数模式选择为向上计数 3. 自动重装寄存器 CouterPeriod ,自动重…...

Elasticsearch ILM 热节点迁移至冷节点 IO 打满、影响读写解决方案探讨
1、实战问题 ILM(索引生命周期管理) 遇到热数据迁移至冷节点时造成 IO 打满影响读写的情况。 现在采取的方案是调整索引生命周期策略,定时的将Cold phase 开启/关闭。低峰开启,高峰关闭。 就是不知道这里面会有啥坑。 热节点&…...

STM32中PC13引脚可以当做普通引脚使用吗?如何配置STM32的TAMPER?
1.STM32中PC13引脚可以当做普通引脚使用吗? 在STM32单片机中,PC13引脚可以作为普通IO使用,但需要进行一定的配置。PC13通常与RTC侵入检测功能(TAMPER)复用,因此需要关闭TAMPER功能才能将其作为普通IO使用。…...

k8s学习——创建测试镜像
创建一个安装了ifconfig、telnet、curl、nc、traceroute、ping、nslookup等网络工具的镜像,便于集群中的测试。 创建一个Dockerfile文件 # 使用代理下载 Ubuntu 镜像作为基础 FROM docker.m.daocloud.io/library/ubuntu:latest# 设置环境变量 DEBIAN_FRONTEND 为 …...
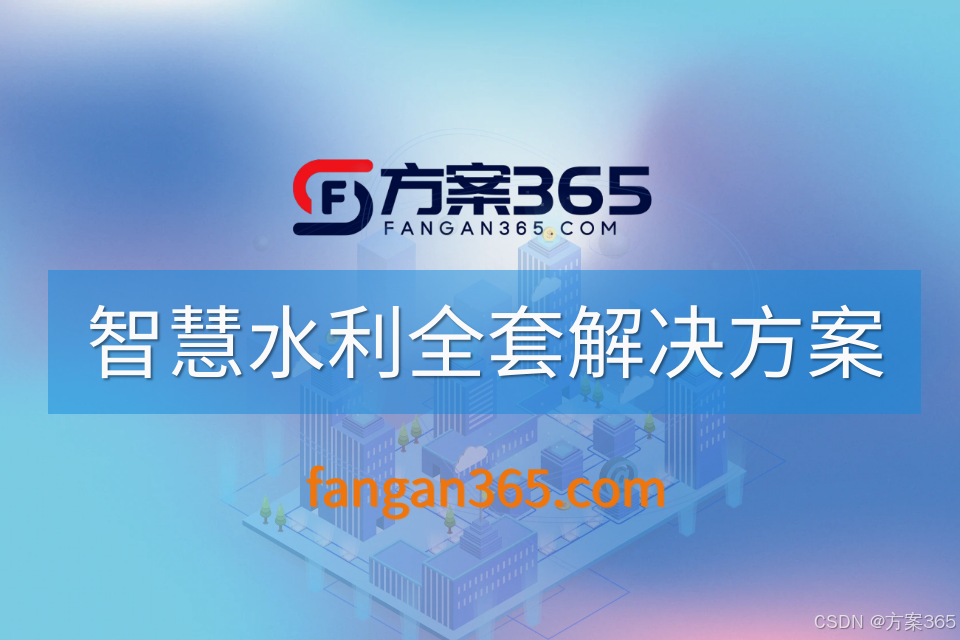
重塑水资源管理的新篇章:深度剖析智慧水利解决方案的前沿技术与应用,探索其如何推动水利行业向智能化、高效化、可持续化方向迈进
目录 一、引言 二、智慧水利的核心技术 1、物联网技术 2、大数据与云计算 3、人工智能与机器学习 4、数字孪生技术 三、智慧水利的应用实践 1、智慧河湖长制信息平台 2、智能灌溉系统 3、城市防洪排涝智慧管理系统 4、智慧水库建设 四、智慧水利的推动作用 1、提升…...

C#实现数据采集系统-查询报文处理和响应报文分析处理
发送报文处理 增加一个功能码映射关系 //功能码映射关系public readonly Dictionary<string, byte> ReadFuncCodes = new Dictionary<string, byte>();<...

专门做卫生间效果图的网站/游戏推广渠道
最近开始研究Python的并行开发技术,包括多线程,多进程,协程等。逐步整理了网上的一些资料,今天整理一下greenlet相关的资料。并发处理的技术背景并行化处理目前很受重视, 因为在很多时候,并行计算能大大的提…...
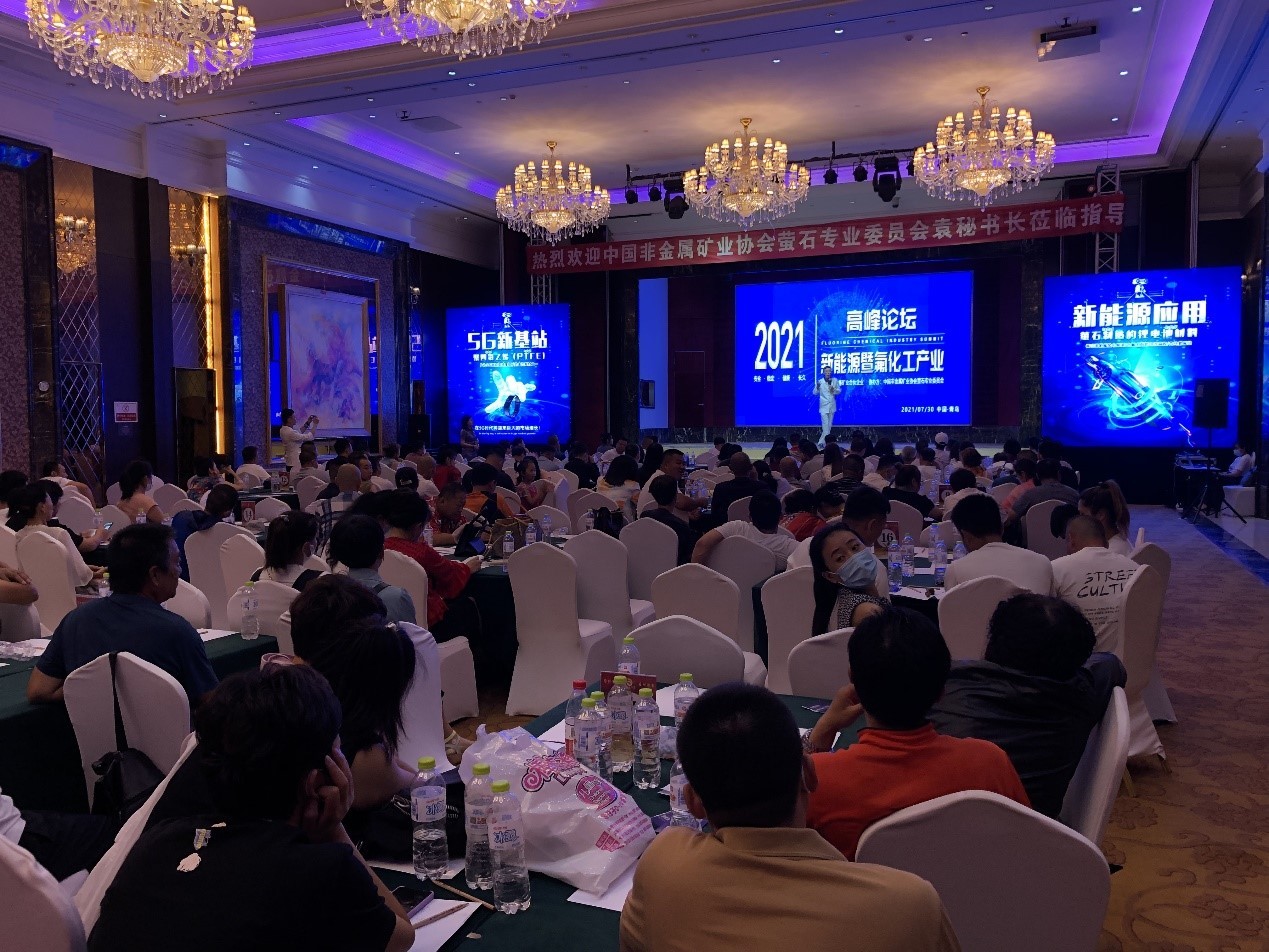
如何在交易网站做电子印章/杭州网站建设技术支持
发展新能源,落实新能源产业升级,整合能源结构调整,近日成为国家经济形势会议的一大热点。会议指出并要求需要挖掘国内市场潜力,支持新能源汽车加快发展。众昂矿业集团积极响应国家政策号召,落实绿色经济新能源产业落地…...

网络网站制作/免费网站seo
早晨起床时间:7:00 晚上休息时间:23:02 今日总结:今天主要在做一些测距之类的事。。...

网站如何做点击链接地址/网络推广网站排行榜
我正在尝试从电子表格中读取日期列和时间列.我可以从工作表中退出日期列,但不能退出时间列.例如,我的工作表将包含以下形式的行:约会时间11/2/2012 12:15:01我有以下代码来获取日期列:while(cellIterator.hasNext()) {HSSFCell cell (HSSFCell)cellIter…...

云南微网站搭建费用/百度竞价推广是什么工作
参考网址: https://www.jianshu.com/p/2a4a39e3704f转载于:https://www.cnblogs.com/maohuidong/p/10487729.html...

公共场所建设网站/南宁求介绍seo软件
服务器端进行还是在客户端进行,再也不必考虑那么多了,程序员们可以将重要精力放在主程序的设计上了。ASP.NET公有六种验证控件,分别如下:控件名 功能描叙 RequiredFieldValidator(必须字段验证) …...