Spring @Profile
1. Overview
In this tutorial, we’ll focus on introducing Profiles in Spring.
Profiles are a core feature of the framework — allowing us to map our beans to different profiles — for example, dev
, test
, and prod
.
We can then activate different profiles in different environments to bootstrap only the beans we need.
2. Use @Profile on a Bean
Let’s start simple and look at how we can make a bean belong to a particular profile. We use the @Profile annotation — we are mapping the bean to that particular profile
; the annotation simply takes the names of one (or multiple) profiles.
Consider a basic scenario: We have a bean that should only be active during development but not deployed in production.
We annotate that bean with a dev profile, and it will only be present in the container during development. In production, the dev simply won’t be active:
@Component
@Profile("dev")
public class DevDatasourceConfig
As a quick sidenote, profile names can also be prefixed with a NOT operator, e.g., !dev, to exclude them from a profile.
In the example, the component is activated only if dev profile is not active:
@Component
@Profile("!dev")
public class DevDatasourceConfig
3. Declare Profiles in XML
Profiles can also be configured in XML. The tag has a profile attribute, which takes comma-separated values of the applicable profiles:
<beans profile="dev"><bean id="devDatasourceConfig" class="org.baeldung.profiles.DevDatasourceConfig" />
</beans>
4. Set Profiles
The next step is to activate and set the profiles so that the respective beans are registered in the container.
This can be done in a variety of ways, which we’ll explore in the following sections.
4.1. Programmatically via WebApplicationInitializer Interface
In web applications, WebApplicationInitializer can be used to configure the ServletContext programmatically.
It’s also a very handy location to set our active profiles programmatically:
@Configuration
public class MyWebApplicationInitializer implements WebApplicationInitializer {@Overridepublic void onStartup(ServletContext servletContext) throws ServletException {servletContext.setInitParameter("spring.profiles.active", "dev");}
}
4.2. Programmatically via ConfigurableEnvironment
We can also set profiles directly on the environment:
@Autowired
private ConfigurableEnvironment env;
...
env.setActiveProfiles("someProfile");
4.3. Context Parameter in web.xml
Similarly, we can define the active profiles in the web.xml file of the web application, using a context parameter:
<context-param><param-name>contextConfigLocation</param-name><param-value>/WEB-INF/app-config.xml</param-value>
</context-param>
<context-param><param-name>spring.profiles.active</param-name><param-value>dev</param-value>
</context-param>
4.4. JVM System Parameter
The profile names can also be passed in via a JVM system parameter. These profiles will be activated during application startup:
-Dspring.profiles.active=dev
4.5. Environment Variable
In a Unix environment, profiles can also be activated via the environment variable
:
export spring_profiles_active=dev
4.6. Maven Profile
Spring profiles can also be activated via Maven profiles, by specifying the spring.profiles.active configuration property
.
In every Maven profile, we can set a spring.profiles.active property
:
<profiles><profile><id>dev</id><activation><activeByDefault>true</activeByDefault></activation><properties><spring.profiles.active>dev</spring.profiles.active></properties></profile><profile><id>prod</id><properties><spring.profiles.active>prod</spring.profiles.active></properties></profile>
</profiles>
Its value will be used to replace the @spring.profiles.active@ placeholder in application.properties
:
spring.profiles.active=@spring.profiles.active@
Now we need to enable resource filtering in pom.xml
:
<build><resources><resource><directory>src/main/resources</directory><filtering>true</filtering></resource></resources>...
</build>
and append a -P parameter to switch which Maven profile will be applied:
mvn clean package -Pprod
This command will package the application for prod profile. It also applies the spring.profiles.active
value prod for this application when it is running.
4.7. @ActiveProfile in Tests
Tests make it very easy to specify what profiles are active using the @ActiveProfile annotation to enable specific profiles:
@ActiveProfiles("dev")
So far, we’ve looked at multiple ways of activating profiles. Let’s now see which one has priority over the other and what happens if we use more than one, from highest to lowest priority:
- Context parameter in web.xml
- WebApplicationInitializer
- JVM System parameter
- Environment variable
- Maven profile
5. The Default Profile
Any bean that does not specify a profile belongs to the default profile.
Spring also provides a way to set the default profile when no other profile is active — by using the spring.profiles.default
property.
6. Get Active Profiles
Spring’s active profiles drive the behavior of the @Profile annotation for enabling/disabling beans. However, we may also wish to access the list of active profiles programmatically.
We have two ways to do it, using Environment or spring.profiles.active
.
6.1. Using Environment
We can access the active profiles from the Environment object by injecting it:
public class ProfileManager {@Autowiredprivate Environment environment;public void getActiveProfiles() {for (String profileName : environment.getActiveProfiles()) {System.out.println("Currently active profile - " + profileName);} }
}
6.2. Using spring.profiles.active
Alternatively, we could access the profiles by injecting the property spring.profiles.active:
@Value("${spring.profiles.active}")
private String activeProfile;
参考:
Spring Profiles
相关文章:

Spring @Profile
1. Overview In this tutorial, we’ll focus on introducing Profiles in Spring. Profiles are a core feature of the framework — allowing us to map our beans to different profiles — for example, dev, test, and prod. We can then activate different profiles…...

Vue3电商项目实战-个人中心模块4【09-订单管理-列表渲染、10-订单管理-条件查询】
文章目录09-订单管理-列表渲染10-订单管理-条件查询09-订单管理-列表渲染 目的:完成订单列表默认渲染。 大致步骤: 定义API接口函数抽取单条订单组件获取数据进行渲染 落的代码: 1.获取订单列表API借口 /*** 查询订单列表* param {Number…...
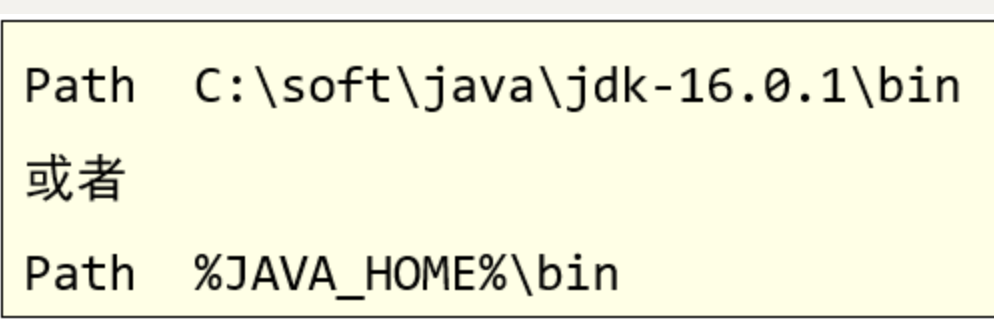
【十二天学java】day01-Java基础语法
day01 - Java基础语法 1. 人机交互 1.1 什么是cmd? 就是在windows操作系统中,利用命令行的方式去操作计算机。 我们可以利用cmd命令去操作计算机,比如:打开文件,打开文件夹,创建文件夹等。 1.2 如何打…...
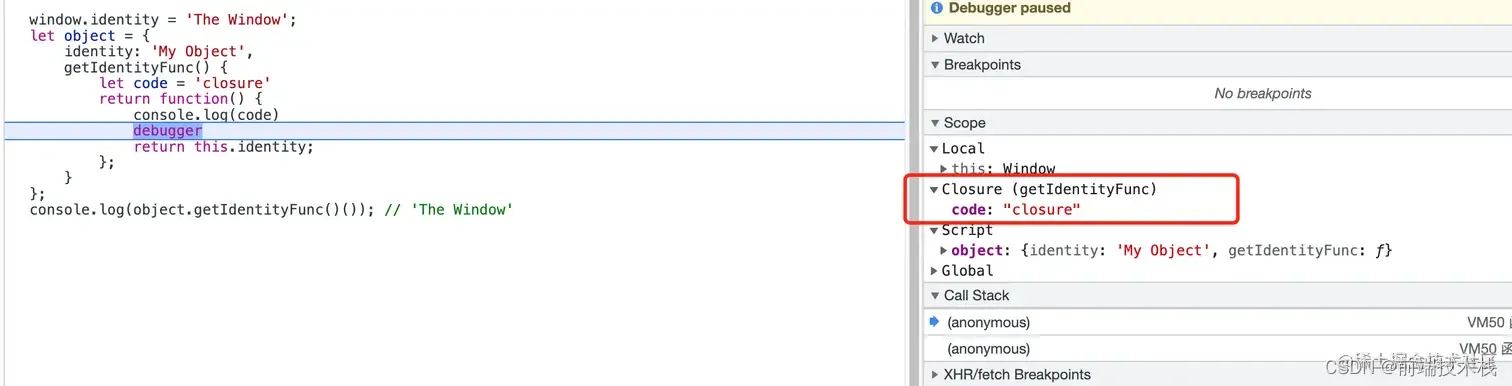
【面试题】闭包是什么?this 到底指向谁?
一通百通,其实函数执行上下文、作用域链、闭包、this、箭头函数是相互关联的,他们的特性并不是孤立的,而是相通的。因为内部函数可以访问外层函数的变量,所以才有了闭包的现象。箭头函数内没有 this 和 arguments,所以…...
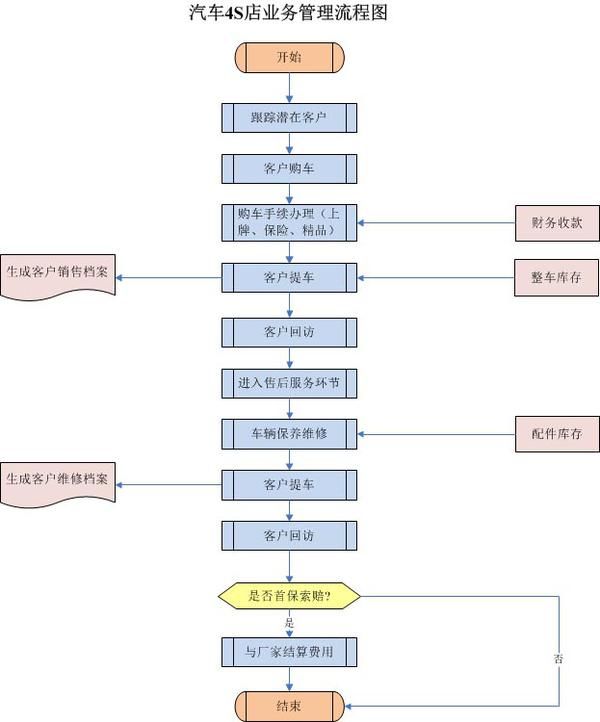
汽车4S店业务管理软件
一、产品简介 它主要提供给汽车4S商店,用于管理各种业务,如汽车销售、售后服务、配件、精品和保险。整个系统以客户为中心,以财务为基础,覆盖4S商店的每一个业务环节,不仅可以提高服务效率和客户满意度,…...
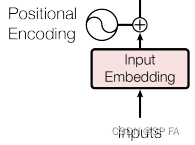
基于 pytorch 的手写 transformer + tokenizer
先放出 transformer 的整体结构图,以便复习,接下来就一个模块一个模块的实现它。 1. Embedding Embedding 部分主要由两部分组成,即 Input Embedding 和 Positional Encoding,位置编码记录了每一个词出现的位置。通过加入位置编码可以提高模型的准确率,因为同一个词出现在…...
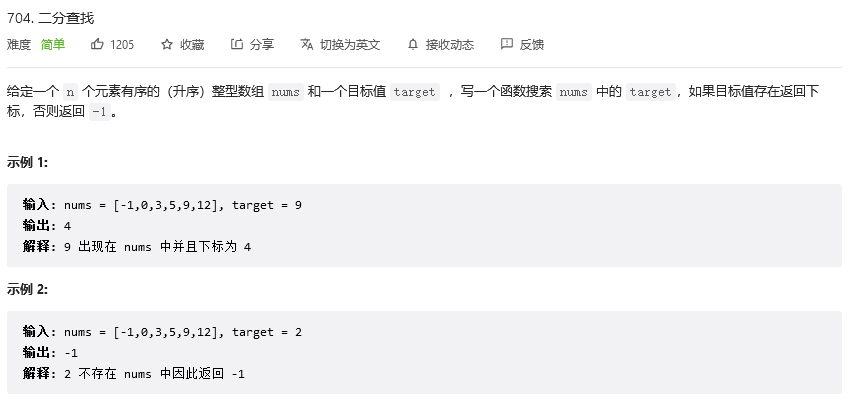
算法小抄6-二分查找
二分查找,又名折半查找,其搜索过程如下: 从数组中间的元素开始,如果元素刚好是要查找的元素,则搜索过程结束如果搜索元素大于或小于中间元素,则排除掉不符合条件的那一半元素,在剩下的数组中进行查找由于每次需要排除掉一半不符合要求的元素,这需要数组是已经排好序的或者是有…...
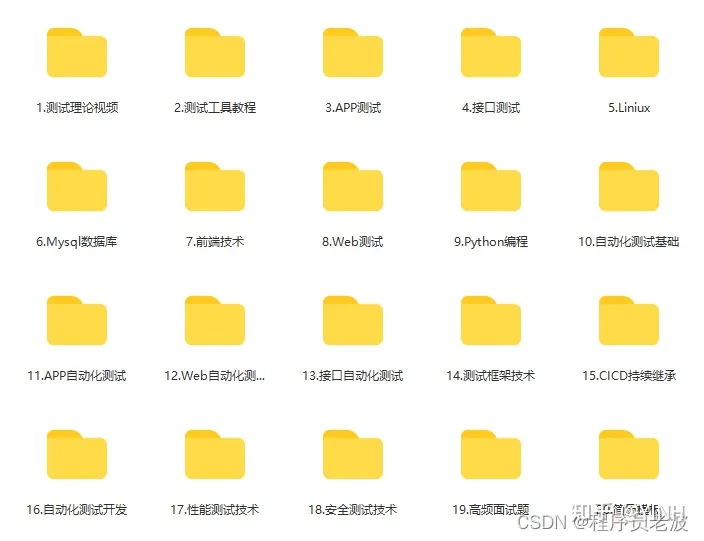
大学四年..就混了毕业证的我,出社会深感无力..辞去工作,从头开始
时间如白驹过隙,一恍就到了2023年,今天最于我来说是一个值得纪念的日子,因为我收获了今年的第一个offer背景18年毕业,二本。大学四年,也就将就混了毕业证和学位证。毕业后,并未想过留在湖南,就回…...

C语言数据结构初阶(6)----链表常见OJ题
CSDN的uu们,大家好!编程能力的提高不仅需要学习新的知识,还需要大量的练习。所以,C语言数据结构初阶的第六讲邀请uu们一起来看看链表的常见oj题目。移除链表元素原题链接:203. 移除链表元素 - 力扣(Leetcod…...

关键字 const
目录 一、符号常量与常变量 二、const的用法 2.1 const常用方法 2.2 const用于指针 2.2.1 p指针所指的对象值不能改变,但是p指针的指向可以改变 2.2.2 常指针p的指向不能改变,但是所指的对象的值可以改变 2.2.3 p所指对象的指向以及对象的值都不可…...
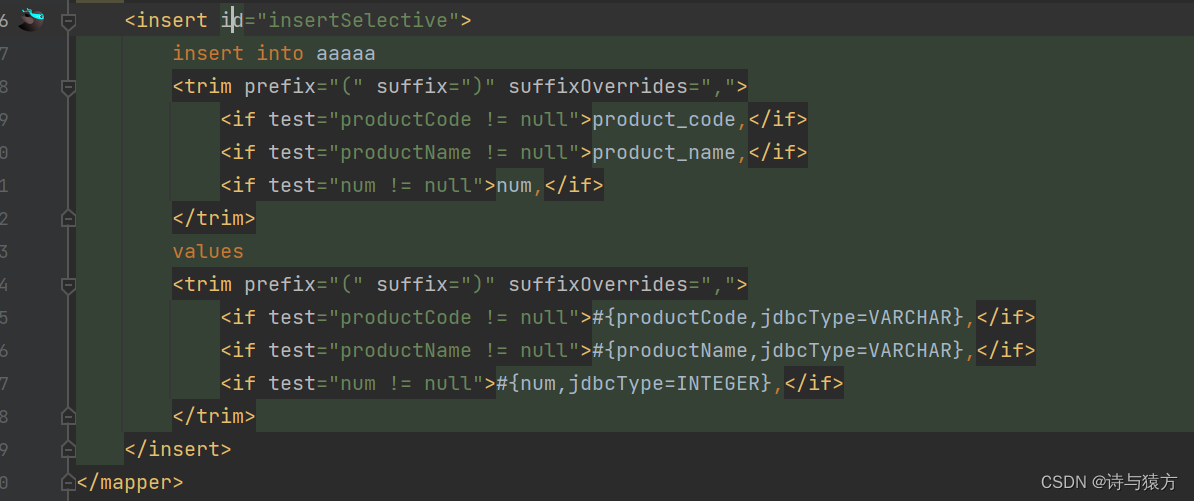
MybatisPlus------MyBatisX插件:快速生成代码以及快速生成CRUD(十二)
MybatisPlus------MyBatisX插件(十二) MyBatisX插件是IDEA插件,如果想要使用它,那么首先需要在IDEA中进行安装。 安装插件 搜索"MyBatisX",点击Install,之后重启IDEA即可。 插件基本用途&…...
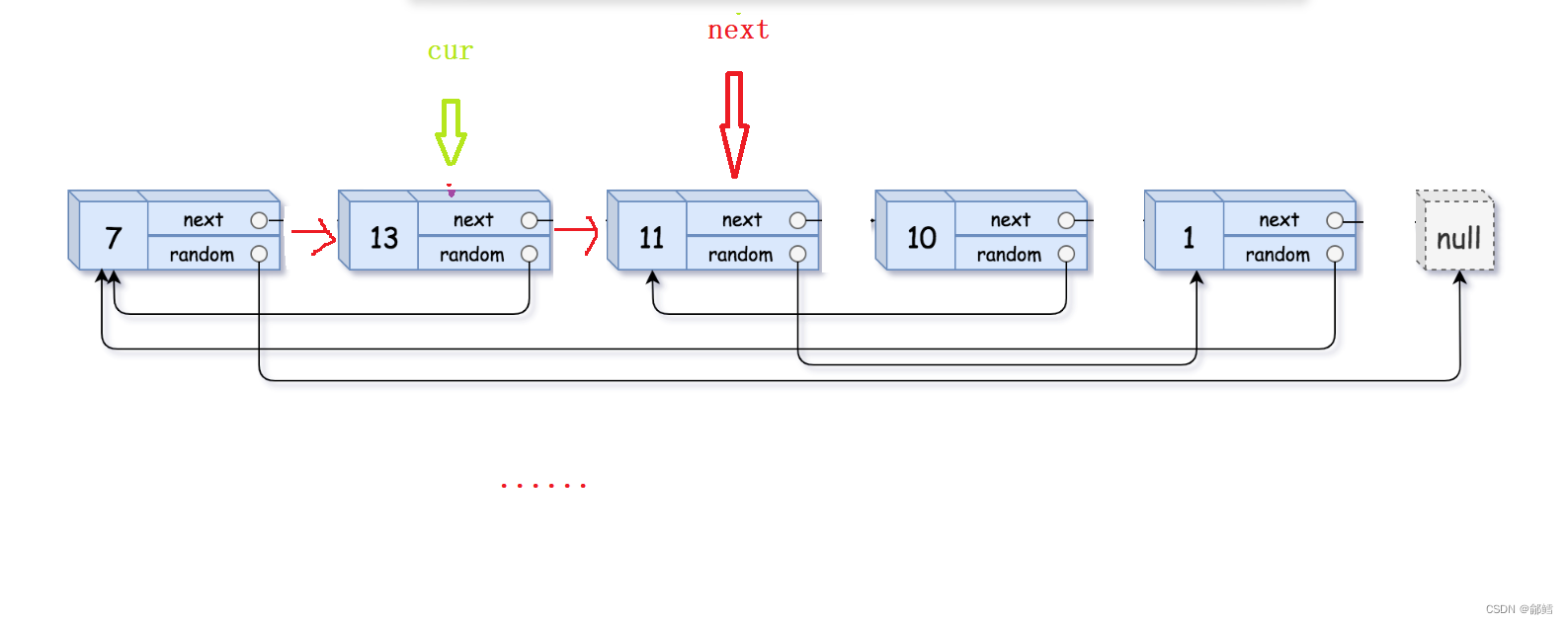
Leetcode138. 复制带随机指针的链表
复制带随机指针的链表 第一步 拷贝节点链接在原节点的后面 第二步拷贝原节点的random , 拷贝节点的 random 在原节点 random 的 next 第三步 将拷贝的节点尾插到一个新链表 ,并且将原链表恢复 从前往后遍历链表 ,将原链表的每个节点进行复制,并l链接到原…...
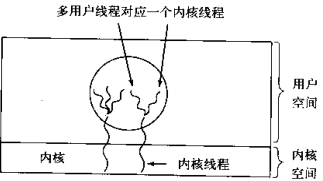
python并发编程多线程
在传统操作系统中,每个进程有一个地址空间,而且默认就有一个控制线程 线程顾名思义,就是一条流水线工作的过程,一条流水线必须属于一个车间,一个车间的工作过程是一个进程 车间负责把资源整合到一起,是一个…...

使用Maven实现Servlet程序
创建Maven项目我们打开idea的新建项目,选中里面Maven即可,如下图:创建完成之后,会看到这样的目录结构其中,main目录存放业务代码,其中的java目录存放的就是java代码,而resources目录存放是程序中依赖的文件,比如:图片,视频等.然后是 test目录,test目录存放的是测试代码.最后一个…...
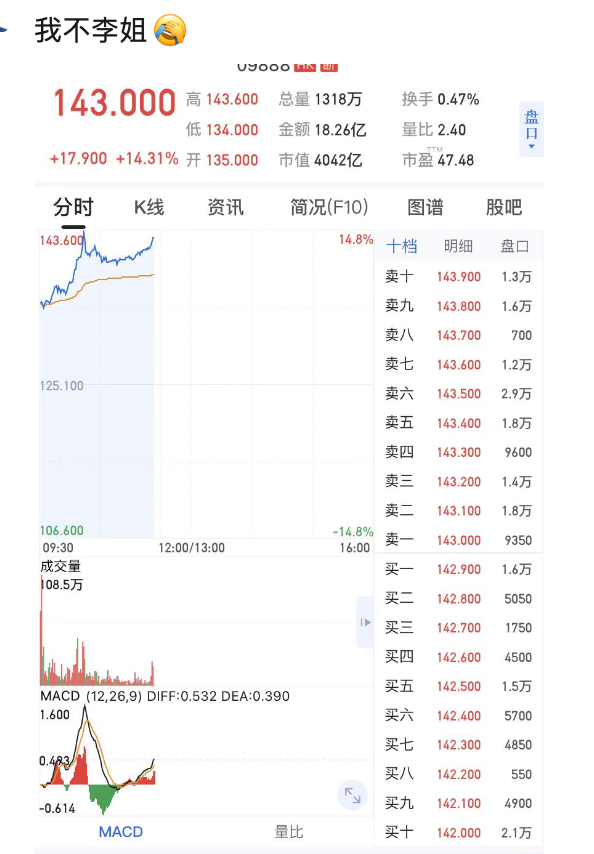
百度的文心一言 ,没有想像中那么差
robin 的演示 我们用 robin 的演示例子来对比一下 文心一言和 ChatGPT 的真实表现(毕竟发布会上是录的)。 注意,我使用的 GPT 版本是 4.0 文学创作 1 三体的作者是哪里人? 文心一言: ChatGPT: 嗯&a…...

文心一言发布的个人看法
文心一言发布宣传视频按照发布会上说的,文心一言并非属于百度赶工抄袭Chat-GPT的作品,而是十几年一直布局AI产业厚积薄发的成果,百度在芯片,机器学习,自然语言处理,知识图谱等方面均有相对深厚的积累。 国…...
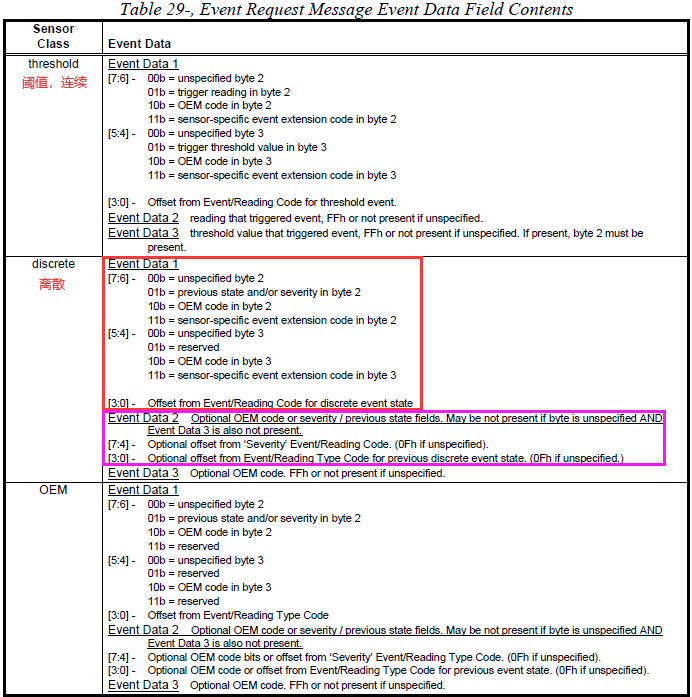
【C5】111
文章目录bmc_wtd:syscpld.c中wd_en和wd_kick节点对应寄存器,crontab,FUNCNAMEAST2500/2600 WDT切换主备:BMC用WDT2作为主备切换的watchdog控制器AC后读取:bmc处于主primary flash(设完后:实际主…...

静态成员,友元函数
🐶博主主页:ᰔᩚ. 一怀明月ꦿ ❤️🔥专栏系列:线性代数,C初学者入门训练,题解C,C的使用文章,「初学」C 🔥座右铭:“不要等到什么都没有了,才下…...

数学分析课程笔记(张平):函数
01 函数 \quad作为数学分析的第一节课,首先深入了解一下函数。 \quad翻看一些教材可以发现,有些教材将“函数”与“映射”区分为两个概念,有些教材(尤其是前苏联时期的一些教材)则将其视为一个概念。实际上,…...

spring事务 只读此文
文章目录一. 事务概述1.1. MySQL 数据库事务1.2 spring的事务支持:1.2.1 编程式事务:1.2.2 声明式事务1.2.3 事务传播行为:1.2.4 事务隔离级别1.2.5 事务的超时时间1.2.6 事务的只读属性1.2.7 事务的回滚策略二. spring事务(注解 Transaction…...
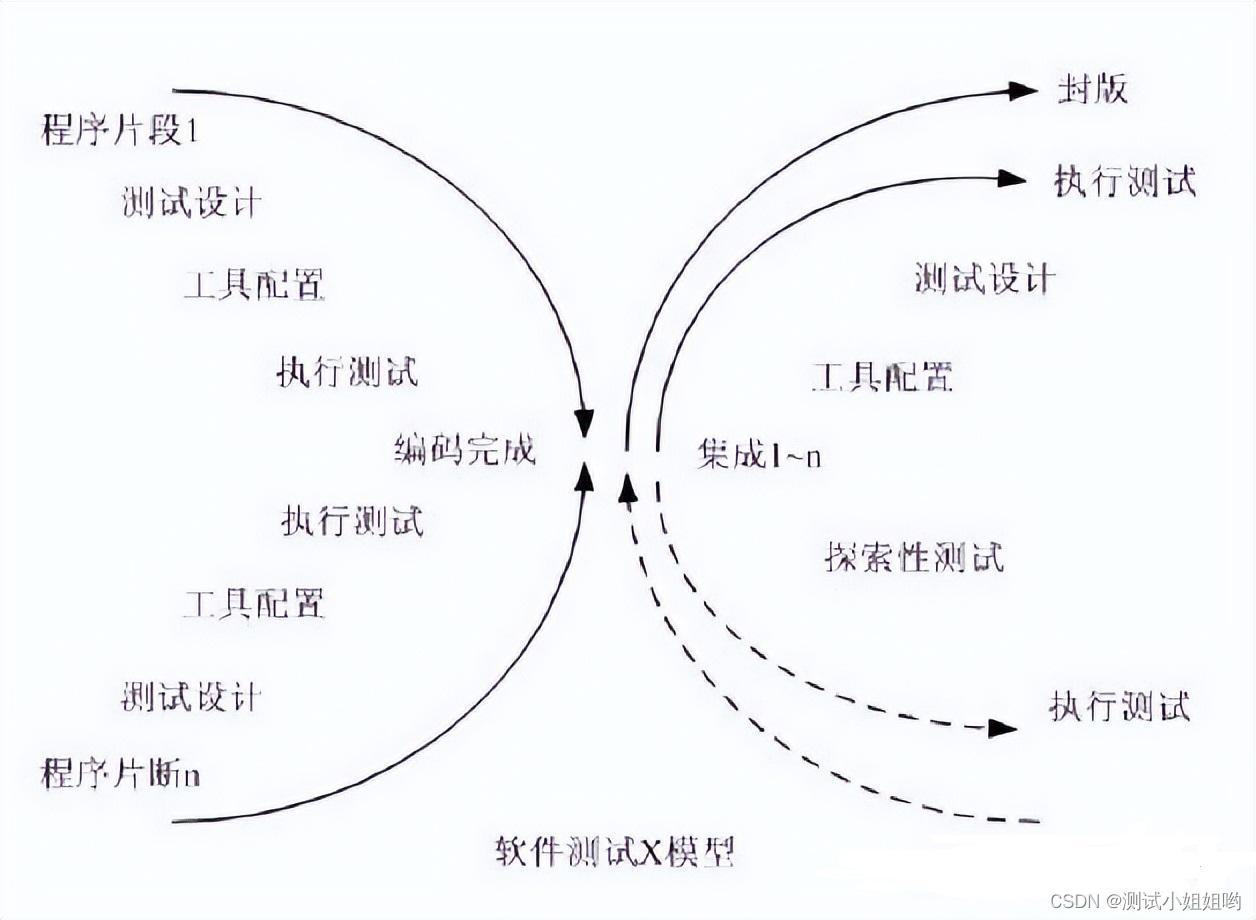
真实的软件测试日常工作是咋样的?
最近很多粉丝问我,小姐姐,现在大环境不景气,传统行业不好做了,想转行软件测试,想知道软件测试日常工作是咋样的?平常的工作内容是什么? 别急,今天跟大家细细说一下一个合格的软件测…...
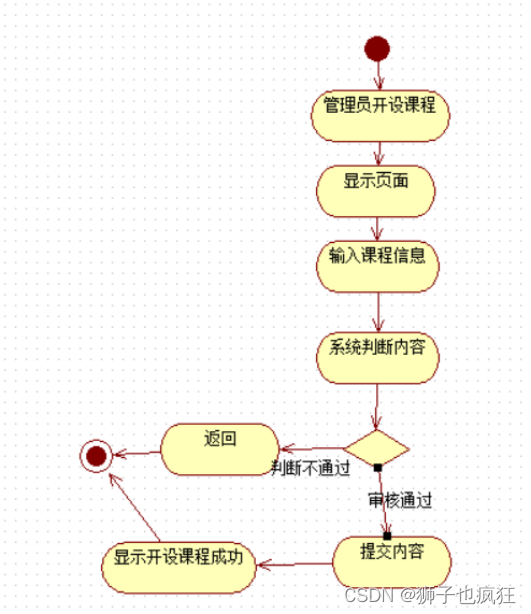
【UML】软件需求说明书
目录🦁 故事的开端一. 🦁 引言1.1编写目的1.2背景1.3定义1.4参考资料二. 🦁 任务概述2.1目标2.2用户的特点2.3假定和约束三. 🦁 需求规定3.1 功能性需求3.1.1系统用例图3.1.2用户登录用例3.1.3学员注册用例3.1.4 学员修改个人信息…...

面试官:html里面哪个元素可以让文字换行展示
在HTML中,可以使用 <br> 元素来强制换行,也可以使用CSS的 word-break 或 white-space 属性来实现自动换行。以下是这些方法的具体说明: 1.使用 <br> 元素 <br> 元素可以在文本中插入一个换行符,使文本从该位置…...

XGBoost和LightGBM时间序列预测对比
XGBoost和LightGBM都是目前非常流行的基于决策树的机器学习模型,它们都有着高效的性能表现,但是在某些情况下,它们也有着不同的特点。 XGBoost和LightGBM简单对比 训练速度 LightGBM相较于xgboost在训练速度方面有明显的优势。这是因为Ligh…...
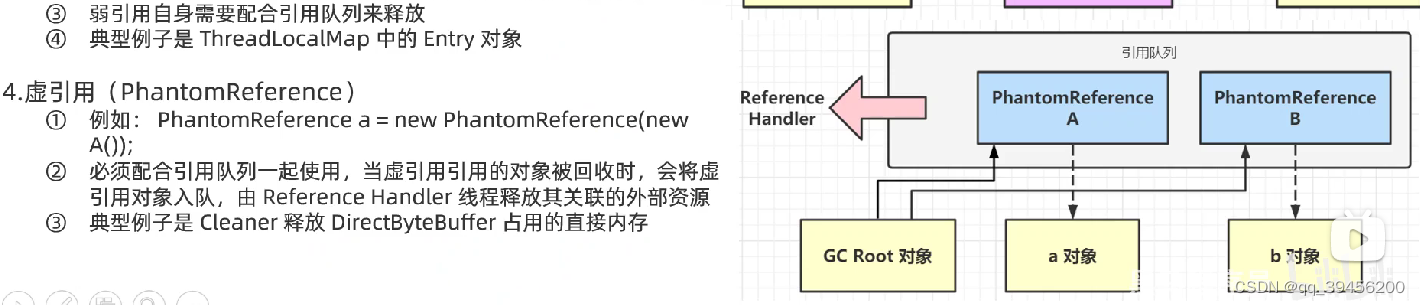
JVM高频面试题
1、项目中什么情况下会内存溢出,怎么解决? (1)误用固定大小线程池导致内存溢出 Excutors.newFixedThreadPool内最大线程数是21亿(2) 误用带缓冲线程池导致内存溢出最大线程数是21亿(3)一次查询太多的数据,导致内存占用…...
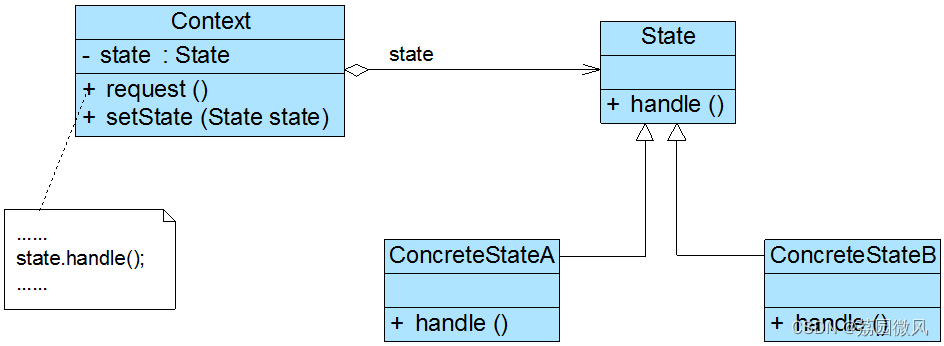
Windows环境下实现设计模式——状态模式(JAVA版)
我是荔园微风,作为一名在IT界整整25年的老兵,今天总结一下Windows环境下如何编程实现状态模式(设计模式)。不知道大家有没有这样的感觉,看了一大堆编程和设计模式的书,却还是很难理解设计模式,无…...

【总结】多个条件排序(pii/struct/bool)
目录 pii struct bool pii 现在小龙同学要吃掉它们,已知他有n颗苹果,并且打算每天吃一个。 但是古人云,早上金苹果,晚上毒苹果。由此可见,早上吃苹果和晚上吃苹果的效果是不一样的。 已知小龙同学在第 i 天早上吃苹果能…...
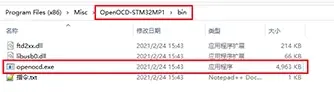
基于stm32mp157 linux开发板ARM裸机开发教程Cortex-A7 开发环境搭建(连载中)
前言:目前针对ARM Cortex-A7裸机开发文档及视频进行了二次升级持续更新中,使其内容更加丰富,讲解更加细致,全文所使用的开发平台均为华清远见FS-MP1A开发板(STM32MP157开发板)针对对FS-MP1A开发板ÿ…...
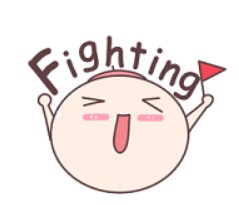
最适合游戏开发的语言是什么?
建议初学者学习主流的开发技术 主流开发技术有大量成熟的教程、很多可以交流的学习者、及时的学习反馈等;技术的内里基本都是相同的,学习主流技术的经验、知识可以更好更快地疏通学习新知识和技术。 因此,对C#或者C二选一进行学习较好。 Un…...
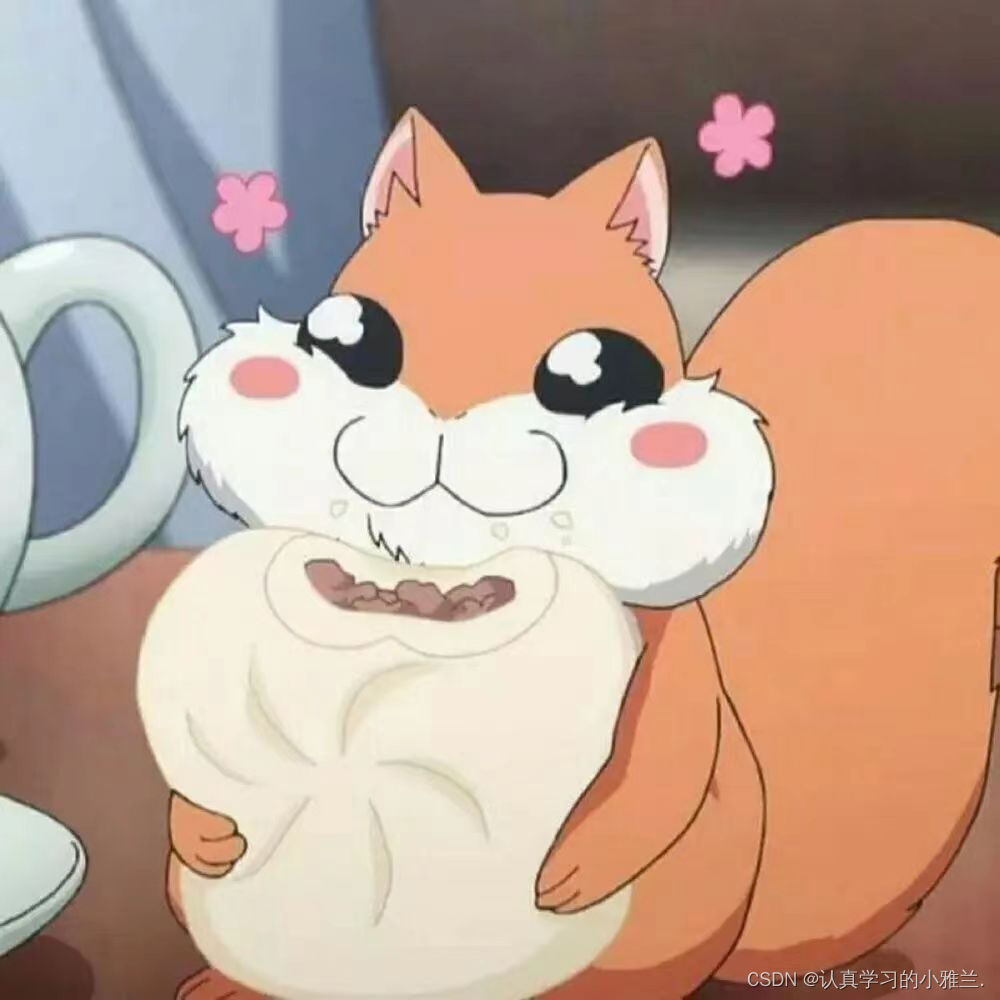
C语言刷题(7)(字符串旋转问题)——“C”
各位CSDN的uu们你们好呀,今天,小雅兰的内容依旧是复习之前的知识点,那么,就是做一道小小的题目啦,下面,让我们进入C语言的世界吧 实现一个函数,可以左旋字符串中的k个字符。 例如: A…...

wordpress role/google全球推广
平板和CRT显示器市场的企业竞争态势 该报告涉及的主要国际市场参与者有Samsung、LG、Philips、AU Optronics、Chi Mei Optoelectronics、Chunghwa Picture Tubes、Hitachi、Panasonic、Royal Philips Electronics、Texas Instruments、Electrograph Technologies、Casio Comput…...

什么网站做全景效果图好/网页设计模板网站免费
Java trim()方法trim() 方法用于删除字符串的头尾空白符。语法publicStringtrim()参数 无返回值删除头尾空白符的字符串。实例public class Test {public static void main(String args[]) {String Str new String(" www.santii.com ");System.out.print(&quo…...

wordpress电商平台插件/站长工具seo综合查询怎么使用的
数据类型 Java虚拟机中,数据类型可以分为两类:基本类型和引用类型。基本类型的变量保存原始值,即:他代表的值就是数值本身;而引用类型的变量保存引用值。“引用值”代表了某个对象的引用,而不是对象本身&am…...

网站建设广告模板/正规拉新推广平台有哪些
问题描述:早上巡检是发现一套RAC的ora.chad一个节点的状态是offline,其他的均正常。crsctl stat res -tora.chadONLINE ONLINE csdb2-bm001 STABLEOFFLINE OFFLINE csdb2-bm002 STABLE解决:ora.chad是Cluster Health Advisor …...
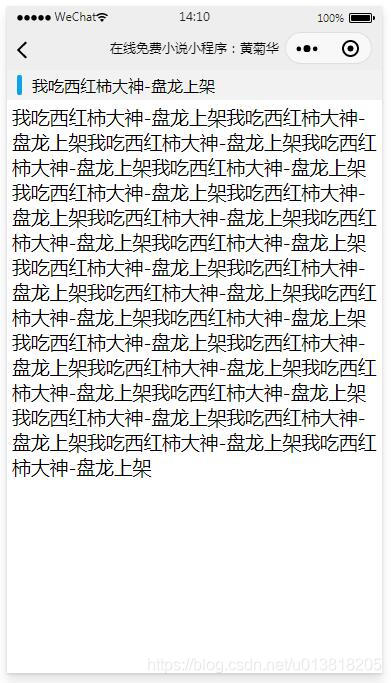
wordpress qq登陆/抖音代运营大概多少钱一个月
在线免费小说平台小程序功能演示...

国外网站制作/产品推广计划怎么写
【内容提要】 明清时期山西洪洞刘氏与当时南方的宗族一样,从明中后期开始组织化。刘氏从默默无闻的家族崛起,成为当地的望族,发展起宗族组织,并从明中叶维持到清末,主要原因在于科举的成功,农商结合的经济以…...